Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2Enum.cs / 1 / COM2Enum.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.Globalization; ////// /// This class mimics a clr enum that we can create at runtime. /// It associates an array of names with an array of values and converts /// between them. /// /// A note here: we compare string values when looking for the value of an item. /// Typically these aren't large lists and the perf is worth it. The reason stems /// from IPerPropertyBrowsing, which supplies a list of names and a list of /// variants to mimic enum functionality. If the actual property value is a DWORD, /// which translates to VT_UI4, and they specify their values as VT_I4 (which is a common /// mistake), they won't compare properly and values can't be updated. /// By comparing strings, we avoid this problem and add flexiblity to the system. /// internal class Com2Enum { ////// /// Our array of value string names /// private string[] names; ////// /// Our values /// private object[] values; ////// /// Our cached array of value.ToString()'s /// private string[] stringValues; ////// /// Should we allow values besides what's in the listbox? /// private bool allowUnknownValues; ////// /// Our one and only ctor /// public Com2Enum(string[] names, object[] values, bool allowUnknownValues) { this.allowUnknownValues = allowUnknownValues; // these have to be null and the same length if (names == null || values == null || names.Length != values.Length) { throw new ArgumentException(SR.GetString(SR.COM2NamesAndValuesNotEqual)); } PopulateArrays(names, values); } ////// /// Can this enum be values other than the strict enum? /// public bool IsStrictEnum { get { return !this.allowUnknownValues; } } ////// /// Retrieve a copy of the value array /// public virtual object[] Values { get { return(object[])this.values.Clone(); } } ////// /// Retrieve a copy of the nme array. /// public virtual string[] Names { get { return(string[])this.names.Clone(); } } ////// /// Associate a string to the appropriate value. /// public virtual object FromString(string s) { int bestMatch = -1; for (int i = 0; i < stringValues.Length; i++) { if (String.Compare(names[i], s, true, CultureInfo.InvariantCulture) == 0 || String.Compare(stringValues[i], s, true, CultureInfo.InvariantCulture) == 0) { return values[i]; } if (bestMatch == -1 && 0 == String.Compare(names[i], s, true, CultureInfo.InvariantCulture)) { bestMatch = i; } } if (bestMatch != -1) { return values[bestMatch]; } return allowUnknownValues ? s : null; } protected virtual void PopulateArrays(string[] names, object[] values) { // setup our values...since we have to walk through // them anyway to do the ToString, we just copy them here. this.names = new string[names.Length]; this.stringValues = new string[names.Length]; this.values = new object[names.Length]; for (int i = 0; i < names.Length; i++) { //Debug.WriteLine(names[i] + ": item " + i.ToString() + ",type=" + values[i].GetType().Name + ", value=" + values[i].ToString()); this.names[i] = names[i]; this.values[i] = values[i]; if (values[i] != null) { this.stringValues[i] = values[i].ToString(); } } } ////// /// Retrieves the string name of a given value. /// public virtual string ToString(object v) { if (v != null) { // in case this is a real enum...try to convert it. // if (values.Length > 0 && v.GetType() != values[0].GetType()) { try { v = Convert.ChangeType(v, values[0].GetType(), CultureInfo.InvariantCulture); } catch{ } } // we have to do this do compensate for small discrpencies // in a lot of objects in COM2 (DWORD -> VT_IU4, value we get is VT_I4, which // convert to Int32, UInt32 respectively string strVal = v.ToString(); for (int i = 0; i < values.Length; i++) { if (String.Compare(stringValues[i], strVal, true, CultureInfo.InvariantCulture) == 0) { return names[i]; } } if (allowUnknownValues) { return strVal; } } return ""; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.ComponentModel; using System.Diagnostics; using System; using System.Collections; using Microsoft.Win32; using System.Globalization; ////// /// This class mimics a clr enum that we can create at runtime. /// It associates an array of names with an array of values and converts /// between them. /// /// A note here: we compare string values when looking for the value of an item. /// Typically these aren't large lists and the perf is worth it. The reason stems /// from IPerPropertyBrowsing, which supplies a list of names and a list of /// variants to mimic enum functionality. If the actual property value is a DWORD, /// which translates to VT_UI4, and they specify their values as VT_I4 (which is a common /// mistake), they won't compare properly and values can't be updated. /// By comparing strings, we avoid this problem and add flexiblity to the system. /// internal class Com2Enum { ////// /// Our array of value string names /// private string[] names; ////// /// Our values /// private object[] values; ////// /// Our cached array of value.ToString()'s /// private string[] stringValues; ////// /// Should we allow values besides what's in the listbox? /// private bool allowUnknownValues; ////// /// Our one and only ctor /// public Com2Enum(string[] names, object[] values, bool allowUnknownValues) { this.allowUnknownValues = allowUnknownValues; // these have to be null and the same length if (names == null || values == null || names.Length != values.Length) { throw new ArgumentException(SR.GetString(SR.COM2NamesAndValuesNotEqual)); } PopulateArrays(names, values); } ////// /// Can this enum be values other than the strict enum? /// public bool IsStrictEnum { get { return !this.allowUnknownValues; } } ////// /// Retrieve a copy of the value array /// public virtual object[] Values { get { return(object[])this.values.Clone(); } } ////// /// Retrieve a copy of the nme array. /// public virtual string[] Names { get { return(string[])this.names.Clone(); } } ////// /// Associate a string to the appropriate value. /// public virtual object FromString(string s) { int bestMatch = -1; for (int i = 0; i < stringValues.Length; i++) { if (String.Compare(names[i], s, true, CultureInfo.InvariantCulture) == 0 || String.Compare(stringValues[i], s, true, CultureInfo.InvariantCulture) == 0) { return values[i]; } if (bestMatch == -1 && 0 == String.Compare(names[i], s, true, CultureInfo.InvariantCulture)) { bestMatch = i; } } if (bestMatch != -1) { return values[bestMatch]; } return allowUnknownValues ? s : null; } protected virtual void PopulateArrays(string[] names, object[] values) { // setup our values...since we have to walk through // them anyway to do the ToString, we just copy them here. this.names = new string[names.Length]; this.stringValues = new string[names.Length]; this.values = new object[names.Length]; for (int i = 0; i < names.Length; i++) { //Debug.WriteLine(names[i] + ": item " + i.ToString() + ",type=" + values[i].GetType().Name + ", value=" + values[i].ToString()); this.names[i] = names[i]; this.values[i] = values[i]; if (values[i] != null) { this.stringValues[i] = values[i].ToString(); } } } ////// /// Retrieves the string name of a given value. /// public virtual string ToString(object v) { if (v != null) { // in case this is a real enum...try to convert it. // if (values.Length > 0 && v.GetType() != values[0].GetType()) { try { v = Convert.ChangeType(v, values[0].GetType(), CultureInfo.InvariantCulture); } catch{ } } // we have to do this do compensate for small discrpencies // in a lot of objects in COM2 (DWORD -> VT_IU4, value we get is VT_I4, which // convert to Int32, UInt32 respectively string strVal = v.ToString(); for (int i = 0; i < values.Length; i++) { if (String.Compare(stringValues[i], strVal, true, CultureInfo.InvariantCulture) == 0) { return names[i]; } } if (allowUnknownValues) { return strVal; } } return ""; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
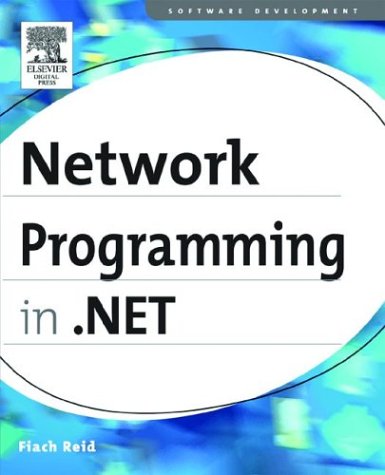
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableCell.cs
- OutKeywords.cs
- Converter.cs
- HttpPostedFile.cs
- RoutedUICommand.cs
- PrintDialog.cs
- DataTable.cs
- BuildManagerHost.cs
- ApplicationSecurityInfo.cs
- ImageListDesigner.cs
- ProjectedSlot.cs
- PackageProperties.cs
- HuffCodec.cs
- arc.cs
- CLRBindingWorker.cs
- ExpandCollapseProviderWrapper.cs
- TcpServerChannel.cs
- HttpRawResponse.cs
- ScrollBarAutomationPeer.cs
- InputLanguageEventArgs.cs
- TextDecorationLocationValidation.cs
- SchemaConstraints.cs
- RotateTransform3D.cs
- Ref.cs
- DrawingContextDrawingContextWalker.cs
- BypassElementCollection.cs
- HMACRIPEMD160.cs
- JumpList.cs
- GridView.cs
- DateTimeValueSerializer.cs
- AssertUtility.cs
- BuildDependencySet.cs
- ColumnHeaderConverter.cs
- PageParserFilter.cs
- ConfigurationFileMap.cs
- FontDialog.cs
- EditingCommands.cs
- UrlPropertyAttribute.cs
- TempEnvironment.cs
- ObjectDataSourceStatusEventArgs.cs
- CategoryAttribute.cs
- PointLight.cs
- Application.cs
- TransactedReceiveScope.cs
- PrimitiveXmlSerializers.cs
- CacheAxisQuery.cs
- GridSplitter.cs
- relpropertyhelper.cs
- ReadWriteObjectLock.cs
- ping.cs
- _SecureChannel.cs
- MenuItemCollectionEditor.cs
- ErrorWrapper.cs
- PageScaling.cs
- JoinCqlBlock.cs
- ValidateNames.cs
- NavigationExpr.cs
- GridView.cs
- JpegBitmapDecoder.cs
- WebEventCodes.cs
- ContextQuery.cs
- TrackingProvider.cs
- UTF8Encoding.cs
- TypeSystemHelpers.cs
- TextTreeUndo.cs
- TextBoxBase.cs
- GroupItem.cs
- AnonymousIdentificationModule.cs
- CompositionAdorner.cs
- AutomationEvent.cs
- DES.cs
- OleCmdHelper.cs
- SecurityCredentialsManager.cs
- TextEffectResolver.cs
- OpacityConverter.cs
- EventSetter.cs
- Vector3D.cs
- SqlFunctionAttribute.cs
- Size.cs
- ElementFactory.cs
- RichTextBoxContextMenu.cs
- Calendar.cs
- TrustSection.cs
- TreeView.cs
- AutomationPropertyChangedEventArgs.cs
- RecognizedAudio.cs
- Point3DKeyFrameCollection.cs
- ReadOnlyNameValueCollection.cs
- PageParserFilter.cs
- IndexOutOfRangeException.cs
- HashHelper.cs
- ImageFormat.cs
- UriScheme.cs
- WindowsGraphicsWrapper.cs
- SqlFlattener.cs
- InlineCollection.cs
- WebException.cs
- DataTemplate.cs
- EmbeddedMailObjectsCollection.cs
- StandardOleMarshalObject.cs