Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / TemplateKey.cs / 1305600 / TemplateKey.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Base class for DataTemplateKey, TableTemplateKey. // //--------------------------------------------------------------------------- using System; using System.Reflection; using System.ComponentModel; using System.Windows.Markup; using MS.Internal.Data; // DataBindEngine.EnglishUSCulture namespace System.Windows { ///The TemplateKey object is used as the resource key for data templates [TypeConverter(typeof(TemplateKeyConverter))] public abstract class TemplateKey : ResourceKey, ISupportInitialize { ///Constructor (called by derived classes only) protected TemplateKey(TemplateType templateType) { _dataType = null; // still needs to be initialized _templateType = templateType; } ///Constructor (called by derived classes only) protected TemplateKey(TemplateType templateType, object dataType) { Exception ex = ValidateDataType(dataType, "dataType"); if (ex != null) throw ex; _dataType = dataType; _templateType = templateType; } #region ISupportInitialize ///Begin Initialization void ISupportInitialize.BeginInit() { _initializing = true; } ///End Initialization, verify that internal state is consistent void ISupportInitialize.EndInit() { if (_dataType == null) { throw new InvalidOperationException(SR.Get(SRID.PropertyMustHaveValue, "DataType", this.GetType().Name)); } _initializing = false; } #endregion ISupportInitialize ////// The type for which the template is designed. This is either /// a Type (for object data), or a string (for XML data). In the latter /// case the string denotes the XML tag name. /// public object DataType { get { return _dataType; } set { if (!_initializing) throw new InvalidOperationException(SR.Get(SRID.PropertyIsInitializeOnly, "DataType", this.GetType().Name)); if (_dataType != null && value != _dataType) throw new InvalidOperationException(SR.Get(SRID.PropertyIsImmutable, "DataType", this.GetType().Name)); Exception ex = ValidateDataType(value, "value"); if (ex != null) throw ex; _dataType = value; } } ///Override of Object.GetHashCode() public override int GetHashCode() { // note that the hash code can change, but only during intialization // and only once (DataType can only be changed once, from null to // non-null, and that can only happen during [Begin/End]Init). // Technically this is still a violation of the "constant during // lifetime" rule, however in practice this is acceptable. It is // very unlikely that someone will put a TemplateKey into a hashtable // before it is initialized. int hashcode = (int)_templateType; if (_dataType != null) { hashcode += _dataType.GetHashCode(); } return hashcode; } ///Override of Object.Equals() public override bool Equals(object o) { TemplateKey key = o as TemplateKey; if (key != null) { return _templateType == key._templateType && Object.Equals(_dataType, key._dataType); } return false; } ///Override of Object.ToString() public override string ToString() { Type type = DataType as Type; return (DataType != null) ? String.Format(TypeConverterHelper.InvariantEnglishUS, "{0}({1})", this.GetType().Name, DataType) : String.Format(TypeConverterHelper.InvariantEnglishUS, "{0}(null)", this.GetType().Name); } ////// Allows SystemResources to know which assembly the template might be defined in. /// public override Assembly Assembly { get { Type type = _dataType as Type; if (type != null) { return type.Assembly; } return null; } } ///The different types of templates that use TemplateKey protected enum TemplateType { ///DataTemplate DataTemplate, ///TableTemplate TableTemplate, } // Validate against these rules // 1. dataType must not be null (except at initialization, which is tested at EndInit) // 2. dataType must be either a Type (object data) or a string (XML tag name) // 3. dataType cannot be typeof(Object) internal static Exception ValidateDataType(object dataType, string argName) { Exception result = null; if (dataType == null) { result = new ArgumentNullException(argName); } else if (!(dataType is Type) && !(dataType is String)) { result = new ArgumentException(SR.Get(SRID.MustBeTypeOrString, dataType.GetType().Name), argName); } else if (typeof(Object).Equals(dataType)) { result = new ArgumentException(SR.Get(SRID.DataTypeCannotBeObject), argName); } return result; } object _dataType; TemplateType _templateType; bool _initializing; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
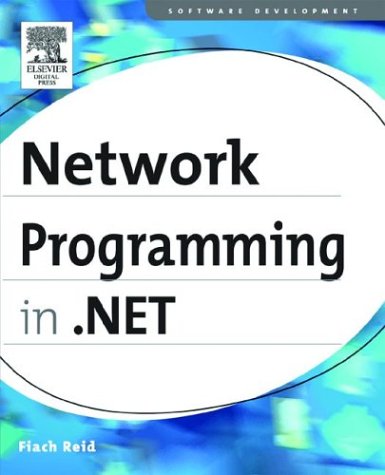
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AVElementHelper.cs
- MetadataFile.cs
- CustomCategoryAttribute.cs
- ConstructorExpr.cs
- DataBoundControlHelper.cs
- UserControlBuildProvider.cs
- Translator.cs
- DesignerLabelAdapter.cs
- ReaderWriterLock.cs
- RtfToken.cs
- AuthenticationService.cs
- RegexCharClass.cs
- TagPrefixAttribute.cs
- ToolStripItemDesigner.cs
- DataViewListener.cs
- SizeChangedInfo.cs
- OSEnvironmentHelper.cs
- AggregationMinMaxHelpers.cs
- SplineKeyFrames.cs
- X509CertificateTrustedIssuerElement.cs
- ShapeTypeface.cs
- HwndAppCommandInputProvider.cs
- ZoneButton.cs
- LinkLabel.cs
- GridViewColumnHeader.cs
- XmlValidatingReaderImpl.cs
- UnsignedPublishLicense.cs
- Attachment.cs
- IItemContainerGenerator.cs
- BindingManagerDataErrorEventArgs.cs
- _HTTPDateParse.cs
- SharedConnectionInfo.cs
- DesignerActionTextItem.cs
- UserControlBuildProvider.cs
- ColumnHeaderConverter.cs
- DetailsViewAutoFormat.cs
- RijndaelCryptoServiceProvider.cs
- HttpValueCollection.cs
- PointAnimation.cs
- DbQueryCommandTree.cs
- SQlBooleanStorage.cs
- CompressedStack.cs
- HttpCachePolicyElement.cs
- SqlCharStream.cs
- SystemInfo.cs
- ReferencedCollectionType.cs
- RuleSet.cs
- XmlSchemaAttributeGroupRef.cs
- MouseBinding.cs
- UserPreferenceChangingEventArgs.cs
- MenuItemBinding.cs
- ResXFileRef.cs
- PersianCalendar.cs
- FixedPageAutomationPeer.cs
- ValidationSummaryDesigner.cs
- PreloadedPackages.cs
- PaintEvent.cs
- XmlDownloadManager.cs
- OleDbCommandBuilder.cs
- SafeSecurityHelper.cs
- Decimal.cs
- MissingMemberException.cs
- Help.cs
- DataObjectPastingEventArgs.cs
- ReachSerializer.cs
- _DomainName.cs
- PasswordPropertyTextAttribute.cs
- DataGridViewSelectedColumnCollection.cs
- PersonalizationProviderCollection.cs
- SmtpClient.cs
- NamespaceCollection.cs
- EditingMode.cs
- NumberFormatter.cs
- WindowsSidIdentity.cs
- SurrogateEncoder.cs
- ComplexTypeEmitter.cs
- smtpconnection.cs
- InitializationEventAttribute.cs
- TreeNodeConverter.cs
- Int32Storage.cs
- ValueUtilsSmi.cs
- PropertyMetadata.cs
- SystemSounds.cs
- FormatException.cs
- SchemaDeclBase.cs
- CmsInterop.cs
- DocumentGridPage.cs
- LiteralControl.cs
- SchemaCollectionPreprocessor.cs
- RouteCollection.cs
- WebContext.cs
- ProgressBar.cs
- Profiler.cs
- FlowDocumentPage.cs
- SqlDataSourceSummaryPanel.cs
- OdbcUtils.cs
- TrustSection.cs
- Variable.cs
- SqlCharStream.cs
- HtmlInputButton.cs