Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Server / System / Data / Services / RequestDescription.cs / 1 / RequestDescription.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a description of the request a client has submitted. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Data.Services.Providers; ////// Use this class to describe the data request a client has /// submitted to the service. /// [DebuggerDisplay("RequestDescription={TargetSource} '{ContainerName}' -> {TargetKind} '{TargetElementType}'")] internal class RequestDescription { ///The name of the container for results. private readonly string containerName; ///List of paths to be expanded, possibly null. private readonly ListexpandPaths; /// The MIME type for the requested resource, if specified. private readonly string mimeType; ///URI for the result (without the query component). private readonly Uri resultUri; ///SegmentInfo containing information about every segment in the uri private readonly SegmentInfo[] segmentInfos; ///Whether the container name should be used to name the result. private readonly bool usesContainerName; ////// Initializes a new RequestDescription for a query specified by the /// request Uri. /// /// The kind of target for the request. /// The source for this target. /// URI to the results requested (with no query component). internal RequestDescription(RequestTargetKind targetKind, RequestTargetSource targetSource, Uri resultUri) { WebUtil.DebugEnumIsDefined(targetKind); Debug.Assert(resultUri != null, "resultUri != null"); Debug.Assert(resultUri.IsAbsoluteUri, "resultUri.IsAbsoluteUri(" + resultUri + ")"); SegmentInfo segment = new SegmentInfo(); segment.TargetKind = targetKind; segment.TargetSource = targetSource; segment.SingleResult = true; this.segmentInfos = new SegmentInfo[] { segment }; this.resultUri = resultUri; } ////// Initializes a new RequestDescription for a query specified by the /// request Uri. /// /// list containing information about each segment of the request uri /// Name of the container source. /// Whether the container name should be used to name the result. /// The MIME type for the requested resource, if specified. /// URI to the results requested (with no query component). internal RequestDescription( SegmentInfo[] segmentInfos, string containerName, bool usesContainerName, string mimeType, Uri resultUri) { Debug.Assert(segmentInfos != null && segmentInfos.Length != 0, "segmentInfos != null && segmentInfos.Length != 0"); Debug.Assert(resultUri != null, "resultUri != null"); Debug.Assert(resultUri.IsAbsoluteUri, "resultUri.IsAbsoluteUri(" + resultUri + ")"); this.segmentInfos = segmentInfos; this.containerName = containerName; this.usesContainerName = usesContainerName; this.mimeType = mimeType; this.resultUri = resultUri; } ///Initializes a new RequestDescription based on an existing one. /// Other description to base new description on. /// Query results for new request description. /// List of paths to be expanded during serialization. internal RequestDescription(RequestDescription other, IEnumerable queryResults, ListexpandPaths) { Debug.Assert( queryResults == null || other.SegmentInfos != null, "queryResults == null || other.SegmentInfos != null -- otherwise there isn't a segment in which to replace the query."); Debug.Assert( expandPaths == null || queryResults != null, "expandPaths == null || queryResults != null -- otherwise there isn't a query to execute and expand"); this.containerName = other.containerName; this.mimeType = other.mimeType; this.usesContainerName = other.usesContainerName; this.resultUri = other.resultUri; this.segmentInfos = other.SegmentInfos; this.expandPaths = expandPaths; if (queryResults == null) { this.segmentInfos = other.SegmentInfos; } else { int lastSegmentIndex = other.SegmentInfos.Length - 1; SegmentInfo lastSegmentInfo = other.SegmentInfos[lastSegmentIndex]; Type elementType = ReflectionServiceProvider.GetIEnumerableElement(queryResults.GetType()); Debug.Assert(elementType != null, "elementType != null -- otherwise query processor should have thrown."); lastSegmentInfo.RequestEnumerable = queryResults; lastSegmentInfo.TargetElementType = elementType; } } /// The name of the container for results. internal string ContainerName { [DebuggerStepThrough] get { return this.containerName; } } ///List of paths to be expanded, possibly null. internal ListExpandPaths { [DebuggerStepThrough] get { return this.expandPaths; } } /// URI for the result (without the query component). internal Uri ResultUri { [DebuggerStepThrough] get { return this.resultUri; } } ///Returns the list containing the information about each segment that make up the request uri internal SegmentInfo[] SegmentInfos { [DebuggerStepThrough] get { return this.segmentInfos; } } ///The base query for the request, before client-specified composition. internal IEnumerable RequestEnumerable { get { return this.LastSegmentInfo.RequestEnumerable; } } ///Whether the result of this request is a single element. internal bool IsSingleResult { get { return this.LastSegmentInfo.SingleResult; } } ///The MIME type for the requested resource, if specified. internal string MimeType { [DebuggerStepThrough] get { return this.mimeType; } } ///The kind of target being requested. internal RequestTargetKind TargetKind { get { return this.LastSegmentInfo.TargetKind; } } ///The type of element targetted by this request. internal Type TargetElementType { get { return this.LastSegmentInfo.TargetElementType; } } ///The type of source for the request target. internal RequestTargetSource TargetSource { get { return this.LastSegmentInfo.TargetSource; } } ////// Returns the resource property on which this query is targeted /// internal ResourceProperty Property { get { return this.LastSegmentInfo.ProjectedProperty; } } ///Whether the container name should be used to name the result. internal bool UsesContainerName { [DebuggerStepThrough] get { return this.usesContainerName; } } ///Returns the last segment internal SegmentInfo LastSegmentInfo { get { return this.segmentInfos[this.segmentInfos.Length - 1]; } } ///Returns true if the request description refers to a link uri. Otherwise returns false. internal bool LinkUri { get { return (this.segmentInfos.Length >= 3 && this.segmentInfos[this.segmentInfos.Length - 2].TargetKind == RequestTargetKind.Link); } } ////// Get the single result from the given segment info /// /// segmentInfo which contains the request query ///query result as returned by the IQueryable query internal static IEnumerator GetSingleResultFromEnumerable(SegmentInfo segmentInfo) { IEnumerator queryResults = WebUtil.GetRequestEnumerator(segmentInfo.RequestEnumerable); bool shouldDispose = true; try { WebUtil.CheckResourceExists(queryResults.MoveNext(), segmentInfo.Identifier); // e.g. /Customers(4) - if there is a direct reference to an entity, it should not be null. // e.g. $value also must not be null, since you are dereferencing the values // Any other case, having null is fine if (segmentInfo.IsDirectReference && queryResults.Current == null) { throw DataServiceException.CreateResourceNotFound(segmentInfo.Identifier); } #if ASTORIA_OPEN_OBJECT IEnumerable enumerable; if (segmentInfo.TargetKind == RequestTargetKind.OpenProperty && WebUtil.IsElementIEnumerable(queryResults.Current, out enumerable)) { throw DataServiceException.CreateSyntaxError( Strings.InvalidUri_OpenCollectionPropertiesMustHaveParenthesis(segmentInfo.Identifier)); } #endif shouldDispose = false; return queryResults; } finally { // Dispose the Enumerator in case of error if (shouldDispose) { WebUtil.Dispose(queryResults); } } } ////// Create a new request description from the given request description and new entity as the result. /// /// Existing request description. /// entity that needs to be the result of the new request. /// container to which the entity belongs to. ///a new instance of request description containing information about the given entity. internal static RequestDescription CreateSingleResultRequestDescription( RequestDescription description, object entity, ResourceContainer container) { // Create a new request description for the results that will be returned. SegmentInfo segmentInfo = new SegmentInfo(); segmentInfo.RequestEnumerable = new object[] { entity }; segmentInfo.TargetKind = description.TargetKind; segmentInfo.TargetSource = description.TargetSource; segmentInfo.SingleResult = true; segmentInfo.ProjectedProperty = description.Property; segmentInfo.TargetElementType = container != null ? container.ResourceType.Type : typeof(object); segmentInfo.TargetContainer = container; segmentInfo.Identifier = description.LastSegmentInfo.Identifier; #if DEBUG segmentInfo.AssertValid(); #endif SegmentInfo[] segmentInfos = description.SegmentInfos; segmentInfos[segmentInfos.Length - 1] = segmentInfo; return new RequestDescription( segmentInfos, container != null ? container.Name : null, description.UsesContainerName, description.MimeType, description.ResultUri); } ////// Returns the last segment info whose target request kind is resource /// ///The index of the parent resource internal int GetIndexOfTargetEntityResource() { Debug.Assert(this.segmentInfos.Length >= 1, "this.segmentInfos.Length >= 1"); int result = -1; if (this.LinkUri) { return this.SegmentInfos.Length - 1; } for (int j = this.SegmentInfos.Length - 1; j >= 0; j--) { if (this.segmentInfos[j].TargetKind == RequestTargetKind.Resource || this.segmentInfos[j].HasKeyValues) { result = j; break; } } Debug.Assert(result != -1, "This method should never be called for request that doesn't have a parent resource"); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a description of the request a client has submitted. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Data.Services.Providers; ////// Use this class to describe the data request a client has /// submitted to the service. /// [DebuggerDisplay("RequestDescription={TargetSource} '{ContainerName}' -> {TargetKind} '{TargetElementType}'")] internal class RequestDescription { ///The name of the container for results. private readonly string containerName; ///List of paths to be expanded, possibly null. private readonly ListexpandPaths; /// The MIME type for the requested resource, if specified. private readonly string mimeType; ///URI for the result (without the query component). private readonly Uri resultUri; ///SegmentInfo containing information about every segment in the uri private readonly SegmentInfo[] segmentInfos; ///Whether the container name should be used to name the result. private readonly bool usesContainerName; ////// Initializes a new RequestDescription for a query specified by the /// request Uri. /// /// The kind of target for the request. /// The source for this target. /// URI to the results requested (with no query component). internal RequestDescription(RequestTargetKind targetKind, RequestTargetSource targetSource, Uri resultUri) { WebUtil.DebugEnumIsDefined(targetKind); Debug.Assert(resultUri != null, "resultUri != null"); Debug.Assert(resultUri.IsAbsoluteUri, "resultUri.IsAbsoluteUri(" + resultUri + ")"); SegmentInfo segment = new SegmentInfo(); segment.TargetKind = targetKind; segment.TargetSource = targetSource; segment.SingleResult = true; this.segmentInfos = new SegmentInfo[] { segment }; this.resultUri = resultUri; } ////// Initializes a new RequestDescription for a query specified by the /// request Uri. /// /// list containing information about each segment of the request uri /// Name of the container source. /// Whether the container name should be used to name the result. /// The MIME type for the requested resource, if specified. /// URI to the results requested (with no query component). internal RequestDescription( SegmentInfo[] segmentInfos, string containerName, bool usesContainerName, string mimeType, Uri resultUri) { Debug.Assert(segmentInfos != null && segmentInfos.Length != 0, "segmentInfos != null && segmentInfos.Length != 0"); Debug.Assert(resultUri != null, "resultUri != null"); Debug.Assert(resultUri.IsAbsoluteUri, "resultUri.IsAbsoluteUri(" + resultUri + ")"); this.segmentInfos = segmentInfos; this.containerName = containerName; this.usesContainerName = usesContainerName; this.mimeType = mimeType; this.resultUri = resultUri; } ///Initializes a new RequestDescription based on an existing one. /// Other description to base new description on. /// Query results for new request description. /// List of paths to be expanded during serialization. internal RequestDescription(RequestDescription other, IEnumerable queryResults, ListexpandPaths) { Debug.Assert( queryResults == null || other.SegmentInfos != null, "queryResults == null || other.SegmentInfos != null -- otherwise there isn't a segment in which to replace the query."); Debug.Assert( expandPaths == null || queryResults != null, "expandPaths == null || queryResults != null -- otherwise there isn't a query to execute and expand"); this.containerName = other.containerName; this.mimeType = other.mimeType; this.usesContainerName = other.usesContainerName; this.resultUri = other.resultUri; this.segmentInfos = other.SegmentInfos; this.expandPaths = expandPaths; if (queryResults == null) { this.segmentInfos = other.SegmentInfos; } else { int lastSegmentIndex = other.SegmentInfos.Length - 1; SegmentInfo lastSegmentInfo = other.SegmentInfos[lastSegmentIndex]; Type elementType = ReflectionServiceProvider.GetIEnumerableElement(queryResults.GetType()); Debug.Assert(elementType != null, "elementType != null -- otherwise query processor should have thrown."); lastSegmentInfo.RequestEnumerable = queryResults; lastSegmentInfo.TargetElementType = elementType; } } /// The name of the container for results. internal string ContainerName { [DebuggerStepThrough] get { return this.containerName; } } ///List of paths to be expanded, possibly null. internal ListExpandPaths { [DebuggerStepThrough] get { return this.expandPaths; } } /// URI for the result (without the query component). internal Uri ResultUri { [DebuggerStepThrough] get { return this.resultUri; } } ///Returns the list containing the information about each segment that make up the request uri internal SegmentInfo[] SegmentInfos { [DebuggerStepThrough] get { return this.segmentInfos; } } ///The base query for the request, before client-specified composition. internal IEnumerable RequestEnumerable { get { return this.LastSegmentInfo.RequestEnumerable; } } ///Whether the result of this request is a single element. internal bool IsSingleResult { get { return this.LastSegmentInfo.SingleResult; } } ///The MIME type for the requested resource, if specified. internal string MimeType { [DebuggerStepThrough] get { return this.mimeType; } } ///The kind of target being requested. internal RequestTargetKind TargetKind { get { return this.LastSegmentInfo.TargetKind; } } ///The type of element targetted by this request. internal Type TargetElementType { get { return this.LastSegmentInfo.TargetElementType; } } ///The type of source for the request target. internal RequestTargetSource TargetSource { get { return this.LastSegmentInfo.TargetSource; } } ////// Returns the resource property on which this query is targeted /// internal ResourceProperty Property { get { return this.LastSegmentInfo.ProjectedProperty; } } ///Whether the container name should be used to name the result. internal bool UsesContainerName { [DebuggerStepThrough] get { return this.usesContainerName; } } ///Returns the last segment internal SegmentInfo LastSegmentInfo { get { return this.segmentInfos[this.segmentInfos.Length - 1]; } } ///Returns true if the request description refers to a link uri. Otherwise returns false. internal bool LinkUri { get { return (this.segmentInfos.Length >= 3 && this.segmentInfos[this.segmentInfos.Length - 2].TargetKind == RequestTargetKind.Link); } } ////// Get the single result from the given segment info /// /// segmentInfo which contains the request query ///query result as returned by the IQueryable query internal static IEnumerator GetSingleResultFromEnumerable(SegmentInfo segmentInfo) { IEnumerator queryResults = WebUtil.GetRequestEnumerator(segmentInfo.RequestEnumerable); bool shouldDispose = true; try { WebUtil.CheckResourceExists(queryResults.MoveNext(), segmentInfo.Identifier); // e.g. /Customers(4) - if there is a direct reference to an entity, it should not be null. // e.g. $value also must not be null, since you are dereferencing the values // Any other case, having null is fine if (segmentInfo.IsDirectReference && queryResults.Current == null) { throw DataServiceException.CreateResourceNotFound(segmentInfo.Identifier); } #if ASTORIA_OPEN_OBJECT IEnumerable enumerable; if (segmentInfo.TargetKind == RequestTargetKind.OpenProperty && WebUtil.IsElementIEnumerable(queryResults.Current, out enumerable)) { throw DataServiceException.CreateSyntaxError( Strings.InvalidUri_OpenCollectionPropertiesMustHaveParenthesis(segmentInfo.Identifier)); } #endif shouldDispose = false; return queryResults; } finally { // Dispose the Enumerator in case of error if (shouldDispose) { WebUtil.Dispose(queryResults); } } } ////// Create a new request description from the given request description and new entity as the result. /// /// Existing request description. /// entity that needs to be the result of the new request. /// container to which the entity belongs to. ///a new instance of request description containing information about the given entity. internal static RequestDescription CreateSingleResultRequestDescription( RequestDescription description, object entity, ResourceContainer container) { // Create a new request description for the results that will be returned. SegmentInfo segmentInfo = new SegmentInfo(); segmentInfo.RequestEnumerable = new object[] { entity }; segmentInfo.TargetKind = description.TargetKind; segmentInfo.TargetSource = description.TargetSource; segmentInfo.SingleResult = true; segmentInfo.ProjectedProperty = description.Property; segmentInfo.TargetElementType = container != null ? container.ResourceType.Type : typeof(object); segmentInfo.TargetContainer = container; segmentInfo.Identifier = description.LastSegmentInfo.Identifier; #if DEBUG segmentInfo.AssertValid(); #endif SegmentInfo[] segmentInfos = description.SegmentInfos; segmentInfos[segmentInfos.Length - 1] = segmentInfo; return new RequestDescription( segmentInfos, container != null ? container.Name : null, description.UsesContainerName, description.MimeType, description.ResultUri); } ////// Returns the last segment info whose target request kind is resource /// ///The index of the parent resource internal int GetIndexOfTargetEntityResource() { Debug.Assert(this.segmentInfos.Length >= 1, "this.segmentInfos.Length >= 1"); int result = -1; if (this.LinkUri) { return this.SegmentInfos.Length - 1; } for (int j = this.SegmentInfos.Length - 1; j >= 0; j--) { if (this.segmentInfos[j].TargetKind == RequestTargetKind.Resource || this.segmentInfos[j].HasKeyValues) { result = j; break; } } Debug.Assert(result != -1, "This method should never be called for request that doesn't have a parent resource"); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
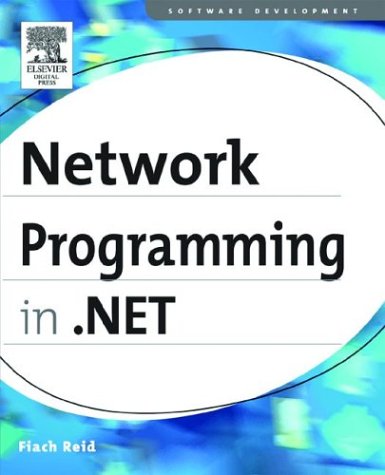
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FolderLevelBuildProviderCollection.cs
- TreeViewImageIndexConverter.cs
- CustomError.cs
- UrlRoutingHandler.cs
- Timer.cs
- BookmarkWorkItem.cs
- BitStack.cs
- LayoutEngine.cs
- StateManagedCollection.cs
- NativeMethods.cs
- PageVisual.cs
- ReadWriteObjectLock.cs
- StickyNote.cs
- RefreshPropertiesAttribute.cs
- XmlSerializerSection.cs
- DictionaryCustomTypeDescriptor.cs
- KeyFrames.cs
- HttpHeaderCollection.cs
- ProxyGenerationError.cs
- ResourceProviderFactory.cs
- WindowsScrollBar.cs
- DrawingContextDrawingContextWalker.cs
- BufferedWebEventProvider.cs
- CroppedBitmap.cs
- InputEventArgs.cs
- TraceData.cs
- SkewTransform.cs
- UInt16Storage.cs
- RootCodeDomSerializer.cs
- RangeValuePattern.cs
- HttpResponse.cs
- ContainerParagraph.cs
- HyperLinkStyle.cs
- TextStore.cs
- ServiceMetadataBehavior.cs
- PersonalizationState.cs
- ToolStripComboBox.cs
- HttpBufferlessInputStream.cs
- GridEntryCollection.cs
- TemplateControlCodeDomTreeGenerator.cs
- ServicePoint.cs
- AuthenticationManager.cs
- Span.cs
- BackoffTimeoutHelper.cs
- OneToOneMappingSerializer.cs
- ResourceManager.cs
- TextFindEngine.cs
- TextBlockAutomationPeer.cs
- ObjectDataSourceEventArgs.cs
- DictionaryChange.cs
- SmtpReplyReader.cs
- DataViewListener.cs
- TextEditorThreadLocalStore.cs
- VoiceObjectToken.cs
- brushes.cs
- StatusBarDesigner.cs
- path.cs
- DataGridAddNewRow.cs
- UnionExpr.cs
- ClientSponsor.cs
- XmlNamespaceManager.cs
- EncryptedPackage.cs
- GridSplitterAutomationPeer.cs
- SchemaImporterExtension.cs
- TextFormattingConverter.cs
- SocketManager.cs
- EnlistmentTraceIdentifier.cs
- DetailsViewPageEventArgs.cs
- DataGridHeaderBorder.cs
- TreeNode.cs
- ConvertersCollection.cs
- SmiRequestExecutor.cs
- FixedSOMContainer.cs
- BindingOperations.cs
- IntSecurity.cs
- CodeEventReferenceExpression.cs
- ValidateNames.cs
- DataGridViewColumnEventArgs.cs
- PingReply.cs
- BulletChrome.cs
- _Connection.cs
- WindowShowOrOpenTracker.cs
- PermissionRequestEvidence.cs
- FontSizeConverter.cs
- GlyphTypeface.cs
- BuildProviderAppliesToAttribute.cs
- RectangleGeometry.cs
- DbConnectionPoolGroupProviderInfo.cs
- GeometryModel3D.cs
- ToolStripPanelRow.cs
- PersianCalendar.cs
- TraceContext.cs
- DocumentPaginator.cs
- Literal.cs
- DataComponentMethodGenerator.cs
- TableProviderWrapper.cs
- SchemaDeclBase.cs
- DataSourceCache.cs
- OutgoingWebRequestContext.cs
- Metadata.cs