Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / SSDLGenerator / TableDetailsRow.cs / 1 / TableDetailsRow.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Entity.Design.Common; using System.Globalization; using System.Data; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed DataTable for TableDetails /// internal sealed class TableDetailsRow : System.Data.DataRow { private TableDetailsCollection _tableTableDetails; [System.Diagnostics.DebuggerNonUserCodeAttribute()] internal TableDetailsRow(System.Data.DataRowBuilder rb) : base(rb) { this._tableTableDetails = ((TableDetailsCollection)(base.Table)); } ////// Gets a strongly typed table /// public new TableDetailsCollection Table { get { return _tableTableDetails; } } ////// Gets the Catalog column value /// public string Catalog { get { try { return ((string)(this[this._tableTableDetails.CatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.CatalogColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.CatalogColumn] = value; } } ////// Gets the Schema column value /// public string Schema { get { try { return ((string)(this[this._tableTableDetails.SchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.SchemaColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.SchemaColumn] = value; } } ////// Gets the TableName column value /// public string TableName { get { try { return ((string)(this[this._tableTableDetails.TableNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.TableNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.TableNameColumn] = value; } } ////// Gets the ColumnName column value /// public string ColumnName { get { try { return ((string)(this[this._tableTableDetails.ColumnNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ColumnNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ColumnNameColumn] = value; } } ////// Gets the IsNullable column value /// public bool IsNullable { get { try { return ((bool)(this[this._tableTableDetails.IsNullableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsNullableColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsNullableColumn] = value; } } ////// Gets the DataType column value /// public string DataType { get { try { return ((string)(this[this._tableTableDetails.DataTypeColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DataTypeColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DataTypeColumn] = value; } } ////// Gets the MaximumLength column value /// public int MaximumLength { get { try { return ((int)(this[this._tableTableDetails.MaximumLengthColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.MaximumLengthColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.MaximumLengthColumn] = value; } } ////// Gets the DateTime Precision column value /// public int DateTimePrecision { get { try { return ((int)(this[this._tableTableDetails.DateTimePrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DateTimePrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DateTimePrecisionColumn] = value; } } ////// Gets the Precision column value /// public int Precision { get { try { return ((int)(this[this._tableTableDetails.PrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.PrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.PrecisionColumn] = value; } } ////// Gets the Scale column value /// public int Scale { get { try { return ((int)(this[this._tableTableDetails.ScaleColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ScaleColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ScaleColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsIdentity { get { try { return ((bool)(this[this._tableTableDetails.IsIdentityColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsIdentityColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsIdentityColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsServerGenerated { get { try { return ((bool)(this[this._tableTableDetails.IsServerGeneratedColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsServerGeneratedColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsServerGeneratedColumn] = value; } } ////// Gets the IsPrimaryKey column value /// public bool IsPrimaryKey { get { try { return ((bool)(this[this._tableTableDetails.IsPrimaryKeyColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsPrimaryKeyColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsPrimaryKeyColumn] = value; } } ////// Determines if the Catalog column value is null /// ///true if the value is null, otherwise false. public bool IsCatalogNull() { return this.IsNull(this._tableTableDetails.CatalogColumn); } ////// Determines if the Schema column value is null /// ///true if the value is null, otherwise false. public bool IsSchemaNull() { return this.IsNull(this._tableTableDetails.SchemaColumn); } ////// Determines if the DataType column value is null /// ///true if the value is null, otherwise false. public bool IsDataTypeNull() { return this.IsNull(this._tableTableDetails.DataTypeColumn); } ////// Determines if the MaximumLength column value is null /// ///true if the value is null, otherwise false. public bool IsMaximumLengthNull() { return this.IsNull(this._tableTableDetails.MaximumLengthColumn); } ////// Determines if the Precision column value is null /// ///true if the value is null, otherwise false. public bool IsPrecisionNull() { return this.IsNull(this._tableTableDetails.PrecisionColumn); } ////// Determines if the DateTime Precision column value is null /// ///true if the value is null, otherwise false. public bool IsDateTimePrecisionNull() { return this.IsNull(this._tableTableDetails.DateTimePrecisionColumn); } ////// Determines if the Scale column value is null /// ///true if the value is null, otherwise false. public bool IsScaleNull() { return this.IsNull(this._tableTableDetails.ScaleColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsIdentityNull() { return this.IsNull(this._tableTableDetails.IsIdentityColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsServerGeneratedNull() { return this.IsNull(this._tableTableDetails.IsServerGeneratedColumn); } public string GetMostQualifiedTableName() { string name = string.Empty; if (!IsCatalogNull()) { name = Catalog; } if (!IsSchemaNull()) { if (name != string.Empty) { name += "."; } name += Schema; } if (name != string.Empty) { name += "."; } // TableName is not allowed to be null name += TableName; return name; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Xml; using System.Data.Entity.Design.Common; using System.Globalization; using System.Data; namespace System.Data.Entity.Design.SsdlGenerator { ////// Strongly typed DataTable for TableDetails /// internal sealed class TableDetailsRow : System.Data.DataRow { private TableDetailsCollection _tableTableDetails; [System.Diagnostics.DebuggerNonUserCodeAttribute()] internal TableDetailsRow(System.Data.DataRowBuilder rb) : base(rb) { this._tableTableDetails = ((TableDetailsCollection)(base.Table)); } ////// Gets a strongly typed table /// public new TableDetailsCollection Table { get { return _tableTableDetails; } } ////// Gets the Catalog column value /// public string Catalog { get { try { return ((string)(this[this._tableTableDetails.CatalogColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.CatalogColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.CatalogColumn] = value; } } ////// Gets the Schema column value /// public string Schema { get { try { return ((string)(this[this._tableTableDetails.SchemaColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.SchemaColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.SchemaColumn] = value; } } ////// Gets the TableName column value /// public string TableName { get { try { return ((string)(this[this._tableTableDetails.TableNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.TableNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.TableNameColumn] = value; } } ////// Gets the ColumnName column value /// public string ColumnName { get { try { return ((string)(this[this._tableTableDetails.ColumnNameColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ColumnNameColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ColumnNameColumn] = value; } } ////// Gets the IsNullable column value /// public bool IsNullable { get { try { return ((bool)(this[this._tableTableDetails.IsNullableColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsNullableColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsNullableColumn] = value; } } ////// Gets the DataType column value /// public string DataType { get { try { return ((string)(this[this._tableTableDetails.DataTypeColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DataTypeColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DataTypeColumn] = value; } } ////// Gets the MaximumLength column value /// public int MaximumLength { get { try { return ((int)(this[this._tableTableDetails.MaximumLengthColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.MaximumLengthColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.MaximumLengthColumn] = value; } } ////// Gets the DateTime Precision column value /// public int DateTimePrecision { get { try { return ((int)(this[this._tableTableDetails.DateTimePrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.DateTimePrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.DateTimePrecisionColumn] = value; } } ////// Gets the Precision column value /// public int Precision { get { try { return ((int)(this[this._tableTableDetails.PrecisionColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.PrecisionColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.PrecisionColumn] = value; } } ////// Gets the Scale column value /// public int Scale { get { try { return ((int)(this[this._tableTableDetails.ScaleColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.ScaleColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.ScaleColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsIdentity { get { try { return ((bool)(this[this._tableTableDetails.IsIdentityColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsIdentityColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsIdentityColumn] = value; } } ////// Gets the IsServerGenerated column value /// public bool IsServerGenerated { get { try { return ((bool)(this[this._tableTableDetails.IsServerGeneratedColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsServerGeneratedColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsServerGeneratedColumn] = value; } } ////// Gets the IsPrimaryKey column value /// public bool IsPrimaryKey { get { try { return ((bool)(this[this._tableTableDetails.IsPrimaryKeyColumn])); } catch (System.InvalidCastException e) { throw EDesignUtil.StonglyTypedAccessToNullValue(_tableTableDetails.IsPrimaryKeyColumn.ColumnName, _tableTableDetails.TableName, e); } } set { this[this._tableTableDetails.IsPrimaryKeyColumn] = value; } } ////// Determines if the Catalog column value is null /// ///true if the value is null, otherwise false. public bool IsCatalogNull() { return this.IsNull(this._tableTableDetails.CatalogColumn); } ////// Determines if the Schema column value is null /// ///true if the value is null, otherwise false. public bool IsSchemaNull() { return this.IsNull(this._tableTableDetails.SchemaColumn); } ////// Determines if the DataType column value is null /// ///true if the value is null, otherwise false. public bool IsDataTypeNull() { return this.IsNull(this._tableTableDetails.DataTypeColumn); } ////// Determines if the MaximumLength column value is null /// ///true if the value is null, otherwise false. public bool IsMaximumLengthNull() { return this.IsNull(this._tableTableDetails.MaximumLengthColumn); } ////// Determines if the Precision column value is null /// ///true if the value is null, otherwise false. public bool IsPrecisionNull() { return this.IsNull(this._tableTableDetails.PrecisionColumn); } ////// Determines if the DateTime Precision column value is null /// ///true if the value is null, otherwise false. public bool IsDateTimePrecisionNull() { return this.IsNull(this._tableTableDetails.DateTimePrecisionColumn); } ////// Determines if the Scale column value is null /// ///true if the value is null, otherwise false. public bool IsScaleNull() { return this.IsNull(this._tableTableDetails.ScaleColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsIdentityNull() { return this.IsNull(this._tableTableDetails.IsIdentityColumn); } ////// Determines if the IsIdentity column value is null /// ///true if the value is null, otherwise false. public bool IsIsServerGeneratedNull() { return this.IsNull(this._tableTableDetails.IsServerGeneratedColumn); } public string GetMostQualifiedTableName() { string name = string.Empty; if (!IsCatalogNull()) { name = Catalog; } if (!IsSchemaNull()) { if (name != string.Empty) { name += "."; } name += Schema; } if (name != string.Empty) { name += "."; } // TableName is not allowed to be null name += TableName; return name; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
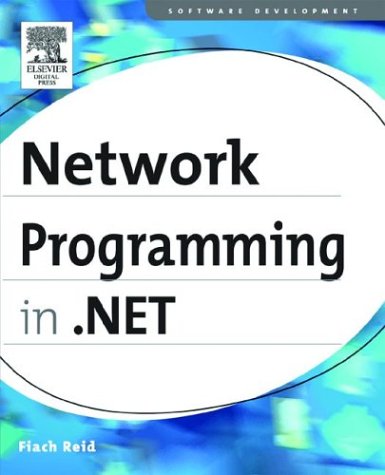
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MetadataArtifactLoader.cs
- DataServiceProviderMethods.cs
- CookieProtection.cs
- StyleTypedPropertyAttribute.cs
- DbExpressionRules.cs
- FormCollection.cs
- CompleteWizardStep.cs
- CompareValidator.cs
- Registration.cs
- CqlGenerator.cs
- StorageSetMapping.cs
- ToolStripDropDownClosedEventArgs.cs
- WindowCollection.cs
- ProgressiveCrcCalculatingStream.cs
- NestedContainer.cs
- LabelLiteral.cs
- TableMethodGenerator.cs
- X509ThumbprintKeyIdentifierClause.cs
- ADRole.cs
- ComNativeDescriptor.cs
- XmlSchemaSimpleType.cs
- HtmlTableRow.cs
- CancellationScope.cs
- EntityDataSourceViewSchema.cs
- HttpContext.cs
- Vector3DIndependentAnimationStorage.cs
- Screen.cs
- invalidudtexception.cs
- MLangCodePageEncoding.cs
- ReadWriteObjectLock.cs
- BackEase.cs
- CloseSequenceResponse.cs
- RuntimeTransactionHandle.cs
- OleServicesContext.cs
- UseLicense.cs
- TypedRowHandler.cs
- IndentedWriter.cs
- ExpandedWrapper.cs
- ProtocolsConfiguration.cs
- NetSectionGroup.cs
- KeyTime.cs
- Zone.cs
- AsyncCompletedEventArgs.cs
- EventEntry.cs
- Keyboard.cs
- SatelliteContractVersionAttribute.cs
- Control.cs
- XMLSchema.cs
- Rect.cs
- FileNotFoundException.cs
- DesignerForm.cs
- XmlSchemaImport.cs
- RectangleHotSpot.cs
- Task.cs
- ConnectionInterfaceCollection.cs
- StrongNamePublicKeyBlob.cs
- ClientConfigPaths.cs
- StopRoutingHandler.cs
- TrackingExtract.cs
- COM2TypeInfoProcessor.cs
- EditorZone.cs
- RTLAwareMessageBox.cs
- Geometry.cs
- WebPartExportVerb.cs
- StylusShape.cs
- TemplateBindingExtensionConverter.cs
- TickBar.cs
- ShaderRenderModeValidation.cs
- DataGridViewRowsAddedEventArgs.cs
- SiteMapNodeCollection.cs
- BitmapEffectGeneralTransform.cs
- GeometryCombineModeValidation.cs
- Dynamic.cs
- MetadataSerializer.cs
- MaskedTextProvider.cs
- ListSourceHelper.cs
- ListControlDesigner.cs
- XmlSignatureProperties.cs
- SymLanguageType.cs
- SymLanguageVendor.cs
- ByteArrayHelperWithString.cs
- shaperfactoryquerycachekey.cs
- RootBuilder.cs
- StoreItemCollection.cs
- safemediahandle.cs
- InstanceHandleConflictException.cs
- TransportChannelListener.cs
- XmlSchemaType.cs
- PropertyGrid.cs
- EventManager.cs
- Substitution.cs
- UnsignedPublishLicense.cs
- ActivityDesigner.cs
- SecurityManager.cs
- WebException.cs
- UnsafeNativeMethods.cs
- IProvider.cs
- base64Transforms.cs
- WebPartCatalogCloseVerb.cs
- ContentValidator.cs