Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Configuration / COAUTHINFO.cs / 1 / COAUTHINFO.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Collections; using System.Configuration; using System.Configuration.Internal; using System.Web; using System.Web.Util; using System.Security; using System.IO; using System.Web.Hosting; using System.Runtime.InteropServices; using System.Reflection; using System.Collections.Specialized; using System.Xml; using System.Security.Principal; using System.Threading; using System.Globalization; [StructLayout(LayoutKind.Sequential, Pack = 4, CharSet = CharSet.Unicode)] internal class COAUTHINFO : IDisposable { internal COAUTHINFO(RpcAuthent authent, RpcAuthor author, string serverprinc, RpcLevel level, RpcImpers impers, IntPtr ciptr) { authnsvc = authent; authzsvc = author; serverprincname = serverprinc; authnlevel = level; impersonationlevel = impers; authidentitydata = ciptr; } internal RpcAuthent authnsvc; internal RpcAuthor authzsvc; [MarshalAs(UnmanagedType.LPWStr)] internal string serverprincname; internal RpcLevel authnlevel; internal RpcImpers impersonationlevel; internal IntPtr authidentitydata; // COAUTHIDENTITY* internal int capabilities = 0; // EOAC_NONE void IDisposable.Dispose() { authidentitydata = IntPtr.Zero; GC.SuppressFinalize(this); } ~COAUTHINFO() { } } [StructLayout(LayoutKind.Sequential, Pack = 4, CharSet = CharSet.Unicode)] internal class COAUTHINFO_X64 : IDisposable { internal COAUTHINFO_X64(RpcAuthent authent, RpcAuthor author, string serverprinc, RpcLevel level, RpcImpers impers, IntPtr ciptr) { authnsvc = authent; authzsvc = author; serverprincname = serverprinc; authnlevel = level; impersonationlevel = impers; authidentitydata = ciptr; } internal RpcAuthent authnsvc; internal RpcAuthor authzsvc; [MarshalAs(UnmanagedType.LPWStr)] internal string serverprincname; internal RpcLevel authnlevel; internal RpcImpers impersonationlevel; internal IntPtr authidentitydata; // COAUTHIDENTITY* internal int capabilities = 0; // EOAC_NONE #pragma warning disable 0649 internal int padding; #pragma warning restore 0649 void IDisposable.Dispose() { authidentitydata = IntPtr.Zero; GC.SuppressFinalize(this); } ~COAUTHINFO_X64() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System.Collections; using System.Configuration; using System.Configuration.Internal; using System.Web; using System.Web.Util; using System.Security; using System.IO; using System.Web.Hosting; using System.Runtime.InteropServices; using System.Reflection; using System.Collections.Specialized; using System.Xml; using System.Security.Principal; using System.Threading; using System.Globalization; [StructLayout(LayoutKind.Sequential, Pack = 4, CharSet = CharSet.Unicode)] internal class COAUTHINFO : IDisposable { internal COAUTHINFO(RpcAuthent authent, RpcAuthor author, string serverprinc, RpcLevel level, RpcImpers impers, IntPtr ciptr) { authnsvc = authent; authzsvc = author; serverprincname = serverprinc; authnlevel = level; impersonationlevel = impers; authidentitydata = ciptr; } internal RpcAuthent authnsvc; internal RpcAuthor authzsvc; [MarshalAs(UnmanagedType.LPWStr)] internal string serverprincname; internal RpcLevel authnlevel; internal RpcImpers impersonationlevel; internal IntPtr authidentitydata; // COAUTHIDENTITY* internal int capabilities = 0; // EOAC_NONE void IDisposable.Dispose() { authidentitydata = IntPtr.Zero; GC.SuppressFinalize(this); } ~COAUTHINFO() { } } [StructLayout(LayoutKind.Sequential, Pack = 4, CharSet = CharSet.Unicode)] internal class COAUTHINFO_X64 : IDisposable { internal COAUTHINFO_X64(RpcAuthent authent, RpcAuthor author, string serverprinc, RpcLevel level, RpcImpers impers, IntPtr ciptr) { authnsvc = authent; authzsvc = author; serverprincname = serverprinc; authnlevel = level; impersonationlevel = impers; authidentitydata = ciptr; } internal RpcAuthent authnsvc; internal RpcAuthor authzsvc; [MarshalAs(UnmanagedType.LPWStr)] internal string serverprincname; internal RpcLevel authnlevel; internal RpcImpers impersonationlevel; internal IntPtr authidentitydata; // COAUTHIDENTITY* internal int capabilities = 0; // EOAC_NONE #pragma warning disable 0649 internal int padding; #pragma warning restore 0649 void IDisposable.Dispose() { authidentitydata = IntPtr.Zero; GC.SuppressFinalize(this); } ~COAUTHINFO_X64() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
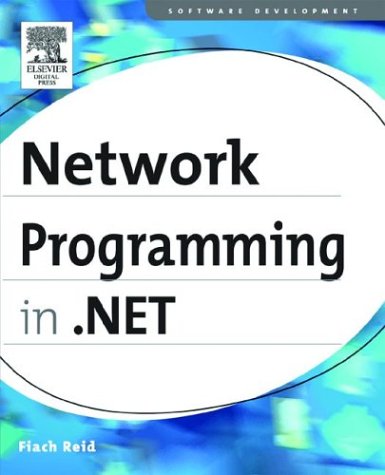
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolboxService.cs
- CmsUtils.cs
- BaseParser.cs
- TraceHandler.cs
- FullTextLine.cs
- FactoryMaker.cs
- UrlMappingsSection.cs
- DataGridView.cs
- XpsSerializerWriter.cs
- WebPart.cs
- BindingMAnagerBase.cs
- RenderCapability.cs
- BatchServiceHost.cs
- CheckPair.cs
- EventManager.cs
- InputLanguageProfileNotifySink.cs
- CryptoKeySecurity.cs
- RemoteWebConfigurationHostStream.cs
- RegisteredDisposeScript.cs
- X509ChainElement.cs
- RequestCacheValidator.cs
- PeerNameRecord.cs
- DataViewListener.cs
- ErrorHandlerModule.cs
- FormView.cs
- WebRequestModuleElement.cs
- XXXOnTypeBuilderInstantiation.cs
- RoutedEventConverter.cs
- Debugger.cs
- WindowsToolbar.cs
- PolicyLevel.cs
- DataTableMappingCollection.cs
- indexingfiltermarshaler.cs
- EntryWrittenEventArgs.cs
- ActivityDelegate.cs
- DocumentXmlWriter.cs
- TaskbarItemInfo.cs
- KeyEvent.cs
- StrongNameKeyPair.cs
- TextViewBase.cs
- ExtensionDataReader.cs
- StyleReferenceConverter.cs
- XmlDomTextWriter.cs
- NativeMethodsCLR.cs
- CompositeDataBoundControl.cs
- RedBlackList.cs
- SendMailErrorEventArgs.cs
- BuilderPropertyEntry.cs
- HostSecurityManager.cs
- EasingQuaternionKeyFrame.cs
- RequiredFieldValidator.cs
- Events.cs
- MbpInfo.cs
- ServerIdentity.cs
- CngAlgorithm.cs
- CopyCodeAction.cs
- WebPart.cs
- TcpHostedTransportConfiguration.cs
- KeyProperty.cs
- HostedTransportConfigurationManager.cs
- SharedPerformanceCounter.cs
- CounterSetInstanceCounterDataSet.cs
- Label.cs
- ServiceNameCollection.cs
- MiniMapControl.xaml.cs
- GroupStyle.cs
- DataControlCommands.cs
- FieldNameLookup.cs
- EventBindingService.cs
- XmlSchema.cs
- NegationPusher.cs
- StateInitialization.cs
- DataGridViewDataConnection.cs
- TabletCollection.cs
- JavascriptXmlWriterWrapper.cs
- SrgsText.cs
- ScaleTransform3D.cs
- RIPEMD160Managed.cs
- ipaddressinformationcollection.cs
- IntegerValidatorAttribute.cs
- DataRelationCollection.cs
- QuadraticBezierSegment.cs
- ServiceObjectContainer.cs
- DbDataSourceEnumerator.cs
- DynamicValueConverter.cs
- EmbeddedMailObject.cs
- VirtualizingStackPanel.cs
- PageThemeParser.cs
- SplineKeyFrames.cs
- QualificationDataItem.cs
- CreateUserWizard.cs
- PageParserFilter.cs
- TextServicesPropertyRanges.cs
- TableItemProviderWrapper.cs
- UIElementParaClient.cs
- NetworkInformationException.cs
- WindowAutomationPeer.cs
- wgx_commands.cs
- XmlWrappingReader.cs
- VisualStyleInformation.cs