Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / GraphicsPathIterator.cs / 1305376 / GraphicsPathIterator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Drawing; using System.ComponentModel; using System.Drawing.Internal; using System.Globalization; using System.Runtime.Versioning; /** * Represent a Path Iterator object */ ////// /// public sealed class GraphicsPathIterator : MarshalByRefObject, IDisposable { /** * Create a new path iterator object */ ////// Provides helper functions for the ///class. /// /// /// Initializes a new instance of the [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public GraphicsPathIterator(GraphicsPath path) { IntPtr nativeIter = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreatePathIter(out nativeIter, new HandleRef(path, (path == null) ? IntPtr.Zero : path.nativePath)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.nativeIter = nativeIter; } /** * Dispose of resources associated with the */ ///class with the specified . /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } void Dispose(bool disposing) { if (nativeIter != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeletePathIter(new HandleRef(this, nativeIter)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeIter = IntPtr.Zero; } } } ///. /// /// /// Cleans up Windows resources for this /// ~GraphicsPathIterator() { Dispose(false); } /** * Next subpath in path */ ///. /// /// /// Returns the number of subpaths in the /// public int NextSubpath(out int startIndex, out int endIndex, out bool isClosed) { int resultCount = 0; int tempStart = 0; int tempEnd = 0; int status = SafeNativeMethods.Gdip.GdipPathIterNextSubpath(new HandleRef(this, nativeIter), out resultCount, out tempStart, out tempEnd, out isClosed); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); else { startIndex = tempStart; endIndex = tempEnd; } return resultCount; } /** * Next subpath in path */ ///. The start index and end index of the /// next subpath are contained in out parameters. /// /// /// public int NextSubpath(GraphicsPath path, out bool isClosed) { int resultCount = 0; int status = SafeNativeMethods.Gdip.GdipPathIterNextSubpathPath(new HandleRef(this, nativeIter), out resultCount, new HandleRef(path, (path == null) ? IntPtr.Zero : path.nativePath), out isClosed); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return resultCount; } /** * Next type in subpath */ ///[To be supplied.] ////// /// public int NextPathType(out byte pathType, out int startIndex, out int endIndex) { int resultCount = 0; int status = SafeNativeMethods.Gdip.GdipPathIterNextPathType(new HandleRef(this, nativeIter), out resultCount, out pathType, out startIndex, out endIndex); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return resultCount; } /** * Next marker in subpath */ ///[To be supplied.] ////// /// public int NextMarker(out int startIndex, out int endIndex) { int resultCount = 0; int status = SafeNativeMethods.Gdip.GdipPathIterNextMarker(new HandleRef(this, nativeIter), out resultCount, out startIndex, out endIndex); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return resultCount; } /** * Next marker in subpath */ ///[To be supplied.] ////// /// public int NextMarker(GraphicsPath path) { int resultCount = 0; int status = SafeNativeMethods.Gdip.GdipPathIterNextMarkerPath(new HandleRef(this, nativeIter), out resultCount, new HandleRef(path, (path == null) ? IntPtr.Zero : path.nativePath)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return resultCount; } ///[To be supplied.] ////// /// public int Count { get { int resultCount = 0; int status = SafeNativeMethods.Gdip.GdipPathIterGetCount(new HandleRef(this, nativeIter), out resultCount); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return resultCount; } } ///[To be supplied.] ////// /// public int SubpathCount { get { int resultCount = 0; int status = SafeNativeMethods.Gdip.GdipPathIterGetSubpathCount(new HandleRef(this, nativeIter), out resultCount); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return resultCount; } } ///[To be supplied.] ////// /// public bool HasCurve() { bool hasCurve = false; int status = SafeNativeMethods.Gdip.GdipPathIterHasCurve(new HandleRef(this, nativeIter), out hasCurve); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return hasCurve; } ///[To be supplied.] ////// /// public void Rewind() { int status = SafeNativeMethods.Gdip.GdipPathIterRewind(new HandleRef(this, nativeIter)); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// public int Enumerate(ref PointF[] points, ref byte[] types) { if (points.Length != types.Length) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); int resultCount = 0; GPPOINTF pt = new GPPOINTF(); int size = (int) Marshal.SizeOf(pt.GetType()); int count = points.Length; IntPtr memoryPts = Marshal.AllocHGlobal(count*size); try { int status = SafeNativeMethods.Gdip.GdipPathIterEnumerate(new HandleRef(this, nativeIter), out resultCount, memoryPts, types, points.Length); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } points = SafeNativeMethods.Gdip.ConvertGPPOINTFArrayF(memoryPts, points.Length); } finally { Marshal.FreeHGlobal(memoryPts); } return resultCount; } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Design", "CA1045:DoNotPassTypesByReference")] public int CopyData(ref PointF[] points, ref byte[] types, int startIndex, int endIndex) { if (points.Length != types.Length) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); int resultCount = 0; GPPOINTF pt = new GPPOINTF(); int size = (int)Marshal.SizeOf(pt.GetType()); int count = points.Length; IntPtr memoryPts = Marshal.AllocHGlobal(count*size); try { int status = SafeNativeMethods.Gdip.GdipPathIterCopyData(new HandleRef(this, nativeIter), out resultCount, memoryPts, types, startIndex, endIndex); if (status != SafeNativeMethods.Gdip.Ok) { throw SafeNativeMethods.Gdip.StatusException(status); } points = SafeNativeMethods.Gdip.ConvertGPPOINTFArrayF(memoryPts, points.Length); } finally { Marshal.FreeHGlobal(memoryPts); } return resultCount; } /* * handle to native path iterator object */ internal IntPtr nativeIter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
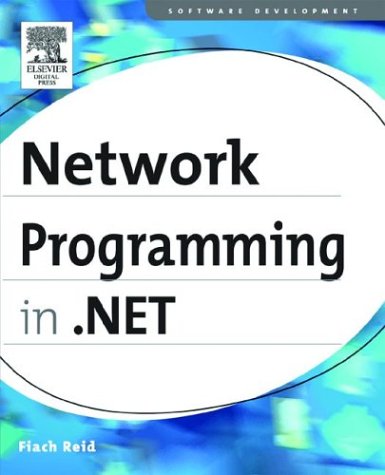
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeStatementCollection.cs
- TypeSystemHelpers.cs
- TrackingProfile.cs
- StoryFragments.cs
- ToolStripHighContrastRenderer.cs
- CustomPopupPlacement.cs
- ServiceDurableInstanceContextProvider.cs
- ObservableDictionary.cs
- SimpleMailWebEventProvider.cs
- Privilege.cs
- ToolStripStatusLabel.cs
- Assembly.cs
- ProvidePropertyAttribute.cs
- XmlElementList.cs
- HostSecurityManager.cs
- DesignSurfaceServiceContainer.cs
- TagMapCollection.cs
- MemoryPressure.cs
- OletxResourceManager.cs
- MailDefinition.cs
- MiniLockedBorderGlyph.cs
- PersistencePipeline.cs
- CompareInfo.cs
- CodeAttributeArgumentCollection.cs
- RelatedCurrencyManager.cs
- ButtonBase.cs
- DependencyProperty.cs
- _NegoStream.cs
- SizeAnimation.cs
- ServiceProviders.cs
- DataGridViewComboBoxColumn.cs
- TextBoxLine.cs
- CompensationExtension.cs
- XPathDocument.cs
- PackageRelationshipCollection.cs
- RequestTimeoutManager.cs
- CacheRequest.cs
- TextEffect.cs
- RSAOAEPKeyExchangeFormatter.cs
- DataGridViewCellToolTipTextNeededEventArgs.cs
- RoutedEventHandlerInfo.cs
- RTLAwareMessageBox.cs
- DocumentPageHost.cs
- BadImageFormatException.cs
- ChangeTracker.cs
- SecurityContext.cs
- DataGridViewDataErrorEventArgs.cs
- InstanceHandleReference.cs
- SchemaMapping.cs
- ExtenderControl.cs
- Automation.cs
- Parameter.cs
- Page.cs
- ConfigurationValidatorBase.cs
- StylusShape.cs
- TimeManager.cs
- ChildrenQuery.cs
- WhitespaceRuleLookup.cs
- ClientUrlResolverWrapper.cs
- InfoCardXmlSerializer.cs
- ClientFormsIdentity.cs
- EntityContainerAssociationSet.cs
- EntityExpressionVisitor.cs
- TemplateControlCodeDomTreeGenerator.cs
- BufferModeSettings.cs
- BuildManagerHost.cs
- RuntimeHandles.cs
- CompiledELinqQueryState.cs
- LayoutDump.cs
- FormsAuthenticationUser.cs
- TripleDESCryptoServiceProvider.cs
- MULTI_QI.cs
- BatchParser.cs
- MembershipUser.cs
- FactoryGenerator.cs
- VoiceInfo.cs
- FilteredXmlReader.cs
- ContentFilePart.cs
- ProfileBuildProvider.cs
- WebHttpSecurityModeHelper.cs
- PhoneCallDesigner.cs
- WbemException.cs
- ResolveInfo.cs
- DataColumnMapping.cs
- MasterPageBuildProvider.cs
- PenContexts.cs
- SynchronizedDispatch.cs
- coordinatorscratchpad.cs
- SwitchCase.cs
- SmtpException.cs
- CmsInterop.cs
- CodeTypeDelegate.cs
- ResourceDefaultValueAttribute.cs
- Calendar.cs
- CustomCredentialPolicy.cs
- IsolatedStorageException.cs
- SerializationIncompleteException.cs
- ProgressBarHighlightConverter.cs
- BinHexDecoder.cs
- OletxTransactionFormatter.cs