Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / ColorConvertedBitmapExtension.cs / 1305600 / ColorConvertedBitmapExtension.cs
/****************************************************************************\ * * File: ColorConvertedBitmapExtension.cs * * Class for Xaml markup extension for static resource references. * * Copyright (C) 2004 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.IO; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Documents; using System.Windows.Markup; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Reflection; using MS.Internal; namespace System.Windows { ////// Class for Xaml markup extension for ColorConvertedBitmap with non-embedded profile. /// [MarkupExtensionReturnType(typeof(ColorConvertedBitmap))] public class ColorConvertedBitmapExtension : MarkupExtension { ////// Constructor that takes no parameters /// public ColorConvertedBitmapExtension() { } ////// Constructor that takes the markup for a "{ColorConvertedBitmap image source.icc destination.icc}" /// public ColorConvertedBitmapExtension( object image) { if (image == null) { throw new ArgumentNullException("image"); } string[] tokens = ((string)image).Split(new char[] { ' ' }); foreach (string str in tokens) { if (str.Length > 0) { if (_image == null) { _image = str; } else if (_sourceProfile == null) { _sourceProfile = str; } else if (_destinationProfile == null) { _destinationProfile = str; } else { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionSyntax)); } } } } ////// Return an object that should be set on the targetObject's targetProperty /// for this markup extension. For ColorConvertedBitmapExtension, this is the object found in /// a resource dictionary in the current parent chain that is keyed by ResourceKey /// ////// The object to set on this property. /// public override object ProvideValue(IServiceProvider serviceProvider) { if (_image == null) { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionNoSourceImage)); } if (_sourceProfile == null) { throw new InvalidOperationException(SR.Get(SRID.ColorConvertedBitmapExtensionNoSourceProfile)); } // [BreakingChange] Dev10 Bug #454108 // We really should throw an ArgumentNullException here for serviceProvider. // Save away the BaseUri. IUriContext uriContext = serviceProvider.GetService(typeof(IUriContext)) as IUriContext; if( uriContext == null ) { throw new InvalidOperationException(SR.Get(SRID.MarkupExtensionNoContext, GetType().Name, "IUriContext" )); } _baseUri = uriContext.BaseUri; Uri imageUri = GetResolvedUri(_image); Uri sourceProfileUri = GetResolvedUri(_sourceProfile); Uri destinationProfileUri = GetResolvedUri(_destinationProfile); ColorContext sourceContext = new ColorContext(sourceProfileUri); ColorContext destinationContext = destinationProfileUri != null ? new ColorContext(destinationProfileUri) : new ColorContext(PixelFormats.Default); BitmapDecoder decoder = BitmapDecoder.Create( imageUri, BitmapCreateOptions.IgnoreColorProfile | BitmapCreateOptions.IgnoreImageCache, BitmapCacheOption.None ); BitmapSource bitmap = decoder.Frames[0]; FormatConvertedBitmap formatConverted = new FormatConvertedBitmap(bitmap, PixelFormats.Bgra32, null, 0.0); object result = formatConverted; try { ColorConvertedBitmap colorConverted = new ColorConvertedBitmap(formatConverted, sourceContext, destinationContext, PixelFormats.Bgra32); result= colorConverted; } catch (FileFormatException) { // Gracefully ignore non-matching profile // If the file contains a bad color context, we catch the exception here // since color transform isn't possible // with the given color context. } return result; } private Uri GetResolvedUri(string uri) { if (uri == null) { return null; } return new Uri(_baseUri,uri); } string _image; string _sourceProfile; Uri _baseUri; string _destinationProfile; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
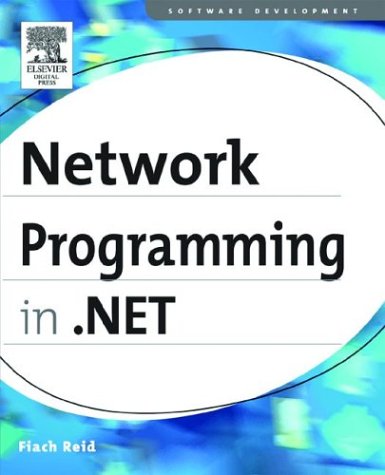
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeDomLoader.cs
- TemplateContainer.cs
- Types.cs
- OleDbEnumerator.cs
- HttpHandlerAction.cs
- EventArgs.cs
- DelegateInArgument.cs
- Style.cs
- LogPolicy.cs
- ChangeProcessor.cs
- ImagingCache.cs
- XmlSchemaCollection.cs
- util.cs
- ObservableCollection.cs
- WebControlsSection.cs
- UnsafeNativeMethods.cs
- ViewGenerator.cs
- SubclassTypeValidatorAttribute.cs
- DbParameterCollection.cs
- DES.cs
- ActivityDesignerResources.cs
- NotifyParentPropertyAttribute.cs
- GenericTextProperties.cs
- Typeface.cs
- Rectangle.cs
- ItemCheckEvent.cs
- DataListItemCollection.cs
- UserPrincipalNameElement.cs
- SingletonConnectionReader.cs
- odbcmetadatacolumnnames.cs
- PriorityRange.cs
- basenumberconverter.cs
- SetterBaseCollection.cs
- TransactedBatchingElement.cs
- XmlCodeExporter.cs
- CapabilitiesUse.cs
- ConsoleCancelEventArgs.cs
- ConnectionConsumerAttribute.cs
- NotImplementedException.cs
- EntityKeyElement.cs
- ComponentConverter.cs
- Throw.cs
- TextSpanModifier.cs
- SQLInt32.cs
- SqlDependencyUtils.cs
- Vector3D.cs
- OutputCacheProviderCollection.cs
- SchemaRegistration.cs
- CfgArc.cs
- RouteTable.cs
- PointLightBase.cs
- InfoCardTraceRecord.cs
- GridViewEditEventArgs.cs
- TraceUtils.cs
- CallSite.cs
- SortExpressionBuilder.cs
- CatalogPartCollection.cs
- LinearKeyFrames.cs
- GeneratedContractType.cs
- CurrentChangingEventArgs.cs
- documentation.cs
- ComboBoxAutomationPeer.cs
- XPathNodeIterator.cs
- ContractSearchPattern.cs
- BitmapFrameEncode.cs
- NavigatorInput.cs
- Processor.cs
- EventMappingSettingsCollection.cs
- FlowDocumentFormatter.cs
- InvalidWMPVersionException.cs
- OleDbInfoMessageEvent.cs
- TextViewBase.cs
- StyleSheetComponentEditor.cs
- HttpWriter.cs
- IisTraceWebEventProvider.cs
- Literal.cs
- DataSysAttribute.cs
- NotSupportedException.cs
- MarshalByValueComponent.cs
- HitTestWithPointDrawingContextWalker.cs
- Grant.cs
- CodeActivityContext.cs
- SerializationInfo.cs
- DataBoundLiteralControl.cs
- DataGridRelationshipRow.cs
- AspCompat.cs
- BufferedWebEventProvider.cs
- AdPostCacheSubstitution.cs
- StringFreezingAttribute.cs
- FacetValueContainer.cs
- IntegerCollectionEditor.cs
- RunClient.cs
- ConnectionsZone.cs
- Dynamic.cs
- AlternationConverter.cs
- PEFileReader.cs
- WebBrowserNavigatingEventHandler.cs
- BidPrivateBase.cs
- TransactionManager.cs
- columnmapkeybuilder.cs