Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / TreeViewItemAutomationPeer.cs / 1477467 / TreeViewItemAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class TreeViewItemAutomationPeer : ItemsControlAutomationPeer, IExpandCollapseProvider, ISelectionItemProvider, IScrollItemProvider { /// public TreeViewItemAutomationPeer(TreeViewItem owner): base(owner) { } /// override protected string GetClassNameCore() { return "TreeViewItem"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.TreeItem; } /// override protected bool IsOffscreenCore() { if (!Owner.IsVisible) return true; Rect boundingRect = CalculateVisibleBoundingRect(); return (boundingRect == Rect.Empty || boundingRect.Height == 0 || boundingRect.Width == 0); } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.ExpandCollapse) { return this; } else if (patternInterface == PatternInterface.SelectionItem) { return this; } else if (patternInterface == PatternInterface.ScrollItem) { return this; } return base.GetPattern(patternInterface); } /// protected override ListGetChildrenCore() { List children = null; ItemPeersStorage oldChildren = ItemPeers; //cache the old ones for possible reuse ItemPeers = new ItemPeersStorage (); TreeViewItem owner = Owner as TreeViewItem; if (owner != null) { iterate(this, owner, (IteratorCallback)delegate(AutomationPeer peer) { if (children == null) children = new List (); children.Add(peer); return (false); }, ItemPeers, oldChildren); } return children; } private delegate bool IteratorCallback(AutomationPeer peer); // private static bool iterate(TreeViewItemAutomationPeer logicalParentAp, DependencyObject parent, IteratorCallback callback, ItemPeersStorage dataChildren, ItemPeersStorage oldChildren) { bool done = false; if (parent != null) { AutomationPeer peer = null; int count = VisualTreeHelper.GetChildrenCount(parent); for (int i = 0; i < count && !done; i++) { DependencyObject child = VisualTreeHelper.GetChild(parent, i); if (child != null && child is UIElement) { if (child is TreeViewItem) { object dataItem = (child as UIElement) != null ? (logicalParentAp.Owner as ItemsControl).GetItemOrContainerFromContainer(child as UIElement) : child; peer = oldChildren[dataItem]; if (peer == null) { peer = logicalParentAp.GetPeerFromWeakRefStorage(dataItem); if (peer != null) { // As cached peer is getting used it must be invalidated. peer.AncestorsInvalid = false; peer.ChildrenValid = false; } } if (peer == null) { peer = logicalParentAp.CreateItemAutomationPeer(dataItem); } //perform hookup so the events sourced from wrapper peer are fired as if from the data item if (peer != null) { AutomationPeer wrapperPeer = (peer as ItemAutomationPeer).GetWrapperPeer(); if (wrapperPeer != null) { wrapperPeer.EventsSource = peer; } if (dataChildren[dataItem] == null && peer is ItemAutomationPeer) { callback(peer); dataChildren[dataItem] = peer as ItemAutomationPeer; } } } else { peer = CreatePeerForElement((UIElement)child); if (peer != null) done = callback(peer); } if(peer == null) done = iterate(logicalParentAp, child, callback, dataChildren, oldChildren); } else { done = iterate(logicalParentAp, child, callback, dataChildren, oldChildren); } } } return done; } /// /// It returns the ItemAutomationPeer if it exist corresponding to the item otherwise it creates /// one and does add the Handle and parent info by calling TrySetParentInfo. /// ////// Security Critical - Calls a Security Critical operation /// SecurityTreatAsSafe - It's being called from this object which is real parent for the item peer. /// /// ///[SecurityCritical, SecurityTreatAsSafe] override internal ItemAutomationPeer FindOrCreateItemAutomationPeer(object item) { ItemAutomationPeer peer = ItemPeers[item]; AutomationPeer parentPeer = this; if (EventsSource as TreeViewDataItemAutomationPeer != null) { parentPeer = EventsSource as TreeViewDataItemAutomationPeer; } if (peer == null) peer = GetPeerFromWeakRefStorage(item); if (peer == null) { peer = CreateItemAutomationPeer(item); if(peer != null) peer.TrySetParentInfo(parentPeer); AutomationPeer wrapperPeer = (peer as ItemAutomationPeer).GetWrapperPeer(); if (wrapperPeer != null) { wrapperPeer.EventsSource = peer; } } return peer; } /// internal override bool IsPropertySupportedByControlForFindItem(int id) { if (base.IsPropertySupportedByControlForFindItem(id)) return true; else { if (SelectionItemPatternIdentifiers.IsSelectedProperty.Id == id) return true; else return false; } } /// /// Support for IsSelectedProperty should come from SelectorAutomationPeer only, /// internal override object GetSupportedPropertyValue(ItemAutomationPeer itemPeer, int propertyId) { if (SelectionItemPatternIdentifiers.IsSelectedProperty.Id == propertyId) { ISelectionItemProvider selectionItem = itemPeer.GetPattern(PatternInterface.SelectionItem) as ISelectionItemProvider; if (selectionItem != null) return selectionItem.IsSelected; else return null; } return base.GetSupportedPropertyValue(itemPeer, propertyId); } /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { return new TreeViewDataItemAutomationPeer(item, this, EventsSource as TreeViewDataItemAutomationPeer); } // override internal void UpdateChildren() { // To ensure that the Updation of children should be initiated from DataPeer so as to have the right parent value stored for children TreeViewDataItemAutomationPeer dataPeer = EventsSource as TreeViewDataItemAutomationPeer; if(dataPeer != null) dataPeer.UpdateChildrenInternal(AutomationInteropProvider.ItemsInvalidateLimit); else UpdateChildrenInternal(AutomationInteropProvider.ItemsInvalidateLimit); WeakRefElementProxyStorage.PurgeWeakRefCollection(); } ///internal void AddDataPeerInfo(TreeViewDataItemAutomationPeer dataPeer) { EventsSource = dataPeer; UpdateWeakRefStorageFromDataPeer(); } /// internal void UpdateWeakRefStorageFromDataPeer() { // To use the already stored WeakRef collection of it's children Items which might be created when last time this item was realized. if(EventsSource as TreeViewDataItemAutomationPeer != null) { if((EventsSource as TreeViewDataItemAutomationPeer).WeakRefElementProxyStorageCache == null) (EventsSource as TreeViewDataItemAutomationPeer).WeakRefElementProxyStorageCache = WeakRefElementProxyStorage; else if(WeakRefElementProxyStorage.Count == 0) { WeakRefElementProxyStorage = (EventsSource as TreeViewDataItemAutomationPeer).WeakRefElementProxyStorageCache; } } } /// void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); TreeViewItem treeViewItem = (TreeViewItem)Owner; if (!treeViewItem.HasItems) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } treeViewItem.IsExpanded = true; } /// void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); TreeViewItem treeViewItem = (TreeViewItem)Owner; if (!treeViewItem.HasItems) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } treeViewItem.IsExpanded = false; } ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { TreeViewItem treeViewItem = (TreeViewItem)Owner; if (treeViewItem.HasItems) return treeViewItem.IsExpanded ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; else return ExpandCollapseState.LeafNode; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { if (EventsSource as TreeViewDataItemAutomationPeer != null) { (EventsSource as TreeViewDataItemAutomationPeer).RaiseExpandCollapseAutomationEvent(oldValue, newValue); } else RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #region ISelectionItemProvider /// /// Selects this element, removing any other element from the selection. /// void ISelectionItemProvider.Select() { ((TreeViewItem)Owner).IsSelected = true; } ////// Selects this item. /// void ISelectionItemProvider.AddToSelection() { TreeView treeView = ((TreeViewItem)Owner).ParentTreeView; // If TreeView already has a selected item different from current - we cannot add to selection and throw if (treeView == null || (treeView.SelectedItem != null && treeView.SelectedContainer != Owner)) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } ((TreeViewItem)Owner).IsSelected = true; } ////// Unselects this item. /// void ISelectionItemProvider.RemoveFromSelection() { ((TreeViewItem)Owner).IsSelected = false; } ////// Returns whether the item is selected. /// bool ISelectionItemProvider.IsSelected { get { return ((TreeViewItem)Owner).IsSelected; } } ////// The logical element that supports the SelectionPattern for this item. /// IRawElementProviderSimple ISelectionItemProvider.SelectionContainer { get { ItemsControl parent = ((TreeViewItem)Owner).ParentItemsControl; if (parent != null) { AutomationPeer peer = UIElementAutomationPeer.FromElement(parent); if (peer != null) return ProviderFromPeer(peer); } return null; } } void IScrollItemProvider.ScrollIntoView() { ((TreeViewItem)Owner).BringIntoView(); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseAutomationIsSelectedChanged(bool isSelected) { if (EventsSource as TreeViewDataItemAutomationPeer != null) { (EventsSource as TreeViewDataItemAutomationPeer).RaiseAutomationIsSelectedChanged(isSelected); } else RaisePropertyChangedEvent( SelectionItemPatternIdentifiers.IsSelectedProperty, !isSelected, isSelected); } // Selection Events needs to be raised on DataItem Peers now when they exist. internal void RaiseAutomationSelectionEvent(AutomationEvents eventId) { if (EventsSource != null) { EventsSource.RaiseAutomationEvent(eventId); } else this.RaiseAutomationEvent(eventId); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class TreeViewItemAutomationPeer : ItemsControlAutomationPeer, IExpandCollapseProvider, ISelectionItemProvider, IScrollItemProvider { /// public TreeViewItemAutomationPeer(TreeViewItem owner): base(owner) { } /// override protected string GetClassNameCore() { return "TreeViewItem"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.TreeItem; } /// override protected bool IsOffscreenCore() { if (!Owner.IsVisible) return true; Rect boundingRect = CalculateVisibleBoundingRect(); return (boundingRect == Rect.Empty || boundingRect.Height == 0 || boundingRect.Width == 0); } /// override public object GetPattern(PatternInterface patternInterface) { if (patternInterface == PatternInterface.ExpandCollapse) { return this; } else if (patternInterface == PatternInterface.SelectionItem) { return this; } else if (patternInterface == PatternInterface.ScrollItem) { return this; } return base.GetPattern(patternInterface); } /// protected override ListGetChildrenCore() { List children = null; ItemPeersStorage oldChildren = ItemPeers; //cache the old ones for possible reuse ItemPeers = new ItemPeersStorage (); TreeViewItem owner = Owner as TreeViewItem; if (owner != null) { iterate(this, owner, (IteratorCallback)delegate(AutomationPeer peer) { if (children == null) children = new List (); children.Add(peer); return (false); }, ItemPeers, oldChildren); } return children; } private delegate bool IteratorCallback(AutomationPeer peer); // private static bool iterate(TreeViewItemAutomationPeer logicalParentAp, DependencyObject parent, IteratorCallback callback, ItemPeersStorage dataChildren, ItemPeersStorage oldChildren) { bool done = false; if (parent != null) { AutomationPeer peer = null; int count = VisualTreeHelper.GetChildrenCount(parent); for (int i = 0; i < count && !done; i++) { DependencyObject child = VisualTreeHelper.GetChild(parent, i); if (child != null && child is UIElement) { if (child is TreeViewItem) { object dataItem = (child as UIElement) != null ? (logicalParentAp.Owner as ItemsControl).GetItemOrContainerFromContainer(child as UIElement) : child; peer = oldChildren[dataItem]; if (peer == null) { peer = logicalParentAp.GetPeerFromWeakRefStorage(dataItem); if (peer != null) { // As cached peer is getting used it must be invalidated. peer.AncestorsInvalid = false; peer.ChildrenValid = false; } } if (peer == null) { peer = logicalParentAp.CreateItemAutomationPeer(dataItem); } //perform hookup so the events sourced from wrapper peer are fired as if from the data item if (peer != null) { AutomationPeer wrapperPeer = (peer as ItemAutomationPeer).GetWrapperPeer(); if (wrapperPeer != null) { wrapperPeer.EventsSource = peer; } if (dataChildren[dataItem] == null && peer is ItemAutomationPeer) { callback(peer); dataChildren[dataItem] = peer as ItemAutomationPeer; } } } else { peer = CreatePeerForElement((UIElement)child); if (peer != null) done = callback(peer); } if(peer == null) done = iterate(logicalParentAp, child, callback, dataChildren, oldChildren); } else { done = iterate(logicalParentAp, child, callback, dataChildren, oldChildren); } } } return done; } /// /// It returns the ItemAutomationPeer if it exist corresponding to the item otherwise it creates /// one and does add the Handle and parent info by calling TrySetParentInfo. /// ////// Security Critical - Calls a Security Critical operation /// SecurityTreatAsSafe - It's being called from this object which is real parent for the item peer. /// /// ///[SecurityCritical, SecurityTreatAsSafe] override internal ItemAutomationPeer FindOrCreateItemAutomationPeer(object item) { ItemAutomationPeer peer = ItemPeers[item]; AutomationPeer parentPeer = this; if (EventsSource as TreeViewDataItemAutomationPeer != null) { parentPeer = EventsSource as TreeViewDataItemAutomationPeer; } if (peer == null) peer = GetPeerFromWeakRefStorage(item); if (peer == null) { peer = CreateItemAutomationPeer(item); if(peer != null) peer.TrySetParentInfo(parentPeer); AutomationPeer wrapperPeer = (peer as ItemAutomationPeer).GetWrapperPeer(); if (wrapperPeer != null) { wrapperPeer.EventsSource = peer; } } return peer; } /// internal override bool IsPropertySupportedByControlForFindItem(int id) { if (base.IsPropertySupportedByControlForFindItem(id)) return true; else { if (SelectionItemPatternIdentifiers.IsSelectedProperty.Id == id) return true; else return false; } } /// /// Support for IsSelectedProperty should come from SelectorAutomationPeer only, /// internal override object GetSupportedPropertyValue(ItemAutomationPeer itemPeer, int propertyId) { if (SelectionItemPatternIdentifiers.IsSelectedProperty.Id == propertyId) { ISelectionItemProvider selectionItem = itemPeer.GetPattern(PatternInterface.SelectionItem) as ISelectionItemProvider; if (selectionItem != null) return selectionItem.IsSelected; else return null; } return base.GetSupportedPropertyValue(itemPeer, propertyId); } /// override protected ItemAutomationPeer CreateItemAutomationPeer(object item) { return new TreeViewDataItemAutomationPeer(item, this, EventsSource as TreeViewDataItemAutomationPeer); } // override internal void UpdateChildren() { // To ensure that the Updation of children should be initiated from DataPeer so as to have the right parent value stored for children TreeViewDataItemAutomationPeer dataPeer = EventsSource as TreeViewDataItemAutomationPeer; if(dataPeer != null) dataPeer.UpdateChildrenInternal(AutomationInteropProvider.ItemsInvalidateLimit); else UpdateChildrenInternal(AutomationInteropProvider.ItemsInvalidateLimit); WeakRefElementProxyStorage.PurgeWeakRefCollection(); } ///internal void AddDataPeerInfo(TreeViewDataItemAutomationPeer dataPeer) { EventsSource = dataPeer; UpdateWeakRefStorageFromDataPeer(); } /// internal void UpdateWeakRefStorageFromDataPeer() { // To use the already stored WeakRef collection of it's children Items which might be created when last time this item was realized. if(EventsSource as TreeViewDataItemAutomationPeer != null) { if((EventsSource as TreeViewDataItemAutomationPeer).WeakRefElementProxyStorageCache == null) (EventsSource as TreeViewDataItemAutomationPeer).WeakRefElementProxyStorageCache = WeakRefElementProxyStorage; else if(WeakRefElementProxyStorage.Count == 0) { WeakRefElementProxyStorage = (EventsSource as TreeViewDataItemAutomationPeer).WeakRefElementProxyStorageCache; } } } /// void IExpandCollapseProvider.Expand() { if(!IsEnabled()) throw new ElementNotEnabledException(); TreeViewItem treeViewItem = (TreeViewItem)Owner; if (!treeViewItem.HasItems) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } treeViewItem.IsExpanded = true; } /// void IExpandCollapseProvider.Collapse() { if(!IsEnabled()) throw new ElementNotEnabledException(); TreeViewItem treeViewItem = (TreeViewItem)Owner; if (!treeViewItem.HasItems) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } treeViewItem.IsExpanded = false; } ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { TreeViewItem treeViewItem = (TreeViewItem)Owner; if (treeViewItem.HasItems) return treeViewItem.IsExpanded ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; else return ExpandCollapseState.LeafNode; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { if (EventsSource as TreeViewDataItemAutomationPeer != null) { (EventsSource as TreeViewDataItemAutomationPeer).RaiseExpandCollapseAutomationEvent(oldValue, newValue); } else RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #region ISelectionItemProvider /// /// Selects this element, removing any other element from the selection. /// void ISelectionItemProvider.Select() { ((TreeViewItem)Owner).IsSelected = true; } ////// Selects this item. /// void ISelectionItemProvider.AddToSelection() { TreeView treeView = ((TreeViewItem)Owner).ParentTreeView; // If TreeView already has a selected item different from current - we cannot add to selection and throw if (treeView == null || (treeView.SelectedItem != null && treeView.SelectedContainer != Owner)) { throw new InvalidOperationException(SR.Get(SRID.UIA_OperationCannotBePerformed)); } ((TreeViewItem)Owner).IsSelected = true; } ////// Unselects this item. /// void ISelectionItemProvider.RemoveFromSelection() { ((TreeViewItem)Owner).IsSelected = false; } ////// Returns whether the item is selected. /// bool ISelectionItemProvider.IsSelected { get { return ((TreeViewItem)Owner).IsSelected; } } ////// The logical element that supports the SelectionPattern for this item. /// IRawElementProviderSimple ISelectionItemProvider.SelectionContainer { get { ItemsControl parent = ((TreeViewItem)Owner).ParentItemsControl; if (parent != null) { AutomationPeer peer = UIElementAutomationPeer.FromElement(parent); if (peer != null) return ProviderFromPeer(peer); } return null; } } void IScrollItemProvider.ScrollIntoView() { ((TreeViewItem)Owner).BringIntoView(); } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseAutomationIsSelectedChanged(bool isSelected) { if (EventsSource as TreeViewDataItemAutomationPeer != null) { (EventsSource as TreeViewDataItemAutomationPeer).RaiseAutomationIsSelectedChanged(isSelected); } else RaisePropertyChangedEvent( SelectionItemPatternIdentifiers.IsSelectedProperty, !isSelected, isSelected); } // Selection Events needs to be raised on DataItem Peers now when they exist. internal void RaiseAutomationSelectionEvent(AutomationEvents eventId) { if (EventsSource != null) { EventsSource.RaiseAutomationEvent(eventId); } else this.RaiseAutomationEvent(eventId); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
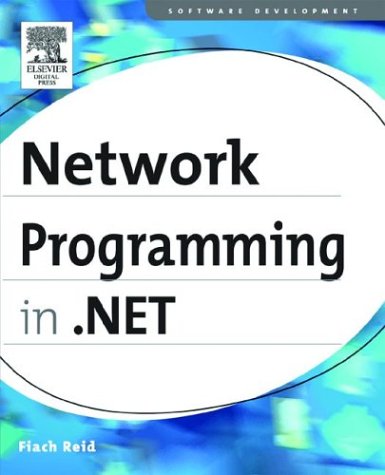
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GestureRecognizer.cs
- OletxDependentTransaction.cs
- BamlVersionHeader.cs
- unsafeIndexingFilterStream.cs
- NoneExcludedImageIndexConverter.cs
- HijriCalendar.cs
- DataListItemCollection.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- KeyFrames.cs
- Task.cs
- TextSearch.cs
- ToolStripDropDown.cs
- AuthenticationModulesSection.cs
- BuildManager.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- XmlRawWriter.cs
- FixedSOMPageConstructor.cs
- SqlDataSourceCommandParser.cs
- HttpResponseHeader.cs
- WebServiceTypeData.cs
- ResXFileRef.cs
- HwndKeyboardInputProvider.cs
- _DomainName.cs
- ArgumentOutOfRangeException.cs
- PerformanceCounterPermissionEntryCollection.cs
- MetadataFile.cs
- UIAgentMonitor.cs
- ByteFacetDescriptionElement.cs
- TypeResolvingOptions.cs
- AssemblyAttributes.cs
- GraphicsContext.cs
- RepeaterItemEventArgs.cs
- ExpressionLink.cs
- shaperfactory.cs
- XmlWriterDelegator.cs
- NamedPipeConnectionPoolSettingsElement.cs
- ListViewHitTestInfo.cs
- ViewKeyConstraint.cs
- StructuredTypeInfo.cs
- DatatypeImplementation.cs
- WindowsEditBox.cs
- ExceptionUtil.cs
- FrameworkElement.cs
- NamespaceList.cs
- PrivilegeNotHeldException.cs
- DataGridViewComboBoxColumnDesigner.cs
- VariableModifiersHelper.cs
- RadioButtonPopupAdapter.cs
- DataGridTableCollection.cs
- DataObject.cs
- QilValidationVisitor.cs
- FormViewModeEventArgs.cs
- FixedTextContainer.cs
- StorageConditionPropertyMapping.cs
- OdbcRowUpdatingEvent.cs
- ProxyWebPart.cs
- DbConnectionFactory.cs
- AncillaryOps.cs
- X509Utils.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- RequestBringIntoViewEventArgs.cs
- EntitySqlQueryBuilder.cs
- RenderContext.cs
- StorageMappingItemCollection.cs
- HttpApplicationFactory.cs
- CatalogZoneBase.cs
- DoubleIndependentAnimationStorage.cs
- XmlBoundElement.cs
- PropertyValueUIItem.cs
- RuntimeResourceSet.cs
- CatalogZoneBase.cs
- PauseStoryboard.cs
- DuplicateWaitObjectException.cs
- AutomationElementCollection.cs
- DataGridViewColumnCollection.cs
- MediaContext.cs
- PackageRelationshipCollection.cs
- DataBoundControlAdapter.cs
- MimeTypeMapper.cs
- VersionConverter.cs
- ListViewItem.cs
- InternalException.cs
- DetailsViewUpdateEventArgs.cs
- FragmentQueryKB.cs
- ContentElement.cs
- COM2Properties.cs
- SafeThreadHandle.cs
- MenuItem.cs
- SerializationHelper.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- GridToolTip.cs
- WindowAutomationPeer.cs
- FontNamesConverter.cs
- RoleManagerSection.cs
- WorkflowServiceAttributes.cs
- NavigationHelper.cs
- PublishLicense.cs
- TemplateControlBuildProvider.cs
- PersonalizationState.cs
- Qualifier.cs