Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / RectAnimationClockResource.cs / 1305600 / RectAnimationClockResource.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; namespace System.Windows.Media.Animation { ////// RectAnimationClockResource class. /// AnimationClockResource classes refer to an AnimationClock and a base /// value. They implement DUCE.IResource, and thus can be used to produce /// a render-side resource which represents the current value of this /// AnimationClock. /// They subscribe to the Changed event on the AnimationClock and ensure /// that the resource's current value is up to date. /// internal class RectAnimationClockResource: AnimationClockResource, DUCE.IResource { ////// Constructor for public RectAnimationClockResource. /// This constructor accepts the base value and AnimationClock. /// Note that since there is no current requirement that we be able to set or replace either the /// base value or the AnimationClock, this is the only way to initialize an instance of /// RectAnimationClockResource. /// Also, we currently Assert that the resource is non-null, since without mutability /// such a resource isn't needed. /// We can easily extend this class if/when new requirements arise. /// /// Rect - The base value. /// AnimationClock - cannot be null. public RectAnimationClockResource( Rect baseValue, AnimationClock animationClock ): base( animationClock ) { _baseValue = baseValue; } #region Public Properties ////// BaseValue Property - typed accessor for BaseValue. /// public Rect BaseValue { get { return _baseValue; } } ////// CurrentValue Property - typed accessor for CurrentValue /// public Rect CurrentValue { get { if (_animationClock != null) { // No handoff for DrawingContext animations so we use the // BaseValue as the defaultOriginValue and the // defaultDestinationValue. We call the Timeline's GetCurrentValue // directly to avoid boxing return ((RectAnimationBase)(_animationClock.Timeline)).GetCurrentValue( _baseValue, // defaultOriginValue _baseValue, // defaultDesinationValue _animationClock); // clock } else { return _baseValue; } } } #endregion Public Properties #region DUCE // // Method which returns the DUCE type of this class. // The base class needs this type when calling CreateOrAddRefOnChannel. // By providing this via a virtual, we avoid a per-instance storage cost. // protected override DUCE.ResourceType ResourceType { get { return DUCE.ResourceType.TYPE_RECTRESOURCE; } } ////// UpdateResource - This method is called to update the render-thread /// resource on a given channel. /// /// The DUCE.ResourceHandle for this resource on this channel. /// The channel on which to update the render-thread resource. ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels can handle bad pointers and will not affect other appdomains or processes /// [SecurityCritical,SecurityTreatAsSafe] protected override void UpdateResource( DUCE.ResourceHandle handle, DUCE.Channel channel) { DUCE.MILCMD_RECTRESOURCE cmd = new DUCE.MILCMD_RECTRESOURCE(); cmd.Type = MILCMD.MilCmdRectResource; cmd.Handle = handle; cmd.Value = CurrentValue; unsafe { channel.SendCommand( (byte*)&cmd, sizeof(DUCE.MILCMD_RECTRESOURCE)); } // Validate this resource IsResourceInvalid = false; } #endregion DUCE private Rect _baseValue; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; namespace System.Windows.Media.Animation { ////// RectAnimationClockResource class. /// AnimationClockResource classes refer to an AnimationClock and a base /// value. They implement DUCE.IResource, and thus can be used to produce /// a render-side resource which represents the current value of this /// AnimationClock. /// They subscribe to the Changed event on the AnimationClock and ensure /// that the resource's current value is up to date. /// internal class RectAnimationClockResource: AnimationClockResource, DUCE.IResource { ////// Constructor for public RectAnimationClockResource. /// This constructor accepts the base value and AnimationClock. /// Note that since there is no current requirement that we be able to set or replace either the /// base value or the AnimationClock, this is the only way to initialize an instance of /// RectAnimationClockResource. /// Also, we currently Assert that the resource is non-null, since without mutability /// such a resource isn't needed. /// We can easily extend this class if/when new requirements arise. /// /// Rect - The base value. /// AnimationClock - cannot be null. public RectAnimationClockResource( Rect baseValue, AnimationClock animationClock ): base( animationClock ) { _baseValue = baseValue; } #region Public Properties ////// BaseValue Property - typed accessor for BaseValue. /// public Rect BaseValue { get { return _baseValue; } } ////// CurrentValue Property - typed accessor for CurrentValue /// public Rect CurrentValue { get { if (_animationClock != null) { // No handoff for DrawingContext animations so we use the // BaseValue as the defaultOriginValue and the // defaultDestinationValue. We call the Timeline's GetCurrentValue // directly to avoid boxing return ((RectAnimationBase)(_animationClock.Timeline)).GetCurrentValue( _baseValue, // defaultOriginValue _baseValue, // defaultDesinationValue _animationClock); // clock } else { return _baseValue; } } } #endregion Public Properties #region DUCE // // Method which returns the DUCE type of this class. // The base class needs this type when calling CreateOrAddRefOnChannel. // By providing this via a virtual, we avoid a per-instance storage cost. // protected override DUCE.ResourceType ResourceType { get { return DUCE.ResourceType.TYPE_RECTRESOURCE; } } ////// UpdateResource - This method is called to update the render-thread /// resource on a given channel. /// /// The DUCE.ResourceHandle for this resource on this channel. /// The channel on which to update the render-thread resource. ////// Critical: This code calls into an unsafe code block /// TreatAsSafe: This code does not return any critical data.It is ok to expose /// Channels can handle bad pointers and will not affect other appdomains or processes /// [SecurityCritical,SecurityTreatAsSafe] protected override void UpdateResource( DUCE.ResourceHandle handle, DUCE.Channel channel) { DUCE.MILCMD_RECTRESOURCE cmd = new DUCE.MILCMD_RECTRESOURCE(); cmd.Type = MILCMD.MilCmdRectResource; cmd.Handle = handle; cmd.Value = CurrentValue; unsafe { channel.SendCommand( (byte*)&cmd, sizeof(DUCE.MILCMD_RECTRESOURCE)); } // Validate this resource IsResourceInvalid = false; } #endregion DUCE private Rect _baseValue; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
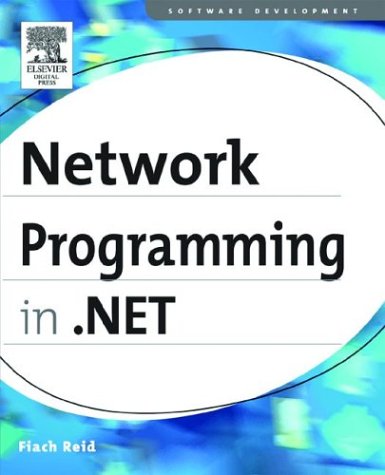
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimeSpanConverter.cs
- DataGridViewCell.cs
- Popup.cs
- XmlAttributes.cs
- ControlCollection.cs
- MissingManifestResourceException.cs
- MappingSource.cs
- Section.cs
- PrinterSettings.cs
- ScrollItemProviderWrapper.cs
- BypassElementCollection.cs
- CurrentChangedEventManager.cs
- UIInitializationException.cs
- DeploymentSection.cs
- DataSourceComponent.cs
- AndMessageFilterTable.cs
- ReferenceSchema.cs
- basenumberconverter.cs
- TaskForm.cs
- ModelFunctionTypeElement.cs
- TextParaClient.cs
- TopClause.cs
- MatrixTransform.cs
- Matrix3DStack.cs
- DbExpressionVisitor_TResultType.cs
- Single.cs
- GetMemberBinder.cs
- DrawingContextDrawingContextWalker.cs
- LineSegment.cs
- PathTooLongException.cs
- QueryOutputWriterV1.cs
- EntityDataSourceConfigureObjectContextPanel.cs
- EncoderReplacementFallback.cs
- ConfigXmlSignificantWhitespace.cs
- GroupQuery.cs
- Effect.cs
- SpinLock.cs
- WindowsFormsSectionHandler.cs
- XPathAncestorIterator.cs
- NetworkInformationException.cs
- DomainConstraint.cs
- XPathBuilder.cs
- NonParentingControl.cs
- _HeaderInfo.cs
- FusionWrap.cs
- DefaultWorkflowLoaderService.cs
- DataGridViewColumnDesignTimeVisibleAttribute.cs
- GridViewUpdateEventArgs.cs
- ProfileModule.cs
- CorePropertiesFilter.cs
- RtfFormatStack.cs
- ExpressionBuilderContext.cs
- ServiceChannelProxy.cs
- TagPrefixInfo.cs
- BindingsCollection.cs
- XmlSchemaException.cs
- Internal.cs
- XNodeNavigator.cs
- ContentPlaceHolder.cs
- State.cs
- WindowsGraphics.cs
- EntityDataSourceSelectingEventArgs.cs
- SortQuery.cs
- diagnosticsswitches.cs
- UnhandledExceptionEventArgs.cs
- ProcessManager.cs
- ConfigurationElement.cs
- GroupDescription.cs
- Rect.cs
- StorageAssociationSetMapping.cs
- PropertyCollection.cs
- FunctionImportMapping.cs
- Documentation.cs
- DataGridSortCommandEventArgs.cs
- StringBuilder.cs
- GlobalizationAssembly.cs
- MessageHeaders.cs
- ManualResetEvent.cs
- ToolstripProfessionalRenderer.cs
- WaitingCursor.cs
- FieldBuilder.cs
- CommentEmitter.cs
- ProcessHost.cs
- HtmlControlPersistable.cs
- Rotation3DKeyFrameCollection.cs
- CodeTypeMember.cs
- SQLMoney.cs
- FunctionNode.cs
- BuildProvider.cs
- returneventsaver.cs
- SecurityResources.cs
- Tag.cs
- MergeFailedEvent.cs
- EventSinkActivityDesigner.cs
- TextTreeInsertUndoUnit.cs
- _ProxyChain.cs
- ScrollEvent.cs
- CodeCompiler.cs
- CapabilitiesRule.cs
- EdgeProfileValidation.cs