Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / CollectionBuilder.cs / 1 / CollectionBuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Classes related to complex property support. * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.Collections; using System.Reflection; using System.Web.Util; [AttributeUsage(AttributeTargets.Property)] internal sealed class IgnoreUnknownContentAttribute : Attribute { internal IgnoreUnknownContentAttribute() {} } ////// internal sealed class CollectionBuilder : ControlBuilder { private Type _itemType; private bool _ignoreUnknownContent; internal CollectionBuilder(bool ignoreUnknownContent) { _ignoreUnknownContent = ignoreUnknownContent; } ///[To be supplied.] ////// public override void Init(TemplateParser parser, ControlBuilder parentBuilder, Type type, string tagName, string ID, IDictionary attribs) { base.Init(parser, parentBuilder, type /*type*/, tagName, ID, attribs); // PropertyInfo propInfo = parentBuilder.ControlType.GetProperty( tagName, BindingFlags.Public | BindingFlags.Instance | BindingFlags.Static | BindingFlags.IgnoreCase); SetControlType(propInfo.PropertyType); Debug.Assert(ControlType != null, "ControlType != null"); BindingFlags bindingFlags = BindingFlags.Public | BindingFlags.Instance; // Look for an "item" property on the collection that takes in an integer index // (similar to IList::Item) propInfo = ControlType.GetProperty("Item", bindingFlags, null, null, new Type[] { typeof(int) }, null); if (propInfo == null) { // fall-back on finding a non-specific Item property // a type with overloaded indexed properties will result in an exception however propInfo = ControlType.GetProperty("Item", bindingFlags); } // If we got one, use it to determine the type of the items if (propInfo != null) _itemType = propInfo.PropertyType; } // This code is only executed when used from the desiger ///[To be supplied.] ////// public override object BuildObject() { return this; } ///[To be supplied.] ////// public override Type GetChildControlType(string tagName, IDictionary attribs) { Type childType = Parser.MapStringToType(tagName, attribs); // If possible, check if the item is of the required type if (_itemType != null) { if (!_itemType.IsAssignableFrom(childType)) { if (_ignoreUnknownContent) return null; string controlTypeName = String.Empty; if (ControlType != null) { controlTypeName = ControlType.FullName; } else { controlTypeName = TagName; } throw new HttpException(SR.GetString( SR.Invalid_collection_item_type, new String[] { controlTypeName, _itemType.FullName, tagName, childType.FullName})); } } return childType; } ///[To be supplied.] ////// public override void AppendLiteralString(string s) { if (_ignoreUnknownContent) return; // Don't allow non-whitespace literal content if (!Util.IsWhiteSpaceString(s)) { throw new HttpException(SR.GetString( SR.Literal_content_not_allowed, ControlType.FullName, s.Trim())); } } } }[To be supplied.] ///
Link Menu
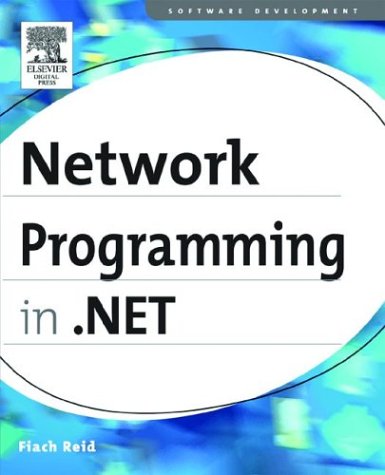
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Number.cs
- StorageBasedPackageProperties.cs
- CrossAppDomainChannel.cs
- _SSPISessionCache.cs
- EditorZone.cs
- SQLInt32.cs
- StreamReader.cs
- CompiledELinqQueryState.cs
- SimplePropertyEntry.cs
- PenContext.cs
- MatrixUtil.cs
- BlurEffect.cs
- TextBoxBase.cs
- UIElement.cs
- BigIntegerStorage.cs
- Clause.cs
- ConvertBinder.cs
- VarRemapper.cs
- StyleTypedPropertyAttribute.cs
- COM2ComponentEditor.cs
- XmlILOptimizerVisitor.cs
- MarkupObject.cs
- ForwardPositionQuery.cs
- XmlEncoding.cs
- DeviceFiltersSection.cs
- Authorization.cs
- DuplicateMessageDetector.cs
- _LocalDataStoreMgr.cs
- Mapping.cs
- PerformanceCounterLib.cs
- AccessedThroughPropertyAttribute.cs
- SweepDirectionValidation.cs
- RightNameExpirationInfoPair.cs
- TableProviderWrapper.cs
- EventProviderBase.cs
- ConstructorExpr.cs
- ParameterCollection.cs
- ImageListStreamer.cs
- OutOfProcStateClientManager.cs
- CompositeFontFamily.cs
- UInt64.cs
- SQLMoneyStorage.cs
- ItemMap.cs
- Helpers.cs
- StorageEntityTypeMapping.cs
- XmlCountingReader.cs
- ListViewGroupConverter.cs
- AddInActivator.cs
- GAC.cs
- FrameworkElementFactoryMarkupObject.cs
- ExpressionPrefixAttribute.cs
- IxmlLineInfo.cs
- Empty.cs
- DataContractSerializerElement.cs
- PropertyEmitter.cs
- DEREncoding.cs
- CharStorage.cs
- HostingEnvironmentSection.cs
- DataGridViewCellLinkedList.cs
- StateMachineSubscriptionManager.cs
- SoapMessage.cs
- AsyncDataRequest.cs
- DataFieldEditor.cs
- WebPartActionVerb.cs
- NegationPusher.cs
- LockCookie.cs
- SurrogateEncoder.cs
- PixelShader.cs
- Site.cs
- PathTooLongException.cs
- LocalFileSettingsProvider.cs
- EventListenerClientSide.cs
- UIPermission.cs
- ReverseInheritProperty.cs
- TextSpan.cs
- DataBindingCollection.cs
- TimeoutTimer.cs
- SymbolMethod.cs
- UrlMappingsModule.cs
- InvalidateEvent.cs
- ImmutablePropertyDescriptorGridEntry.cs
- SocketInformation.cs
- EntityContainer.cs
- StrokeNode.cs
- SinglePageViewer.cs
- SafeFileMappingHandle.cs
- AttributeSetAction.cs
- QueryCacheKey.cs
- ValueTable.cs
- WpfXamlLoader.cs
- MimePart.cs
- OutputCacheProfileCollection.cs
- WindowsFormsHostPropertyMap.cs
- TimerElapsedEvenArgs.cs
- BindableTemplateBuilder.cs
- DefaultValueAttribute.cs
- QuadraticBezierSegment.cs
- StringUtil.cs
- OracleColumn.cs
- ControlAdapter.cs