Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / WindowsUserNameSecurityTokenAuthenticator.cs / 1305376 / WindowsUserNameSecurityTokenAuthenticator.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Collections.ObjectModel; using System.ComponentModel; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; using System.Runtime.InteropServices; using System.Security; using System.Security.Principal; public class WindowsUserNameSecurityTokenAuthenticator : UserNameSecurityTokenAuthenticator { bool includeWindowsGroups; public WindowsUserNameSecurityTokenAuthenticator() : this(WindowsClaimSet.DefaultIncludeWindowsGroups) { } public WindowsUserNameSecurityTokenAuthenticator(bool includeWindowsGroups) { this.includeWindowsGroups = includeWindowsGroups; } protected override ReadOnlyCollectionValidateUserNamePasswordCore(string userName, string password) { string domain = null; string[] strings = userName.Split('\\'); if (strings.Length != 1) { if (strings.Length != 2 || String.IsNullOrEmpty(strings[0])) { // Only support one slash and domain cannot be empty (consistent with windowslogon). throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument(SR.GetString(SR.IncorrectUserNameFormat)); } // This is the downlevel case - domain\userName userName = strings[1]; domain = strings[0]; } const uint LOGON32_PROVIDER_DEFAULT = 0; const uint LOGON32_LOGON_NETWORK_CLEARTEXT = 8; SafeCloseHandle tokenHandle = null; try { if (!NativeMethods.LogonUser(userName, domain, password, LOGON32_LOGON_NETWORK_CLEARTEXT, LOGON32_PROVIDER_DEFAULT, out tokenHandle)) { int error = Marshal.GetLastWin32Error(); throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new SecurityTokenValidationException(SR.GetString(SR.FailLogonUser, userName), new Win32Exception(error))); } WindowsIdentity windowsIdentity = new WindowsIdentity(tokenHandle.DangerousGetHandle(), SecurityUtils.AuthTypeBasic); WindowsClaimSet claimSet = new WindowsClaimSet(windowsIdentity, SecurityUtils.AuthTypeBasic, this.includeWindowsGroups, false); return SecurityUtils.CreateAuthorizationPolicies(claimSet, claimSet.ExpirationTime); } finally { if (tokenHandle != null) tokenHandle.Close(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
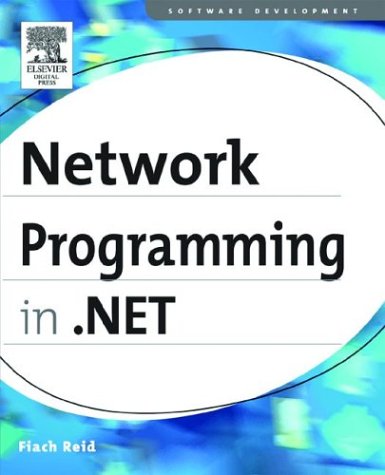
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SessionStateUtil.cs
- Utils.cs
- IRCollection.cs
- BuildManager.cs
- PersonalizationProviderHelper.cs
- KnownIds.cs
- WebScriptMetadataMessageEncoderFactory.cs
- CustomLineCap.cs
- MaterialCollection.cs
- SeverityFilter.cs
- GlyphElement.cs
- HierarchicalDataTemplate.cs
- NonClientArea.cs
- DateTimeStorage.cs
- AdPostCacheSubstitution.cs
- TemplateXamlParser.cs
- DocumentViewerHelper.cs
- TraceLevelStore.cs
- ExpressionEditor.cs
- EventMappingSettingsCollection.cs
- JavaScriptString.cs
- BaseParaClient.cs
- JapaneseLunisolarCalendar.cs
- MustUnderstandBehavior.cs
- SecurityDescriptor.cs
- SafeLocalMemHandle.cs
- SafeNativeMethods.cs
- OracleInternalConnection.cs
- ProxyWebPartManager.cs
- StylusShape.cs
- StoreUtilities.cs
- IdleTimeoutMonitor.cs
- MetadataHelper.cs
- MaterialGroup.cs
- DataSourceUtil.cs
- unsafenativemethodsother.cs
- NonValidatingSecurityTokenAuthenticator.cs
- dtdvalidator.cs
- ProgressBarAutomationPeer.cs
- FormClosingEvent.cs
- ButtonBaseAdapter.cs
- EditableRegion.cs
- HttpServerVarsCollection.cs
- TextEditorMouse.cs
- SQLInt16Storage.cs
- HashRepartitionEnumerator.cs
- TableLayoutSettings.cs
- DeleteIndexBinder.cs
- OperationSelectorBehavior.cs
- MediaTimeline.cs
- IListConverters.cs
- RulePatternOps.cs
- Pair.cs
- ClientFormsIdentity.cs
- ErasingStroke.cs
- XmlDeclaration.cs
- WebPartChrome.cs
- ScrollChangedEventArgs.cs
- RowToParametersTransformer.cs
- TextRange.cs
- ImageDrawing.cs
- UpWmlMobileTextWriter.cs
- _KerberosClient.cs
- HttpChannelFactory.cs
- AuthStoreRoleProvider.cs
- ProtocolInformationReader.cs
- InlineCollection.cs
- ObjectAnimationBase.cs
- ObjectDataSourceFilteringEventArgs.cs
- SqlFunctions.cs
- FamilyCollection.cs
- XPathConvert.cs
- WindowsSysHeader.cs
- StringConcat.cs
- MD5HashHelper.cs
- HealthMonitoringSectionHelper.cs
- SystemException.cs
- DtdParser.cs
- BufferedWebEventProvider.cs
- NamespaceList.cs
- SoapConverter.cs
- PkcsUtils.cs
- StringToken.cs
- Menu.cs
- WebMessageEncoderFactory.cs
- CodeLinePragma.cs
- TemplatedWizardStep.cs
- ArrayTypeMismatchException.cs
- Parser.cs
- JsonServiceDocumentSerializer.cs
- ClientType.cs
- FileDialog.cs
- FileInfo.cs
- WebBrowserContainer.cs
- CaseInsensitiveOrdinalStringComparer.cs
- Label.cs
- HttpServerVarsCollection.cs
- TextEncodedRawTextWriter.cs
- SafeHandle.cs
- ValueUnavailableException.cs