Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Dispatcher / InputChannelBinder.cs / 1 / InputChannelBinder.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Dispatcher { using System; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Diagnostics; class InputChannelBinder : IChannelBinder { IInputChannel channel; Uri listenUri; internal InputChannelBinder(IInputChannel channel, Uri listenUri) { if (!((channel != null))) { DiagnosticUtility.DebugAssert("InputChannelBinder.InputChannelBinder: (channel != null)"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("channel"); } this.channel = channel; this.listenUri = listenUri; } public IChannel Channel { get { return this.channel; } } public bool HasSession { get { return this.channel is ISessionChannel; } } public Uri ListenUri { get { return this.listenUri; } } public EndpointAddress LocalAddress { get { return this.channel.LocalAddress; } } public EndpointAddress RemoteAddress { get { #pragma warning suppress 56503 // [....], the property is really not implemented, cannot lie, API not public throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } } public void Abort() { this.channel.Abort(); } public void CloseAfterFault(TimeSpan timeout) { this.channel.Close(timeout); } public IAsyncResult BeginTryReceive(TimeSpan timeout, AsyncCallback callback, object state) { return this.channel.BeginTryReceive(timeout, callback, state); } public bool EndTryReceive(IAsyncResult result, out RequestContext requestContext) { Message message; if (this.channel.EndTryReceive(result, out message)) { requestContext = this.WrapMessage(message); return true; } else { requestContext = null; return false; } } public IAsyncResult BeginSend(Message message, TimeSpan timeout, AsyncCallback callback, object state) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public void EndSend(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public void Send(Message message, TimeSpan timeout) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public bool TryReceive(TimeSpan timeout, out RequestContext requestContext) { Message message; if (this.channel.TryReceive(timeout, out message)) { requestContext = this.WrapMessage(message); return true; } else { requestContext = null; return false; } } public IAsyncResult BeginRequest(Message message, TimeSpan timeout, AsyncCallback callback, object state) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public Message EndRequest(IAsyncResult result) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotImplementedException()); } public Message Request(Message message, TimeSpan timeout) { throw TraceUtility.ThrowHelperError(new NotImplementedException(), message); } public bool WaitForMessage(TimeSpan timeout) { return this.channel.WaitForMessage(timeout); } public IAsyncResult BeginWaitForMessage(TimeSpan timeout, AsyncCallback callback, object state) { return this.channel.BeginWaitForMessage(timeout, callback, state); } public bool EndWaitForMessage(IAsyncResult result) { return this.channel.EndWaitForMessage(result); } RequestContext WrapMessage(Message message) { if (message == null) { return null; } else { return new InputRequestContext(message, this); } } class InputRequestContext : RequestContextBase { InputChannelBinder binder; internal InputRequestContext(Message request, InputChannelBinder binder) : base(request, TimeSpan.Zero, TimeSpan.Zero) { this.binder = binder; } protected override void OnAbort() { } protected override void OnClose(TimeSpan timeout) { } protected override void OnReply(Message message, TimeSpan timeout) { } protected override IAsyncResult OnBeginReply(Message message, TimeSpan timeout, AsyncCallback callback, object state) { return new CompletedAsyncResult(callback, state); } protected override void OnEndReply(IAsyncResult result) { CompletedAsyncResult.End(result); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
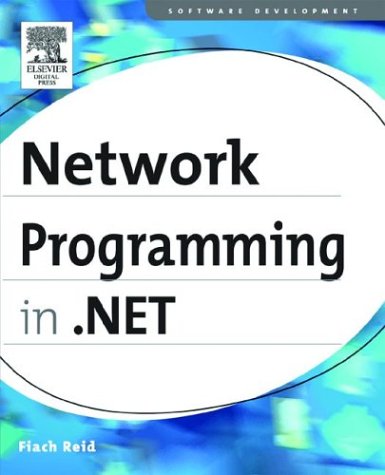
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SchemaTableOptionalColumn.cs
- SqlResolver.cs
- StringFormat.cs
- PictureBox.cs
- DataGridViewRowStateChangedEventArgs.cs
- TextRangeBase.cs
- TriState.cs
- Attachment.cs
- DetailsViewCommandEventArgs.cs
- MouseWheelEventArgs.cs
- GridPattern.cs
- BuildDependencySet.cs
- TextParaClient.cs
- BaseDataListActionList.cs
- TextOnlyOutput.cs
- TextTreeRootNode.cs
- LabelAutomationPeer.cs
- EventWaitHandle.cs
- HttpClientChannel.cs
- SkinBuilder.cs
- FontInfo.cs
- TaskFileService.cs
- FixedDocumentSequencePaginator.cs
- ParameterCollection.cs
- RecognitionResult.cs
- SiteMapNodeItem.cs
- ViewStateModeByIdAttribute.cs
- StateRuntime.cs
- InvalidateEvent.cs
- IteratorFilter.cs
- Container.cs
- DesignTimeHTMLTextWriter.cs
- Geometry.cs
- XmlChildEnumerator.cs
- XmlNamespaceDeclarationsAttribute.cs
- DispatcherExceptionEventArgs.cs
- ContentTextAutomationPeer.cs
- IndexedString.cs
- ConstrainedDataObject.cs
- EventDescriptorCollection.cs
- Int32RectValueSerializer.cs
- DBSchemaTable.cs
- NamedObject.cs
- SchemaType.cs
- PointKeyFrameCollection.cs
- EntityDataSourceWrapper.cs
- RSACryptoServiceProvider.cs
- XamlFrame.cs
- ReadingWritingEntityEventArgs.cs
- UpdateManifestForBrowserApplication.cs
- HashMembershipCondition.cs
- CompensationExtension.cs
- ClientType.cs
- XmlCustomFormatter.cs
- BitmapMetadataBlob.cs
- Pointer.cs
- TraceHandlerErrorFormatter.cs
- DataServiceExpressionVisitor.cs
- FileDialogCustomPlacesCollection.cs
- SqlBulkCopyColumnMappingCollection.cs
- MimePart.cs
- FlagsAttribute.cs
- LabelDesigner.cs
- Cursor.cs
- BuildResult.cs
- UserMapPath.cs
- ByteRangeDownloader.cs
- CompatibleComparer.cs
- GroupQuery.cs
- DataControlFieldHeaderCell.cs
- DispatcherOperation.cs
- DataGrid.cs
- WsatServiceAddress.cs
- DrawingImage.cs
- SqlCacheDependencySection.cs
- FactoryGenerator.cs
- ToolStripLabel.cs
- XPathEmptyIterator.cs
- XmlSchemaAttributeGroupRef.cs
- CalendarDay.cs
- XmlSchemaInfo.cs
- SmiEventSink_DeferedProcessing.cs
- NetworkInformationException.cs
- XmlDataSourceNodeDescriptor.cs
- StorageFunctionMapping.cs
- DataGridRowHeaderAutomationPeer.cs
- LoadedOrUnloadedOperation.cs
- XmlNavigatorStack.cs
- LogLogRecordHeader.cs
- TextBoxBase.cs
- SmtpDigestAuthenticationModule.cs
- SignerInfo.cs
- PlatformCulture.cs
- WindowInteractionStateTracker.cs
- BreakRecordTable.cs
- UnsafeNativeMethods.cs
- HtmlTableRow.cs
- JsonUriDataContract.cs
- DataGridViewRowPrePaintEventArgs.cs
- CodeActivity.cs