Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbQueryCommandTree.cs / 1 / DbQueryCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Represents a query operation expressed as a canonical command tree. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbQueryCommandTree : DbCommandTree { private ExpressionLink _query; ////// Gets or sets an ///that defines the logic of the query operation. /// The expression is null ///The expression is associated with a different command tree public DbExpression Query { get { return _query.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { using (new EntityBid.ScopeAuto("%d#", this.ObjectId)) { EntityBid.Trace(" %d#, value=%d#, %d{cqt.DbExpressionKind}\n", this.ObjectId, DbExpression.GetObjectId(value), DbExpression.GetExpressionKind(value)); _query.Expression = value; } } } /// /// Constructs a new DbQueryCommandTree that uses the specified metadata workspace. /// /// The metadata workspace that the command tree should use. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. ////// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbQueryCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) : base(metadata, dataSpace) { using (new EntityBid.ScopeAuto(" does not represent a valid data space %d#", this.ObjectId)) { _query = new ExpressionLink("Query", this); } } /// /// Clones this command tree. This operation creates a new DbQueryCommandTree for which each expression property value is a clone of the corresponding property of this command tree. /// ///The cloned command tree /*CQT_PUBLIC_API(*/internal/*)*/ DbQueryCommandTree Clone() { using (new EntityBid.ScopeAuto("%d#", this.ObjectId)) { DbQueryCommandTree newTree = new DbQueryCommandTree( this.MetadataWorkspace, this.DataSpace ); this.CopyParametersTo(newTree); if (this.Query != null) { newTree.Query = ExpressionCopier.Copy(newTree, this.Query); } return newTree; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Query; } } internal override void DumpStructure(ExpressionDumper dumper) { if (this.Query != null) { dumper.Dump(this.Query, "Query"); } } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal override void Replace(ExpressionReplacer replacer) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { if (this.Query != null) { DbExpression newQuery = replacer.Replace(this.Query); if (!object.ReferenceEquals(newQuery, this.Query)) { this.Query = newQuery; } } } } internal override void Validate(System.Data.Common.CommandTrees.Internal.Validator v) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { v.Validate(this); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { ////// Represents a query operation expressed as a canonical command tree. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbQueryCommandTree : DbCommandTree { private ExpressionLink _query; ////// Gets or sets an ///that defines the logic of the query operation. /// The expression is null ///The expression is associated with a different command tree public DbExpression Query { get { return _query.Expression; } /*CQT_PUBLIC_API(*/internal/*)*/ set { using (new EntityBid.ScopeAuto("%d#", this.ObjectId)) { EntityBid.Trace(" %d#, value=%d#, %d{cqt.DbExpressionKind}\n", this.ObjectId, DbExpression.GetObjectId(value), DbExpression.GetExpressionKind(value)); _query.Expression = value; } } } /// /// Constructs a new DbQueryCommandTree that uses the specified metadata workspace. /// /// The metadata workspace that the command tree should use. /// The logical 'space' that metadata in the expressions used in this command tree must belong to. ////// or is null /*CQT_PUBLIC_API(*/internal/*)*/ DbQueryCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) : base(metadata, dataSpace) { using (new EntityBid.ScopeAuto(" does not represent a valid data space %d#", this.ObjectId)) { _query = new ExpressionLink("Query", this); } } /// /// Clones this command tree. This operation creates a new DbQueryCommandTree for which each expression property value is a clone of the corresponding property of this command tree. /// ///The cloned command tree /*CQT_PUBLIC_API(*/internal/*)*/ DbQueryCommandTree Clone() { using (new EntityBid.ScopeAuto("%d#", this.ObjectId)) { DbQueryCommandTree newTree = new DbQueryCommandTree( this.MetadataWorkspace, this.DataSpace ); this.CopyParametersTo(newTree); if (this.Query != null) { newTree.Query = ExpressionCopier.Copy(newTree, this.Query); } return newTree; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Query; } } internal override void DumpStructure(ExpressionDumper dumper) { if (this.Query != null) { dumper.Dump(this.Query, "Query"); } } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal override void Replace(ExpressionReplacer replacer) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { if (this.Query != null) { DbExpression newQuery = replacer.Replace(this.Query); if (!object.ReferenceEquals(newQuery, this.Query)) { this.Query = newQuery; } } } } internal override void Validate(System.Data.Common.CommandTrees.Internal.Validator v) { using (new EntityBid.ScopeAuto(" %d#", this.ObjectId)) { v.Validate(this); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
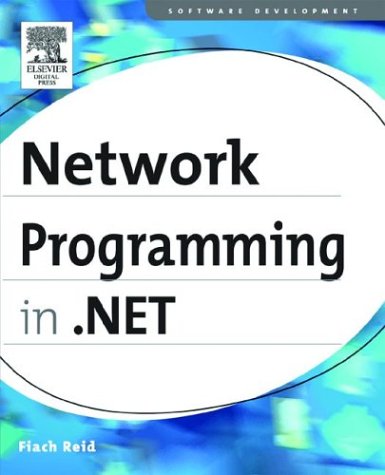
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlQualifiedName.cs
- TripleDESCryptoServiceProvider.cs
- StructuredProperty.cs
- JsonWriter.cs
- Visual.cs
- XmlCharCheckingWriter.cs
- UrlAuthFailedErrorFormatter.cs
- BordersPage.cs
- CallbackCorrelationInitializer.cs
- MouseEvent.cs
- WebRequest.cs
- DataBoundControlHelper.cs
- TableItemPatternIdentifiers.cs
- DescendentsWalker.cs
- ScrollPattern.cs
- DataColumnCollection.cs
- EdmProviderManifest.cs
- TextAdaptor.cs
- BufferBuilder.cs
- Normalization.cs
- DataGridState.cs
- ByteStreamMessageEncoder.cs
- FilteredXmlReader.cs
- ManipulationDevice.cs
- OptimizedTemplateContent.cs
- EventEntry.cs
- DeferredElementTreeState.cs
- BindingListCollectionView.cs
- ObsoleteAttribute.cs
- DataGridViewBindingCompleteEventArgs.cs
- ProfilePropertySettings.cs
- StandardToolWindows.cs
- ApplicationContext.cs
- PasswordDeriveBytes.cs
- BitmapCache.cs
- RulePatternOps.cs
- Mutex.cs
- ImageDrawing.cs
- PrintSystemException.cs
- XsltException.cs
- OperatingSystemVersionCheck.cs
- CustomAttributeBuilder.cs
- ErrorFormatterPage.cs
- TreeNode.cs
- Pen.cs
- TextContainerChangeEventArgs.cs
- AmbientValueAttribute.cs
- QueryContext.cs
- OperationResponse.cs
- ListItemParagraph.cs
- DataSourceXmlElementAttribute.cs
- Translator.cs
- OdbcError.cs
- RemotingConfiguration.cs
- Int32Animation.cs
- FixedSOMPage.cs
- ReferencedCollectionType.cs
- FixedTextContainer.cs
- mediaclock.cs
- UpdateManifestForBrowserApplication.cs
- TrackingDataItem.cs
- XmlSchemaSimpleType.cs
- WeakReference.cs
- CodeDomLocalizationProvider.cs
- BufferModesCollection.cs
- SymLanguageVendor.cs
- WebPartPersonalization.cs
- UpdatePanelTriggerCollection.cs
- TextTreeObjectNode.cs
- DataBoundControlHelper.cs
- SizeConverter.cs
- VirtualPathUtility.cs
- Sequence.cs
- FormDesigner.cs
- HostingMessageProperty.cs
- ConfigurationFileMap.cs
- FontCacheUtil.cs
- TargetFrameworkAttribute.cs
- EntityChangedParams.cs
- TextEditor.cs
- InfiniteTimeSpanConverter.cs
- AudioSignalProblemOccurredEventArgs.cs
- TextParagraphCache.cs
- SafeNativeMethodsOther.cs
- LinqDataSourceInsertEventArgs.cs
- CodeVariableReferenceExpression.cs
- ProcessProtocolHandler.cs
- QuaternionValueSerializer.cs
- SafeNativeMethods.cs
- HtmlDocument.cs
- DataListItemCollection.cs
- DataContractFormatAttribute.cs
- WebPartZoneBase.cs
- EventEntry.cs
- FixedSOMElement.cs
- SchemaObjectWriter.cs
- CheckBoxList.cs
- MailDefinition.cs
- PrivateFontCollection.cs
- FacetValueContainer.cs