Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / PrivateFontCollection.cs / 1 / PrivateFontCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Text { using System.Diagnostics; using System; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Globalization; ////// /// Encapsulates a collection of public sealed class PrivateFontCollection : FontCollection { ///objecs. /// /// /// public PrivateFontCollection() { nativeFontCollection = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipNewPrivateFontCollection(out nativeFontCollection); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Initializes a new instance of the ///class. /// /// /// protected override void Dispose(bool disposing) { if (nativeFontCollection != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeletePrivateFontCollection(out nativeFontCollection); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeFontCollection = IntPtr.Zero; } } base.Dispose(disposing); } ////// Cleans up Windows resources for this /// ///. /// /// /// public void AddFontFile (string filename) { IntSecurity.DemandReadFileIO(filename); int status = SafeNativeMethods.Gdip.GdipPrivateAddFontFile(new HandleRef(this, nativeFontCollection), filename); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); // Register private font with GDI as well so pure GDI-based controls (TextBox, Button for instance) can access it. SafeNativeMethods.AddFontFile( filename ); } // public void AddMemoryFont (IntPtr memory, int length) { IntSecurity.ObjectFromWin32Handle.Demand(); int status = SafeNativeMethods.Gdip.GdipPrivateAddMemoryFont(new HandleRef(this, nativeFontCollection), new HandleRef(null, memory), length); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Adds a font from the specified file to /// this ///. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Text { using System.Diagnostics; using System; using System.Drawing; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.ComponentModel; using Microsoft.Win32; using System.Security; using System.Security.Permissions; using System.Globalization; ////// /// Encapsulates a collection of public sealed class PrivateFontCollection : FontCollection { ///objecs. /// /// /// public PrivateFontCollection() { nativeFontCollection = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipNewPrivateFontCollection(out nativeFontCollection); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Initializes a new instance of the ///class. /// /// /// protected override void Dispose(bool disposing) { if (nativeFontCollection != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeletePrivateFontCollection(out nativeFontCollection); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsSecurityOrCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeFontCollection = IntPtr.Zero; } } base.Dispose(disposing); } ////// Cleans up Windows resources for this /// ///. /// /// /// public void AddFontFile (string filename) { IntSecurity.DemandReadFileIO(filename); int status = SafeNativeMethods.Gdip.GdipPrivateAddFontFile(new HandleRef(this, nativeFontCollection), filename); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); // Register private font with GDI as well so pure GDI-based controls (TextBox, Button for instance) can access it. SafeNativeMethods.AddFontFile( filename ); } // public void AddMemoryFont (IntPtr memory, int length) { IntSecurity.ObjectFromWin32Handle.Demand(); int status = SafeNativeMethods.Gdip.GdipPrivateAddMemoryFont(new HandleRef(this, nativeFontCollection), new HandleRef(null, memory), length); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Adds a font from the specified file to /// this ///. ///
Link Menu
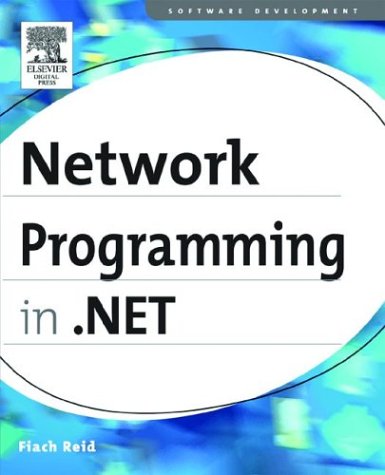
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ButtonColumn.cs
- PropertySourceInfo.cs
- GenericIdentity.cs
- StylusTouchDevice.cs
- ActivityCodeGenerator.cs
- ClientFactory.cs
- ListViewItem.cs
- SamlDoNotCacheCondition.cs
- EditingCoordinator.cs
- IList.cs
- QueryPageSettingsEventArgs.cs
- NativeMethods.cs
- WindowsFont.cs
- BrowserCapabilitiesCodeGenerator.cs
- ToolStripCustomTypeDescriptor.cs
- TextSelectionHighlightLayer.cs
- TypeSystemProvider.cs
- CodeCommentStatementCollection.cs
- ApplicationBuildProvider.cs
- DecimalFormatter.cs
- FunctionCommandText.cs
- QuotedPairReader.cs
- CellCreator.cs
- Processor.cs
- PrimarySelectionAdorner.cs
- OrderPreservingMergeHelper.cs
- NamespaceQuery.cs
- EntityDataSourceContainerNameConverter.cs
- ConfigXmlWhitespace.cs
- BooleanConverter.cs
- URLIdentityPermission.cs
- WebPartDisplayMode.cs
- OletxDependentTransaction.cs
- InputBinding.cs
- DispatcherTimer.cs
- EventLogPermissionEntryCollection.cs
- JsonSerializer.cs
- MaterializeFromAtom.cs
- LocalFileSettingsProvider.cs
- InstancePersistence.cs
- Calendar.cs
- TextBoxBase.cs
- SamlAttribute.cs
- SqlClientWrapperSmiStream.cs
- documentsequencetextcontainer.cs
- NotifyInputEventArgs.cs
- ServiceAuthorizationManager.cs
- IgnoreDataMemberAttribute.cs
- SourceLocationProvider.cs
- CLRBindingWorker.cs
- _emptywebproxy.cs
- linebase.cs
- Calendar.cs
- ITreeGenerator.cs
- SiteMapNodeItemEventArgs.cs
- FormsAuthenticationUserCollection.cs
- TheQuery.cs
- SqlDataReader.cs
- IndentedWriter.cs
- COM2EnumConverter.cs
- TraceSource.cs
- DataService.cs
- URI.cs
- ApplicationSettingsBase.cs
- CustomErrorsSectionWrapper.cs
- QueryContext.cs
- MailHeaderInfo.cs
- QilBinary.cs
- EntityDataSourceColumn.cs
- DataGridLinkButton.cs
- BoundPropertyEntry.cs
- SecurityKeyUsage.cs
- WCFBuildProvider.cs
- NamespaceQuery.cs
- ParsedAttributeCollection.cs
- CharUnicodeInfo.cs
- PanelDesigner.cs
- XmlSchemaExporter.cs
- TextAutomationPeer.cs
- DynamicILGenerator.cs
- ActivityMarkupSerializer.cs
- ModelTreeManager.cs
- EllipticalNodeOperations.cs
- EntityDataSourceMemberPath.cs
- InputBinding.cs
- TextViewSelectionProcessor.cs
- TimeEnumHelper.cs
- HeaderFilter.cs
- PipelineModuleStepContainer.cs
- UIElementParaClient.cs
- MasterPageBuildProvider.cs
- BuildResultCache.cs
- ProcessProtocolHandler.cs
- ClickablePoint.cs
- HttpRawResponse.cs
- SqlBulkCopyColumnMappingCollection.cs
- PermissionToken.cs
- ViewUtilities.cs
- SafeEventLogReadHandle.cs
- DecimalStorage.cs