Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / LiteralControl.cs / 1 / LiteralControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * Control that holds a literal string * * Copyright (c) 1999 Microsoft Corporation */ namespace System.Web.UI { using System; using System.ComponentModel; using System.ComponentModel.Design; using System.IO; using System.Security.Permissions; ////// [ ToolboxItem(false) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class LiteralControl : Control, ITextControl #if ORCAS , IPaginationInfo #endif { internal string _text; ///Defines the properties and methods of the LiteralControl class. A /// literal control is usually rendered as HTML text on a page. ////// LiteralControls behave as text holders, i.e., the parent of a LiteralControl may decide /// to extract its text, and remove the control from its Control collection (typically for /// performance reasons). /// Therefore a control derived from LiteralControl must do any preprocessing of its Text /// when it hands it out, that it would otherwise have done in its Render implementation. /// ////// public LiteralControl() { PreventAutoID(); SetEnableViewStateInternal(false); } ///Creates a control that holds a literal string. ////// public LiteralControl(string text) : this() { _text = (text != null) ? text : String.Empty; } ///Initializes a new instance of the LiteralControl class with /// the specified text. ////// public virtual string Text { get { return _text; } set { _text = (value != null) ? value : String.Empty; } } protected override ControlCollection CreateControlCollection() { return new EmptyControlCollection(this); } #if ORCAS ///Gets or sets the text content of the literal control. ///bool IPaginationInfo.PaginateChildren { get { return PaginateChildren; } } /// protected virtual bool PaginateChildren { get { return false; } } /// int IPaginationInfo.Weight { get { return Weight; } } /// protected virtual int Weight { get { int length = _text.Length; if (length >= 50) { return 100; } for (int i = 0; i < length; i++) { if (i==50 || !Char.IsWhiteSpace(_text, i)) { return 100; } } return 0; } } #endif /// /// protected internal override void Render(HtmlTextWriter output) { output.Write(_text); } internal override void InitRecursive(Control namingContainer) { ResolveAdapter(); if (_adapter != null) { _adapter.OnInit(EventArgs.Empty); } else { OnInit(EventArgs.Empty); } } internal override void LoadRecursive() { if(_adapter != null) { _adapter.OnLoad(EventArgs.Empty); } else { OnLoad(EventArgs.Empty); } } internal override void PreRenderRecursiveInternal() { if(_adapter != null) { _adapter.OnPreRender(EventArgs.Empty); } else { OnPreRender(EventArgs.Empty); } } internal override void UnloadRecursive(bool dispose) { if(_adapter != null) { _adapter.OnUnload(EventArgs.Empty); } else { OnUnload(EventArgs.Empty); } // if (dispose) Dispose(); } } /* * Class used to access literal strings stored in a resource (perf optimization). * This class is only public because it needs to be used by the generated classes. * Users should not use directly. */ internal sealed class ResourceBasedLiteralControl : LiteralControl { private TemplateControl _tplControl; private int _offset; // Offset of the start of this string in the resource private int _size; // Size of this string in bytes private bool _fAsciiOnly; // Does the string contain only 7-bit ascii characters internal ResourceBasedLiteralControl(TemplateControl tplControl, int offset, int size, bool fAsciiOnly) { // Make sure we don't access invalid data if (offset < 0 || offset+size > tplControl.MaxResourceOffset) throw new ArgumentException(); _tplControl = tplControl; _offset = offset; _size = size; _fAsciiOnly = fAsciiOnly; PreventAutoID(); EnableViewState = false; } public override string Text { get { // If it's just a normal string, call the base if (_size == 0) return base.Text; return StringResourceManager.ResourceToString( _tplControl.StringResourcePointer, _offset, _size); } set { // From now on, this will behave like a normal LiteralControl _size = 0; base.Text = value; } } protected internal override void Render(HtmlTextWriter output) { // If it's just a normal string, call the base if (_size == 0) { base.Render(output); return; } output.WriteUTF8ResourceString(_tplControl.StringResourcePointer, _offset, _size, _fAsciiOnly); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Saves any state that was modified after mark. ///
Link Menu
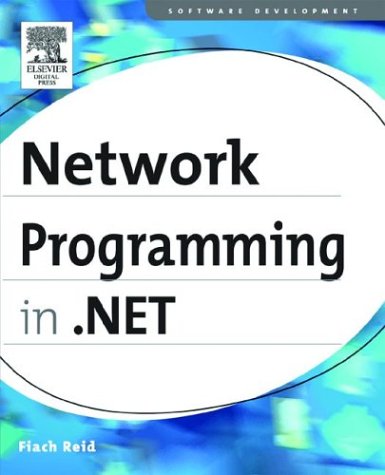
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CustomMenuItemCollection.cs
- MatrixKeyFrameCollection.cs
- ExpanderAutomationPeer.cs
- GeometryValueSerializer.cs
- Trigger.cs
- X509Certificate.cs
- CacheChildrenQuery.cs
- SimpleTableProvider.cs
- PropertyTab.cs
- QilBinary.cs
- DataBindingList.cs
- SocketPermission.cs
- Empty.cs
- FieldBuilder.cs
- XmlSchemaObjectTable.cs
- SimpleHandlerBuildProvider.cs
- IMembershipProvider.cs
- XmlKeywords.cs
- SimpleApplicationHost.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- HotSpotCollection.cs
- ThumbAutomationPeer.cs
- contentDescriptor.cs
- ConfigPathUtility.cs
- XslTransform.cs
- COM2EnumConverter.cs
- Assert.cs
- DecimalKeyFrameCollection.cs
- RectAnimationClockResource.cs
- SecurityTokenValidationException.cs
- BindingBase.cs
- BlockUIContainer.cs
- WebPartExportVerb.cs
- DragDeltaEventArgs.cs
- ModuleConfigurationInfo.cs
- DataColumn.cs
- FormsIdentity.cs
- Tokenizer.cs
- X509CertificateInitiatorServiceCredential.cs
- Attributes.cs
- BitmapCache.cs
- SchemaCollectionPreprocessor.cs
- DataGridViewToolTip.cs
- BuiltInPermissionSets.cs
- WmpBitmapDecoder.cs
- SymmetricSecurityBindingElement.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- HtmlImage.cs
- InfoCardArgumentException.cs
- Base64Encoder.cs
- ReflectTypeDescriptionProvider.cs
- TextAction.cs
- CategoryGridEntry.cs
- NullableDecimalSumAggregationOperator.cs
- BindingListCollectionView.cs
- RemotingSurrogateSelector.cs
- EtwTrace.cs
- DecoderBestFitFallback.cs
- HttpModuleCollection.cs
- SQLBytesStorage.cs
- EntityObject.cs
- ArraySubsetEnumerator.cs
- HtmlString.cs
- TextEditorMouse.cs
- MailSettingsSection.cs
- SubMenuStyleCollection.cs
- _IPv4Address.cs
- SqlDeflator.cs
- MarkupProperty.cs
- CollectionTraceRecord.cs
- RadioButtonBaseAdapter.cs
- MessageAction.cs
- ComEventsMethod.cs
- ActivityCodeDomReferenceService.cs
- XmlSchema.cs
- CLRBindingWorker.cs
- CreateUserWizardStep.cs
- XslCompiledTransform.cs
- EvidenceTypeDescriptor.cs
- CmsInterop.cs
- ComNativeDescriptor.cs
- StackOverflowException.cs
- UIElement3DAutomationPeer.cs
- ThreadStaticAttribute.cs
- WebContext.cs
- Literal.cs
- ComponentConverter.cs
- Stylesheet.cs
- WebPartManagerInternals.cs
- backend.cs
- SingleTagSectionHandler.cs
- HashMembershipCondition.cs
- HijriCalendar.cs
- ExtenderProvidedPropertyAttribute.cs
- TextReader.cs
- Deserializer.cs
- FactoryGenerator.cs
- _ConnectOverlappedAsyncResult.cs
- DataRecord.cs
- WSFederationHttpBindingCollectionElement.cs