Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / MS / Internal / FreezableDefaultValueFactory.cs / 1 / FreezableDefaultValueFactory.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DefaultvalueFactory for Freezables // // History: // 2005/11/08 : jordanpa - Created from PresentationCore's old // MutableDefaultPropertyMetadata // //--------------------------------------------------------------------------- using MS.Internal.WindowsBase; using System; using System.Diagnostics; using System.Windows; namespace MS.Internal { //// FreezableDefaultValueFactory is a DefaultValueFactory implementation which // is inserted by the property system for any DP registered with a default // value of type Freezable. The user’s given default value is frozen and // used as a template to create unfrozen copies on a per DP per DO basis. If // the default value is modified it is automatically promoted from default to // local. // [FriendAccessAllowed] // built into Base, used by Core + Framework internal class FreezableDefaultValueFactory : DefaultValueFactory { ////// Stores a frozen copy of defaultValue /// internal FreezableDefaultValueFactory(Freezable defaultValue) { Debug.Assert(defaultValue != null, "Null can not be made mutable. Do not use FreezableDefaultValueFactory."); Debug.Assert(defaultValue.CanFreeze, "The defaultValue prototype must be freezable."); _defaultValuePrototype = defaultValue.GetAsFrozen(); } ////// Returns our frozen sentinel /// internal override object DefaultValue { get { Debug.Assert(_defaultValuePrototype.IsFrozen); return _defaultValuePrototype; } } ////// If the DO is frozen, we'll return our frozen sentinel. Otherwise we'll make /// an unfrozen copy. /// internal override object CreateDefaultValue(DependencyObject owner, DependencyProperty property) { Debug.Assert(owner != null && property != null, "It is the caller responsibility to ensure that owner and property are non-null."); Freezable result = _defaultValuePrototype; Freezable ownerFreezable = owner as Freezable; // If the owner is frozen, just return the frozen prototype. if (ownerFreezable != null && ownerFreezable.IsFrozen) { return result; } result = _defaultValuePrototype.Clone(); // Wire up a FreezableDefaultPromoter to observe the default value we // just created and automatically promote it to local if it is modified. FreezableDefaultPromoter promoter = new FreezableDefaultPromoter(owner, property); promoter.SetFreezableDefaultValue(result); result.Changed += promoter.OnDefaultValueChanged; return result; } // This is the prototype that CreateDefaultValue copies to create the // mutable default value for this property. See also the ctor. private readonly Freezable _defaultValuePrototype; ////// The FreezableDefaultPromoter observes the mutable defaults we hand out /// for changed events. If the default is ever modified this class will /// promote it to a local value by writing it to the local store and /// clear the cached default value so we will generate a new default /// the next time the property system is asked for one. /// private class FreezableDefaultPromoter { internal FreezableDefaultPromoter(DependencyObject owner, DependencyProperty property) { Debug.Assert(owner != null && property != null, "Caller is responsible for ensuring that owner and property are non-null."); Debug.Assert(!(owner is Freezable) || !((Freezable)owner).IsFrozen, "We should not be observing mutables on a frozen owner."); Debug.Assert(property.GetMetadata(owner.DependencyObjectType).UsingDefaultValueFactory, "How did we end up observing a mutable if we were not registered for the factory pattern?"); // We hang on to the property and owner so we can write the default // value back to the local store if it changes. See also // OnDefaultValueChanged. _owner = owner; _property = property; } internal void OnDefaultValueChanged(object sender, EventArgs e) { Debug.Assert(_mutableDefaultValue != null, "Promoter's creator should have called SetFreezableDefaultValue."); PropertyMetadata metadata = _property.GetMetadata(_owner.DependencyObjectType); // Remove this value from the DefaultValue cache so we stop // handing it out as the default value now that it has changed. metadata.ClearCachedDefaultValue(_owner, _property); // Since Changed is raised when the user freezes the default // value, we need to check before removing our handler. // (If the value is frozen, it will remove it's own handlers.) if (!_mutableDefaultValue.IsFrozen) { _mutableDefaultValue.Changed -= OnDefaultValueChanged; } // If someone else hasn't already written a local local value, // promote the default value to local. if (_owner.ReadLocalValue(_property) == DependencyProperty.UnsetValue) { _owner.SetMutableDefaultValue(_property, _mutableDefaultValue); } } private readonly DependencyObject _owner; private readonly DependencyProperty _property; #region DefaultValue // The creator of a FreezableDefaultValuePromoter should call this method // so that we can verify that the changed sender is the mutable default // value we handed out. internal void SetFreezableDefaultValue(Freezable mutableDefaultValue) { _mutableDefaultValue = mutableDefaultValue; } private Freezable _mutableDefaultValue; #endregion DefaultValue } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DefaultvalueFactory for Freezables // // History: // 2005/11/08 : jordanpa - Created from PresentationCore's old // MutableDefaultPropertyMetadata // //--------------------------------------------------------------------------- using MS.Internal.WindowsBase; using System; using System.Diagnostics; using System.Windows; namespace MS.Internal { //// FreezableDefaultValueFactory is a DefaultValueFactory implementation which // is inserted by the property system for any DP registered with a default // value of type Freezable. The user’s given default value is frozen and // used as a template to create unfrozen copies on a per DP per DO basis. If // the default value is modified it is automatically promoted from default to // local. // [FriendAccessAllowed] // built into Base, used by Core + Framework internal class FreezableDefaultValueFactory : DefaultValueFactory { ////// Stores a frozen copy of defaultValue /// internal FreezableDefaultValueFactory(Freezable defaultValue) { Debug.Assert(defaultValue != null, "Null can not be made mutable. Do not use FreezableDefaultValueFactory."); Debug.Assert(defaultValue.CanFreeze, "The defaultValue prototype must be freezable."); _defaultValuePrototype = defaultValue.GetAsFrozen(); } ////// Returns our frozen sentinel /// internal override object DefaultValue { get { Debug.Assert(_defaultValuePrototype.IsFrozen); return _defaultValuePrototype; } } ////// If the DO is frozen, we'll return our frozen sentinel. Otherwise we'll make /// an unfrozen copy. /// internal override object CreateDefaultValue(DependencyObject owner, DependencyProperty property) { Debug.Assert(owner != null && property != null, "It is the caller responsibility to ensure that owner and property are non-null."); Freezable result = _defaultValuePrototype; Freezable ownerFreezable = owner as Freezable; // If the owner is frozen, just return the frozen prototype. if (ownerFreezable != null && ownerFreezable.IsFrozen) { return result; } result = _defaultValuePrototype.Clone(); // Wire up a FreezableDefaultPromoter to observe the default value we // just created and automatically promote it to local if it is modified. FreezableDefaultPromoter promoter = new FreezableDefaultPromoter(owner, property); promoter.SetFreezableDefaultValue(result); result.Changed += promoter.OnDefaultValueChanged; return result; } // This is the prototype that CreateDefaultValue copies to create the // mutable default value for this property. See also the ctor. private readonly Freezable _defaultValuePrototype; ////// The FreezableDefaultPromoter observes the mutable defaults we hand out /// for changed events. If the default is ever modified this class will /// promote it to a local value by writing it to the local store and /// clear the cached default value so we will generate a new default /// the next time the property system is asked for one. /// private class FreezableDefaultPromoter { internal FreezableDefaultPromoter(DependencyObject owner, DependencyProperty property) { Debug.Assert(owner != null && property != null, "Caller is responsible for ensuring that owner and property are non-null."); Debug.Assert(!(owner is Freezable) || !((Freezable)owner).IsFrozen, "We should not be observing mutables on a frozen owner."); Debug.Assert(property.GetMetadata(owner.DependencyObjectType).UsingDefaultValueFactory, "How did we end up observing a mutable if we were not registered for the factory pattern?"); // We hang on to the property and owner so we can write the default // value back to the local store if it changes. See also // OnDefaultValueChanged. _owner = owner; _property = property; } internal void OnDefaultValueChanged(object sender, EventArgs e) { Debug.Assert(_mutableDefaultValue != null, "Promoter's creator should have called SetFreezableDefaultValue."); PropertyMetadata metadata = _property.GetMetadata(_owner.DependencyObjectType); // Remove this value from the DefaultValue cache so we stop // handing it out as the default value now that it has changed. metadata.ClearCachedDefaultValue(_owner, _property); // Since Changed is raised when the user freezes the default // value, we need to check before removing our handler. // (If the value is frozen, it will remove it's own handlers.) if (!_mutableDefaultValue.IsFrozen) { _mutableDefaultValue.Changed -= OnDefaultValueChanged; } // If someone else hasn't already written a local local value, // promote the default value to local. if (_owner.ReadLocalValue(_property) == DependencyProperty.UnsetValue) { _owner.SetMutableDefaultValue(_property, _mutableDefaultValue); } } private readonly DependencyObject _owner; private readonly DependencyProperty _property; #region DefaultValue // The creator of a FreezableDefaultValuePromoter should call this method // so that we can verify that the changed sender is the mutable default // value we handed out. internal void SetFreezableDefaultValue(Freezable mutableDefaultValue) { _mutableDefaultValue = mutableDefaultValue; } private Freezable _mutableDefaultValue; #endregion DefaultValue } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
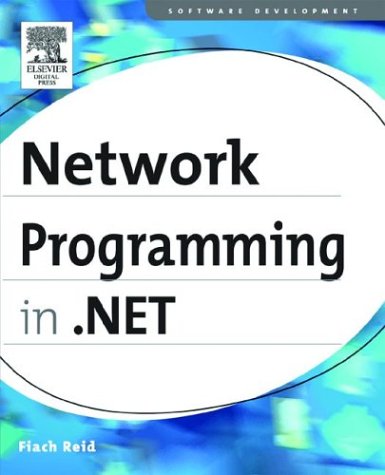
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItem.cs
- IconHelper.cs
- SafeFileMappingHandle.cs
- QueuePropertyVariants.cs
- XmlStreamStore.cs
- ConnectionPoint.cs
- SizeF.cs
- MetadataItemEmitter.cs
- TableLayoutStyle.cs
- DiagnosticTraceSource.cs
- EventWaitHandle.cs
- SoundPlayerAction.cs
- PathGradientBrush.cs
- TokenBasedSet.cs
- LineUtil.cs
- WmlTextViewAdapter.cs
- WebPartEditorCancelVerb.cs
- BroadcastEventHelper.cs
- HealthMonitoringSectionHelper.cs
- EntityTemplateUserControl.cs
- TaskFileService.cs
- ErrorFormatterPage.cs
- ColorConverter.cs
- LOSFormatter.cs
- DataServiceRequestException.cs
- Point.cs
- RawStylusInputCustomDataList.cs
- MimePart.cs
- OleDbCommandBuilder.cs
- ProbeDuplexCD1AsyncResult.cs
- HwndSubclass.cs
- CellParaClient.cs
- BridgeDataRecord.cs
- DoWhileDesigner.xaml.cs
- PeerNameResolver.cs
- MimeReflector.cs
- ToolStripRendererSwitcher.cs
- XmlNodeChangedEventArgs.cs
- ListItemConverter.cs
- EncryptedReference.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- BindMarkupExtensionSerializer.cs
- ContextMenuAutomationPeer.cs
- ViewLoader.cs
- StreamingContext.cs
- DirectoryObjectSecurity.cs
- Command.cs
- DBConnectionString.cs
- XmlElementAttribute.cs
- Guid.cs
- DataGridDesigner.cs
- LeftCellWrapper.cs
- CompositeActivityCodeGenerator.cs
- MailWriter.cs
- DependencyPropertyAttribute.cs
- ListViewSelectEventArgs.cs
- CompiledAction.cs
- ViewStateException.cs
- MasterPage.cs
- RegexCompiler.cs
- NameValueConfigurationCollection.cs
- BuildProviderAppliesToAttribute.cs
- MSHTMLHostUtil.cs
- ListViewCancelEventArgs.cs
- ReliableMessagingVersion.cs
- NotifyCollectionChangedEventArgs.cs
- WrappingXamlSchemaContext.cs
- NamespaceList.cs
- ImmComposition.cs
- DesignConnectionCollection.cs
- SchemaAttDef.cs
- Size.cs
- CodeAccessSecurityEngine.cs
- FixedSOMSemanticBox.cs
- PathFigure.cs
- SR.cs
- XhtmlMobileTextWriter.cs
- Model3DGroup.cs
- XmlCharCheckingWriter.cs
- EnvelopedSignatureTransform.cs
- StringExpressionSet.cs
- GeometryValueSerializer.cs
- PropertyOverridesDialog.cs
- SemaphoreFullException.cs
- DataGridViewCellConverter.cs
- CodeAccessSecurityEngine.cs
- NameTable.cs
- pingexception.cs
- ConsoleKeyInfo.cs
- SqlTransaction.cs
- CacheForPrimitiveTypes.cs
- PageContentCollection.cs
- Tuple.cs
- RangeValuePattern.cs
- StylusEditingBehavior.cs
- IteratorFilter.cs
- DropTarget.cs
- WebPartConnectionsConfigureVerb.cs
- IpcPort.cs
- Ray3DHitTestResult.cs