Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / MainMenu.cs / 1 / MainMenu.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Security.Permissions; ////// /// [ToolboxItemFilter("System.Windows.Forms.MainMenu")] public class MainMenu : Menu { internal Form form; internal Form ownerForm; // this is the form that created this menu, and is the only form allowed to dispose it. private RightToLeft rightToLeft = System.Windows.Forms.RightToLeft.Inherit; private EventHandler onCollapse; ////// Represents /// a menu structure for a form. ////// /// Creates a new MainMenu control. /// public MainMenu() : base(null) { } ////// /// public MainMenu(IContainer container) : this() { container.Add(this); } ///Initializes a new instance of the ///class with the specified container. /// /// Creates a new MainMenu control with the given items to start /// with. /// public MainMenu(MenuItem[] items) : base(items) { } ////// /// [SRDescription(SR.MainMenuCollapseDescr)] public event EventHandler Collapse { add { onCollapse += value; } remove { onCollapse -= value; } } ///[To be supplied.] ////// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, /// text alignment and reading order will be from right to left. /// // VSWhidbey 94189: Add an AmbientValue attribute so that the Reset context menu becomes available in the Property Grid. [ Localizable(true), AmbientValue(RightToLeft.Inherit), SRDescription(SR.MenuRightToLeftDescr) ] public virtual RightToLeft RightToLeft { get { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { if (form != null) { return form.RightToLeft; } else { return RightToLeft.Inherit; } } else { return rightToLeft; } } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)RightToLeft.No, (int)RightToLeft.Inherit)){ throw new InvalidEnumArgumentException("RightToLeft", (int)value, typeof(RightToLeft)); } if (rightToLeft != value) { rightToLeft = value; UpdateRtl((value == System.Windows.Forms.RightToLeft.Yes)); } } } internal override bool RenderIsRightToLeft { get { return (RightToLeft == System.Windows.Forms.RightToLeft.Yes && (form == null || !form.IsMirrored)); } } ////// /// Creates a new MainMenu object which is a dupliate of this one. /// public virtual MainMenu CloneMenu() { MainMenu newMenu = new MainMenu(); newMenu.CloneMenu(this); return newMenu; } ////// /// ///protected override IntPtr CreateMenuHandle() { return UnsafeNativeMethods.CreateMenu(); } /// /// /// Clears out this MainMenu object and discards all of it's resources. /// If the menu is parented in a form, it is disconnected from that as /// well. /// protected override void Dispose(bool disposing) { if (disposing) { if (form != null && (ownerForm == null || form == ownerForm)) { form.Menu = null; } } base.Dispose(disposing); } ////// /// Indicates which form in which we are currently residing [if any] /// [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] public Form GetForm() { return form; } internal Form GetFormUnsafe() { return form; } ////// /// ///internal override void ItemsChanged(int change) { base.ItemsChanged(change); if (form != null) form.MenuChanged(change, this); } /// /// /// ///internal virtual void ItemsChanged(int change, Menu menu) { if (form != null) form.MenuChanged(change, menu); } /// /// /// Fires the collapse event /// protected internal virtual void OnCollapse(EventArgs e) { if (onCollapse != null) { onCollapse(this, e); } } ////// /// Returns true if the RightToLeft should be persisted in code gen. /// internal virtual bool ShouldSerializeRightToLeft() { if (System.Windows.Forms.RightToLeft.Inherit == RightToLeft) { return false; } return true; } ////// /// Returns a string representation for this control. /// ///public override string ToString() { // VSWhidbey 495300: removing GetForm information return base.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; using System.Security.Permissions; ////// /// [ToolboxItemFilter("System.Windows.Forms.MainMenu")] public class MainMenu : Menu { internal Form form; internal Form ownerForm; // this is the form that created this menu, and is the only form allowed to dispose it. private RightToLeft rightToLeft = System.Windows.Forms.RightToLeft.Inherit; private EventHandler onCollapse; ////// Represents /// a menu structure for a form. ////// /// Creates a new MainMenu control. /// public MainMenu() : base(null) { } ////// /// public MainMenu(IContainer container) : this() { container.Add(this); } ///Initializes a new instance of the ///class with the specified container. /// /// Creates a new MainMenu control with the given items to start /// with. /// public MainMenu(MenuItem[] items) : base(items) { } ////// /// [SRDescription(SR.MainMenuCollapseDescr)] public event EventHandler Collapse { add { onCollapse += value; } remove { onCollapse -= value; } } ///[To be supplied.] ////// /// This is used for international applications where the language /// is written from RightToLeft. When this property is true, /// text alignment and reading order will be from right to left. /// // VSWhidbey 94189: Add an AmbientValue attribute so that the Reset context menu becomes available in the Property Grid. [ Localizable(true), AmbientValue(RightToLeft.Inherit), SRDescription(SR.MenuRightToLeftDescr) ] public virtual RightToLeft RightToLeft { get { if (System.Windows.Forms.RightToLeft.Inherit == rightToLeft) { if (form != null) { return form.RightToLeft; } else { return RightToLeft.Inherit; } } else { return rightToLeft; } } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)RightToLeft.No, (int)RightToLeft.Inherit)){ throw new InvalidEnumArgumentException("RightToLeft", (int)value, typeof(RightToLeft)); } if (rightToLeft != value) { rightToLeft = value; UpdateRtl((value == System.Windows.Forms.RightToLeft.Yes)); } } } internal override bool RenderIsRightToLeft { get { return (RightToLeft == System.Windows.Forms.RightToLeft.Yes && (form == null || !form.IsMirrored)); } } ////// /// Creates a new MainMenu object which is a dupliate of this one. /// public virtual MainMenu CloneMenu() { MainMenu newMenu = new MainMenu(); newMenu.CloneMenu(this); return newMenu; } ////// /// ///protected override IntPtr CreateMenuHandle() { return UnsafeNativeMethods.CreateMenu(); } /// /// /// Clears out this MainMenu object and discards all of it's resources. /// If the menu is parented in a form, it is disconnected from that as /// well. /// protected override void Dispose(bool disposing) { if (disposing) { if (form != null && (ownerForm == null || form == ownerForm)) { form.Menu = null; } } base.Dispose(disposing); } ////// /// Indicates which form in which we are currently residing [if any] /// [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] public Form GetForm() { return form; } internal Form GetFormUnsafe() { return form; } ////// /// ///internal override void ItemsChanged(int change) { base.ItemsChanged(change); if (form != null) form.MenuChanged(change, this); } /// /// /// ///internal virtual void ItemsChanged(int change, Menu menu) { if (form != null) form.MenuChanged(change, menu); } /// /// /// Fires the collapse event /// protected internal virtual void OnCollapse(EventArgs e) { if (onCollapse != null) { onCollapse(this, e); } } ////// /// Returns true if the RightToLeft should be persisted in code gen. /// internal virtual bool ShouldSerializeRightToLeft() { if (System.Windows.Forms.RightToLeft.Inherit == RightToLeft) { return false; } return true; } ////// /// Returns a string representation for this control. /// ///public override string ToString() { // VSWhidbey 495300: removing GetForm information return base.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
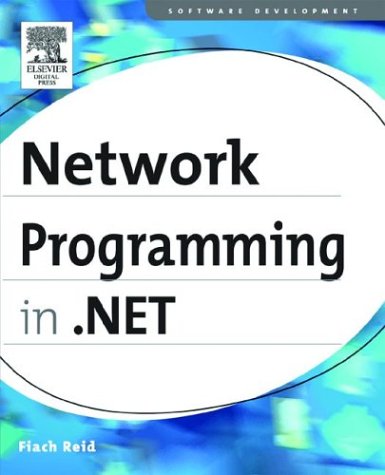
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlWriter.cs
- SHA384.cs
- ListDictionary.cs
- HexParser.cs
- AesCryptoServiceProvider.cs
- ApplicationId.cs
- XmlWrappingReader.cs
- TextLineBreak.cs
- DelegateArgument.cs
- BatchServiceHost.cs
- FileSystemInfo.cs
- ProgressBarRenderer.cs
- MessageEncoder.cs
- TransformerConfigurationWizardBase.cs
- ImageFormat.cs
- WorkflowInstanceRecord.cs
- SharedPersonalizationStateInfo.cs
- SqlMultiplexer.cs
- SoapDocumentServiceAttribute.cs
- OleDbParameter.cs
- ProcessManager.cs
- FigureParaClient.cs
- ConnectorMovedEventArgs.cs
- UnsafeNativeMethods.cs
- NameTable.cs
- XmlSiteMapProvider.cs
- LineSegment.cs
- WebPartConnectionsCloseVerb.cs
- ExpandCollapsePattern.cs
- RoutedEventConverter.cs
- PolicyManager.cs
- QilInvokeEarlyBound.cs
- TextFormatterImp.cs
- SecurityAttributeGenerationHelper.cs
- XamlTemplateSerializer.cs
- TemplateField.cs
- GridViewRow.cs
- StatusBar.cs
- CookielessHelper.cs
- ZipIORawDataFileBlock.cs
- SignatureDescription.cs
- parserscommon.cs
- SelectionPatternIdentifiers.cs
- StringValueConverter.cs
- EventHandlerService.cs
- XmlNamespaceMappingCollection.cs
- CodeMethodReturnStatement.cs
- BindingSource.cs
- ChangePassword.cs
- Math.cs
- ProviderBase.cs
- HostedImpersonationContext.cs
- DataRecordInfo.cs
- MenuBindingsEditor.cs
- DodSequenceMerge.cs
- ProviderSettings.cs
- PointLight.cs
- XsltLoader.cs
- ModelChangedEventArgsImpl.cs
- WindowsGraphicsCacheManager.cs
- SeekableMessageNavigator.cs
- RequestDescription.cs
- ResourceExpression.cs
- ProcessHostMapPath.cs
- SoapSchemaMember.cs
- ProxyOperationRuntime.cs
- DiscreteKeyFrames.cs
- WebPartCatalogCloseVerb.cs
- TimeStampChecker.cs
- DesignerTransactionCloseEvent.cs
- ChineseLunisolarCalendar.cs
- COM2ExtendedUITypeEditor.cs
- StaticTextPointer.cs
- ReadOnlyDataSourceView.cs
- CollectionBase.cs
- CustomErrorsSectionWrapper.cs
- GroupBox.cs
- ImageListDesigner.cs
- UnicodeEncoding.cs
- PointLight.cs
- DesignerVerbCollection.cs
- SmtpClient.cs
- FixedFlowMap.cs
- ISAPIWorkerRequest.cs
- ConfigXmlText.cs
- WorkflowTraceTransfer.cs
- LinqDataSourceContextEventArgs.cs
- DocumentsTrace.cs
- WmiInstallComponent.cs
- RichTextBoxConstants.cs
- PreservationFileReader.cs
- XmlArrayItemAttribute.cs
- DataGridPageChangedEventArgs.cs
- Base64Encoder.cs
- XmlEntityReference.cs
- XmlSchemaCollection.cs
- RecognizedPhrase.cs
- Parser.cs
- Header.cs
- SecurityDocument.cs