Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWebControlsDesign / System / Data / WebControls / Design / EntityDataSourceContainerNameConverter.cs / 1305376 / EntityDataSourceContainerNameConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Web.UI.WebControls; namespace System.Web.UI.Design.WebControls { internal class EntityDataSourceContainerNameConverter : StringConverter { public EntityDataSourceContainerNameConverter() : base() { } public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { // We can only get a list of possible DefaultContainerName values if we have: // (1) Connection string so we can load metadata // Even if this value is set, it may not be possible to actually load the metadata, but at least we can try the lookup if requested EntityDataSource entityDataSource = context.Instance as EntityDataSource; if (entityDataSource != null && !String.IsNullOrEmpty(entityDataSource.ConnectionString)) { ListcontainerNameItems = new EntityDataSourceDesignerHelper(entityDataSource, false /*interactiveMode*/).GetContainerNames(true /*sortResults*/); string[] containers = new string[containerNameItems.Count]; for (int i = 0; i < containerNameItems.Count; i++) { containers[i] = containerNameItems[i].ToString(); } return new StandardValuesCollection(containers); } return null; } public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design; using System.Diagnostics; using System.Web.UI.WebControls; namespace System.Web.UI.Design.WebControls { internal class EntityDataSourceContainerNameConverter : StringConverter { public EntityDataSourceContainerNameConverter() : base() { } public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { // We can only get a list of possible DefaultContainerName values if we have: // (1) Connection string so we can load metadata // Even if this value is set, it may not be possible to actually load the metadata, but at least we can try the lookup if requested EntityDataSource entityDataSource = context.Instance as EntityDataSource; if (entityDataSource != null && !String.IsNullOrEmpty(entityDataSource.ConnectionString)) { ListcontainerNameItems = new EntityDataSourceDesignerHelper(entityDataSource, false /*interactiveMode*/).GetContainerNames(true /*sortResults*/); string[] containers = new string[containerNameItems.Count]; for (int i = 0; i < containerNameItems.Count; i++) { containers[i] = containerNameItems[i].ToString(); } return new StandardValuesCollection(containers); } return null; } public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
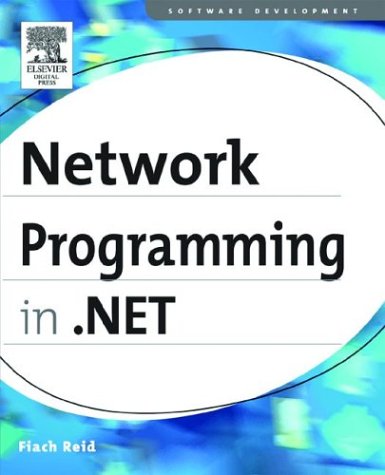
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CryptoApi.cs
- AuthenticatedStream.cs
- Decorator.cs
- ViewStateModeByIdAttribute.cs
- HttpDebugHandler.cs
- ProxyHwnd.cs
- ContentPresenter.cs
- FontSourceCollection.cs
- TypeSystem.cs
- DocumentationServerProtocol.cs
- GuidelineSet.cs
- Membership.cs
- DataViewSetting.cs
- ExpressionContext.cs
- Delay.cs
- IOException.cs
- SimpleTypeResolver.cs
- Vector3DValueSerializer.cs
- WindowsTitleBar.cs
- ISO2022Encoding.cs
- SafeHandles.cs
- WsdlWriter.cs
- QueryCacheEntry.cs
- SourceFileBuildProvider.cs
- XmlAttributeProperties.cs
- KnownAssembliesSet.cs
- SourceFileBuildProvider.cs
- GestureRecognizer.cs
- CapiHashAlgorithm.cs
- DaylightTime.cs
- VideoDrawing.cs
- WebReferencesBuildProvider.cs
- SqlNamer.cs
- CompilerResults.cs
- RuntimeConfigLKG.cs
- PrintController.cs
- IntAverageAggregationOperator.cs
- ipaddressinformationcollection.cs
- PresentationTraceSources.cs
- StylusPointPropertyId.cs
- PrimitiveXmlSerializers.cs
- recordstatescratchpad.cs
- glyphs.cs
- VisualStyleInformation.cs
- SqlClientFactory.cs
- DesignerAutoFormatCollection.cs
- MetadataPropertyAttribute.cs
- XMLSchema.cs
- VersionedStream.cs
- MapPathBasedVirtualPathProvider.cs
- StateDesigner.Layouts.cs
- TreeViewEvent.cs
- DataGridViewControlCollection.cs
- InstanceView.cs
- ControlBuilder.cs
- UTF7Encoding.cs
- SqlInternalConnection.cs
- DBNull.cs
- SchemaNotation.cs
- AttachInfo.cs
- NotSupportedException.cs
- ServicesSection.cs
- WindowsToolbar.cs
- RelationshipWrapper.cs
- GcSettings.cs
- XmlNamespaceMappingCollection.cs
- EdmSchemaError.cs
- TypeConverterHelper.cs
- DataGridRelationshipRow.cs
- InstanceDataCollectionCollection.cs
- RegexGroup.cs
- CompModSwitches.cs
- ComPlusTraceRecord.cs
- ServiceAuthorizationManager.cs
- WebPartMenuStyle.cs
- PartialTrustVisibleAssembliesSection.cs
- ApplicationBuildProvider.cs
- QueryRewriter.cs
- _BaseOverlappedAsyncResult.cs
- DeferredElementTreeState.cs
- OracleString.cs
- SystemException.cs
- RsaKeyGen.cs
- OleDbWrapper.cs
- SelectionChangedEventArgs.cs
- TdsParser.cs
- BinaryWriter.cs
- UnsafeNativeMethods.cs
- cryptoapiTransform.cs
- Application.cs
- AttachedPropertyBrowsableAttribute.cs
- RemoteWebConfigurationHostServer.cs
- DispatcherSynchronizationContext.cs
- ActivationWorker.cs
- SchemaTableColumn.cs
- UnsafeNativeMethods.cs
- LockCookie.cs
- RegisteredExpandoAttribute.cs
- WorkflowApplicationAbortedEventArgs.cs
- HandleCollector.cs