Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextParagraphProperties.cs / 1305600 / TextParagraphProperties.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextParagraphProperties.cs // // Contents: Text paragraph properties // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-7-2005 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows; using MS.Internal.PresentationCore; namespace System.Windows.Media.TextFormatting { ////// Properties that can change from one paragraph to the next, such as /// text flow direction, text alignment, or indentation. /// public abstract class TextParagraphProperties { ////// This property specifies whether the primary text advance /// direction shall be left-to-right, right-to-left, or top-to-bottom. /// public abstract FlowDirection FlowDirection { get; } ////// This property describes how inline content of a block is aligned. /// public abstract TextAlignment TextAlignment { get; } ////// Paragraph's line height /// public abstract double LineHeight { get; } ////// Indicates the first line of the paragraph. /// public abstract bool FirstLineInParagraph { get; } ////// If true, the formatted line may always be collapsed. If false (the default), /// only lines that overflow the paragraph width are collapsed. /// public virtual bool AlwaysCollapsible { get { return false; } } ////// Paragraph's default run properties /// public abstract TextRunProperties DefaultTextRunProperties { get; } ////// If not null, text decorations to apply to all runs in the line. This is in addition /// to any text decorations specified by the TextRunProperties for individual text runs. /// public virtual TextDecorationCollection TextDecorations { get { return null; } } ////// This property controls whether or not text wraps when it reaches the flow edge /// of its containing block box /// public abstract TextWrapping TextWrapping { get; } ////// This property specifies marker characteristics of the first line in paragraph /// public abstract TextMarkerProperties TextMarkerProperties { get; } ////// Line indentation /// public abstract double Indent { get; } ////// Paragraph indentation /// public virtual double ParagraphIndent { get { return 0; } } ////// Default Incremental Tab /// public virtual double DefaultIncrementalTab { get { return 4 * DefaultTextRunProperties.FontRenderingEmSize; } } ////// Collection of tab definitions /// public virtual IListTabs { get { return null; } } /// /// Lexical component providing hyphenation opportunity. /// #if HYPHENATION_API public virtual TextLexicalService Hyphenator { get { return null; } } #else private TextLexicalService _hyphenator; internal virtual TextLexicalService Hyphenator { [FriendAccessAllowed] // used by Framework get { return _hyphenator; } [FriendAccessAllowed] // used by Framework set { _hyphenator = value; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
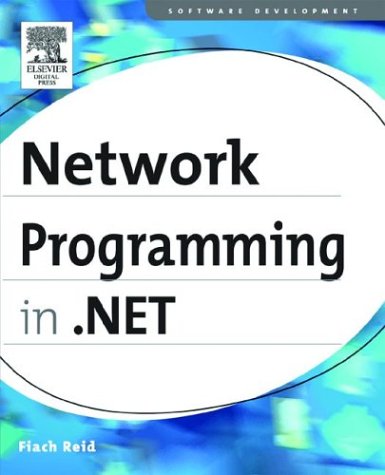
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NativeStructs.cs
- ReachSerializableProperties.cs
- SecurityPolicySection.cs
- NoResizeSelectionBorderGlyph.cs
- DataGridBoolColumn.cs
- SelectionManager.cs
- DbDataRecord.cs
- XNodeNavigator.cs
- XmlCharCheckingReader.cs
- TrackingProvider.cs
- AxWrapperGen.cs
- TreeViewHitTestInfo.cs
- ListBoxAutomationPeer.cs
- TypeInfo.cs
- QueryCacheKey.cs
- BitmapPalette.cs
- ListItemCollection.cs
- DependencySource.cs
- DataConnectionHelper.cs
- InputLangChangeRequestEvent.cs
- OracleNumber.cs
- DesignerValidatorAdapter.cs
- DbBuffer.cs
- RemotingAttributes.cs
- BitStream.cs
- COAUTHINFO.cs
- StringConcat.cs
- IndicFontClient.cs
- DtdParser.cs
- ContextStack.cs
- XPathParser.cs
- HierarchicalDataSourceControl.cs
- ObjectReaderCompiler.cs
- ModifierKeysConverter.cs
- IndexedSelectQueryOperator.cs
- QilXmlWriter.cs
- PasswordPropertyTextAttribute.cs
- Geometry3D.cs
- DependencyPropertyKind.cs
- WebServiceData.cs
- RSAPKCS1SignatureFormatter.cs
- DataGridViewCellParsingEventArgs.cs
- BlockingCollection.cs
- StorageEntitySetMapping.cs
- WorkBatch.cs
- _NegoState.cs
- PeerContact.cs
- _RequestLifetimeSetter.cs
- Errors.cs
- newinstructionaction.cs
- Win32.cs
- ICspAsymmetricAlgorithm.cs
- RegexCapture.cs
- Bidi.cs
- SessionPageStateSection.cs
- ArrayTypeMismatchException.cs
- ExpressionPrinter.cs
- StoragePropertyMapping.cs
- ProcessProtocolHandler.cs
- UnsafeCollabNativeMethods.cs
- TextSimpleMarkerProperties.cs
- StateItem.cs
- MiniLockedBorderGlyph.cs
- RadialGradientBrush.cs
- Options.cs
- RelativeSource.cs
- PlaceHolder.cs
- KeySplineConverter.cs
- HandlerBase.cs
- PackageDigitalSignature.cs
- SqlFunctionAttribute.cs
- InternalConfigConfigurationFactory.cs
- QueryPageSettingsEventArgs.cs
- ObjectFullSpanRewriter.cs
- URL.cs
- DataBinder.cs
- RawMouseInputReport.cs
- BinaryNode.cs
- EntityContainerEmitter.cs
- RegexMatchCollection.cs
- MsmqIntegrationSecurity.cs
- XamlTemplateSerializer.cs
- Image.cs
- UriExt.cs
- DiscreteKeyFrames.cs
- TemplatingOptionsDialog.cs
- RefExpr.cs
- LoadWorkflowByInstanceKeyCommand.cs
- TripleDESCryptoServiceProvider.cs
- EtwTrackingParticipant.cs
- streamingZipPartStream.cs
- CodeParameterDeclarationExpressionCollection.cs
- FixedSOMContainer.cs
- SamlAttributeStatement.cs
- GridProviderWrapper.cs
- CapabilitiesUse.cs
- PathTooLongException.cs
- ValidationContext.cs
- UnsafeNativeMethods.cs
- NavigationService.cs