Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / Accessible.cs / 1 / Accessible.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Wraps some of IAccessible to support Focus and top-level window creates // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Automation; using System; using Accessibility; using System.Text; using System.Diagnostics; using MS.Win32; // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace MS.Internal.Automation { internal class Accessible { internal Accessible(IAccessible acc, object child) { Debug.Assert(acc != null, "null IAccessible"); _acc = acc; _child = child; } // Create an Accessible object; may return null internal static Accessible Create( IntPtr hwnd, int idObject, int idChild ) { IAccessible acc = null; object child = null; if( UnsafeNativeMethods.AccessibleObjectFromEvent( hwnd, idObject, idChild, ref acc, ref child ) != 0 /*S_OK*/ || acc == null ) return null; // Per SDK must use the ppacc and pvarChild from AccessibleObjectFromEvent // to access information about this UI element. return new Accessible( acc, child ); } internal int State { get { try { return (int)_acc.get_accState(_child); } catch (Exception e) { if (IsCriticalMSAAException(e)) // PRESHARP will flag this as a warning 56503/6503: Property get methods should not throw exceptions // Since this Property is internal, we CAN throw an exception #pragma warning suppress 6503 throw; return UnsafeNativeMethods.STATE_SYSTEM_UNAVAILABLE; } } } internal IntPtr Window { get { if (_hwnd == IntPtr.Zero) { try { if (UnsafeNativeMethods.WindowFromAccessibleObject(_acc, ref _hwnd) != 0/*S_OK*/) { _hwnd = IntPtr.Zero; } } catch( Exception e ) { if (IsCriticalMSAAException(e)) // PRESHARP will flag this as a warning 56503/6503: Property get methods should not throw exceptions // Since this Property is internal, we CAN throw an exception #pragma warning suppress 6503 throw; _hwnd = IntPtr.Zero; } } return _hwnd; } } // miscellaneous functions used with Accessible internal static bool CompareClass(IntPtr hwnd, string szClass) { return ProxyManager.GetClassName(NativeMethods.HWND.Cast(hwnd)) == szClass; } internal static bool IsComboDropdown(IntPtr hwnd) { return CompareClass(hwnd, "ComboLBox"); } internal static bool IsStatic(IntPtr hwnd) { return CompareClass(hwnd, "Static"); } private bool IsCriticalMSAAException(Exception e) { // Some OLEACC proxies produce out-of-memory for non-critical reasons: // notably, the treeview proxy will raise this if the target HWND no longer exists, // GetWindowThreadProcessID fails and it therefore won't be able to allocate shared // memory in the target process, so it incorrectly assumes OOM. // Some Native impls (media player) return E_POINTER, which COM Interop translates // into NullRefException; need to ignore those too. // should ignore those return !(e is OutOfMemoryException) && !(e is NullReferenceException) && Misc.IsCriticalException(e); } private IntPtr _hwnd; private IAccessible _acc; private Object _child; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Wraps some of IAccessible to support Focus and top-level window creates // // History: // 06/02/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System.Windows.Automation; using System; using Accessibility; using System.Text; using System.Diagnostics; using MS.Win32; // PRESHARP: In order to avoid generating warnings about unkown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace MS.Internal.Automation { internal class Accessible { internal Accessible(IAccessible acc, object child) { Debug.Assert(acc != null, "null IAccessible"); _acc = acc; _child = child; } // Create an Accessible object; may return null internal static Accessible Create( IntPtr hwnd, int idObject, int idChild ) { IAccessible acc = null; object child = null; if( UnsafeNativeMethods.AccessibleObjectFromEvent( hwnd, idObject, idChild, ref acc, ref child ) != 0 /*S_OK*/ || acc == null ) return null; // Per SDK must use the ppacc and pvarChild from AccessibleObjectFromEvent // to access information about this UI element. return new Accessible( acc, child ); } internal int State { get { try { return (int)_acc.get_accState(_child); } catch (Exception e) { if (IsCriticalMSAAException(e)) // PRESHARP will flag this as a warning 56503/6503: Property get methods should not throw exceptions // Since this Property is internal, we CAN throw an exception #pragma warning suppress 6503 throw; return UnsafeNativeMethods.STATE_SYSTEM_UNAVAILABLE; } } } internal IntPtr Window { get { if (_hwnd == IntPtr.Zero) { try { if (UnsafeNativeMethods.WindowFromAccessibleObject(_acc, ref _hwnd) != 0/*S_OK*/) { _hwnd = IntPtr.Zero; } } catch( Exception e ) { if (IsCriticalMSAAException(e)) // PRESHARP will flag this as a warning 56503/6503: Property get methods should not throw exceptions // Since this Property is internal, we CAN throw an exception #pragma warning suppress 6503 throw; _hwnd = IntPtr.Zero; } } return _hwnd; } } // miscellaneous functions used with Accessible internal static bool CompareClass(IntPtr hwnd, string szClass) { return ProxyManager.GetClassName(NativeMethods.HWND.Cast(hwnd)) == szClass; } internal static bool IsComboDropdown(IntPtr hwnd) { return CompareClass(hwnd, "ComboLBox"); } internal static bool IsStatic(IntPtr hwnd) { return CompareClass(hwnd, "Static"); } private bool IsCriticalMSAAException(Exception e) { // Some OLEACC proxies produce out-of-memory for non-critical reasons: // notably, the treeview proxy will raise this if the target HWND no longer exists, // GetWindowThreadProcessID fails and it therefore won't be able to allocate shared // memory in the target process, so it incorrectly assumes OOM. // Some Native impls (media player) return E_POINTER, which COM Interop translates // into NullRefException; need to ignore those too. // should ignore those return !(e is OutOfMemoryException) && !(e is NullReferenceException) && Misc.IsCriticalException(e); } private IntPtr _hwnd; private IAccessible _acc; private Object _child; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
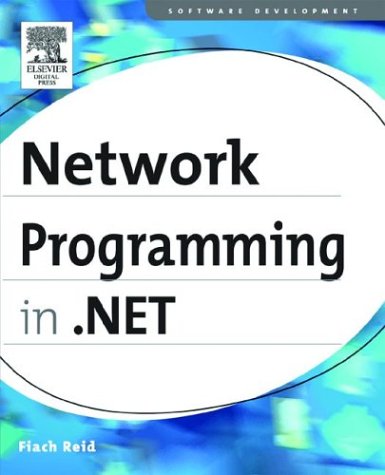
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DateTime.cs
- DelayedRegex.cs
- DbConnectionPool.cs
- DesignerEventService.cs
- PathData.cs
- ProcessHostConfigUtils.cs
- Point3DValueSerializer.cs
- ZoneButton.cs
- ServicePoint.cs
- FieldDescriptor.cs
- SafeTokenHandle.cs
- TaiwanLunisolarCalendar.cs
- CompilationLock.cs
- FontSource.cs
- TCPListener.cs
- DataGridViewColumnEventArgs.cs
- KeyInstance.cs
- CodeArgumentReferenceExpression.cs
- NotCondition.cs
- NonSerializedAttribute.cs
- CfgParser.cs
- PingReply.cs
- XmlSerializableReader.cs
- Lease.cs
- Trigger.cs
- FormViewRow.cs
- RowUpdatingEventArgs.cs
- StrokeCollectionConverter.cs
- Page.cs
- ControlParameter.cs
- Pair.cs
- URLIdentityPermission.cs
- IxmlLineInfo.cs
- CheckBoxRenderer.cs
- WinFormsComponentEditor.cs
- SmiEventSink_Default.cs
- RIPEMD160.cs
- Not.cs
- BinaryUtilClasses.cs
- PathSegmentCollection.cs
- TextSpanModifier.cs
- DemultiplexingClientMessageFormatter.cs
- FreezableDefaultValueFactory.cs
- DecoderNLS.cs
- SmtpLoginAuthenticationModule.cs
- DataGridViewRowCancelEventArgs.cs
- EventArgs.cs
- DataGridDetailsPresenter.cs
- TemplateControl.cs
- TextViewSelectionProcessor.cs
- ScriptReference.cs
- DbConnectionPoolGroup.cs
- Rect.cs
- ProbeMatchesMessage11.cs
- MobileFormsAuthentication.cs
- AsyncPostBackErrorEventArgs.cs
- PartialClassGenerationTaskInternal.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- XmlReflectionMember.cs
- SqlHelper.cs
- TypeNameParser.cs
- OrderByQueryOptionExpression.cs
- DataGridViewRowCancelEventArgs.cs
- UserControl.cs
- DesignerActionService.cs
- SafeHandle.cs
- SessionStateModule.cs
- AmbiguousMatchException.cs
- EmptyCollection.cs
- Brush.cs
- WebBrowsableAttribute.cs
- VectorConverter.cs
- HealthMonitoringSectionHelper.cs
- ClearTypeHintValidation.cs
- InputChannelBinder.cs
- Geometry.cs
- FontStretches.cs
- FixUp.cs
- ResponseStream.cs
- GraphicsPathIterator.cs
- CodeBlockBuilder.cs
- PrePrepareMethodAttribute.cs
- WebScriptMetadataFormatter.cs
- Transactions.cs
- XmlSchemaAttribute.cs
- MessageDecoder.cs
- ContainerControl.cs
- MetadataItemEmitter.cs
- UseManagedPresentationBindingElement.cs
- MarkupCompilePass1.cs
- Rect3D.cs
- MemberMaps.cs
- MouseGesture.cs
- selecteditemcollection.cs
- AutomationProperty.cs
- DocumentApplication.cs
- SR.cs
- UseLicense.cs
- SingleKeyFrameCollection.cs
- SQLByteStorage.cs