Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / Utils / CdpEqualityComparer.cs / 1 / CdpEqualityComparer.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Globalization; using System.Data.SqlTypes; using System.Diagnostics; namespace System.Data.Common.Utils { ////// Equality comparer implementation that uses case-sensitive ordinal comparison for strings. /// internal sealed class CdpEqualityComparer : IEqualityComparer { ////// Private constructor to ensure comparer is only accessed through the private CdpEqualityComparer() { } private static readonly IEqualityComparer s_defaultEqualityComparer = new CdpEqualityComparer(); ////// property. /// /// Gets instance of CdpEqualityComparer. /// static internal IEqualityComparer DefaultEqualityComparer { get { return s_defaultEqualityComparer; } } ////// This mirrors the .NET implementation of System.Collections.Comparer but /// uses an ordinal comparer for strings rather than a linguistic compare. /// /// First object to compare /// Second object to compare ///True if the arguments are equivalent; false otherwise bool IEqualityComparer.Equals(object x, object y) { // if the objects are equal by reference, return immediately if (x == y) { return true; } // if either one or the other objects is null, return false // (they cannot both be null, because otherwise the reference equality check // would have succeeded) if (null == x || null == y) { return false; } // if we're dealing with strings, special handling is required string xAsString = x as string; if (null != xAsString) { string yAsString = y as string; if (null != yAsString) { return StringComparer.Ordinal.Equals(xAsString, yAsString); } else { // string implements IComparable, so we can avoid a second cast return 0 == xAsString.CompareTo(y); } } // use first argument as IComparable IComparable xAsComparable = x as IComparable; if (null != xAsComparable) { return 0 == xAsComparable.CompareTo(y); } else { // default to Equals implementation return x.Equals(y); } } ////// Retursn hash code for given object. /// /// Object for which to produce hash code (throws if null) ///Hash code for argument int IEqualityComparer.GetHashCode(object obj) { Debug.Assert(null != obj); return obj.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Text; using System.Globalization; using System.Data.SqlTypes; using System.Diagnostics; namespace System.Data.Common.Utils { ////// Equality comparer implementation that uses case-sensitive ordinal comparison for strings. /// internal sealed class CdpEqualityComparer : IEqualityComparer { ////// Private constructor to ensure comparer is only accessed through the private CdpEqualityComparer() { } private static readonly IEqualityComparer s_defaultEqualityComparer = new CdpEqualityComparer(); ////// property. /// /// Gets instance of CdpEqualityComparer. /// static internal IEqualityComparer DefaultEqualityComparer { get { return s_defaultEqualityComparer; } } ////// This mirrors the .NET implementation of System.Collections.Comparer but /// uses an ordinal comparer for strings rather than a linguistic compare. /// /// First object to compare /// Second object to compare ///True if the arguments are equivalent; false otherwise bool IEqualityComparer.Equals(object x, object y) { // if the objects are equal by reference, return immediately if (x == y) { return true; } // if either one or the other objects is null, return false // (they cannot both be null, because otherwise the reference equality check // would have succeeded) if (null == x || null == y) { return false; } // if we're dealing with strings, special handling is required string xAsString = x as string; if (null != xAsString) { string yAsString = y as string; if (null != yAsString) { return StringComparer.Ordinal.Equals(xAsString, yAsString); } else { // string implements IComparable, so we can avoid a second cast return 0 == xAsString.CompareTo(y); } } // use first argument as IComparable IComparable xAsComparable = x as IComparable; if (null != xAsComparable) { return 0 == xAsComparable.CompareTo(y); } else { // default to Equals implementation return x.Equals(y); } } ////// Retursn hash code for given object. /// /// Object for which to produce hash code (throws if null) ///Hash code for argument int IEqualityComparer.GetHashCode(object obj) { Debug.Assert(null != obj); return obj.GetHashCode(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
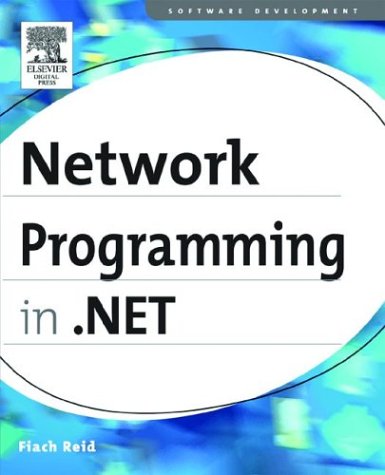
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeParameterWriter.cs
- ToolBarPanel.cs
- ExceptionHandlers.cs
- LocalizationComments.cs
- ActivityMarkupSerializationProvider.cs
- BrowserCapabilitiesCodeGenerator.cs
- FileUtil.cs
- BaseValidator.cs
- UInt16Storage.cs
- RuleSettingsCollection.cs
- ISAPIApplicationHost.cs
- MulticastDelegate.cs
- BaseCollection.cs
- QilName.cs
- SqlConnectionPoolGroupProviderInfo.cs
- ModelTypeConverter.cs
- PasswordDeriveBytes.cs
- GestureRecognitionResult.cs
- TextReader.cs
- DataGridViewRowStateChangedEventArgs.cs
- InkSerializer.cs
- ColorInterpolationModeValidation.cs
- RangeBaseAutomationPeer.cs
- HashMembershipCondition.cs
- PropertyGridView.cs
- DependencySource.cs
- HtmlImage.cs
- ImpersonationContext.cs
- OrderedDictionary.cs
- AccessedThroughPropertyAttribute.cs
- OrderToken.cs
- EntityDesignerDataSourceView.cs
- WorkflowHostingResponseContext.cs
- XmlBaseReader.cs
- EventSinkActivity.cs
- PerformanceCountersElement.cs
- SiteMapHierarchicalDataSourceView.cs
- CfgParser.cs
- PagerSettings.cs
- KeyValueConfigurationCollection.cs
- GetChildSubtree.cs
- GridViewEditEventArgs.cs
- ColorTransform.cs
- UnsafeNativeMethodsPenimc.cs
- WebServiceEnumData.cs
- AsyncOperation.cs
- PeerReferralPolicy.cs
- DataStorage.cs
- SafeHandles.cs
- _NegotiateClient.cs
- TriggerActionCollection.cs
- XsdBuildProvider.cs
- DockPanel.cs
- ResourceSet.cs
- NameValueCollection.cs
- TextEncodedRawTextWriter.cs
- WorkItem.cs
- BitmapMetadataBlob.cs
- SaveFileDialog.cs
- OdbcRowUpdatingEvent.cs
- ZeroOpNode.cs
- FormViewDeletedEventArgs.cs
- UnsafeNativeMethods.cs
- Pair.cs
- DispatcherExceptionEventArgs.cs
- ReflectPropertyDescriptor.cs
- AcceleratedTokenProvider.cs
- WebBrowser.cs
- CreateRefExpr.cs
- XmlSignificantWhitespace.cs
- DefaultAuthorizationContext.cs
- HttpModuleCollection.cs
- ReachDocumentPageSerializer.cs
- BooleanExpr.cs
- EntityClassGenerator.cs
- MetabaseSettings.cs
- StorageMappingFragment.cs
- InterleavedZipPartStream.cs
- ToolStripItem.cs
- Matrix3DConverter.cs
- XpsThumbnail.cs
- WorkflowControlEndpoint.cs
- XmlByteStreamWriter.cs
- DocumentPropertiesDialog.cs
- ResetableIterator.cs
- DrawingImage.cs
- OutputCacheSection.cs
- ListView.cs
- AdPostCacheSubstitution.cs
- MessageAction.cs
- IPCCacheManager.cs
- CaseInsensitiveOrdinalStringComparer.cs
- SrgsDocument.cs
- HttpClientCertificate.cs
- DataGridAutomationPeer.cs
- documentsequencetextpointer.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- DataServiceClientException.cs
- ToolStripSystemRenderer.cs
- GeometryCombineModeValidation.cs