Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Security / Principal / GenericPrincipal.cs / 1 / GenericPrincipal.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // GenericPrincipal.cs // namespace System.Security.Principal { using System.Runtime.Remoting; using System; using System.Security.Util; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class GenericPrincipal : IPrincipal { private IIdentity m_identity; private string[] m_roles; public GenericPrincipal (IIdentity identity, string[] roles) { if (identity == null) throw new ArgumentNullException("identity"); m_identity = identity; if (roles != null) { m_roles = new string[roles.Length]; for (int i = 0; i < roles.Length; ++i) { m_roles[i] = roles[i]; } } else { m_roles = null; } } public virtual IIdentity Identity { get { return m_identity; } } public virtual bool IsInRole (string role) { if (role == null || m_roles == null) return false; for (int i = 0; i < m_roles.Length; ++i) { if (m_roles[i] != null && String.Compare(m_roles[i], role, StringComparison.OrdinalIgnoreCase) == 0) return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // // GenericPrincipal.cs // namespace System.Security.Principal { using System.Runtime.Remoting; using System; using System.Security.Util; using System.Globalization; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class GenericPrincipal : IPrincipal { private IIdentity m_identity; private string[] m_roles; public GenericPrincipal (IIdentity identity, string[] roles) { if (identity == null) throw new ArgumentNullException("identity"); m_identity = identity; if (roles != null) { m_roles = new string[roles.Length]; for (int i = 0; i < roles.Length; ++i) { m_roles[i] = roles[i]; } } else { m_roles = null; } } public virtual IIdentity Identity { get { return m_identity; } } public virtual bool IsInRole (string role) { if (role == null || m_roles == null) return false; for (int i = 0; i < m_roles.Length; ++i) { if (m_roles[i] != null && String.Compare(m_roles[i], role, StringComparison.OrdinalIgnoreCase) == 0) return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
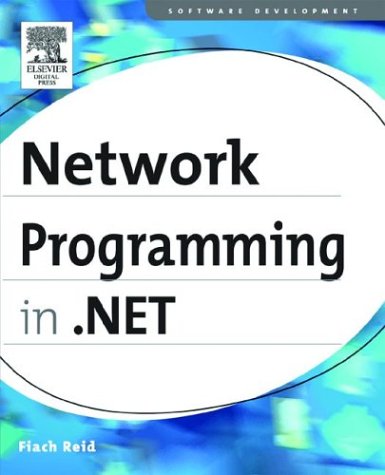
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Semaphore.cs
- InvalidOperationException.cs
- SemanticResultValue.cs
- CompilerErrorCollection.cs
- MDIWindowDialog.cs
- WebPartConnectVerb.cs
- LastQueryOperator.cs
- DomainConstraint.cs
- DesignerAttribute.cs
- DbProviderFactories.cs
- GridItemPattern.cs
- TextRange.cs
- Material.cs
- TableLayoutPanelCellPosition.cs
- TableHeaderCell.cs
- VisualBrush.cs
- SessionSwitchEventArgs.cs
- ChildDocumentBlock.cs
- SchemaAttDef.cs
- Pair.cs
- SQLDecimal.cs
- TagNameToTypeMapper.cs
- CompilerCollection.cs
- BlurEffect.cs
- TextRenderer.cs
- HtmlTableRowCollection.cs
- SqlGenericUtil.cs
- adornercollection.cs
- SecurityManager.cs
- EntityAdapter.cs
- SoapSchemaExporter.cs
- OdbcUtils.cs
- SmtpFailedRecipientsException.cs
- IncrementalReadDecoders.cs
- BookmarkCallbackWrapper.cs
- RadioButtonFlatAdapter.cs
- ControlAdapter.cs
- ErasingStroke.cs
- HtmlHistory.cs
- RuntimeEnvironment.cs
- VBIdentifierNameEditor.cs
- ManipulationLogic.cs
- HitTestFilterBehavior.cs
- ResourceDisplayNameAttribute.cs
- EqualityArray.cs
- MultiView.cs
- XsdDuration.cs
- PipelineModuleStepContainer.cs
- IndexingContentUnit.cs
- XmlCharType.cs
- TimeSpanHelper.cs
- ServiceMemoryGates.cs
- BreakSafeBase.cs
- DateTimeFormatInfoScanner.cs
- OleDbRowUpdatedEvent.cs
- ListViewPagedDataSource.cs
- TimerElapsedEvenArgs.cs
- RemotingSurrogateSelector.cs
- JsonEnumDataContract.cs
- IsolatedStorageFile.cs
- ContentFilePart.cs
- TransformGroup.cs
- MethodBuilder.cs
- ComponentConverter.cs
- XmlCharCheckingReader.cs
- SocketException.cs
- ChildChangedEventArgs.cs
- WebPartTransformer.cs
- AsyncResult.cs
- WebResourceAttribute.cs
- Activity.cs
- ExtractCollection.cs
- PropertyDescriptor.cs
- PublishLicense.cs
- PagerSettings.cs
- LassoSelectionBehavior.cs
- X500Name.cs
- ColorKeyFrameCollection.cs
- SiteMapDataSourceView.cs
- VisualStyleRenderer.cs
- FileSystemWatcher.cs
- XmlCustomFormatter.cs
- WorkflowOwnershipException.cs
- DeclaredTypeValidator.cs
- TableLayoutSettings.cs
- COM2Enum.cs
- CacheMode.cs
- State.cs
- DynamicField.cs
- MailDefinition.cs
- ToolStripItemEventArgs.cs
- printdlgexmarshaler.cs
- RemotingAttributes.cs
- ToolboxItemLoader.cs
- PropertyGridCommands.cs
- TriggerBase.cs
- ValuePattern.cs
- CircleHotSpot.cs
- Visual3DCollection.cs
- SerializationException.cs