Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Map / Update / Internal / RecordConverter.cs / 2 / RecordConverter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Collections.Generic; using System.Data.Common.Utils; using System.Collections; using System.Data.Entity; namespace System.Data.Mapping.Update.Internal { ////// Converts records to new instance expressions. Assumes that all inputs come from a single data reader (because /// it caches record layout). If multiple readers are used, multiple converters must be constructed in case /// the different readers return different layouts for types. /// ////// Conventions for modifiedProperties enumeration: null means all properties are modified, empty means none, /// non-empty means some. /// internal class RecordConverter { #region Constructors ////// Initializes a new converter given a command tree context. Initializes a new record layout cache. /// /// Setsinternal RecordConverter(UpdateTranslator updateTranslator) { m_updateTranslator = updateTranslator; } #endregion #region Fields /// /// Context used to produce expressions. /// private UpdateTranslator m_updateTranslator; #endregion #region Methods ////// Converts original values in a state entry to a DbNewInstanceExpression. The record must be either an entity or /// a relationship set instance. /// ////// This method is not thread safe. /// /// Gets state entry this record is associated with. /// A list of modified properties. See remarks on this class for details. ///New instance expression. internal PropagatorResult ConvertOriginalValuesToPropagatorResult(IEntityStateEntry stateEntry, IEnumerablemodifiedProperties) { return ConvertStateEntryToPropagatorResult(stateEntry, modifiedProperties, true /*useOriginalValues*/); } /// /// Converts current values in a state entry to a DbNewInstanceExpression. The record must be either an entity or /// a relationship set instance. /// ////// This method is not thread safe. /// /// Gets state entry this record is associated with. /// A list of modified properties. See remarks on this class for details. ///New instance expression. internal PropagatorResult ConvertCurrentValuesToPropagatorResult(IEntityStateEntry stateEntry, IEnumerablemodifiedProperties) { return ConvertStateEntryToPropagatorResult(stateEntry, modifiedProperties, false /*useOriginalValues*/); } private PropagatorResult ConvertStateEntryToPropagatorResult(IEntityStateEntry stateEntry, IEnumerable modifiedProperties, bool useOriginalValues) { try { EntityUtil.CheckArgumentNull(stateEntry, "stateEntry"); IExtendedDataRecord record = useOriginalValues ? EntityUtil.CheckArgumentNull(stateEntry.OriginalValues as IExtendedDataRecord, "stateEntry.OriginalValues") : EntityUtil.CheckArgumentNull(stateEntry.CurrentValues as IExtendedDataRecord, "stateEntry.CurrentValues"); bool isModified = false; // the root of the state entry is unchanged because the type is static return ExtractorMetadata.ExtractResultFromRecord(stateEntry, isModified, record, modifiedProperties, m_updateTranslator); } catch (Exception e) { if (UpdateTranslator.RequiresContext(e)) { throw EntityUtil.Update(Strings.Update_ErrorLoadingRecord, e, stateEntry); } throw; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Collections.Generic; using System.Data.Common.Utils; using System.Collections; using System.Data.Entity; namespace System.Data.Mapping.Update.Internal { ////// Converts records to new instance expressions. Assumes that all inputs come from a single data reader (because /// it caches record layout). If multiple readers are used, multiple converters must be constructed in case /// the different readers return different layouts for types. /// ////// Conventions for modifiedProperties enumeration: null means all properties are modified, empty means none, /// non-empty means some. /// internal class RecordConverter { #region Constructors ////// Initializes a new converter given a command tree context. Initializes a new record layout cache. /// /// Setsinternal RecordConverter(UpdateTranslator updateTranslator) { m_updateTranslator = updateTranslator; } #endregion #region Fields /// /// Context used to produce expressions. /// private UpdateTranslator m_updateTranslator; #endregion #region Methods ////// Converts original values in a state entry to a DbNewInstanceExpression. The record must be either an entity or /// a relationship set instance. /// ////// This method is not thread safe. /// /// Gets state entry this record is associated with. /// A list of modified properties. See remarks on this class for details. ///New instance expression. internal PropagatorResult ConvertOriginalValuesToPropagatorResult(IEntityStateEntry stateEntry, IEnumerablemodifiedProperties) { return ConvertStateEntryToPropagatorResult(stateEntry, modifiedProperties, true /*useOriginalValues*/); } /// /// Converts current values in a state entry to a DbNewInstanceExpression. The record must be either an entity or /// a relationship set instance. /// ////// This method is not thread safe. /// /// Gets state entry this record is associated with. /// A list of modified properties. See remarks on this class for details. ///New instance expression. internal PropagatorResult ConvertCurrentValuesToPropagatorResult(IEntityStateEntry stateEntry, IEnumerablemodifiedProperties) { return ConvertStateEntryToPropagatorResult(stateEntry, modifiedProperties, false /*useOriginalValues*/); } private PropagatorResult ConvertStateEntryToPropagatorResult(IEntityStateEntry stateEntry, IEnumerable modifiedProperties, bool useOriginalValues) { try { EntityUtil.CheckArgumentNull(stateEntry, "stateEntry"); IExtendedDataRecord record = useOriginalValues ? EntityUtil.CheckArgumentNull(stateEntry.OriginalValues as IExtendedDataRecord, "stateEntry.OriginalValues") : EntityUtil.CheckArgumentNull(stateEntry.CurrentValues as IExtendedDataRecord, "stateEntry.CurrentValues"); bool isModified = false; // the root of the state entry is unchanged because the type is static return ExtractorMetadata.ExtractResultFromRecord(stateEntry, isModified, record, modifiedProperties, m_updateTranslator); } catch (Exception e) { if (UpdateTranslator.RequiresContext(e)) { throw EntityUtil.Update(Strings.Update_ErrorLoadingRecord, e, stateEntry); } throw; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
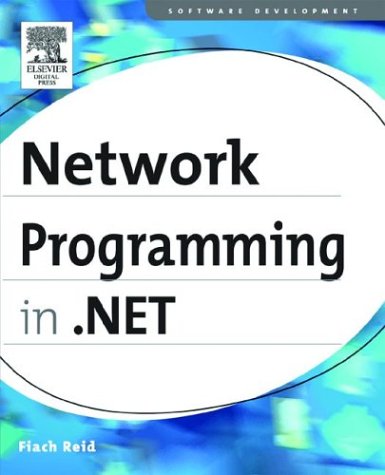
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LinkLabel.cs
- ListContractAdapter.cs
- ArrangedElementCollection.cs
- MdImport.cs
- Matrix3DStack.cs
- DocobjHost.cs
- WebPartConnectionsCancelEventArgs.cs
- DesignerFrame.cs
- ProcessHostServerConfig.cs
- EmbeddedMailObject.cs
- FilterEventArgs.cs
- TextDecorationCollection.cs
- ApplicationInfo.cs
- WSFederationHttpBindingElement.cs
- DataSourceDesigner.cs
- DocobjHost.cs
- CompilationUtil.cs
- WebServiceHandler.cs
- StringToken.cs
- KeyFrames.cs
- HtmlListAdapter.cs
- TypeDelegator.cs
- RowUpdatedEventArgs.cs
- TextViewBase.cs
- AlternationConverter.cs
- ItemsPresenter.cs
- ComboBoxDesigner.cs
- KeyValueConfigurationCollection.cs
- NativeMethods.cs
- VerificationException.cs
- HostedBindingBehavior.cs
- LayoutTable.cs
- ResizeGrip.cs
- FactoryId.cs
- InfocardExtendedInformationEntry.cs
- WeakReference.cs
- XmlRawWriter.cs
- XmlNodeComparer.cs
- ColorConvertedBitmapExtension.cs
- FileSystemWatcher.cs
- SecurityKeyType.cs
- ReflectPropertyDescriptor.cs
- PairComparer.cs
- DataSource.cs
- TextControlDesigner.cs
- HideDisabledControlAdapter.cs
- ISessionStateStore.cs
- PreviewPageInfo.cs
- WSTrustFeb2005.cs
- EdmItemCollection.cs
- StringKeyFrameCollection.cs
- DocumentAutomationPeer.cs
- ModelFunctionTypeElement.cs
- XmlnsDefinitionAttribute.cs
- DesignerSerializationOptionsAttribute.cs
- TreeWalkHelper.cs
- InkCanvasAutomationPeer.cs
- BasePattern.cs
- WebHttpSecurity.cs
- ViewStateException.cs
- ColumnBinding.cs
- FirewallWrapper.cs
- XmlSchemaExternal.cs
- SiteMapProvider.cs
- ReferencedAssembly.cs
- MiniMapControl.xaml.cs
- DataGridViewRowPrePaintEventArgs.cs
- CompressStream.cs
- PeerInputChannelListener.cs
- LineServices.cs
- StrokeDescriptor.cs
- Thread.cs
- WindowsButton.cs
- GlyphInfoList.cs
- PageContentAsyncResult.cs
- InputDevice.cs
- validationstate.cs
- DoWorkEventArgs.cs
- EntityTypeEmitter.cs
- TransactionContext.cs
- CodeNamespaceImportCollection.cs
- AnnouncementInnerClientCD1.cs
- TextEditorCopyPaste.cs
- HwndAppCommandInputProvider.cs
- DefaultBindingPropertyAttribute.cs
- PropertyDescriptorGridEntry.cs
- VarInfo.cs
- RootBrowserWindowProxy.cs
- NonClientArea.cs
- Metadata.cs
- LocationUpdates.cs
- DataGridViewCellStyleChangedEventArgs.cs
- PropertyDescriptorComparer.cs
- DeclarativeCatalogPart.cs
- EmbeddedObject.cs
- SqlBulkCopyColumnMappingCollection.cs
- DataGridTextBox.cs
- Span.cs
- SourceChangedEventArgs.cs
- BinaryCommonClasses.cs