Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Internal / ExpandedWrapper.cs / 1407647 / ExpandedWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a base class implementing IExpandedResult over // projections. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Internal { using System; ///Provides a base class implementing IExpandedResult over projections. ///Type of element whose properties are expanded. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public abstract class ExpandedWrapper: IExpandedResult { #region Fields. /// Text description of properties, in comma-separated format. private string description; ///Element whose properties are being expanded. private TExpandedElement expandedElement; ///Parsed property names. private string[] propertyNames; #endregion Fields. #region Public properties. ///Text description of properties, in comma-separated format. public string Description { get { return this.description; } set { this.description = WebUtil.CheckArgumentNull(value, "value"); this.propertyNames = WebUtil.StringToSimpleArray(this.description); int expectedLength = this.GetType().GetGenericArguments().Length - 1; if (this.propertyNames.Length != expectedLength) { throw new InvalidOperationException( Strings.BasicExpandProvider_DescriptionInitializerCountMismatch(value, expectedLength)); } } } ///Element whose properties are being expanded. public TExpandedElement ExpandedElement { get { return this.expandedElement; } set { this.expandedElement = value; } } ///The element with expanded properties. object IExpandedResult.ExpandedElement { get { return ProjectedWrapper.ProcessResultInstance(this.ExpandedElement); } } #endregion Public properties. #region Methods. ///Gets the value for named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. ////// If the element returned in turn has properties which are expanded out-of-band /// of the object model, then the result will also be of type public object GetExpandedPropertyValue(string name) { if (name == null) { throw new ArgumentNullException("name"); } if (this.propertyNames == null) { throw new InvalidOperationException(Strings.BasicExpandProvider_ExpandedPropertiesNotInitialized); } int nameIndex = -1; for (int i = 0; i < this.propertyNames.Length; i++) { if (this.propertyNames[i] == name) { nameIndex = i; break; } } if (nameIndex == -1 && name == XmlConstants.HttpQueryStringSkipToken) { return null; } object resource = this.InternalGetExpandedPropertyValue(nameIndex); resource = ProjectedWrapper.ProcessResultInstance(resource); return ProjectedWrapper.ProcessResultEnumeration(resource); } ///, /// and the value will be available through . /// A special case is the handling of $skiptoken property. In case the $skiptoken property does not /// exist on the current wrapper object, instead of throw-ing we return null which will /// be an indication to the caller that the property does not exist. /// Gets the value for the property for the result. /// Index of property for which to get enumerable results. ///The value for the property of the result. protected abstract object InternalGetExpandedPropertyValue(int nameIndex); #endregion Methods. } #region ExpandedWrapper types with numbered properties. ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets or sets a projected property. public TProperty6 ProjectedProperty6 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; if (nameIndex == 6) return this.ProjectedProperty6; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets or sets a projected property. public TProperty6 ProjectedProperty6 { get; set; } ///Gets or sets a projected property. public TProperty7 ProjectedProperty7 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; if (nameIndex == 6) return this.ProjectedProperty6; if (nameIndex == 7) return this.ProjectedProperty7; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets or sets a projected property. public TProperty6 ProjectedProperty6 { get; set; } ///Gets or sets a projected property. public TProperty7 ProjectedProperty7 { get; set; } ///Gets or sets a projected property. public TProperty8 ProjectedProperty8 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; if (nameIndex == 6) return this.ProjectedProperty6; if (nameIndex == 7) return this.ProjectedProperty7; if (nameIndex == 8) return this.ProjectedProperty8; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets or sets a projected property. public TProperty6 ProjectedProperty6 { get; set; } ///Gets or sets a projected property. public TProperty7 ProjectedProperty7 { get; set; } ///Gets or sets a projected property. public TProperty8 ProjectedProperty8 { get; set; } ///Gets or sets a projected property. public TProperty9 ProjectedProperty9 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; if (nameIndex == 6) return this.ProjectedProperty6; if (nameIndex == 7) return this.ProjectedProperty7; if (nameIndex == 8) return this.ProjectedProperty8; if (nameIndex == 9) return this.ProjectedProperty9; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets or sets a projected property. public TProperty6 ProjectedProperty6 { get; set; } ///Gets or sets a projected property. public TProperty7 ProjectedProperty7 { get; set; } ///Gets or sets a projected property. public TProperty8 ProjectedProperty8 { get; set; } ///Gets or sets a projected property. public TProperty9 ProjectedProperty9 { get; set; } ///Gets or sets a projected property. public TProperty10 ProjectedProperty10 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; if (nameIndex == 6) return this.ProjectedProperty6; if (nameIndex == 7) return this.ProjectedProperty7; if (nameIndex == 8) return this.ProjectedProperty8; if (nameIndex == 9) return this.ProjectedProperty9; if (nameIndex == 10) return this.ProjectedProperty10; throw Error.NotSupported(); } } ///Provides a wrapper over an element expanded with projections. ///Type of expanded element. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///Type of projected property. ///This class supports the WCF Data Services infrastructure and is not meant to be used directly from your code. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.MSInternal", "CA903", Justification = "Type is already under System namespace.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1005", Justification = "More than two parameter types used for wide projections.")] [System.ComponentModel.EditorBrowsable(System.ComponentModel.EditorBrowsableState.Never)] public sealed class ExpandedWrapper: ExpandedWrapper { /// Gets or sets a projected property. public TProperty0 ProjectedProperty0 { get; set; } ///Gets or sets a projected property. public TProperty1 ProjectedProperty1 { get; set; } ///Gets or sets a projected property. public TProperty2 ProjectedProperty2 { get; set; } ///Gets or sets a projected property. public TProperty3 ProjectedProperty3 { get; set; } ///Gets or sets a projected property. public TProperty4 ProjectedProperty4 { get; set; } ///Gets or sets a projected property. public TProperty5 ProjectedProperty5 { get; set; } ///Gets or sets a projected property. public TProperty6 ProjectedProperty6 { get; set; } ///Gets or sets a projected property. public TProperty7 ProjectedProperty7 { get; set; } ///Gets or sets a projected property. public TProperty8 ProjectedProperty8 { get; set; } ///Gets or sets a projected property. public TProperty9 ProjectedProperty9 { get; set; } ///Gets or sets a projected property. public TProperty10 ProjectedProperty10 { get; set; } ///Gets or sets a projected property. public TProperty11 ProjectedProperty11 { get; set; } ///Gets the value for the named property for the result. /// Name of property for which to get enumerable results. ///The value for the named property of the result. protected override object InternalGetExpandedPropertyValue(int nameIndex) { if (nameIndex == 0) return this.ProjectedProperty0; if (nameIndex == 1) return this.ProjectedProperty1; if (nameIndex == 2) return this.ProjectedProperty2; if (nameIndex == 3) return this.ProjectedProperty3; if (nameIndex == 4) return this.ProjectedProperty4; if (nameIndex == 5) return this.ProjectedProperty5; if (nameIndex == 6) return this.ProjectedProperty6; if (nameIndex == 7) return this.ProjectedProperty7; if (nameIndex == 8) return this.ProjectedProperty8; if (nameIndex == 9) return this.ProjectedProperty9; if (nameIndex == 10) return this.ProjectedProperty10; if (nameIndex == 11) return this.ProjectedProperty11; throw Error.NotSupported(); } } #endregion ExpandedWrapper types with numbered properties. } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
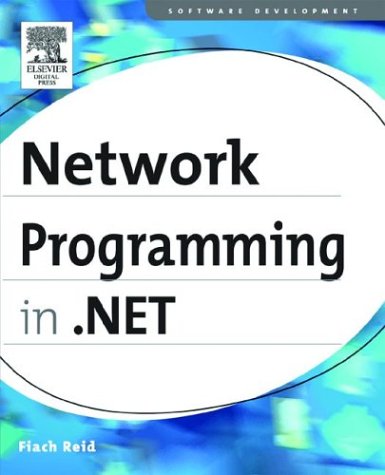
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextElementEditingBehaviorAttribute.cs
- DirectoryObjectSecurity.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- FrameworkElement.cs
- DependencySource.cs
- DataViewListener.cs
- CodeCompileUnit.cs
- DynamicResourceExtensionConverter.cs
- RightsDocument.cs
- FontStyle.cs
- MetadataUtil.cs
- DetailsViewInsertedEventArgs.cs
- TextFindEngine.cs
- MarkupProperty.cs
- Input.cs
- Rotation3DAnimation.cs
- MouseDevice.cs
- AccessKeyManager.cs
- RootAction.cs
- Action.cs
- EventHandlersStore.cs
- WebDisplayNameAttribute.cs
- UInt16Storage.cs
- ScriptingSectionGroup.cs
- RequestQueryProcessor.cs
- CommandConverter.cs
- HttpCachePolicyElement.cs
- RuleSetDialog.Designer.cs
- WindowsGraphics.cs
- SimpleHandlerFactory.cs
- BamlTreeNode.cs
- TypeSemantics.cs
- DataListItemEventArgs.cs
- TrackBarDesigner.cs
- CompositeFontParser.cs
- AssociativeAggregationOperator.cs
- ImageDrawing.cs
- CryptoStream.cs
- VideoDrawing.cs
- PropertyPanel.cs
- ToolBar.cs
- StylusEditingBehavior.cs
- InvalidWMPVersionException.cs
- NamespaceList.cs
- SetterBase.cs
- PostBackTrigger.cs
- OleDbStruct.cs
- GraphicsPathIterator.cs
- MenuItemStyleCollection.cs
- MimeReturn.cs
- SiteMapNodeItemEventArgs.cs
- ButtonBaseAdapter.cs
- EntityTypeBase.cs
- DefaultCommandExtensionCallback.cs
- Int32Converter.cs
- BamlLocalizationDictionary.cs
- CollectionViewGroupRoot.cs
- ViewEvent.cs
- XPathChildIterator.cs
- WindowHelperService.cs
- GeometryHitTestParameters.cs
- QueueNameHelper.cs
- SqlTrackingQuery.cs
- GlobalEventManager.cs
- LowerCaseStringConverter.cs
- StickyNoteContentControl.cs
- ParameterEditorUserControl.cs
- RightsManagementProvider.cs
- IBuiltInEvidence.cs
- PermissionSetTriple.cs
- TreeNodeCollection.cs
- DynamicHyperLink.cs
- DataControlImageButton.cs
- RightsManagementEncryptedStream.cs
- EdmRelationshipRoleAttribute.cs
- ToolStripDesignerAvailabilityAttribute.cs
- PolicyException.cs
- OrderByLifter.cs
- DataGrid.cs
- DataGridRowAutomationPeer.cs
- FunctionImportMapping.ReturnTypeRenameMapping.cs
- ActiveXHelper.cs
- parserscommon.cs
- PolyQuadraticBezierSegment.cs
- HttpApplication.cs
- _BufferOffsetSize.cs
- ActivityDesigner.cs
- SqlDataSourceQueryEditor.cs
- ByteKeyFrameCollection.cs
- ListControlConvertEventArgs.cs
- ListBox.cs
- FrameworkContextData.cs
- GridViewUpdateEventArgs.cs
- SuppressIldasmAttribute.cs
- WebPartsPersonalizationAuthorization.cs
- BackgroundWorker.cs
- SplitterCancelEvent.cs
- SaveFileDialog.cs
- TextTreeDeleteContentUndoUnit.cs
- MessageProperties.cs