Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / RSATokenProvider.cs / 1 / RSATokenProvider.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- // // Presharp uses the c# pragma mechanism to supress its warnings. // These are not recognised by the base compiler so we need to explictly // disable the following warnings. See http://winweb/cse/Tools/PREsharp/userguide/default.asp // for details. // #pragma warning disable 1634, 1691 // unknown message, unknown pragma namespace Microsoft.InfoCards { using System; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.ServiceModel; using System.ServiceModel.Security; using System.ServiceModel.Security.Tokens; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.IdentityModel; using System.Security.Cryptography.Xml; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; using System.Collections.ObjectModel; using System.Collections.Generic; // // This class is used to provide a custom token provider for adding an endorsing signature token to the RST // message sent to a managed card STS. The endorsing signature must be added if the UseKey field is present // in the RST. // internal class RSATokenProvider : SecurityTokenProvider, IDisposable { InfoCardPolicy m_policy; InfoCard m_card; SelfIssuedAuthProofToken m_RSAToken; public RSATokenProvider( InfoCardPolicy policy, InfoCard card ) { IDT.ThrowInvalidArgumentConditional( null == policy, "policy" ); IDT.ThrowInvalidArgumentConditional( null == card, "card" ); m_policy = policy; m_card = card; } public void Dispose() { // // We must dispose this token explicitly. // if ( null != m_RSAToken ) { m_RSAToken.Dispose(); m_RSAToken = null; } } // // Summary // Retrieves a token from the system // // Parameters // timeout - The time span till the call times out // // Returns // The security token. // protected override SecurityToken GetTokenCore(TimeSpan timeout) { if( null == m_RSAToken ) { // // The SelfIssuedAuthProofToken should be renamed. In this case it's just acting as a generic // wrapper for an RSA key. // m_RSAToken = new SelfIssuedAuthProofToken( m_card.GetPrivateCryptography( m_policy.Recipient.GetIdentifier() ), DateTime.UtcNow + timeout ); } return m_RSAToken; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
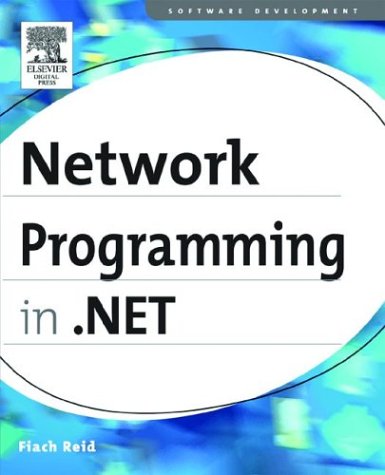
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SiteMapDataSourceView.cs
- LogEntryDeserializer.cs
- XamlSerializerUtil.cs
- HttpException.cs
- ResourceLoader.cs
- BehaviorDragDropEventArgs.cs
- BitmapCodecInfoInternal.cs
- RenderingEventArgs.cs
- ParameterModifier.cs
- SizeChangedEventArgs.cs
- GestureRecognizer.cs
- RelatedEnd.cs
- ObjectHandle.cs
- OverrideMode.cs
- ToolstripProfessionalRenderer.cs
- GrammarBuilderPhrase.cs
- LazyTextWriterCreator.cs
- NamespaceCollection.cs
- FileEnumerator.cs
- DeviceOverridableAttribute.cs
- ISFClipboardData.cs
- HtmlInputFile.cs
- RegionData.cs
- DivideByZeroException.cs
- OrderedDictionaryStateHelper.cs
- XmlWellformedWriter.cs
- SystemMulticastIPAddressInformation.cs
- WebHttpEndpointElement.cs
- Rule.cs
- SafeReadContext.cs
- linebase.cs
- DragDrop.cs
- CodeTypeDelegate.cs
- SessionChannels.cs
- TreeChangeInfo.cs
- IndexedString.cs
- UIElementParagraph.cs
- DataExchangeServiceBinder.cs
- ComEventsHelper.cs
- MemoryStream.cs
- Visual3D.cs
- ScrollItemPattern.cs
- DateTimeParse.cs
- RecognitionResult.cs
- AccessibleObject.cs
- FocusTracker.cs
- HtmlInputText.cs
- BitmapEffectGeneralTransform.cs
- FixedBufferAttribute.cs
- Int32AnimationUsingKeyFrames.cs
- TemplatingOptionsDialog.cs
- ObjectAnimationBase.cs
- XmlAutoDetectWriter.cs
- SqlRowUpdatedEvent.cs
- OrderedDictionaryStateHelper.cs
- Stack.cs
- DataFieldEditor.cs
- SystemUdpStatistics.cs
- TableRow.cs
- XmlMemberMapping.cs
- CodeCastExpression.cs
- SpecialFolderEnumConverter.cs
- SerializationEventsCache.cs
- TextDecorationLocationValidation.cs
- OdbcRowUpdatingEvent.cs
- SpeechSynthesizer.cs
- COAUTHINFO.cs
- InfocardChannelParameter.cs
- SQLConvert.cs
- SerializationInfoEnumerator.cs
- Set.cs
- TextComposition.cs
- PriorityQueue.cs
- DbConnectionStringCommon.cs
- PropertyPath.cs
- InstanceContext.cs
- HostSecurityManager.cs
- RoleGroup.cs
- SqlSupersetValidator.cs
- ToolStrip.cs
- WebControlsSection.cs
- AssemblyBuilder.cs
- DbProviderFactory.cs
- XmlTextReader.cs
- EventLogPermissionEntry.cs
- NGCPageContentCollectionSerializerAsync.cs
- TableRowGroup.cs
- MethodBuilderInstantiation.cs
- messageonlyhwndwrapper.cs
- BooleanExpr.cs
- ObjectConverter.cs
- PaintValueEventArgs.cs
- OrthographicCamera.cs
- LostFocusEventManager.cs
- ConstructorNeedsTagAttribute.cs
- TypeDependencyAttribute.cs
- RecordBuilder.cs
- DataStorage.cs
- MultiAsyncResult.cs
- Propagator.JoinPropagator.cs