Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / HTTPNotFoundHandler.cs / 1 / HTTPNotFoundHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Synchronous Http request handler interface * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { ////// internal class HttpNotFoundHandler : IHttpHandler { internal HttpNotFoundHandler() { } ///Provides a synchronous Http request handler interface. ////// public void ProcessRequest(HttpContext context) { PerfCounters.IncrementCounter(AppPerfCounter.REQUESTS_NOT_FOUND); throw new HttpException(404, SR.GetString(SR.Path_not_found, context.Request.Path)); } ///Drives web processing execution. ////// public bool IsReusable { get { return true; } } } internal class HttpForbiddenHandler : IHttpHandler { internal HttpForbiddenHandler() { } ///Indicates whether an HttpNotFoundHandler instance can be recycled and used /// for another request. ////// public void ProcessRequest(HttpContext context) { PerfCounters.IncrementCounter(AppPerfCounter.REQUESTS_NOT_FOUND); throw new HttpException(403, SR.GetString(SR.Path_forbidden, context.Request.Path)); } ///Drives web processing execution. ////// public bool IsReusable { get { return true; } } } ///Indicates whether an HttpForbiddenHandler instance can be recycled and used /// for another request. ////// internal class HttpMethodNotAllowedHandler : IHttpHandler { internal HttpMethodNotAllowedHandler() { } ///Provides a synchronous Http request handler interface. ////// public void ProcessRequest(HttpContext context) { throw new HttpException(405, SR.GetString(SR.Path_forbidden, context.Request.HttpMethod)); } ///Drives /// web processing execution. ////// public bool IsReusable { get { return true; } } } ///Indicates whether an HttpForbiddenHandler instance can be recycled and used /// for another request. ////// internal class HttpNotImplementedHandler : IHttpHandler { internal HttpNotImplementedHandler() { } ///Provides a synchronous Http request handler interface. ////// public void ProcessRequest(HttpContext context) { throw new HttpException(501, SR.GetString(SR.Method_for_path_not_implemented, context.Request.HttpMethod, context.Request.Path)); } ///Drives web processing execution. ////// public bool IsReusable { get { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ //Indicates whether an HttpNotImplementedHandler instance can be recycled and /// used for another request. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Synchronous Http request handler interface * * Copyright (c) 1998 Microsoft Corporation */ namespace System.Web { ////// internal class HttpNotFoundHandler : IHttpHandler { internal HttpNotFoundHandler() { } ///Provides a synchronous Http request handler interface. ////// public void ProcessRequest(HttpContext context) { PerfCounters.IncrementCounter(AppPerfCounter.REQUESTS_NOT_FOUND); throw new HttpException(404, SR.GetString(SR.Path_not_found, context.Request.Path)); } ///Drives web processing execution. ////// public bool IsReusable { get { return true; } } } internal class HttpForbiddenHandler : IHttpHandler { internal HttpForbiddenHandler() { } ///Indicates whether an HttpNotFoundHandler instance can be recycled and used /// for another request. ////// public void ProcessRequest(HttpContext context) { PerfCounters.IncrementCounter(AppPerfCounter.REQUESTS_NOT_FOUND); throw new HttpException(403, SR.GetString(SR.Path_forbidden, context.Request.Path)); } ///Drives web processing execution. ////// public bool IsReusable { get { return true; } } } ///Indicates whether an HttpForbiddenHandler instance can be recycled and used /// for another request. ////// internal class HttpMethodNotAllowedHandler : IHttpHandler { internal HttpMethodNotAllowedHandler() { } ///Provides a synchronous Http request handler interface. ////// public void ProcessRequest(HttpContext context) { throw new HttpException(405, SR.GetString(SR.Path_forbidden, context.Request.HttpMethod)); } ///Drives /// web processing execution. ////// public bool IsReusable { get { return true; } } } ///Indicates whether an HttpForbiddenHandler instance can be recycled and used /// for another request. ////// internal class HttpNotImplementedHandler : IHttpHandler { internal HttpNotImplementedHandler() { } ///Provides a synchronous Http request handler interface. ////// public void ProcessRequest(HttpContext context) { throw new HttpException(501, SR.GetString(SR.Method_for_path_not_implemented, context.Request.HttpMethod, context.Request.Path)); } ///Drives web processing execution. ////// public bool IsReusable { get { return true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Indicates whether an HttpNotImplementedHandler instance can be recycled and /// used for another request. ///
Link Menu
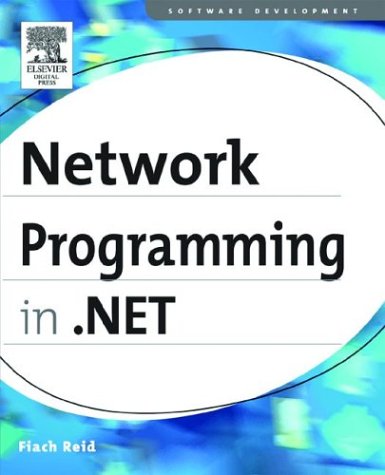
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CustomTypeDescriptor.cs
- FreezableOperations.cs
- StandardToolWindows.cs
- base64Transforms.cs
- UserMapPath.cs
- TemplateManager.cs
- ExtensionSimplifierMarkupObject.cs
- MarkedHighlightComponent.cs
- DoubleAverageAggregationOperator.cs
- InputBuffer.cs
- Common.cs
- ButtonDesigner.cs
- TargetControlTypeCache.cs
- Attributes.cs
- ObjectSecurity.cs
- HandleExceptionArgs.cs
- FakeModelItemImpl.cs
- EventRoute.cs
- DataGridViewAutoSizeModeEventArgs.cs
- SqlColumnizer.cs
- OracleDateTime.cs
- SqlConnectionManager.cs
- ConfigurationPermission.cs
- MetadataFile.cs
- RecognizedPhrase.cs
- RawTextInputReport.cs
- CellIdBoolean.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- BasicSecurityProfileVersion.cs
- Application.cs
- MsmqTransportElement.cs
- EntitySetBase.cs
- SqlAliasesReferenced.cs
- CroppedBitmap.cs
- ValidationResult.cs
- FacetDescriptionElement.cs
- LinqDataSourceInsertEventArgs.cs
- baseaxisquery.cs
- nulltextnavigator.cs
- FromRequest.cs
- PointConverter.cs
- IriParsingElement.cs
- HttpHandlerActionCollection.cs
- XmlByteStreamReader.cs
- CmsUtils.cs
- PermissionSetEnumerator.cs
- ScrollProviderWrapper.cs
- SizeValueSerializer.cs
- AppSettingsExpressionBuilder.cs
- PenCursorManager.cs
- PngBitmapDecoder.cs
- AtomMaterializer.cs
- X509SecurityToken.cs
- DataGridSortCommandEventArgs.cs
- WorkerRequest.cs
- HebrewCalendar.cs
- LogFlushAsyncResult.cs
- _Win32.cs
- SegmentInfo.cs
- ConstraintConverter.cs
- entitydatasourceentitysetnameconverter.cs
- PassportPrincipal.cs
- ValidationSummary.cs
- ServiceOperationParameter.cs
- NamespaceEmitter.cs
- RootBrowserWindowAutomationPeer.cs
- WebBrowserContainer.cs
- MetabaseServerConfig.cs
- Dictionary.cs
- ZipPackage.cs
- StringDictionary.cs
- CngKey.cs
- _NestedSingleAsyncResult.cs
- JoinGraph.cs
- DockAndAnchorLayout.cs
- DocumentXPathNavigator.cs
- EdmProperty.cs
- WpfPayload.cs
- KeyTime.cs
- OutputCacheProfileCollection.cs
- XmlNodeChangedEventManager.cs
- SchemaImporter.cs
- TripleDES.cs
- BmpBitmapEncoder.cs
- DataGridItem.cs
- AlternationConverter.cs
- CommandHelper.cs
- FontSource.cs
- SocketException.cs
- HandlerFactoryWrapper.cs
- DataServiceResponse.cs
- shaperfactoryquerycacheentry.cs
- ResourceReferenceExpression.cs
- SelectionListDesigner.cs
- FormDesigner.cs
- Roles.cs
- StylusPointPropertyInfo.cs
- TagMapInfo.cs
- AlternateView.cs
- CompositeActivityValidator.cs