Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DLinq / Dlinq / SqlClient / Common / InheritanceRules.cs / 1305376 / InheritanceRules.cs
using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Linq.Expressions; using System.Reflection; using System.Text; using System.Linq; using System.Data.Linq.Mapping; using System.Data.Linq.Provider; namespace System.Data.Linq.SqlClient { ////// This class defines the rules for inheritance behaviors. The rules: /// /// (1) The same field may not be mapped to different database columns. /// The DistinguishedMemberName and AreSameMember methods describe what 'same' means between two MemberInfos. /// (2) Discriminators held in fixed-length fields in the database don't need /// to be manually padded in inheritance mapping [InheritanceMapping(Code='x')]. /// /// static class InheritanceRules { ////// Creates a name that is the same when the member should be considered 'same' /// for the purposes of the inheritance feature. /// internal static object DistinguishedMemberName(MemberInfo mi) { PropertyInfo pi = mi as PropertyInfo; FieldInfo fi = mi as FieldInfo; if (fi != null) { // Human readable variant: // return "fi:" + mi.Name + ":" + mi.DeclaringType; return new MetaPosition(mi); } else if (pi != null) { MethodInfo meth = null; if (pi.CanRead) { meth = pi.GetGetMethod(); } if (meth == null && pi.CanWrite) { meth = pi.GetSetMethod(); } bool isVirtual = meth != null && meth.IsVirtual; // Human readable variant: // return "pi:" + mi.Name + ":" + (isVirtual ? "virtual" : mi.DeclaringType.ToString()); if (isVirtual) { return mi.Name; } else { return new MetaPosition(mi); } } else { throw Error.ArgumentOutOfRange("mi"); } } ////// Compares two MemberInfos for 'same-ness'. /// internal static bool AreSameMember(MemberInfo mi1, MemberInfo mi2) { return DistinguishedMemberName(mi1).Equals(DistinguishedMemberName(mi2)); } ////// The representation of a inheritance code when mapped to a specific provider type. /// internal static object InheritanceCodeForClientCompare(object rawCode, System.Data.Linq.SqlClient.ProviderType providerType) { // If its a fixed-size string type in the store then pad it with spaces so that // comparing the string on the client agrees with the value returnd on the store. if (providerType.IsFixedSize && rawCode.GetType()==typeof(string)) { string s = (string) rawCode; if (providerType.Size.HasValue && s.Length!=providerType.Size) { s = s.PadRight(providerType.Size.Value).Substring(0,providerType.Size.Value); } return s; } return rawCode; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Linq.Expressions; using System.Reflection; using System.Text; using System.Linq; using System.Data.Linq.Mapping; using System.Data.Linq.Provider; namespace System.Data.Linq.SqlClient { ////// This class defines the rules for inheritance behaviors. The rules: /// /// (1) The same field may not be mapped to different database columns. /// The DistinguishedMemberName and AreSameMember methods describe what 'same' means between two MemberInfos. /// (2) Discriminators held in fixed-length fields in the database don't need /// to be manually padded in inheritance mapping [InheritanceMapping(Code='x')]. /// /// static class InheritanceRules { ////// Creates a name that is the same when the member should be considered 'same' /// for the purposes of the inheritance feature. /// internal static object DistinguishedMemberName(MemberInfo mi) { PropertyInfo pi = mi as PropertyInfo; FieldInfo fi = mi as FieldInfo; if (fi != null) { // Human readable variant: // return "fi:" + mi.Name + ":" + mi.DeclaringType; return new MetaPosition(mi); } else if (pi != null) { MethodInfo meth = null; if (pi.CanRead) { meth = pi.GetGetMethod(); } if (meth == null && pi.CanWrite) { meth = pi.GetSetMethod(); } bool isVirtual = meth != null && meth.IsVirtual; // Human readable variant: // return "pi:" + mi.Name + ":" + (isVirtual ? "virtual" : mi.DeclaringType.ToString()); if (isVirtual) { return mi.Name; } else { return new MetaPosition(mi); } } else { throw Error.ArgumentOutOfRange("mi"); } } ////// Compares two MemberInfos for 'same-ness'. /// internal static bool AreSameMember(MemberInfo mi1, MemberInfo mi2) { return DistinguishedMemberName(mi1).Equals(DistinguishedMemberName(mi2)); } ////// The representation of a inheritance code when mapped to a specific provider type. /// internal static object InheritanceCodeForClientCompare(object rawCode, System.Data.Linq.SqlClient.ProviderType providerType) { // If its a fixed-size string type in the store then pad it with spaces so that // comparing the string on the client agrees with the value returnd on the store. if (providerType.IsFixedSize && rawCode.GetType()==typeof(string)) { string s = (string) rawCode; if (providerType.Size.HasValue && s.Length!=providerType.Size) { s = s.PadRight(providerType.Size.Value).Substring(0,providerType.Size.Value); } return s; } return rawCode; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
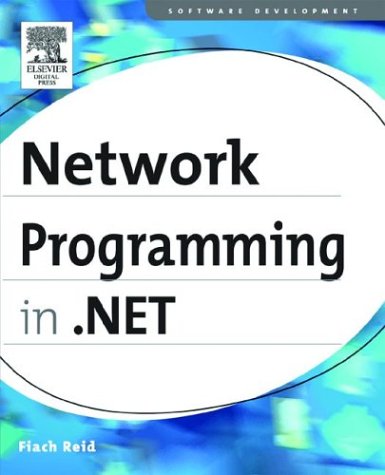
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IndexedWhereQueryOperator.cs
- AddressHeaderCollectionElement.cs
- GregorianCalendar.cs
- CompositeControl.cs
- UserPreferenceChangingEventArgs.cs
- BasePattern.cs
- CommonObjectSecurity.cs
- CodeGotoStatement.cs
- TableChangeProcessor.cs
- ConfigurationStrings.cs
- ReadOnlyCollectionBase.cs
- MappingSource.cs
- SmiContextFactory.cs
- externdll.cs
- EntityConnectionStringBuilder.cs
- FilteredReadOnlyMetadataCollection.cs
- input.cs
- WindowsListBox.cs
- RoutedEvent.cs
- StaticSiteMapProvider.cs
- TypeInfo.cs
- AutomationPattern.cs
- PropertyTabAttribute.cs
- CodeCommentStatementCollection.cs
- CellParaClient.cs
- PageBuildProvider.cs
- InkCanvasFeedbackAdorner.cs
- BrushMappingModeValidation.cs
- AuthenticationSection.cs
- WebDescriptionAttribute.cs
- Normalizer.cs
- FixedSOMGroup.cs
- RegistryConfigurationProvider.cs
- Relationship.cs
- ConfigurationValues.cs
- DataSourceIDConverter.cs
- _HTTPDateParse.cs
- ContextMenuService.cs
- CodeEventReferenceExpression.cs
- FormView.cs
- Transform3DCollection.cs
- ManipulationDeltaEventArgs.cs
- DesignerAttribute.cs
- Codec.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- HostAdapter.cs
- ComponentCodeDomSerializer.cs
- CachedFontFace.cs
- SystemPens.cs
- DataServiceExpressionVisitor.cs
- TransactionTable.cs
- StatusBarItem.cs
- GenericEnumConverter.cs
- SystemWebSectionGroup.cs
- SelectionItemProviderWrapper.cs
- ConfigXmlComment.cs
- Models.cs
- UnsettableComboBox.cs
- XpsViewerException.cs
- GridViewDeletedEventArgs.cs
- ButtonBaseAutomationPeer.cs
- ValidationResult.cs
- PersonalizationDictionary.cs
- AppDomainManager.cs
- TypeConstant.cs
- TrustSection.cs
- TextAnchor.cs
- SafeBitVector32.cs
- Function.cs
- TypeBuilderInstantiation.cs
- DisplayInformation.cs
- DeliveryStrategy.cs
- BrowserCapabilitiesFactoryBase.cs
- InkPresenterAutomationPeer.cs
- LoginName.cs
- UrlPath.cs
- XPathSelectionIterator.cs
- DelegateTypeInfo.cs
- PropertyManager.cs
- WebServicesSection.cs
- Control.cs
- WrapperEqualityComparer.cs
- QilList.cs
- StorageAssociationTypeMapping.cs
- EncryptedPackage.cs
- BitmapDecoder.cs
- Viewport3DAutomationPeer.cs
- AuthenticationException.cs
- AbsoluteQuery.cs
- ValueType.cs
- DeadCharTextComposition.cs
- CommunicationException.cs
- RootBuilder.cs
- AssemblyInfo.cs
- ObjectStateFormatter.cs
- WindowsMenu.cs
- ToolStripDropDownClosingEventArgs.cs
- XmlLangPropertyAttribute.cs
- SqlCacheDependency.cs
- RadialGradientBrush.cs