Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / ExpressionHelper.cs / 1305376 / ExpressionHelper.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Activities.Presentation.Model; using Microsoft.VisualBasic.Activities; using System.Reflection; using System.Globalization; using System.Activities.XamlIntegration; using System.Activities.Expressions; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Runtime; using System.Activities.Presentation.View; namespace System.Activities.Presentation { internal static class ExpressionHelper { internal static string GetExpressionString(Activity expression) { return ExpressionHelper.GetExpressionString(expression, null as ParserContext); } internal static string GetExpressionString(Activity expression, ModelItem owner) { ParserContext context = new ParserContext(owner); return ExpressionHelper.GetExpressionString(expression, context); } internal static string GetExpressionString(Activity expression, ParserContext context) { string expressionString = null; if (expression != null) { Type expressionType = expression.GetType(); Type expressionArgumentType = expressionType.IsGenericType ? expressionType.GetGenericArguments()[0] : typeof(object); bool isLiteral = expressionType.IsGenericType ? Type.Equals(typeof(Literal<>), expressionType.GetGenericTypeDefinition()) : false; //handle VB expression family if (expressionType.IsGenericType && (expressionType.GetGenericTypeDefinition() == typeof(VisualBasicValue<>) || expressionType.GetGenericTypeDefinition() == typeof(VisualBasicReference<>))) { expressionString = string.Empty; PropertyInfo propInfo = expressionType.GetProperty("ExpressionText"); expressionString = (string)propInfo.GetValue(expression, null); } //handle Literals Expression else if (isLiteral) { TypeConverter converter = XamlUtilities.GetConverter(expressionArgumentType); if (converter != null && converter.CanConvertTo(context, typeof(string))) { PropertyInfo literalValueProperty = expressionType.GetProperty("Value"); Fx.Assert(literalValueProperty != null && literalValueProperty.GetGetMethod() != null, "Literalmust have the Value property with a public get accessor."); object literalValue = literalValueProperty.GetValue(expression, null); string convertedString = null; if (literalValue != null) { try { convertedString = converter.ConvertToString(context, literalValue); } catch (ArgumentException) { convertedString = literalValue.ToString(); } } else { convertedString = "Nothing"; } expressionString = expressionArgumentType == typeof(string) ? ("\"" + convertedString + "\"") : convertedString; } } } return expressionString; } [SuppressMessage("Microsoft.Design", "CA1031:DoNotCatchGeneralExceptionTypes", Justification = "The conversion to an expression might fail due to invalid user input. Propagating exceptions might lead to VS crash.")] [SuppressMessage("Reliability", "Reliability108:IsFatalRule", Justification = "The conversion to an expression might fail due to invalid user input. Propagating exceptions might lead to VS crash.")] internal static ActivityWithResult TryCreateLiteral(Type type, string expressionText, ParserContext context) { //try easy way first - look if there is a type converer which supports convertsion between expression type and string TypeConverter literalValueConverter = null; bool isQuotedString = false; if (CanTypeBeSerializedAsLiteral(type)) { bool shouldBeQuoted = typeof(string) == type; //whether string begins and ends with quotes '"'. also, if there are //more quotes within than those begining and ending ones, do not bother with literal - assume this is an expression. isQuotedString = shouldBeQuoted && expressionText.StartsWith("\"", StringComparison.CurrentCulture) && expressionText.EndsWith("\"", StringComparison.CurrentCulture) && expressionText.IndexOf("\"", 1, StringComparison.CurrentCulture) == expressionText.Length - 1; //if expression is a string, we must ensure it is quoted, in case of other types - just get the converter if ((shouldBeQuoted && isQuotedString) || !shouldBeQuoted) { literalValueConverter = TypeDescriptor.GetConverter(type); } } //if there is converter - try to convert if (null != literalValueConverter && literalValueConverter.CanConvertFrom(context, typeof(string))) { try { var valueToConvert = isQuotedString ? expressionText.Substring(1, expressionText.Length - 2) : expressionText; var converterValue = literalValueConverter.ConvertFrom(context, CultureInfo.CurrentCulture, valueToConvert); //ok, succeeded - create literal of given type var concreteExpType = typeof(Literal<>).MakeGenericType(type); return (ActivityWithResult)Activator.CreateInstance(concreteExpType, converterValue); } //conversion failed - do nothing, let VB compiler take care of the expression catch { } } return null; } internal static bool CanTypeBeSerializedAsLiteral(Type type) { //type must be set and cannot be object if (null == type || typeof(object) == type) { return false; } return type.IsPrimitive || type == typeof(string) || type == typeof(TimeSpan) || type == typeof(DateTime); } internal static ActivityWithResult CreateExpression(Type type, string expressionText, bool isLocation, ParserContext context) { ActivityWithResult newExpression; if (!isLocation) { newExpression = TryCreateLiteral(type, expressionText, context); if (newExpression != null) { return newExpression; } } Type targetExpressionType = null; if (isLocation) { targetExpressionType = typeof(VisualBasicReference<>).MakeGenericType(type); } else { targetExpressionType = typeof(VisualBasicValue<>).MakeGenericType(type); } //create new visual basic value and pass expression text into it newExpression = (ActivityWithResult)Activator.CreateInstance(targetExpressionType, expressionText); //targetExpressionType.GetProperty("Settings").SetValue(newExpression, settings, null); //this code below is never executed - it is placed here only to enable compilation support whenver VisualBasicValue constructor //changes its parameter list. if (null == newExpression) { //if this gives compilation error, please update CreateInstance parameter list above as well! newExpression = new VisualBasicValue (expressionText); } return newExpression; } // Test whether this activity is a VB Exporession (either a VB Value or VB Reference). internal static bool IsVisualBasicExpression(this Activity activity) { Type activityType = activity.GetType(); return (activityType.IsGenericType && (activityType.GetGenericTypeDefinition() == typeof(VisualBasicValue<>) || activityType.GetGenericTypeDefinition() == typeof(VisualBasicReference<>))); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
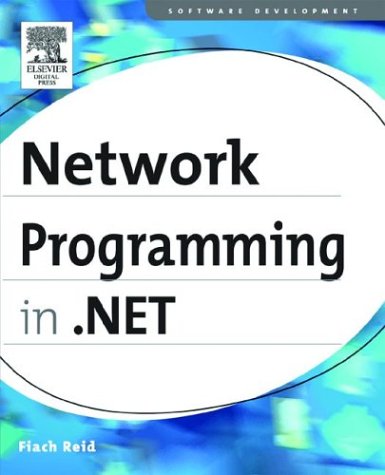
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CfgArc.cs
- ArgumentOutOfRangeException.cs
- OracleException.cs
- TextElement.cs
- Rfc4050KeyFormatter.cs
- ControlOperationInvoker.cs
- DocumentGridPage.cs
- DateTimeFormatInfoScanner.cs
- Lease.cs
- CertificateReferenceElement.cs
- MimeParameters.cs
- DigestTraceRecordHelper.cs
- Help.cs
- PatternMatcher.cs
- JournalEntryStack.cs
- Cursor.cs
- HitTestParameters3D.cs
- TemplateBindingExpressionConverter.cs
- ClientSponsor.cs
- HttpException.cs
- BamlTreeNode.cs
- PeerNameRecordCollection.cs
- FileCodeGroup.cs
- AdapterDictionary.cs
- BoundField.cs
- ResXDataNode.cs
- WindowPattern.cs
- TimeoutException.cs
- GreenMethods.cs
- ProjectedWrapper.cs
- ListDataHelper.cs
- FormatConvertedBitmap.cs
- PathParser.cs
- NullableConverter.cs
- ProviderSettings.cs
- SpellerHighlightLayer.cs
- PropertiesTab.cs
- TypeExtensionConverter.cs
- DbDataAdapter.cs
- IdleTimeoutMonitor.cs
- OleDbFactory.cs
- DataGridPageChangedEventArgs.cs
- ToolStripArrowRenderEventArgs.cs
- MasterPage.cs
- Brush.cs
- InfoCardRSAOAEPKeyExchangeDeformatter.cs
- PageThemeCodeDomTreeGenerator.cs
- VirtualPathData.cs
- FrameworkRichTextComposition.cs
- JsonWriter.cs
- TextEffect.cs
- Translator.cs
- FunctionImportMapping.cs
- AQNBuilder.cs
- SspiSafeHandles.cs
- HttpListenerRequest.cs
- MouseGestureValueSerializer.cs
- WebBrowserBase.cs
- SiteMapNode.cs
- ListParagraph.cs
- XmlSiteMapProvider.cs
- TreeNodeStyleCollectionEditor.cs
- VirtualPath.cs
- CreateUserErrorEventArgs.cs
- JpegBitmapEncoder.cs
- SignatureToken.cs
- GridItem.cs
- ActiveXHelper.cs
- VirtualPathUtility.cs
- XamlClipboardData.cs
- SourceSwitch.cs
- ProfilePropertySettingsCollection.cs
- ViewgenContext.cs
- MergablePropertyAttribute.cs
- DictionaryTraceRecord.cs
- XmlArrayItemAttribute.cs
- TemplateColumn.cs
- ToolStripContentPanelDesigner.cs
- SectionRecord.cs
- AppSettingsSection.cs
- ObjectListDataBindEventArgs.cs
- SelectionRange.cs
- RealProxy.cs
- SqlOuterApplyReducer.cs
- XmlSchemaObject.cs
- DrawingState.cs
- EmissiveMaterial.cs
- UpdateManifestForBrowserApplication.cs
- XsdCachingReader.cs
- BCLDebug.cs
- CookieHandler.cs
- Part.cs
- CharacterString.cs
- TagPrefixCollection.cs
- AppDomainEvidenceFactory.cs
- Substitution.cs
- SQLInt32Storage.cs
- DynamicMethod.cs
- safex509handles.cs
- FormattedTextSymbols.cs