Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / SamlAttributeStatement.cs / 1 / SamlAttributeStatement.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Globalization; using System.IdentityModel; using System.IdentityModel.Claims; using System.IdentityModel.Selectors; using System.Runtime.Serialization; using System.Xml.Serialization; using System.Xml; public class SamlAttributeStatement : SamlSubjectStatement { readonly ImmutableCollectionattributes = new ImmutableCollection (); bool isReadOnly = false; public SamlAttributeStatement() { } public SamlAttributeStatement(SamlSubject samlSubject, IEnumerable attributes) : base(samlSubject) { if (attributes == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("attributes")); foreach (SamlAttribute attribute in attributes) { if (attribute == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.SAMLEntityCannotBeNullOrEmpty, XD.SamlDictionary.Attribute.Value)); this.attributes.Add(attribute); } CheckObjectValidity(); } public IList Attributes { get { return this.attributes; } } public override bool IsReadOnly { get { return this.isReadOnly; } } public override void MakeReadOnly() { if (!this.isReadOnly) { foreach (SamlAttribute attribute in attributes) { attribute.MakeReadOnly(); } this.attributes.MakeReadOnly(); this.isReadOnly = true; } } void CheckObjectValidity() { if (this.SamlSubject == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLSubjectStatementRequiresSubject))); if (this.attributes.Count == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeShouldHaveOneValue))); } public override void ReadXml(XmlDictionaryReader reader, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer, SecurityTokenResolver outOfBandTokenResolver) { if (reader == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("reader")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; reader.MoveToContent(); reader.Read(); if (reader.IsStartElement(dictionary.Subject, dictionary.Namespace)) { SamlSubject subject = new SamlSubject(); subject.ReadXml(reader, samlSerializer, keyInfoSerializer, outOfBandTokenResolver); base.SamlSubject = subject; } else { // SAML Subject is a required Attribute Statement clause. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeStatementMissingSubjectOnRead))); } while (reader.IsStartElement()) { if (reader.IsStartElement(dictionary.Attribute, dictionary.Namespace)) { // SAML Attribute is a extensibility point. So ask the SAML serializer // to load this part. SamlAttribute attribute = samlSerializer.LoadAttribute(reader, keyInfoSerializer, outOfBandTokenResolver); if (attribute == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLUnableToLoadAttribute))); this.attributes.Add(attribute); } else { break; } } if (this.attributes.Count == 0) { // Each Attribute statement should have at least one attribute. throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenException(SR.GetString(SR.SAMLAttributeStatementMissingAttributeOnRead))); } reader.MoveToContent(); reader.ReadEndElement(); } public override void WriteXml(XmlDictionaryWriter writer, SamlSerializer samlSerializer, SecurityTokenSerializer keyInfoSerializer) { CheckObjectValidity(); if (writer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("writer")); if (samlSerializer == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentNullException("samlSerializer")); #pragma warning suppress 56506 // samlSerializer.DictionaryManager is never null. SamlDictionary dictionary = samlSerializer.DictionaryManager.SamlDictionary; writer.WriteStartElement(dictionary.PreferredPrefix.Value, dictionary.AttributeStatement, dictionary.Namespace); this.SamlSubject.WriteXml(writer, samlSerializer, keyInfoSerializer); for (int i = 0; i < this.attributes.Count; i++) { this.attributes[i].WriteXml(writer, samlSerializer, keyInfoSerializer); } writer.WriteEndElement(); } protected override void AddClaimsToList(IList claims) { if (claims == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("claims"); for (int i = 0; i < attributes.Count; i++) { if (attributes[i] != null) { ReadOnlyCollection attributeClaims = attributes[i].ExtractClaims(); if (attributeClaims != null) { for (int j = 0; j < attributeClaims.Count; ++j) if (attributeClaims[j] != null) claims.Add(attributeClaims[j]); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
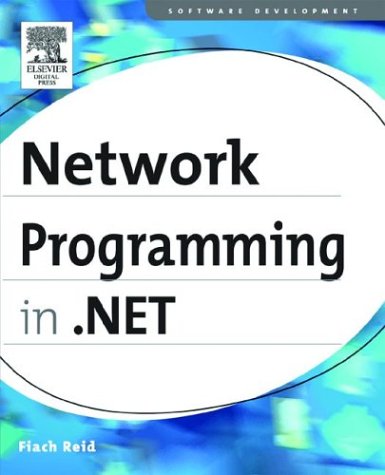
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _DigestClient.cs
- DependencyPropertyDescriptor.cs
- SiteMapDesignerDataSourceView.cs
- ListViewDataItem.cs
- CodeSnippetCompileUnit.cs
- UnionExpr.cs
- HostingEnvironment.cs
- PropertyBuilder.cs
- TreeView.cs
- GeneralTransform3DTo2D.cs
- TextBoxDesigner.cs
- GenerateTemporaryTargetAssembly.cs
- Utils.cs
- Journaling.cs
- RadioButtonFlatAdapter.cs
- XmlValueConverter.cs
- WebPartConnectionsConfigureVerb.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- RenderData.cs
- TableLayoutPanelCellPosition.cs
- Double.cs
- MultiAsyncResult.cs
- AccessDataSourceView.cs
- MemberPathMap.cs
- StoryFragments.cs
- XhtmlBasicValidationSummaryAdapter.cs
- IncrementalCompileAnalyzer.cs
- RemoteArgument.cs
- XpsFilter.cs
- MarkupExtensionReturnTypeAttribute.cs
- InitializationEventAttribute.cs
- SoapParser.cs
- GAC.cs
- WebServiceHandler.cs
- SimpleLine.cs
- Boolean.cs
- OdbcConnectionPoolProviderInfo.cs
- PageAsyncTaskManager.cs
- DatePickerDateValidationErrorEventArgs.cs
- BrowserInteropHelper.cs
- UrlMapping.cs
- DataGridViewColumn.cs
- ImportContext.cs
- SafeThemeHandle.cs
- PlatformCulture.cs
- TemplateEditingFrame.cs
- CollectionView.cs
- SqlDataAdapter.cs
- TransportContext.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- SymLanguageType.cs
- CollectionBase.cs
- Globals.cs
- ResourceProperty.cs
- NumericExpr.cs
- XmlSyndicationContent.cs
- MainMenu.cs
- ObjectQuery_EntitySqlExtensions.cs
- CompressEmulationStream.cs
- _NegoState.cs
- BufferAllocator.cs
- IdentitySection.cs
- ReliabilityContractAttribute.cs
- SqlUtils.cs
- AuthenticatedStream.cs
- Schema.cs
- DragDeltaEventArgs.cs
- DiscoveryMessageSequenceGenerator.cs
- DrawingContextDrawingContextWalker.cs
- CachedResourceDictionaryExtension.cs
- ChtmlTextWriter.cs
- InvalidStoreProtectionKeyException.cs
- ThreadStartException.cs
- RowToFieldTransformer.cs
- WebEventCodes.cs
- OdbcCommand.cs
- mediaeventshelper.cs
- CatalogPartDesigner.cs
- BrowserCapabilitiesFactoryBase.cs
- XmlUrlResolver.cs
- AnnotationAuthorChangedEventArgs.cs
- SubclassTypeValidator.cs
- FieldNameLookup.cs
- AnimatedTypeHelpers.cs
- PersistenceException.cs
- HttpBindingExtension.cs
- File.cs
- RegexBoyerMoore.cs
- XPathNavigator.cs
- LassoHelper.cs
- RequestCachePolicy.cs
- Triangle.cs
- DataMemberConverter.cs
- User.cs
- Stackframe.cs
- DataGridViewAutoSizeModeEventArgs.cs
- ColumnHeader.cs
- XmlDataSource.cs
- TemplateControlCodeDomTreeGenerator.cs
- exports.cs