Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media3D / Model3D.cs / 1 / Model3D.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D model implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/18/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using MS.Internal.Media3D; namespace System.Windows.Media.Media3D { ////// Model3D is the abstract model that everything builds from. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public abstract partial class Model3D : Animatable { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Prevent 3rd parties from extending this abstract base class. internal Model3D() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Gets bounds for this model. /// public Rect3D Bounds { get { ReadPreamble(); return CalculateSubgraphBoundsOuterSpace(); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods // NOTE: Model3D hit testing takes the rayParams in the outer space of the // Model3D. That is, RayHitTest() will apply this model's transform // to the ray for the caller. // // This is different than Visual hit testing which does not transform // the hit testing parameters by the Visual's transform. internal void RayHitTest(RayHitTestParameters rayParams) { Transform3D transform = Transform; rayParams.PushModelTransform(transform); RayHitTestCore(rayParams); rayParams.PopTransform(transform); } internal abstract void RayHitTestCore(RayHitTestParameters rayParams); ////// Returns the bounds of the Model3D subgraph rooted at this Model3D. /// /// Outer space refers to the space after this Model's Transform is /// applied -- or said another way, applying a transform to this Model /// affects it's outer bounds. (While its inner bounds remain unchanged.) /// internal Rect3D CalculateSubgraphBoundsOuterSpace() { Rect3D innerBounds = CalculateSubgraphBoundsInnerSpace(); return M3DUtil.ComputeTransformedAxisAlignedBoundingBox(ref innerBounds, Transform); } ////// Returns the bounds of the Model3D subgraph rooted at this Model3D. /// /// Inner space refers to the space before this Model's Transform is /// applied -- or said another way, applying a transform to this Model /// only affects it's outer bounds. Its inner bounds remain unchanged. /// internal abstract Rect3D CalculateSubgraphBoundsInnerSpace(); // NTRAID#Longhorn-1591973-2006/03/31-[....] - Should be "UpdateRealizations" like Drawings internal abstract void MarkVisibleRealizations(RealizationContext rc); // NTRAID#Longhorn-1591973-2006/03/31-[....] - Should be using inherited "Precompute" from Animatable internal abstract void PreCompute(); #endregion Internal Methods #region Model3D Flags // These flags aren't in an enum to avoid adding another Type to the assembly and // because BitVector32 requires ints // If this is true, then the Model3D needs PreCompute() called on it. // Currently, this is only true for GeometryModel3D's when their Material // property changes (and any Model3DGroup that contains such a GM3D) // // As needed in the future, DirtyForPreComputeFlag can be used for other // things (i.e. if we ever cache Transform in PreCompute()) internal const int DirtyForPreComputeFlag = 1 << 0; // If this is true, then MarkVisibleRealizations() needs to be called on // the Model3D. // // Currently, this is only true for a GeometryModel3D that has text in // it's material (and any Model3DGroup that contains such a GM3D) // // Unlike Visuals, we make no distinction between if something under // this Model3D requires realization or if this Model3D itself requires // realization internal const int RequiresRealizationFlag = 1 << 1; // NTRAID#Longhorn-1591973-2006/03/31-[....] - Should be using inherited "RequiresRealizationUpdates" from Animatable // // Important: start off dirty internal BitVector32 _flags = new BitVector32(DirtyForPreComputeFlag); #endregion Model3D Flags } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D model implementation. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/18/2003 : t-gregr - Created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using MS.Internal.Media3D; namespace System.Windows.Media.Media3D { ////// Model3D is the abstract model that everything builds from. /// [Localizability(LocalizationCategory.None, Readability = Readability.Unreadable)] // cannot be read & localized as string public abstract partial class Model3D : Animatable { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors // Prevent 3rd parties from extending this abstract base class. internal Model3D() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Gets bounds for this model. /// public Rect3D Bounds { get { ReadPreamble(); return CalculateSubgraphBoundsOuterSpace(); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods // NOTE: Model3D hit testing takes the rayParams in the outer space of the // Model3D. That is, RayHitTest() will apply this model's transform // to the ray for the caller. // // This is different than Visual hit testing which does not transform // the hit testing parameters by the Visual's transform. internal void RayHitTest(RayHitTestParameters rayParams) { Transform3D transform = Transform; rayParams.PushModelTransform(transform); RayHitTestCore(rayParams); rayParams.PopTransform(transform); } internal abstract void RayHitTestCore(RayHitTestParameters rayParams); ////// Returns the bounds of the Model3D subgraph rooted at this Model3D. /// /// Outer space refers to the space after this Model's Transform is /// applied -- or said another way, applying a transform to this Model /// affects it's outer bounds. (While its inner bounds remain unchanged.) /// internal Rect3D CalculateSubgraphBoundsOuterSpace() { Rect3D innerBounds = CalculateSubgraphBoundsInnerSpace(); return M3DUtil.ComputeTransformedAxisAlignedBoundingBox(ref innerBounds, Transform); } ////// Returns the bounds of the Model3D subgraph rooted at this Model3D. /// /// Inner space refers to the space before this Model's Transform is /// applied -- or said another way, applying a transform to this Model /// only affects it's outer bounds. Its inner bounds remain unchanged. /// internal abstract Rect3D CalculateSubgraphBoundsInnerSpace(); // NTRAID#Longhorn-1591973-2006/03/31-[....] - Should be "UpdateRealizations" like Drawings internal abstract void MarkVisibleRealizations(RealizationContext rc); // NTRAID#Longhorn-1591973-2006/03/31-[....] - Should be using inherited "Precompute" from Animatable internal abstract void PreCompute(); #endregion Internal Methods #region Model3D Flags // These flags aren't in an enum to avoid adding another Type to the assembly and // because BitVector32 requires ints // If this is true, then the Model3D needs PreCompute() called on it. // Currently, this is only true for GeometryModel3D's when their Material // property changes (and any Model3DGroup that contains such a GM3D) // // As needed in the future, DirtyForPreComputeFlag can be used for other // things (i.e. if we ever cache Transform in PreCompute()) internal const int DirtyForPreComputeFlag = 1 << 0; // If this is true, then MarkVisibleRealizations() needs to be called on // the Model3D. // // Currently, this is only true for a GeometryModel3D that has text in // it's material (and any Model3DGroup that contains such a GM3D) // // Unlike Visuals, we make no distinction between if something under // this Model3D requires realization or if this Model3D itself requires // realization internal const int RequiresRealizationFlag = 1 << 1; // NTRAID#Longhorn-1591973-2006/03/31-[....] - Should be using inherited "RequiresRealizationUpdates" from Animatable // // Important: start off dirty internal BitVector32 _flags = new BitVector32(DirtyForPreComputeFlag); #endregion Model3D Flags } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
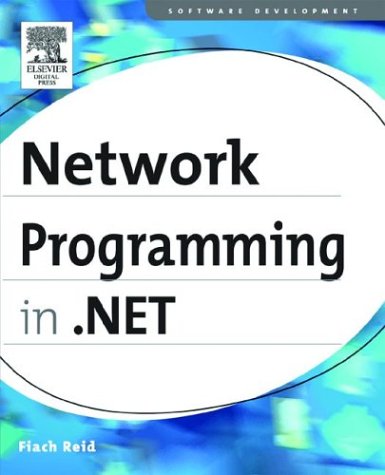
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CompositeFontInfo.cs
- HtmlToClrEventProxy.cs
- PersonalizationAdministration.cs
- HashHelper.cs
- _CacheStreams.cs
- ProfileGroupSettings.cs
- ReferencedAssembly.cs
- ComponentChangingEvent.cs
- DbExpressionVisitor.cs
- FocusWithinProperty.cs
- FixedSOMFixedBlock.cs
- TraceSource.cs
- DataControlButton.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- _FtpDataStream.cs
- CrossAppDomainChannel.cs
- DbProviderServices.cs
- IPAddressCollection.cs
- ExceptionRoutedEventArgs.cs
- FlowDocumentView.cs
- RoleManagerModule.cs
- StrokeCollection2.cs
- ProtocolViolationException.cs
- Size3D.cs
- DbProviderManifest.cs
- TagPrefixCollection.cs
- DbCommandDefinition.cs
- ByteRangeDownloader.cs
- TypeContext.cs
- TypeSystem.cs
- SineEase.cs
- IntranetCredentialPolicy.cs
- ControlParameter.cs
- UnsafeNativeMethods.cs
- ErrorEventArgs.cs
- FontFamily.cs
- XmlSerializerAssemblyAttribute.cs
- DataGridViewTextBoxCell.cs
- ExpressionBinding.cs
- MediaContext.cs
- XmlNodeComparer.cs
- MergeLocalizationDirectives.cs
- ReadOnlyObservableCollection.cs
- SimpleMailWebEventProvider.cs
- ServiceInfoCollection.cs
- Evidence.cs
- AxHost.cs
- XmlSignificantWhitespace.cs
- SpeechRecognitionEngine.cs
- UpdatePanelTrigger.cs
- Reference.cs
- ColumnBinding.cs
- FlowSwitchLink.cs
- CqlBlock.cs
- ButtonRenderer.cs
- BigInt.cs
- InputBindingCollection.cs
- User.cs
- WebPartVerbCollection.cs
- DataSourceExpressionCollection.cs
- DispatcherEventArgs.cs
- Calendar.cs
- Add.cs
- ServiceContractListItemList.cs
- Subtree.cs
- OrderingQueryOperator.cs
- SslStream.cs
- TypeBuilderInstantiation.cs
- SHA1.cs
- RequestSecurityToken.cs
- ConcatQueryOperator.cs
- ScriptReferenceBase.cs
- OletxVolatileEnlistment.cs
- ToolStripItemRenderEventArgs.cs
- SqlFormatter.cs
- SafeUserTokenHandle.cs
- NativeMethods.cs
- ErrorFormatter.cs
- FontStyle.cs
- CodeBlockBuilder.cs
- LinqDataSourceView.cs
- TextModifierScope.cs
- DbExpressionRules.cs
- PartitionerStatic.cs
- XPathNodeList.cs
- SqlBulkCopyColumnMapping.cs
- MatrixConverter.cs
- PointValueSerializer.cs
- TransactionScope.cs
- BaseProcessor.cs
- NativeMethodsOther.cs
- WebUtil.cs
- ResourceReferenceKeyNotFoundException.cs
- ObjectCloneHelper.cs
- BaseDataBoundControl.cs
- StateMachineWorkflowInstance.cs
- RSAProtectedConfigurationProvider.cs
- InternalConfigRoot.cs
- BinHexDecoder.cs
- AutomationFocusChangedEventArgs.cs