Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / MediaTimeline.cs / 1 / MediaTimeline.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: MediaTimeline.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using System.Collections; using System.Collections.Generic; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region MediaTimeline ////// MediaTimeline functions as a template for creating MediaClocks. Whenever /// you create a MediaClock it inherits all of the properties and events of /// the MediaTimeline. Whenever you change a property or register for an /// event on a MediaTimeline, all of those changes get propagated down to /// every MediaClock created off of that MediaTimeline (and any future /// MediaClocks created from it too). /// public partial class MediaTimeline : Timeline, IUriContext { internal const uint LastTimelineFlag = 0x1; #region Constructor ////// Creates a new MediaTimeline. /// /// Source of the media. public MediaTimeline(Uri source) : this() { Source = source; } ////// Creates a new MediaTimeline. /// /// Context used to resolve relative URIs /// Source of the media. internal MediaTimeline(ITypeDescriptorContext context, Uri source) : this() { _context = context; Source = source; } ////// Creates a new MediaTimeline. /// public MediaTimeline() { } ////// Creates a new MediaTimeline. /// /// The value for the BeginTime property public MediaTimeline(NullablebeginTime) : this() { BeginTime = beginTime; } /// /// Creates a new MediaTimeline. /// /// The value for the BeginTime property /// The value for the Duration property public MediaTimeline(NullablebeginTime, Duration duration) : this() { BeginTime = beginTime; Duration = duration; } /// /// Creates a new MediaTimeline. /// /// The value for the BeginTime property /// The value for the Duration property /// The value for the RepeatBehavior property public MediaTimeline(NullablebeginTime, Duration duration, RepeatBehavior repeatBehavior) : this() { BeginTime = beginTime; Duration = duration; RepeatBehavior = repeatBehavior; } #endregion #region IUriContext implementation /// /// Base Uri to use when resolving relative Uri's /// Uri IUriContext.BaseUri { get { return _baseUri; } set { _baseUri = value; } } #endregion #region Timeline ////// Called by the Clock to create a type-specific clock for this /// timeline. /// ////// A clock for this timeline. /// ////// If a derived class overrides this method, it should only create /// and return an object of a class inheriting from Clock. /// protected internal override Clock AllocateClock() { if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Media_UriNotSpecified)); } MediaClock mediaClock = new MediaClock(this); return mediaClock; } ////// Called by the base Freezable class to make this object /// frozen. /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); if (!canFreeze) { return false; } // First, if checking, make sure that we don't have any expressions // on our properties. (base.FreezeCore takes care of animations) if (isChecking) { canFreeze &= !HasExpression(LookupEntry(SourceProperty.GlobalIndex), SourceProperty); } return canFreeze; } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { MediaTimeline sourceTimeline = (MediaTimeline) sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceTimeline); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { MediaTimeline sourceTimeline = (MediaTimeline) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceTimeline); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable source) { MediaTimeline sourceTimeline = (MediaTimeline) source; base.GetAsFrozenCore(source); CopyCommon(sourceTimeline); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable source) { MediaTimeline sourceTimeline = (MediaTimeline) source; base.GetCurrentValueAsFrozenCore(source); CopyCommon(sourceTimeline); } private void CopyCommon(MediaTimeline sourceTimeline) { _context = sourceTimeline._context; _baseUri = sourceTimeline._baseUri; } ///Freezable.GetCurrentValueAsFrozenCore . ////// Creates a new MediaClock using this MediaTimeline. /// ///A new MediaClock. public new MediaClock CreateClock() { return (MediaClock)base.CreateClock(); } ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { MediaClock mc = (MediaClock)clock; if (mc.Player == null) { return Duration.Automatic; } else { return mc.Player.NaturalDuration; } } #endregion #region ToString ////// Persist MediaTimeline in a string /// public override string ToString() { if (null == Source) throw new InvalidOperationException(SR.Get(SRID.Media_UriNotSpecified)); return Source.ToString(); } #endregion internal ITypeDescriptorContext _context = null; private Uri _baseUri = null; } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: MediaTimeline.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using System.Collections; using System.Collections.Generic; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region MediaTimeline ////// MediaTimeline functions as a template for creating MediaClocks. Whenever /// you create a MediaClock it inherits all of the properties and events of /// the MediaTimeline. Whenever you change a property or register for an /// event on a MediaTimeline, all of those changes get propagated down to /// every MediaClock created off of that MediaTimeline (and any future /// MediaClocks created from it too). /// public partial class MediaTimeline : Timeline, IUriContext { internal const uint LastTimelineFlag = 0x1; #region Constructor ////// Creates a new MediaTimeline. /// /// Source of the media. public MediaTimeline(Uri source) : this() { Source = source; } ////// Creates a new MediaTimeline. /// /// Context used to resolve relative URIs /// Source of the media. internal MediaTimeline(ITypeDescriptorContext context, Uri source) : this() { _context = context; Source = source; } ////// Creates a new MediaTimeline. /// public MediaTimeline() { } ////// Creates a new MediaTimeline. /// /// The value for the BeginTime property public MediaTimeline(NullablebeginTime) : this() { BeginTime = beginTime; } /// /// Creates a new MediaTimeline. /// /// The value for the BeginTime property /// The value for the Duration property public MediaTimeline(NullablebeginTime, Duration duration) : this() { BeginTime = beginTime; Duration = duration; } /// /// Creates a new MediaTimeline. /// /// The value for the BeginTime property /// The value for the Duration property /// The value for the RepeatBehavior property public MediaTimeline(NullablebeginTime, Duration duration, RepeatBehavior repeatBehavior) : this() { BeginTime = beginTime; Duration = duration; RepeatBehavior = repeatBehavior; } #endregion #region IUriContext implementation /// /// Base Uri to use when resolving relative Uri's /// Uri IUriContext.BaseUri { get { return _baseUri; } set { _baseUri = value; } } #endregion #region Timeline ////// Called by the Clock to create a type-specific clock for this /// timeline. /// ////// A clock for this timeline. /// ////// If a derived class overrides this method, it should only create /// and return an object of a class inheriting from Clock. /// protected internal override Clock AllocateClock() { if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Media_UriNotSpecified)); } MediaClock mediaClock = new MediaClock(this); return mediaClock; } ////// Called by the base Freezable class to make this object /// frozen. /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); if (!canFreeze) { return false; } // First, if checking, make sure that we don't have any expressions // on our properties. (base.FreezeCore takes care of animations) if (isChecking) { canFreeze &= !HasExpression(LookupEntry(SourceProperty.GlobalIndex), SourceProperty); } return canFreeze; } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { MediaTimeline sourceTimeline = (MediaTimeline) sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceTimeline); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { MediaTimeline sourceTimeline = (MediaTimeline) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceTimeline); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable source) { MediaTimeline sourceTimeline = (MediaTimeline) source; base.GetAsFrozenCore(source); CopyCommon(sourceTimeline); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable source) { MediaTimeline sourceTimeline = (MediaTimeline) source; base.GetCurrentValueAsFrozenCore(source); CopyCommon(sourceTimeline); } private void CopyCommon(MediaTimeline sourceTimeline) { _context = sourceTimeline._context; _baseUri = sourceTimeline._baseUri; } ///Freezable.GetCurrentValueAsFrozenCore . ////// Creates a new MediaClock using this MediaTimeline. /// ///A new MediaClock. public new MediaClock CreateClock() { return (MediaClock)base.CreateClock(); } ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { MediaClock mc = (MediaClock)clock; if (mc.Player == null) { return Duration.Automatic; } else { return mc.Player.NaturalDuration; } } #endregion #region ToString ////// Persist MediaTimeline in a string /// public override string ToString() { if (null == Source) throw new InvalidOperationException(SR.Get(SRID.Media_UriNotSpecified)); return Source.ToString(); } #endregion internal ITypeDescriptorContext _context = null; private Uri _baseUri = null; } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
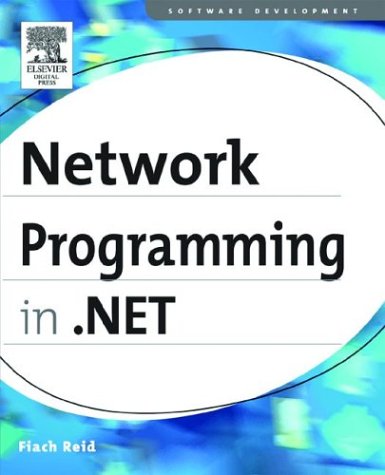
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaElement.cs
- AttributeEmitter.cs
- SharedPersonalizationStateInfo.cs
- ToolZone.cs
- ConfigXmlWhitespace.cs
- EditorBrowsableAttribute.cs
- ExternalCalls.cs
- TextEvent.cs
- _NegoStream.cs
- WebPartDescription.cs
- ProcessManager.cs
- OperationAbortedException.cs
- EditorBrowsableAttribute.cs
- QueryCursorEventArgs.cs
- HttpListenerPrefixCollection.cs
- WSIdentityFaultException.cs
- EncoderExceptionFallback.cs
- ColorMap.cs
- TextTreeUndo.cs
- HttpHandlerAction.cs
- MemoryMappedFile.cs
- PolyLineSegment.cs
- CodeTypeParameterCollection.cs
- FontWeight.cs
- DPAPIProtectedConfigurationProvider.cs
- FillBehavior.cs
- Debug.cs
- ThumbAutomationPeer.cs
- IListConverters.cs
- IImplicitResourceProvider.cs
- Group.cs
- RadioButton.cs
- SystemIcmpV6Statistics.cs
- HostProtectionPermission.cs
- Visual3D.cs
- RadialGradientBrush.cs
- SQLByte.cs
- SqlUserDefinedTypeAttribute.cs
- DbProviderServices.cs
- SQLDoubleStorage.cs
- ApplyTemplatesAction.cs
- DebugView.cs
- Span.cs
- DesignTimeVisibleAttribute.cs
- CategoryGridEntry.cs
- RuntimeHelpers.cs
- SQlBooleanStorage.cs
- ObjectContextServiceProvider.cs
- ASCIIEncoding.cs
- StateChangeEvent.cs
- SqlReferenceCollection.cs
- UInt16.cs
- WebControlAdapter.cs
- SqlDataSourceCommandParser.cs
- HttpBindingExtension.cs
- NameValuePair.cs
- InvalidProgramException.cs
- PreProcessor.cs
- CngKey.cs
- BindingExpression.cs
- ReflectPropertyDescriptor.cs
- ContentPlaceHolder.cs
- DataProtection.cs
- serverconfig.cs
- BamlRecordHelper.cs
- CellParagraph.cs
- RenderDataDrawingContext.cs
- SourceFileBuildProvider.cs
- XPathDocumentBuilder.cs
- _ConnectStream.cs
- StateMachineHelpers.cs
- TranslateTransform3D.cs
- Variant.cs
- Token.cs
- MinMaxParagraphWidth.cs
- DirectoryInfo.cs
- AutomationPeer.cs
- GraphicsContext.cs
- ResourcePermissionBase.cs
- AlignmentYValidation.cs
- DependencySource.cs
- TextSimpleMarkerProperties.cs
- XPathDocumentBuilder.cs
- HasRunnableWorkflowEvent.cs
- MsmqIntegrationMessageProperty.cs
- NumberSubstitution.cs
- ProtectedConfigurationSection.cs
- TextTreeTextBlock.cs
- EditorAttribute.cs
- DBPropSet.cs
- Stream.cs
- SystemResourceHost.cs
- RMPublishingDialog.cs
- BuildProvider.cs
- DirectoryInfo.cs
- DataTableMapping.cs
- Rotation3D.cs
- LabelLiteral.cs
- PassportAuthenticationModule.cs
- ASCIIEncoding.cs