Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / ReaderContextStackData.cs / 1305600 / ReaderContextStackData.cs
/****************************************************************************\ * * File: ReaderContextStackData.cs * * Copyright (C) 2003 by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System; using System.Collections; using System.Reflection; using System.Diagnostics; namespace System.Windows.Markup { // Data maintained on the reader's context stack. The root of the tree is at the bottom // of the stack. internal class ReaderContextStackData { // // NOTE: If you add a field here, be sure to update ClearData // ReaderFlags _contextFlags; object _contextData; object _contextKey; string _uid; string _name; object _contentProperty; Type _expectedType; short _expectedTypeId; bool _createUsingTypeConverter; // // NOTE: If you add a field here, be sure to update ClearData // // Returns just the part of the flags field corresponding to the context type internal ReaderFlags ContextType { get { return (ReaderFlags)(_contextFlags & ReaderFlags.ContextTypeMask); } } // The data object for this point in the stack. Typically the element at // this scoping level internal object ObjectData { get { return _contextData; } set { _contextData = value; } } // The key attribute defined for the current context, whose parent context is expected // to be an IDictionary internal object Key { get { return _contextKey; } set { _contextKey = value; } } // The x:Uid of this object, if it has one and has been read yet. internal string Uid { get { return _uid; } set { _uid = value; } } // The x:Name (or Name) of this object, if it has one and has been read yet. // Alternatively if this context object represents a property this member // gives you the name of the property. This is used to find a fallback value // for this property in the event of an exception during property parsing. internal string ElementNameOrPropertyName { get { return _name; } set { _name = value; } } internal object ContentProperty { get { return _contentProperty; } set { _contentProperty = value; } } // If an object has not yet been created at this point, this is the type of // element to created internal Type ExpectedType { get { return _expectedType; } set { _expectedType = value; } } // If an object has not yet been created at this point, this is the Baml type id // of the element. This is used for faster creation of known types. internal short ExpectedTypeId { get { return _expectedTypeId; } set { _expectedTypeId = value; } } // This object is expected to be created using a TypeConverter. If this // is true, the following are also expected to be true: // -ObjectData is null // -ExpectedType is non-null // -ExpectedTypeId is non-null internal bool CreateUsingTypeConverter { get { return _createUsingTypeConverter; } set { _createUsingTypeConverter = value; } } // Context identifying what this reader stack item represents internal ReaderFlags ContextFlags { get { return _contextFlags; } set { _contextFlags = value; } } // True if this element has not yet been added to the tree, but needs to be. internal bool NeedToAddToTree { get { return CheckFlag(ReaderFlags.NeedToAddToTree); } } // simple helper method to remove the NeedToAddToTree flag and add the AddedToTree flag internal void MarkAddedToTree() { ContextFlags = ((ContextFlags | ReaderFlags.AddedToTree) & ~ReaderFlags.NeedToAddToTree); } // simple helper method that returns true if the context contains the given flag or flags. // If multiple flags are passed in, the context must contain all the flags. internal bool CheckFlag(ReaderFlags flag) { return (ContextFlags & flag) == flag; } // simple helper method adds the flag to the context internal void SetFlag(ReaderFlags flag) { ContextFlags |= flag; } // simple helper method that removes the flag from the context internal void ClearFlag(ReaderFlags flag) { ContextFlags &= ~flag; } // Helper to determine if this represents an object element. internal bool IsObjectElement { get { return ContextType == ReaderFlags.DependencyObject || ContextType == ReaderFlags.ClrObject; } } // Clear all the fields on this instance before it put into the factory cache internal void ClearData() { _contextFlags = 0; _contextData = null; _contextKey = null; _contentProperty = null; _expectedType = null; _expectedTypeId = 0; _createUsingTypeConverter = false; _uid = null; _name = null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
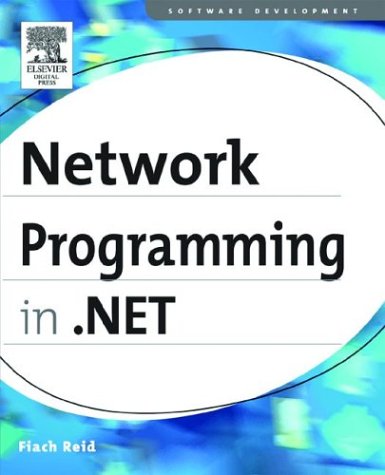
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Control.cs
- FileResponseElement.cs
- MenuTracker.cs
- _LoggingObject.cs
- DesignerOptionService.cs
- TaskForm.cs
- PropertyDescriptorComparer.cs
- FunctionNode.cs
- PassportAuthentication.cs
- ChannelReliableSession.cs
- BevelBitmapEffect.cs
- DelegatingStream.cs
- BackStopAuthenticationModule.cs
- Empty.cs
- StateMachineHistory.cs
- SqlBooleanizer.cs
- CodeDirectoryCompiler.cs
- ConfigurationCollectionAttribute.cs
- SiteOfOriginContainer.cs
- WindowsToolbar.cs
- WorkflowQueuingService.cs
- FileCodeGroup.cs
- SpnegoTokenAuthenticator.cs
- CalendarDay.cs
- validation.cs
- KeyInterop.cs
- SafeHandle.cs
- StylusPointPropertyInfoDefaults.cs
- PowerModeChangedEventArgs.cs
- ConsoleCancelEventArgs.cs
- StateDesignerConnector.cs
- mediaeventargs.cs
- DbDataRecord.cs
- COM2AboutBoxPropertyDescriptor.cs
- ValueConversionAttribute.cs
- SizeKeyFrameCollection.cs
- GeneralTransform.cs
- ValueOfAction.cs
- UInt16Storage.cs
- DisplayNameAttribute.cs
- DbTransaction.cs
- XmlNavigatorFilter.cs
- SettingsContext.cs
- Pen.cs
- RealizedColumnsBlock.cs
- Classification.cs
- TypeSemantics.cs
- ReadContentAsBinaryHelper.cs
- Empty.cs
- MenuItemCollection.cs
- Base64Decoder.cs
- PathSegmentCollection.cs
- ExpressionLexer.cs
- DeclarativeCatalogPart.cs
- ConstraintStruct.cs
- StateWorkerRequest.cs
- QueueAccessMode.cs
- HttpWebRequestElement.cs
- DataGridViewCellStyle.cs
- VideoDrawing.cs
- RijndaelManaged.cs
- ObjectStateFormatter.cs
- TypeReference.cs
- DescendantQuery.cs
- RegexRunnerFactory.cs
- GridViewRowPresenterBase.cs
- Int16.cs
- DataTableMappingCollection.cs
- SafeArrayTypeMismatchException.cs
- SrgsRulesCollection.cs
- AttributeSetAction.cs
- FieldNameLookup.cs
- HwndHost.cs
- Parser.cs
- ListBase.cs
- SpanIndex.cs
- ArrangedElement.cs
- HyperlinkAutomationPeer.cs
- coordinatorscratchpad.cs
- DataSourceExpressionCollection.cs
- ValueSerializer.cs
- BitVector32.cs
- CodeSubDirectory.cs
- ReadOnlyAttribute.cs
- WebFormsRootDesigner.cs
- SpanIndex.cs
- ApplicationSettingsBase.cs
- SemanticResultKey.cs
- controlskin.cs
- SecurityTokenTypes.cs
- Win32Native.cs
- SettingsAttributeDictionary.cs
- FolderBrowserDialog.cs
- XmlSerializableReader.cs
- AtomMaterializerLog.cs
- MulticastOption.cs
- Debug.cs
- LowerCaseStringConverter.cs
- GenericPrincipal.cs
- WsdlInspector.cs