Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Generated / SkewTransform.cs / 2 / SkewTransform.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media { sealed partial class SkewTransform : Transform { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new SkewTransform Clone() { return (SkewTransform)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new SkewTransform CloneCurrentValue() { return (SkewTransform)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void AngleXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { SkewTransform target = ((SkewTransform) d); target.PropertyChanged(AngleXProperty); } private static void AngleYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { SkewTransform target = ((SkewTransform) d); target.PropertyChanged(AngleYProperty); } private static void CenterXPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { SkewTransform target = ((SkewTransform) d); target.PropertyChanged(CenterXProperty); } private static void CenterYPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { SkewTransform target = ((SkewTransform) d); target.PropertyChanged(CenterYProperty); } #region Public Properties ////// AngleX - double. Default value is 0.0. /// public double AngleX { get { return (double) GetValue(AngleXProperty); } set { SetValueInternal(AngleXProperty, value); } } ////// AngleY - double. Default value is 0.0 . /// public double AngleY { get { return (double) GetValue(AngleYProperty); } set { SetValueInternal(AngleYProperty, value); } } ////// CenterX - double. Default value is 0.0. /// public double CenterX { get { return (double) GetValue(CenterXProperty); } set { SetValueInternal(CenterXProperty, value); } } ////// CenterY - double. Default value is 0.0. /// public double CenterY { get { return (double) GetValue(CenterYProperty); } set { SetValueInternal(CenterYProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new SkewTransform(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels are safe to call into and do not go cross domain and cross process /// [SecurityCritical,SecurityTreatAsSafe] internal override void UpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { base.UpdateResource(channel, skipOnChannelCheck); // Obtain handles for animated properties DUCE.ResourceHandle hAngleXAnimations = GetAnimationResourceHandle(AngleXProperty, channel); DUCE.ResourceHandle hAngleYAnimations = GetAnimationResourceHandle(AngleYProperty, channel); DUCE.ResourceHandle hCenterXAnimations = GetAnimationResourceHandle(CenterXProperty, channel); DUCE.ResourceHandle hCenterYAnimations = GetAnimationResourceHandle(CenterYProperty, channel); // Pack & send command packet DUCE.MILCMD_SKEWTRANSFORM data; unsafe { data.Type = MILCMD.MilCmdSkewTransform; data.Handle = _duceResource.GetHandle(channel); if (hAngleXAnimations.IsNull) { data.AngleX = AngleX; } data.hAngleXAnimations = hAngleXAnimations; if (hAngleYAnimations.IsNull) { data.AngleY = AngleY; } data.hAngleYAnimations = hAngleYAnimations; if (hCenterXAnimations.IsNull) { data.CenterX = CenterX; } data.hCenterXAnimations = hCenterXAnimations; if (hCenterYAnimations.IsNull) { data.CenterY = CenterY; } data.hCenterYAnimations = hCenterYAnimations; // Send packed command structure channel.SendCommand( (byte*)&data, sizeof(DUCE.MILCMD_SKEWTRANSFORM)); } } } internal override DUCE.ResourceHandle AddRefOnChannelCore(DUCE.Channel channel) { if (_duceResource.CreateOrAddRefOnChannel(channel, System.Windows.Media.Composition.DUCE.ResourceType.TYPE_SKEWTRANSFORM)) { AddRefOnChannelAnimations(channel); UpdateResource(channel, true /* skip "on channel" check - we already know that we're on channel */ ); } return _duceResource.GetHandle(channel); } internal override void ReleaseOnChannelCore(DUCE.Channel channel) { Debug.Assert(_duceResource.IsOnChannel(channel)); if (_duceResource.ReleaseOnChannel(channel)) { ReleaseOnChannelAnimations(channel); } } internal override DUCE.ResourceHandle GetHandleCore(DUCE.Channel channel) { // Note that we are in a lock here already. return _duceResource.GetHandle(channel); } internal override int GetChannelCountCore() { // must already be in composition lock here return _duceResource.GetChannelCount(); } internal override DUCE.Channel GetChannelCore(int index) { // Note that we are in a lock here already. return _duceResource.GetChannel(index); } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the SkewTransform.AngleX property. /// public static readonly DependencyProperty AngleXProperty = RegisterProperty("AngleX", typeof(double), typeof(SkewTransform), 0.0, new PropertyChangedCallback(AngleXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the SkewTransform.AngleY property. /// public static readonly DependencyProperty AngleYProperty = RegisterProperty("AngleY", typeof(double), typeof(SkewTransform), 0.0 , new PropertyChangedCallback(AngleYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the SkewTransform.CenterX property. /// public static readonly DependencyProperty CenterXProperty = RegisterProperty("CenterX", typeof(double), typeof(SkewTransform), 0.0, new PropertyChangedCallback(CenterXPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the SkewTransform.CenterY property. /// public static readonly DependencyProperty CenterYProperty = RegisterProperty("CenterY", typeof(double), typeof(SkewTransform), 0.0, new PropertyChangedCallback(CenterYPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal System.Windows.Media.Composition.DUCE.MultiChannelResource _duceResource = new System.Windows.Media.Composition.DUCE.MultiChannelResource(); internal const double c_AngleX = 0.0; internal const double c_AngleY = 0.0 ; internal const double c_CenterX = 0.0; internal const double c_CenterY = 0.0; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
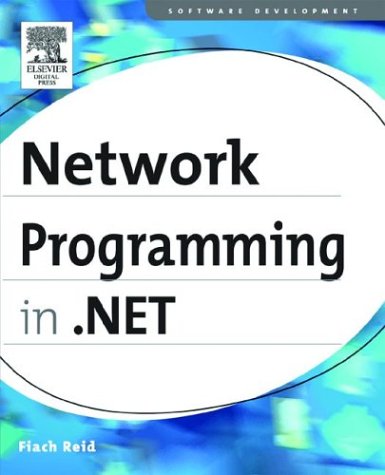
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CLRBindingWorker.cs
- ProfileInfo.cs
- BitmapDecoder.cs
- WebEvents.cs
- WebMessageEncodingBindingElement.cs
- BoundsDrawingContextWalker.cs
- ParseElement.cs
- RowCache.cs
- GenericEnumerator.cs
- EdmProviderManifest.cs
- ProtocolsConfigurationHandler.cs
- documentsequencetextcontainer.cs
- EntityDataSourceSelectingEventArgs.cs
- TdsParserStateObject.cs
- ProviderConnectionPointCollection.cs
- SerializationObjectManager.cs
- TreeViewItemAutomationPeer.cs
- errorpatternmatcher.cs
- GraphicsContainer.cs
- EasingQuaternionKeyFrame.cs
- CheckBoxField.cs
- BoundPropertyEntry.cs
- ToolboxItemAttribute.cs
- DataGridViewRowStateChangedEventArgs.cs
- SyntaxCheck.cs
- wmiutil.cs
- IntPtr.cs
- BinaryWriter.cs
- RegistryConfigurationProvider.cs
- SHA384Managed.cs
- ExpandedProjectionNode.cs
- ReachFixedPageSerializer.cs
- MetadataItemEmitter.cs
- SystemIcmpV6Statistics.cs
- SByteStorage.cs
- AttachedPropertyBrowsableAttribute.cs
- Monitor.cs
- FormsAuthenticationEventArgs.cs
- XmlReturnWriter.cs
- BitmapEffectDrawing.cs
- XPathChildIterator.cs
- InputGestureCollection.cs
- shaper.cs
- Int64.cs
- CommandField.cs
- IApplicationTrustManager.cs
- SqlUserDefinedTypeAttribute.cs
- AssemblyGen.cs
- IndividualDeviceConfig.cs
- XamlHostingSectionGroup.cs
- IisTraceWebEventProvider.cs
- SimpleType.cs
- InitializerFacet.cs
- CqlLexer.cs
- GridViewColumnCollection.cs
- BulletDecorator.cs
- ViewStateChangedEventArgs.cs
- TiffBitmapEncoder.cs
- Matrix.cs
- RankException.cs
- ExpressionHelper.cs
- CipherData.cs
- Dump.cs
- SubstitutionList.cs
- DataStreamFromComStream.cs
- HTTPNotFoundHandler.cs
- WindowsAuthenticationEventArgs.cs
- ContentElement.cs
- AllMembershipCondition.cs
- SecureUICommand.cs
- DiscoveryExceptionDictionary.cs
- ActivityBuilderHelper.cs
- _CommandStream.cs
- ReadOnlyKeyedCollection.cs
- BamlTreeUpdater.cs
- SQLDecimal.cs
- TreeViewDataItemAutomationPeer.cs
- ActivityStateRecord.cs
- EdmTypeAttribute.cs
- DataMemberAttribute.cs
- HwndMouseInputProvider.cs
- EndEvent.cs
- AdjustableArrowCap.cs
- CssClassPropertyAttribute.cs
- StoreAnnotationsMap.cs
- KeyFrames.cs
- OutOfProcStateClientManager.cs
- RectAnimationBase.cs
- IMembershipProvider.cs
- CharacterString.cs
- Int32CAMarshaler.cs
- DeobfuscatingStream.cs
- DeploymentSection.cs
- ResourceExpressionBuilder.cs
- XsltSettings.cs
- TransportContext.cs
- PersonalizableAttribute.cs
- FrameworkElement.cs
- RegexParser.cs
- CdpEqualityComparer.cs