Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / xsp / System / Web / Extensions / Handlers / ScriptModule.cs / 2 / ScriptModule.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Handlers { using System; using System.Collections.Generic; using System.Globalization; using System.Net; using System.Security.Permissions; using System.Web; using System.Web.ApplicationServices; using System.Web.UI; using System.Web.Resources; using System.Web.Script.Services; using System.Web.Security; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal) ] public class ScriptModule : IHttpModule { private static Type _authenticationServiceType = typeof(System.Web.Security.AuthenticationService); // disambiguation required private static bool ShouldSkipAuthorization(HttpContext context) { if (context == null || context.Request == null) { return false; } string path = context.Request.FilePath; if (ScriptResourceHandler.IsScriptResourceRequest(path)) { return true; } // if auth service is disabled, dont bother checking. // (NOTE: if a custom webservice is used, it will be up to them to enable anon access to it) // if it isn't a rest request dont bother checking. if(!ApplicationServiceHelper.AuthenticationServiceEnabled || !RestHandlerFactory.IsRestRequest(context)) { return false; } if(context.SkipAuthorization) { return true; } // it may be a rest request to a webservice. It must end in axd if it is an app service. if((path == null) || !path.EndsWith(".axd", StringComparison.OrdinalIgnoreCase)) { return false; } // WebServiceData caches the object in cache, so this should be a quick lookup. // If it hasnt been cached yet, this will cause it to be cached, so later in the request // it will be a cache-hit anyway. WebServiceData wsd = WebServiceData.GetWebServiceData(context, path, false, false); if((wsd != null) && (_authenticationServiceType == wsd.TypeData.Type)) { return true; } return false; } protected virtual void Dispose() { } private void AuthenticateRequestHandler(object sender, EventArgs e) { // flags the request with SkipAuthorization if it is a request for // the script Authentication webservice. HttpApplication app = (HttpApplication)sender; if (app != null && ShouldSkipAuthorization(app.Context)) { app.Context.SkipAuthorization = true; } } private void EndRequestHandler(object sender, EventArgs e){ // DevDiv 100198: Send error response from EndRequest so Page and Application error handlers still fire on async posts // DevDiv 118737: Call Response.Clear as well as Response.ClearHeaders to force status code reset in integrated mode and // to ensure there are no errant headers such as a caching policy. Do not call Response.End or app.CompleteRequest as they // are pointless from the EndRequest event. HttpApplication app = (HttpApplication)sender; HttpContext context = app.Context; object o = context.Items[PageRequestManager.AsyncPostBackErrorKey]; if ((o != null) && ((bool)o == true)) { context.ClearError(); context.Response.ClearHeaders(); context.Response.Clear(); context.Response.Cache.SetCacheability(HttpCacheability.NoCache); context.Response.ContentType = "text/plain"; string errorMessage = (string)context.Items[PageRequestManager.AsyncPostBackErrorMessageKey]; o = context.Items[PageRequestManager.AsyncPostBackErrorHttpCodeKey]; // o should definitely be an int, but user code could overwrite it int httpCode = (o is int) ? (int)o : 500; PageRequestManager.EncodeString(context.Response.Output, PageRequestManager.ErrorToken, httpCode.ToString(CultureInfo.InvariantCulture), errorMessage); } } protected virtual void Init(HttpApplication context) { context.PreSendRequestHeaders += new EventHandler(PreSendRequestHeadersHandler); context.PostAcquireRequestState += new EventHandler(OnPostAcquireRequestState); context.AuthenticateRequest += new EventHandler(AuthenticateRequestHandler); // DevDiv 100198: Send error response from EndRequest so Page and Application error handlers still fire on async posts context.EndRequest += new EventHandler(EndRequestHandler); } private void OnPostAcquireRequestState(object sender, EventArgs eventArgs) { HttpApplication app = (HttpApplication)sender; HttpRequest request = app.Context.Request; if (app.Context.Handler is Page && RestHandlerFactory.IsRestMethodCall(request)) { // Get the data about the web service being invoked WebServiceData webServiceData = WebServiceData.GetWebServiceData(HttpContext.Current, request.FilePath, false, true); // Get the method name string methodName = request.PathInfo.Substring(1); // Get the data about the specific method being called WebServiceMethodData methodData = webServiceData.GetMethodData(methodName); RestHandler.ExecuteWebServiceCall(HttpContext.Current, methodData); // Skip the rest of the page lifecycle app.CompleteRequest(); } } private void PreSendRequestHeadersHandler(object sender, EventArgs args) { HttpApplication application = (HttpApplication)sender; HttpResponse response = application.Response; if (response.StatusCode == 302) { // Is in async postback, get status code and check for 302 if (PageRequestManager.IsAsyncPostBackRequest(new HttpRequestWrapper(application.Request))) { // Save the redirect location and other data before we clear it string redirectLocation = response.RedirectLocation; Listcookies = new List (response.Cookies.Count); for (int i = 0; i < response.Cookies.Count; i++) { cookies.Add(response.Cookies[i]); } // Clear the entire response and send a custom response that the client script can process response.ClearContent(); response.ClearHeaders(); for (int i = 0; i < cookies.Count; i++) { response.AppendCookie(cookies[i]); } response.Cache.SetCacheability(HttpCacheability.NoCache); response.ContentType = "text/plain"; PageRequestManager.EncodeString(response.Output, PageRequestManager.PageRedirectToken, String.Empty, redirectLocation); } else if (RestHandlerFactory.IsRestRequest(application.Context)) { // We need to special case webservice redirects, as we want them to fail (always are auth failures) RestHandler.WriteExceptionJsonString(application.Context, new InvalidOperationException(AtlasWeb.WebService_RedirectError), (int)HttpStatusCode.Unauthorized); } } } #region IHttpModule Members void IHttpModule.Dispose() { Dispose(); } void IHttpModule.Init(HttpApplication context) { Init(context); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Handlers { using System; using System.Collections.Generic; using System.Globalization; using System.Net; using System.Security.Permissions; using System.Web; using System.Web.ApplicationServices; using System.Web.UI; using System.Web.Resources; using System.Web.Script.Services; using System.Web.Security; [ AspNetHostingPermission(SecurityAction.LinkDemand, Level = AspNetHostingPermissionLevel.Minimal), AspNetHostingPermission(SecurityAction.InheritanceDemand, Level = AspNetHostingPermissionLevel.Minimal) ] public class ScriptModule : IHttpModule { private static Type _authenticationServiceType = typeof(System.Web.Security.AuthenticationService); // disambiguation required private static bool ShouldSkipAuthorization(HttpContext context) { if (context == null || context.Request == null) { return false; } string path = context.Request.FilePath; if (ScriptResourceHandler.IsScriptResourceRequest(path)) { return true; } // if auth service is disabled, dont bother checking. // (NOTE: if a custom webservice is used, it will be up to them to enable anon access to it) // if it isn't a rest request dont bother checking. if(!ApplicationServiceHelper.AuthenticationServiceEnabled || !RestHandlerFactory.IsRestRequest(context)) { return false; } if(context.SkipAuthorization) { return true; } // it may be a rest request to a webservice. It must end in axd if it is an app service. if((path == null) || !path.EndsWith(".axd", StringComparison.OrdinalIgnoreCase)) { return false; } // WebServiceData caches the object in cache, so this should be a quick lookup. // If it hasnt been cached yet, this will cause it to be cached, so later in the request // it will be a cache-hit anyway. WebServiceData wsd = WebServiceData.GetWebServiceData(context, path, false, false); if((wsd != null) && (_authenticationServiceType == wsd.TypeData.Type)) { return true; } return false; } protected virtual void Dispose() { } private void AuthenticateRequestHandler(object sender, EventArgs e) { // flags the request with SkipAuthorization if it is a request for // the script Authentication webservice. HttpApplication app = (HttpApplication)sender; if (app != null && ShouldSkipAuthorization(app.Context)) { app.Context.SkipAuthorization = true; } } private void EndRequestHandler(object sender, EventArgs e){ // DevDiv 100198: Send error response from EndRequest so Page and Application error handlers still fire on async posts // DevDiv 118737: Call Response.Clear as well as Response.ClearHeaders to force status code reset in integrated mode and // to ensure there are no errant headers such as a caching policy. Do not call Response.End or app.CompleteRequest as they // are pointless from the EndRequest event. HttpApplication app = (HttpApplication)sender; HttpContext context = app.Context; object o = context.Items[PageRequestManager.AsyncPostBackErrorKey]; if ((o != null) && ((bool)o == true)) { context.ClearError(); context.Response.ClearHeaders(); context.Response.Clear(); context.Response.Cache.SetCacheability(HttpCacheability.NoCache); context.Response.ContentType = "text/plain"; string errorMessage = (string)context.Items[PageRequestManager.AsyncPostBackErrorMessageKey]; o = context.Items[PageRequestManager.AsyncPostBackErrorHttpCodeKey]; // o should definitely be an int, but user code could overwrite it int httpCode = (o is int) ? (int)o : 500; PageRequestManager.EncodeString(context.Response.Output, PageRequestManager.ErrorToken, httpCode.ToString(CultureInfo.InvariantCulture), errorMessage); } } protected virtual void Init(HttpApplication context) { context.PreSendRequestHeaders += new EventHandler(PreSendRequestHeadersHandler); context.PostAcquireRequestState += new EventHandler(OnPostAcquireRequestState); context.AuthenticateRequest += new EventHandler(AuthenticateRequestHandler); // DevDiv 100198: Send error response from EndRequest so Page and Application error handlers still fire on async posts context.EndRequest += new EventHandler(EndRequestHandler); } private void OnPostAcquireRequestState(object sender, EventArgs eventArgs) { HttpApplication app = (HttpApplication)sender; HttpRequest request = app.Context.Request; if (app.Context.Handler is Page && RestHandlerFactory.IsRestMethodCall(request)) { // Get the data about the web service being invoked WebServiceData webServiceData = WebServiceData.GetWebServiceData(HttpContext.Current, request.FilePath, false, true); // Get the method name string methodName = request.PathInfo.Substring(1); // Get the data about the specific method being called WebServiceMethodData methodData = webServiceData.GetMethodData(methodName); RestHandler.ExecuteWebServiceCall(HttpContext.Current, methodData); // Skip the rest of the page lifecycle app.CompleteRequest(); } } private void PreSendRequestHeadersHandler(object sender, EventArgs args) { HttpApplication application = (HttpApplication)sender; HttpResponse response = application.Response; if (response.StatusCode == 302) { // Is in async postback, get status code and check for 302 if (PageRequestManager.IsAsyncPostBackRequest(new HttpRequestWrapper(application.Request))) { // Save the redirect location and other data before we clear it string redirectLocation = response.RedirectLocation; Listcookies = new List (response.Cookies.Count); for (int i = 0; i < response.Cookies.Count; i++) { cookies.Add(response.Cookies[i]); } // Clear the entire response and send a custom response that the client script can process response.ClearContent(); response.ClearHeaders(); for (int i = 0; i < cookies.Count; i++) { response.AppendCookie(cookies[i]); } response.Cache.SetCacheability(HttpCacheability.NoCache); response.ContentType = "text/plain"; PageRequestManager.EncodeString(response.Output, PageRequestManager.PageRedirectToken, String.Empty, redirectLocation); } else if (RestHandlerFactory.IsRestRequest(application.Context)) { // We need to special case webservice redirects, as we want them to fail (always are auth failures) RestHandler.WriteExceptionJsonString(application.Context, new InvalidOperationException(AtlasWeb.WebService_RedirectError), (int)HttpStatusCode.Unauthorized); } } } #region IHttpModule Members void IHttpModule.Dispose() { Dispose(); } void IHttpModule.Init(HttpApplication context) { Init(context); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
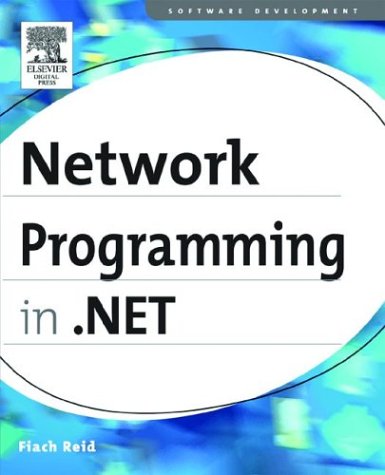
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlTextReaderImpl.cs
- RegexWorker.cs
- GridViewColumnCollectionChangedEventArgs.cs
- TemplateColumn.cs
- NetSectionGroup.cs
- COM2PictureConverter.cs
- DataContractSerializerSection.cs
- TemplateApplicationHelper.cs
- ViewStateException.cs
- UIntPtr.cs
- assemblycache.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- ExportOptions.cs
- RecordConverter.cs
- BuildDependencySet.cs
- ModuleConfigurationInfo.cs
- TextRunTypographyProperties.cs
- DbConnectionStringBuilder.cs
- CodeTypeReferenceExpression.cs
- EditBehavior.cs
- ZipIOModeEnforcingStream.cs
- TableLayoutPanel.cs
- RectAnimationBase.cs
- ResolvedKeyFrameEntry.cs
- UrlMappingsSection.cs
- BamlWriter.cs
- TriggerBase.cs
- TemplateManager.cs
- SplineKeyFrames.cs
- CacheDependency.cs
- Oci.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- BinaryNode.cs
- HiddenField.cs
- NotifyIcon.cs
- Timer.cs
- NonSerializedAttribute.cs
- ImageMapEventArgs.cs
- GridView.cs
- StylusEventArgs.cs
- XmlSchemaAll.cs
- Nullable.cs
- ColumnCollectionEditor.cs
- StringSorter.cs
- XMLDiffLoader.cs
- ConnectionsZone.cs
- HostingPreferredMapPath.cs
- DBNull.cs
- RangeBaseAutomationPeer.cs
- QuaternionAnimationUsingKeyFrames.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- VerticalAlignConverter.cs
- ContentIterators.cs
- DefinitionBase.cs
- TypedDataSetSchemaImporterExtensionFx35.cs
- BezierSegment.cs
- ModelFunctionTypeElement.cs
- ContractMapping.cs
- FlowLayout.cs
- MissingMemberException.cs
- TextTreeTextBlock.cs
- ObjectDataSourceSelectingEventArgs.cs
- DrawingImage.cs
- MethodExecutor.cs
- ProcessingInstructionAction.cs
- WebControlAdapter.cs
- TableCell.cs
- ServiceObjectContainer.cs
- TransformerInfoCollection.cs
- CuspData.cs
- AnnotationComponentChooser.cs
- GlobalizationSection.cs
- VariableQuery.cs
- ItemsChangedEventArgs.cs
- Triangle.cs
- PropertyIDSet.cs
- PublishLicense.cs
- SizeF.cs
- ImageConverter.cs
- ConfigXmlSignificantWhitespace.cs
- DSACryptoServiceProvider.cs
- HttpFileCollectionBase.cs
- ClaimTypes.cs
- LinkUtilities.cs
- Funcletizer.cs
- MailAddressCollection.cs
- SecureStringHasher.cs
- VisualBasicSettingsHandler.cs
- Pool.cs
- GacUtil.cs
- Splitter.cs
- ConfigurationSectionGroup.cs
- PolygonHotSpot.cs
- HotCommands.cs
- DtdParser.cs
- BuildProvider.cs
- configsystem.cs
- CodeGenerator.cs
- InProcStateClientManager.cs
- ZoneButton.cs