Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / System / data / design / DataSourceComponent.cs / 1 / DataSourceComponent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Data.Design { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; internal abstract class DataSourceComponent : Component, ICustomTypeDescriptor, IObjectWithParent, IDataSourceCollectionMember, IDataSourceRenamableObject { private DataSourceCollectionBase collectionParent; internal protected virtual DataSourceCollectionBase CollectionParent { get { return collectionParent; } set { collectionParent = value; } } ////// return the object supports external properties /// protected virtual object ExternalPropertyHost { get { return null; } } ////// for IObjectWithParent /// [Browsable(false)] public virtual object Parent { get { return collectionParent; } } ////// Retrieves an array of member attributes for the given object. /// ////// the array of attributes on the class. This will never be null. /// ///AttributeCollection ICustomTypeDescriptor.GetAttributes() { return TypeDescriptor.GetAttributes(GetType()); } /// /// Retrieves the class name for this object. If null is returned, /// the type name is used. /// ////// The class name for the object, or null if the default will be used. /// ///string ICustomTypeDescriptor.GetClassName() { if (this is IDataSourceNamedObject) { return ((IDataSourceNamedObject)this).PublicTypeName; } return null; } /// /// Retrieves the name for this object. If null is returned, /// the default is used. /// ////// The name for the object, or null if the default will be used. /// ///string ICustomTypeDescriptor.GetComponentName() { INamedObject namedObject = this as INamedObject; return namedObject != null ? namedObject.Name : null; } /// /// Retrieves the type converter for this object. /// ////// A TypeConverter. If null is returned, the default is used. /// ///TypeConverter ICustomTypeDescriptor.GetConverter() { return TypeDescriptor.GetConverter(GetType()); } /// /// Retrieves the default event. /// ////// the default event, or null if there are no /// events /// ///EventDescriptor ICustomTypeDescriptor.GetDefaultEvent() { return TypeDescriptor.GetDefaultEvent(GetType()); } /// /// Retrieves the default property. /// ////// the default property, or null if there are no /// properties /// ///PropertyDescriptor ICustomTypeDescriptor.GetDefaultProperty() { return TypeDescriptor.GetDefaultProperty(GetType()); } /// /// Retrieves the an editor for this object. /// ////// An editor of the requested type, or null. /// ///object ICustomTypeDescriptor.GetEditor(Type editorBaseType) { return TypeDescriptor.GetEditor(GetType(), editorBaseType); } /// /// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. /// ////// an array of events this component surfaces. /// ///EventDescriptorCollection ICustomTypeDescriptor.GetEvents() { return TypeDescriptor.GetEvents(GetType()); } /// /// Retrieves an array of events that the given component instance /// provides. This may differ from the set of events the class /// provides. If the component is sited, the site may add or remove /// additional events. The returned array of events will be /// filtered by the given set of attributes. /// /// /// A set of attributes to use as a filter. /// /// If a MemberAttribute instance is specified and /// the event does not have an instance of that attribute's /// class, this will still include the event if the /// MemberAttribute is the same as it's Default property. /// ////// an array of events this component surfaces that match /// the given set of attributes.. /// ///EventDescriptorCollection ICustomTypeDescriptor.GetEvents(Attribute[] attributes) { return TypeDescriptor.GetEvents(GetType(), attributes); } /// /// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. /// ////// an array of properties this component surfaces. /// ///PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties() { return GetProperties(null); } /// /// Retrieves an array of properties that the given component instance /// provides. This may differ from the set of properties the class /// provides. If the component is sited, the site may add or remove /// additional properties. The returned array of properties will be /// filtered by the given set of attributes. /// /// /// A set of attributes to use as a filter. /// /// If a MemberAttribute instance is specified and /// the property does not have an instance of that attribute's /// class, this will still include the property if the /// MemberAttribute is the same as it's Default property. /// ////// an array of properties this component surfaces that match /// the given set of attributes.. /// ///PropertyDescriptorCollection ICustomTypeDescriptor.GetProperties(Attribute[] attributes) { return GetProperties(attributes); } private PropertyDescriptorCollection GetProperties(Attribute[] attributes) { return TypeDescriptor.GetProperties(GetType(), attributes); } /// /// Retrieves the object that directly depends on this value being edited. This is /// generally the object that is required for the PropertyDescriptor's GetValue and SetValue /// methods. If 'null' is passed for the PropertyDescriptor, the ICustomComponent /// descripotor implemementation should return the default object, that is the main /// object that exposes the properties and attributes, /// /// /// The PropertyDescriptor to find the owner for. This call should return an object /// such that the call "pd.GetValue(GetPropertyOwner(pd));" will generally succeed. /// If 'null' is passed for pd, the main object that owns the properties and attributes /// should be returned. /// ////// valueOwner /// ///object ICustomTypeDescriptor.GetPropertyOwner(PropertyDescriptor pd) { return this; } /// /// Returns an object representing a service provided by the component. /// /// type of the service ///protected override object GetService(Type service) { // Query service from its parent node if the object is not sited. // DataSourceComponent component = this; while (component != null && component.Site == null) { if (component.CollectionParent != null) { component = component.CollectionParent.CollectionHost; } else if (component.Parent != null && component.Parent is DataSourceComponent) { component = component.Parent as DataSourceComponent; } else { component = null; } } if (component != null && component.Site != null) { return component.Site.GetService(service); } return null; } /// /// IDataSourceCollectionMember implementation /// public virtual void SetCollection(DataSourceCollectionBase collection) { CollectionParent = collection; } ////// call this function to set value, so there will be componentChanging/Changed events /// /// call this function to set value, so there will be componentChanging/Changed events internal void SetPropertyValue(string propertyName, object value) { //bugbug removed } internal virtual StringCollection NamingPropertyNames { get { return null; } } // IDataSourceRenamableObject implementation [Browsable(false)] public virtual string GeneratorName { get { return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
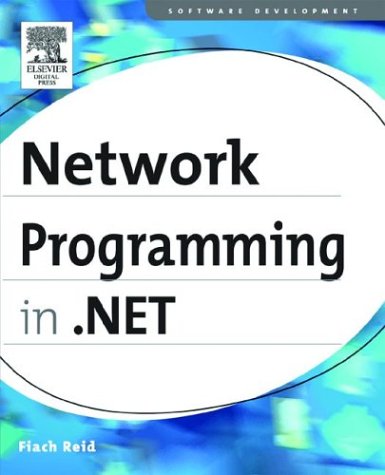
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Point3DCollection.cs
- HttpWebResponse.cs
- DeferredElementTreeState.cs
- EventMappingSettings.cs
- UInt32.cs
- WebPartCollection.cs
- WindowsPen.cs
- PropertyEmitter.cs
- UnicodeEncoding.cs
- ConfigurationStrings.cs
- DbConnectionStringCommon.cs
- XmlSchemaSet.cs
- AdornerLayer.cs
- XPathParser.cs
- TextRunTypographyProperties.cs
- SurrogateEncoder.cs
- WebControl.cs
- IntegerFacetDescriptionElement.cs
- OrElse.cs
- Point3DAnimation.cs
- DeobfuscatingStream.cs
- FixedTextView.cs
- CatalogPartDesigner.cs
- SchemaDeclBase.cs
- CompensationParticipant.cs
- NativeObjectSecurity.cs
- KeyFrames.cs
- ColorPalette.cs
- OwnerDrawPropertyBag.cs
- ThreadStaticAttribute.cs
- Add.cs
- Sql8ConformanceChecker.cs
- ResourceBinder.cs
- ListChangedEventArgs.cs
- XmlSerializerOperationGenerator.cs
- TextBox.cs
- EdmToObjectNamespaceMap.cs
- Renderer.cs
- ReceiveActivityDesigner.cs
- CipherData.cs
- SubpageParagraph.cs
- ScriptManagerProxy.cs
- ParseElementCollection.cs
- ListenerAdaptersInstallComponent.cs
- codemethodreferenceexpression.cs
- ComboBoxAutomationPeer.cs
- Control.cs
- SqlBulkCopyColumnMappingCollection.cs
- TextFindEngine.cs
- Quad.cs
- IsolatedStorageFileStream.cs
- AddingNewEventArgs.cs
- StagingAreaInputItem.cs
- PanelDesigner.cs
- HttpWebRequest.cs
- ObjectListCommandCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- NetWebProxyFinder.cs
- CustomValidator.cs
- DaylightTime.cs
- HttpDictionary.cs
- Rotation3DAnimationUsingKeyFrames.cs
- ToolStripControlHost.cs
- ReadWriteObjectLock.cs
- PointAnimationUsingKeyFrames.cs
- ImpersonationContext.cs
- RuntimeResourceSet.cs
- AppDomainAttributes.cs
- _LazyAsyncResult.cs
- FormViewPagerRow.cs
- DataServiceExpressionVisitor.cs
- NavigationFailedEventArgs.cs
- ContractHandle.cs
- sqlmetadatafactory.cs
- PropertyGrid.cs
- ParameterCollection.cs
- WebZone.cs
- RoleServiceManager.cs
- UnwrappedTypesXmlSerializerManager.cs
- UniqueSet.cs
- EntityUtil.cs
- COM2PictureConverter.cs
- ComponentChangingEvent.cs
- DesignerRegionCollection.cs
- ResourceDescriptionAttribute.cs
- PositiveTimeSpanValidator.cs
- AdRotatorDesigner.cs
- UIElementHelper.cs
- TrackingStringDictionary.cs
- PrimitiveXmlSerializers.cs
- PassportAuthenticationModule.cs
- _TimerThread.cs
- InvalidDataContractException.cs
- DbUpdateCommandTree.cs
- Menu.cs
- CacheChildrenQuery.cs
- UIElementParagraph.cs
- TTSEngineProxy.cs
- ToolStripItemClickedEventArgs.cs
- UrlPath.cs