Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmSyndicationContentDeSerializer.cs / 1305376 / EpmSyndicationContentDeSerializer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Deserializer for the EPM content on the server // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Data.Services.Providers; using System.ServiceModel.Syndication; using System.Diagnostics; using System.Xml; #endregion ///Syndication content reader for EPM content internal sealed class EpmSyndicationContentDeSerializer : EpmContentDeSerializerBase { ///Constructor ///to read content from /// State of the deserializer internal EpmSyndicationContentDeSerializer(SyndicationItem item, EpmContentDeSerializer.EpmContentDeserializerState state) : base(item, state) { } /// Publicly accessible deserialization entry point /// Type of resource to deserialize /// Token corresponding to object ofinternal void DeSerialize(ResourceType resourceType, object element) { this.DeSerialize(resourceType.EpmTargetTree.SyndicationRoot, resourceType, element); } /// Used for deserializing each of syndication specific content nodes /// Node in the target path being processed /// ResourceType /// object being deserialized private void DeSerialize(EpmTargetPathSegment currentRoot, ResourceType resourceType, object element) { foreach (EpmTargetPathSegment newRoot in currentRoot.SubSegments) { if (newRoot.HasContent) { if (!EpmContentDeSerializerBase.Match(newRoot, this.PropertiesApplied)) { switch (newRoot.EpmInfo.Attribute.TargetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: if (this.Item.Authors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Authors[0].Email, this); } break; case SyndicationItemProperty.AuthorName: if (this.Item.Authors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Authors[0].Name, this); } break; case SyndicationItemProperty.AuthorUri: if (this.Item.Authors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Authors[0].Uri, this); } break; case SyndicationItemProperty.ContributorEmail: if (this.Item.Contributors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Contributors[0].Email, this); } break; case SyndicationItemProperty.ContributorName: if (this.Item.Contributors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Contributors[0].Name, this); } break; case SyndicationItemProperty.ContributorUri: if (this.Item.Contributors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Contributors[0].Uri, this); } break; case SyndicationItemProperty.Updated: // If this.Item.LastUpdatedTime == DateTimeOffset.MinValue we assume that the date has not been provided // by the user. This is the same assumption Syndication Api does (see Atom10FeedFormatter.WriteItemContents()). // If the date was not provided by the user we should not touch it - otherwise the response will not be // compatible with response sent for the same request and the same resource type but having KeepInContent set to true if (this.Item.LastUpdatedTime > DateTimeOffset.MinValue) { resourceType.SetEpmValue(newRoot, element, XmlConvert.ToString(this.Item.LastUpdatedTime), this); } break; case SyndicationItemProperty.Published: if (this.Item.PublishDate > DateTimeOffset.MinValue) { resourceType.SetEpmValue(newRoot, element, XmlConvert.ToString(this.Item.PublishDate), this); } break; case SyndicationItemProperty.Rights: if (this.Item.Copyright != null) { resourceType.SetEpmValue(newRoot, element, this.Item.Copyright.Text, this); } break; case SyndicationItemProperty.Summary: if (this.Item.Summary != null) { resourceType.SetEpmValue(newRoot, element, this.Item.Summary.Text, this); } break; case SyndicationItemProperty.Title: if (this.Item.Title != null) { resourceType.SetEpmValue(newRoot, element, this.Item.Title.Text, this); } break; default: Debug.Fail("Unhandled SyndicationItemProperty enum value - should never get here."); break; } } } else { this.DeSerialize(newRoot, resourceType, element); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Deserializer for the EPM content on the server // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { #region Namespaces using System.Data.Services.Providers; using System.ServiceModel.Syndication; using System.Diagnostics; using System.Xml; #endregion ///Syndication content reader for EPM content internal sealed class EpmSyndicationContentDeSerializer : EpmContentDeSerializerBase { ///Constructor ///to read content from /// State of the deserializer internal EpmSyndicationContentDeSerializer(SyndicationItem item, EpmContentDeSerializer.EpmContentDeserializerState state) : base(item, state) { } /// Publicly accessible deserialization entry point /// Type of resource to deserialize /// Token corresponding to object ofinternal void DeSerialize(ResourceType resourceType, object element) { this.DeSerialize(resourceType.EpmTargetTree.SyndicationRoot, resourceType, element); } /// Used for deserializing each of syndication specific content nodes /// Node in the target path being processed /// ResourceType /// object being deserialized private void DeSerialize(EpmTargetPathSegment currentRoot, ResourceType resourceType, object element) { foreach (EpmTargetPathSegment newRoot in currentRoot.SubSegments) { if (newRoot.HasContent) { if (!EpmContentDeSerializerBase.Match(newRoot, this.PropertiesApplied)) { switch (newRoot.EpmInfo.Attribute.TargetSyndicationItem) { case SyndicationItemProperty.AuthorEmail: if (this.Item.Authors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Authors[0].Email, this); } break; case SyndicationItemProperty.AuthorName: if (this.Item.Authors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Authors[0].Name, this); } break; case SyndicationItemProperty.AuthorUri: if (this.Item.Authors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Authors[0].Uri, this); } break; case SyndicationItemProperty.ContributorEmail: if (this.Item.Contributors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Contributors[0].Email, this); } break; case SyndicationItemProperty.ContributorName: if (this.Item.Contributors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Contributors[0].Name, this); } break; case SyndicationItemProperty.ContributorUri: if (this.Item.Contributors.Count > 0) { resourceType.SetEpmValue(newRoot, element, this.Item.Contributors[0].Uri, this); } break; case SyndicationItemProperty.Updated: // If this.Item.LastUpdatedTime == DateTimeOffset.MinValue we assume that the date has not been provided // by the user. This is the same assumption Syndication Api does (see Atom10FeedFormatter.WriteItemContents()). // If the date was not provided by the user we should not touch it - otherwise the response will not be // compatible with response sent for the same request and the same resource type but having KeepInContent set to true if (this.Item.LastUpdatedTime > DateTimeOffset.MinValue) { resourceType.SetEpmValue(newRoot, element, XmlConvert.ToString(this.Item.LastUpdatedTime), this); } break; case SyndicationItemProperty.Published: if (this.Item.PublishDate > DateTimeOffset.MinValue) { resourceType.SetEpmValue(newRoot, element, XmlConvert.ToString(this.Item.PublishDate), this); } break; case SyndicationItemProperty.Rights: if (this.Item.Copyright != null) { resourceType.SetEpmValue(newRoot, element, this.Item.Copyright.Text, this); } break; case SyndicationItemProperty.Summary: if (this.Item.Summary != null) { resourceType.SetEpmValue(newRoot, element, this.Item.Summary.Text, this); } break; case SyndicationItemProperty.Title: if (this.Item.Title != null) { resourceType.SetEpmValue(newRoot, element, this.Item.Title.Text, this); } break; default: Debug.Fail("Unhandled SyndicationItemProperty enum value - should never get here."); break; } } } else { this.DeSerialize(newRoot, resourceType, element); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
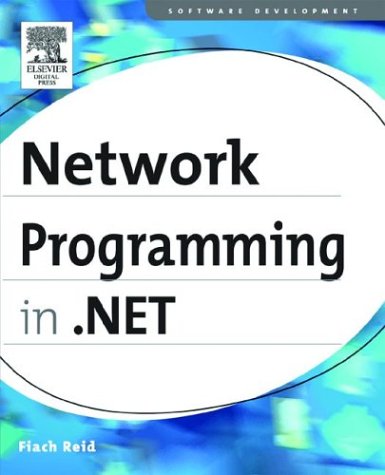
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultParameterValueAttribute.cs
- ControlPropertyNameConverter.cs
- IndexedString.cs
- WeakEventManager.cs
- BreadCrumbTextConverter.cs
- CodeDOMProvider.cs
- TextParaClient.cs
- ResourceExpressionEditorSheet.cs
- ValueUtilsSmi.cs
- SharedDp.cs
- WindowsBrush.cs
- ProgressChangedEventArgs.cs
- ClipboardProcessor.cs
- AsymmetricSignatureFormatter.cs
- TimelineGroup.cs
- DataListAutoFormat.cs
- UIServiceHelper.cs
- DoubleLink.cs
- AutomationEventArgs.cs
- PerspectiveCamera.cs
- ViewCellSlot.cs
- EdmFunction.cs
- HtmlEmptyTagControlBuilder.cs
- Char.cs
- PaperSource.cs
- EntityClientCacheKey.cs
- FunctionImportMapping.ReturnTypeRenameMapping.cs
- pingexception.cs
- TextServicesProperty.cs
- SQLBytes.cs
- XmlEnumAttribute.cs
- SendDesigner.xaml.cs
- MailBnfHelper.cs
- MenuItem.cs
- DependencyPropertyChangedEventArgs.cs
- wgx_exports.cs
- RefreshEventArgs.cs
- DbConnectionPool.cs
- FormatControl.cs
- BypassElementCollection.cs
- RadioButtonPopupAdapter.cs
- SvcMapFileSerializer.cs
- TextEmbeddedObject.cs
- TaskFormBase.cs
- BypassElementCollection.cs
- ParameterCollection.cs
- InternalUserCancelledException.cs
- TemplateControl.cs
- Calendar.cs
- TextBoxLine.cs
- ConsoleTraceListener.cs
- DelegatingTypeDescriptionProvider.cs
- SQLGuid.cs
- DataGridHyperlinkColumn.cs
- XmlWrappingWriter.cs
- OleDbFactory.cs
- ServiceOperationParameter.cs
- DataViewListener.cs
- WebPartMovingEventArgs.cs
- DataRow.cs
- HighlightVisual.cs
- ClientTargetCollection.cs
- ClaimSet.cs
- GridViewRowPresenterBase.cs
- CircleHotSpot.cs
- StringPropertyBuilder.cs
- _ServiceNameStore.cs
- ViewPort3D.cs
- Compiler.cs
- ToolStripProgressBar.cs
- SymbolEqualComparer.cs
- HttpCookie.cs
- SocketAddress.cs
- DataGridViewBindingCompleteEventArgs.cs
- SecondaryViewProvider.cs
- TextParaLineResult.cs
- DomainUpDown.cs
- RuleValidation.cs
- RelatedEnd.cs
- RecommendedAsConfigurableAttribute.cs
- Debug.cs
- __FastResourceComparer.cs
- ComponentEditorForm.cs
- OpenTypeLayout.cs
- StateRuntime.cs
- WorkflowMarkupSerializationManager.cs
- ScrollBarRenderer.cs
- InstancePersistenceCommandException.cs
- EncryptedPackageFilter.cs
- UnsafeNativeMethods.cs
- ObjectItemLoadingSessionData.cs
- RotateTransform3D.cs
- MissingManifestResourceException.cs
- BulletChrome.cs
- SqlServer2KCompatibilityAnnotation.cs
- ExpandedProjectionNode.cs
- DataServiceException.cs
- FormsAuthenticationModule.cs
- OrthographicCamera.cs
- EnumValidator.cs