Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / Emitters / AttributeEmitter.cs / 1 / AttributeEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); object[] keys = emitter.Item.KeyMembers.Select(km => (object) km.Name).ToArray(); typeDecl.CustomAttributes.Add(EmitSimpleAttribute(Utils.WebFrameworkCommonNamespace + "." + "DataServiceKeyAttribute", keys)); } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // not emitting System.Runtime.Serializaton.DataContractAttribute // not emitting System.Serializable } #region Static Methods ////// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { // not emitting System.Xml.Serialization.XmlIgnoreAttribute // not emitting System.Xml.Serialization.SoapIgnoreAttribute } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { // not emitting System.ComponentModel.BrowsableAttribute } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.CodeDom; using System.Collections.Generic; using System.Data.Services.Design; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Linq; namespace System.Data.EntityModel.Emitters { ////// Summary description for AttributeEmitter. /// internal sealed class AttributeEmitter { TypeReference _typeReference; internal TypeReference TypeReference { get { return _typeReference; } } internal AttributeEmitter(TypeReference typeReference) { _typeReference = typeReference; } ////// The method to be called to create the type level attributes for the ItemTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(EntityTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); object[] keys = emitter.Item.KeyMembers.Select(km => (object) km.Name).ToArray(); typeDecl.CustomAttributes.Add(EmitSimpleAttribute(Utils.WebFrameworkCommonNamespace + "." + "DataServiceKeyAttribute", keys)); } ////// The method to be called to create the type level attributes for the StructuredTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(StructuredTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // nothing to do here yet } ////// The method to be called to create the type level attributes for the SchemaTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(SchemaTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); } ////// The method to be called to create the property level attributes for the PropertyEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. /// Additional attributes to emit public void EmitPropertyAttributes(PropertyEmitter emitter, CodeMemberProperty propertyDecl, ListadditionalAttributes) { if (additionalAttributes != null && additionalAttributes.Count > 0) { try { propertyDecl.CustomAttributes.AddRange(additionalAttributes.ToArray()); } catch (ArgumentNullException e) { emitter.Generator.AddError(Strings.InvalidAttributeSuppliedForProperty(emitter.Item.Name), ModelBuilderErrorCode.InvalidAttributeSuppliedForProperty, EdmSchemaErrorSeverity.Error, e); } } } /// /// The method to be called to create the type level attributes for the NestedTypeEmitter /// /// The strongly typed emitter /// The type declaration to add the attribues to. public void EmitTypeAttributes(ComplexTypeEmitter emitter, CodeTypeDeclaration typeDecl) { Debug.Assert(emitter != null, "emitter should not be null"); Debug.Assert(typeDecl != null, "typeDecl should not be null"); // not emitting System.Runtime.Serializaton.DataContractAttribute // not emitting System.Serializable } #region Static Methods ////// /// /// /// ///public CodeAttributeDeclaration EmitSimpleAttribute(string attributeType, params object[] arguments) { CodeAttributeDeclaration attribute = new CodeAttributeDeclaration(TypeReference.FromString(attributeType, true)); AddAttributeArguments(attribute, arguments); return attribute; } /// /// /// /// /// public static void AddAttributeArguments(CodeAttributeDeclaration attribute, object[] arguments) { foreach (object argument in arguments) { CodeExpression expression = argument as CodeExpression; if (expression == null) expression = new CodePrimitiveExpression(argument); attribute.Arguments.Add(new CodeAttributeArgument(expression)); } } ////// Adds an XmlIgnore attribute to the given property declaration. This is /// used to explicitly skip certain properties during XML serialization. /// /// the property to mark with XmlIgnore public void AddIgnoreAttributes(CodeMemberProperty propertyDecl) { // not emitting System.Xml.Serialization.XmlIgnoreAttribute // not emitting System.Xml.Serialization.SoapIgnoreAttribute } ////// Adds an Browsable(false) attribute to the given property declaration. /// This is used to explicitly avoid display property in the PropertyGrid. /// /// the property to mark with XmlIgnore public void AddBrowsableAttribute(CodeMemberProperty propertyDecl) { // not emitting System.ComponentModel.BrowsableAttribute } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
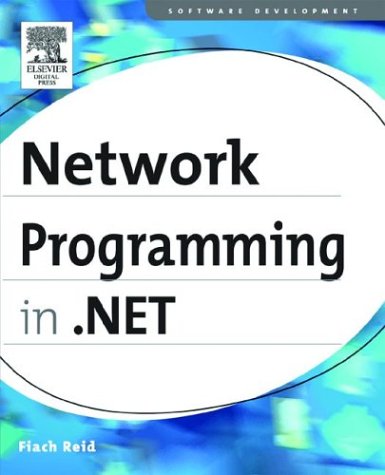
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DispatcherTimer.cs
- BufferModesCollection.cs
- ComponentTray.cs
- ValueHandle.cs
- RelationshipEntry.cs
- CriticalExceptions.cs
- Comparer.cs
- Evaluator.cs
- CardSpaceShim.cs
- FilterElement.cs
- PenThreadPool.cs
- StorageBasedPackageProperties.cs
- ByteStorage.cs
- ValidatorCompatibilityHelper.cs
- UpdatePanel.cs
- HtmlHead.cs
- RootBrowserWindowProxy.cs
- DataQuery.cs
- _UriSyntax.cs
- ServiceChannelProxy.cs
- DetailsView.cs
- XmlSchemaSimpleTypeRestriction.cs
- SupportsEventValidationAttribute.cs
- CollectionBuilder.cs
- updatecommandorderer.cs
- ProcessModuleCollection.cs
- Itemizer.cs
- FileLevelControlBuilderAttribute.cs
- ProcessHostFactoryHelper.cs
- Sql8ExpressionRewriter.cs
- SqlDataSourceView.cs
- GroupedContextMenuStrip.cs
- DataGridColumnHeadersPresenter.cs
- AutomationProperties.cs
- EnvelopedSignatureTransform.cs
- SmiEventStream.cs
- String.cs
- SimpleTextLine.cs
- SerializationInfo.cs
- TileModeValidation.cs
- HttpFormatExtensions.cs
- PathFigureCollectionConverter.cs
- DynamicEndpoint.cs
- FixUpCollection.cs
- MemoryFailPoint.cs
- XmlSchemaInferenceException.cs
- PropertyRecord.cs
- XmlSerializerFactory.cs
- FixedSOMGroup.cs
- Grant.cs
- SqlNotificationEventArgs.cs
- XmlHelper.cs
- DataGridViewRowsAddedEventArgs.cs
- DataServices.cs
- basevalidator.cs
- GridViewColumnHeaderAutomationPeer.cs
- HtmlValidatorAdapter.cs
- TreeNodeStyleCollection.cs
- CompiledQueryCacheKey.cs
- IntegrationExceptionEventArgs.cs
- CqlParser.cs
- XmlDictionaryReaderQuotas.cs
- SaveFileDialog.cs
- EncoderReplacementFallback.cs
- SchemaTableOptionalColumn.cs
- _emptywebproxy.cs
- RouteCollection.cs
- SafeIUnknown.cs
- InputMethodStateTypeInfo.cs
- ErrorWrapper.cs
- EdmType.cs
- XmlRawWriterWrapper.cs
- DataReceivedEventArgs.cs
- InternalCache.cs
- LineUtil.cs
- WorkItem.cs
- CompressedStack.cs
- ChannelSinkStacks.cs
- TaiwanCalendar.cs
- TextServicesContext.cs
- SettingsBase.cs
- XmlAttributeCache.cs
- TripleDES.cs
- Decoder.cs
- RootProfilePropertySettingsCollection.cs
- SQLBinaryStorage.cs
- ThreadAbortException.cs
- ByteStream.cs
- ZipArchive.cs
- FrameworkRichTextComposition.cs
- InstanceData.cs
- DesignerResources.cs
- TaiwanLunisolarCalendar.cs
- RSATokenProvider.cs
- SystemFonts.cs
- LinqDataSourceDisposeEventArgs.cs
- ObjectParameter.cs
- ExpressionPrinter.cs
- WinEventWrap.cs
- PrintPreviewGraphics.cs