Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / TransactionBridge / Microsoft / Transactions / Bridge / StateMachineHistory.cs / 1 / StateMachineHistory.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; namespace Microsoft.Transactions.Bridge { struct StringCount { public StringCount (string name) { this.Name = name; this.Count = 1; } public string Name; public int Count; static StringCount nullCount = new StringCount (null); public static StringCount Null { get { return nullCount; } } } // // This class is implicitly single-threaded // class StateMachineHistory : IXmlSerializable { // The list contains a sequence of strings // States are preceded by a null string, events follow a state Listhistory = new List (30); string ReadState (ref int cursor) { int entries = this.history.Count; if (cursor >= entries) return null; // If this slot has a name, it's not a state if (this.history[cursor].Name != null) return null; // We saw a null, so there must be a state name too cursor ++; if (cursor >= entries) { // The cursor should never advance pass the end of the list. DiagnosticUtility.DebugAssert("The state machine history store is corrupt."); } return this.history[cursor ++].Name; } StringCount ReadEvent (ref int cursor) { if (cursor >= this.history.Count) return StringCount.Null; // If this slot doesn't have a name, it's not an event if (this.history[cursor].Name == null) return StringCount.Null; return this.history[cursor ++]; } // // Public interface // public void AddState (string newState) { this.history.Add (new StringCount (null)); this.history.Add (new StringCount (newState)); } // An event should always arrive in the context of a state // It is an error to call the first AddEvent without having called AddStateChange public void AddEvent (string newEvent) { int count = this.history.Count; if (count > 0) { StringCount lastEvent = this.history[count - 1]; if (lastEvent.Name == newEvent) { lastEvent.Count ++; this.history[count - 1] = lastEvent; return; } } this.history.Add (new StringCount (newEvent)); } /* Example: [State1] {Event1} {Event2} [State2] {Event3} [State3] */ public override string ToString() { int entries = this.history.Count; if (entries == 0) { return string.Empty; } StringBuilder builder = new StringBuilder(); int cursor = 0; while (cursor < entries) { // Read a state string state = ReadState (ref cursor); if (state == null) { // The state should never be null. DiagnosticUtility.DebugAssert("The state machine history store is corrupt."); } builder.Append ("["); builder.Append (state); builder.AppendLine ("]"); // Read the events that occurred on the state while (true) { StringCount newEvent = ReadEvent (ref cursor); if (newEvent.Name == null) break; for (int i = 0; i < newEvent.Count; i ++) { builder.Append ("\t{"); builder.Append (newEvent.Name); builder.AppendLine ("}"); } } } // We should have consumed all the entries if (cursor != entries) { DiagnosticUtility.DebugAssert("The state machine history store is corrupt."); } return builder.ToString(); } // // IXmlSerializable // XmlSchema IXmlSerializable.GetSchema() { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } void IXmlSerializable.ReadXml (XmlReader reader) { throw Microsoft.Transactions.Bridge.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new NotSupportedException()); } /* Example: */ void IXmlSerializable.WriteXml (XmlWriter writer) { // Open the envelope writer.WriteStartElement ("StateMachineHistory"); int cursor = 0, entries = this.history.Count; while (cursor < entries) { // Read a state string state = ReadState (ref cursor); if (state == null) { // The state should never be null. DiagnosticUtility.DebugAssert("The state machine history store is corrupt."); } writer.WriteStartElement ("state"); writer.WriteAttributeString ("name", state); // Read the events that occurred on the state while (true) { StringCount newEvent = ReadEvent (ref cursor); if (newEvent.Name == null) break; for (int i = 0; i < newEvent.Count; i ++) { writer.WriteStartElement ("event"); writer.WriteAttributeString ("name", newEvent.Name); writer.WriteEndElement(); } } // Close the state element writer.WriteEndElement(); } // Close the envelope writer.WriteEndElement(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
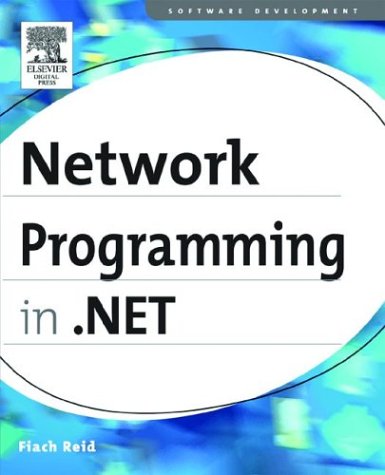
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProcessManager.cs
- ToolBarOverflowPanel.cs
- CuspData.cs
- ELinqQueryState.cs
- PeerNameRecordCollection.cs
- StringFormat.cs
- DataColumn.cs
- PropertyDescriptorCollection.cs
- RadioButtonAutomationPeer.cs
- SingleAnimationUsingKeyFrames.cs
- DefaultShape.cs
- FixedPage.cs
- EpmContentSerializer.cs
- ReferencedAssemblyResolver.cs
- RulePatternOps.cs
- GatewayDefinition.cs
- XmlSchemaProviderAttribute.cs
- WebHttpBindingElement.cs
- ErrorRuntimeConfig.cs
- GridViewSortEventArgs.cs
- Delegate.cs
- Stackframe.cs
- HwndStylusInputProvider.cs
- BatchStream.cs
- MiniConstructorInfo.cs
- WebContext.cs
- ChtmlCommandAdapter.cs
- UserControlDesigner.cs
- MD5.cs
- _LazyAsyncResult.cs
- BasicExpandProvider.cs
- TrackingProfile.cs
- BStrWrapper.cs
- DataTableMapping.cs
- SrgsDocument.cs
- UserInitiatedRoutedEventPermissionAttribute.cs
- AsyncOperationManager.cs
- TabControlEvent.cs
- ServiceReference.cs
- CompareValidator.cs
- SystemIPv6InterfaceProperties.cs
- uribuilder.cs
- PeerCustomResolverSettings.cs
- HostingEnvironment.cs
- _CookieModule.cs
- ProvidersHelper.cs
- CreateUserWizardStep.cs
- SqlDataSourceConfigureFilterForm.cs
- MdbDataFileEditor.cs
- DoWorkEventArgs.cs
- ReversePositionQuery.cs
- BidOverLoads.cs
- DataSet.cs
- IProvider.cs
- _StreamFramer.cs
- BindingCollection.cs
- PropertyInformationCollection.cs
- DataGridColumnEventArgs.cs
- ListViewCommandEventArgs.cs
- UmAlQuraCalendar.cs
- MulticastIPAddressInformationCollection.cs
- HTMLTextWriter.cs
- TableDetailsRow.cs
- CodeRemoveEventStatement.cs
- CryptographicAttribute.cs
- ApplicationBuildProvider.cs
- InheritanceAttribute.cs
- KeyManager.cs
- XmlValidatingReader.cs
- PackageStore.cs
- DetailsViewInsertEventArgs.cs
- SkewTransform.cs
- ValuePatternIdentifiers.cs
- IOThreadTimer.cs
- RelatedEnd.cs
- DataGridRow.cs
- BitmapVisualManager.cs
- XDeferredAxisSource.cs
- AssemblySettingAttributes.cs
- NodeInfo.cs
- ExceptionWrapper.cs
- Panel.cs
- XmlJsonWriter.cs
- SkinBuilder.cs
- IsolatedStorageFile.cs
- StylusDownEventArgs.cs
- TTSEvent.cs
- TextMarkerSource.cs
- DefaultProxySection.cs
- RootProfilePropertySettingsCollection.cs
- Operators.cs
- DelegateArgument.cs
- ProfileModule.cs
- OdbcErrorCollection.cs
- BaseCodeDomTreeGenerator.cs
- DependentTransaction.cs
- LowerCaseStringConverter.cs
- ChangePassword.cs
- WebPartsPersonalization.cs
- HttpDebugHandler.cs