Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Input / Command / InputBinding.cs / 1 / InputBinding.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; // SecurityCritical, TreatAsSafe using System.Security.Permissions; using System.Windows; using System.Windows.Markup; using System.ComponentModel; namespace System.Windows.Input { ////// InputBinding - InputGesture and ICommand combination /// Used to specify the binding between Gesture and Command at Element level. /// public class InputBinding : DependencyObject, ICommandSource { #region Constructor ////// Default Constructor - needed to allow markup creation /// protected InputBinding() { } ////// Constructor /// /// Command /// Input Gesture ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical] public InputBinding(ICommand command, InputGesture gesture) { if (command == null) throw new ArgumentNullException("command"); if (gesture == null) throw new ArgumentNullException("gesture"); // Check before assignment to avoid continuation CheckSecureCommand(command, gesture); _command = command; _gesture = gesture; } #endregion Constructor //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Command Object associated /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return _command; } [SecurityCritical] set { if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(value, _gesture); _command = value; } } } ////// A parameter for the command. /// public object CommandParameter { get { return _commandParameter; } set { lock (_dataLock) { _commandParameter = value; } } } ////// Where the command should be raised. /// public IInputElement CommandTarget { get { return _commandTarget; } set { lock (_dataLock) { _commandTarget = value; } } } ////// InputGesture associated with the Command /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// public virtual InputGesture Gesture { // We would like to make this getter non-virtual but that's not legal // in C#. Luckily there is no security issue with leaving it virtual. get { return _gesture; } [SecurityCritical] set { if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(_command, value); _gesture = value; } } } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- ////// Critical - determines if a command will later make an /// assert. This is critical to be right, because /// later we assume that the binding was protected. /// TreatAsSafe - Demand() is not an unsafe operation /// [SecurityCritical, SecurityTreatAsSafe] void CheckSecureCommand(ICommand command, InputGesture gesture) { ISecureCommand secure = command as ISecureCommand; if (secure != null) { secure.UserInitiatedPermission.Demand(); } } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private ICommand _command = null ; private InputGesture _gesture = null ; private object _commandParameter; private IInputElement _commandTarget; internal static object _dataLock = new object(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Security; // SecurityCritical, TreatAsSafe using System.Security.Permissions; using System.Windows; using System.Windows.Markup; using System.ComponentModel; namespace System.Windows.Input { ////// InputBinding - InputGesture and ICommand combination /// Used to specify the binding between Gesture and Command at Element level. /// public class InputBinding : DependencyObject, ICommandSource { #region Constructor ////// Default Constructor - needed to allow markup creation /// protected InputBinding() { } ////// Constructor /// /// Command /// Input Gesture ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// TreatAsSafe - Calls CheckSecureCommand which does the appropriate demand. /// [SecurityCritical] public InputBinding(ICommand command, InputGesture gesture) { if (command == null) throw new ArgumentNullException("command"); if (gesture == null) throw new ArgumentNullException("gesture"); // Check before assignment to avoid continuation CheckSecureCommand(command, gesture); _command = command; _gesture = gesture; } #endregion Constructor //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Command Object associated /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// [TypeConverter("System.Windows.Input.CommandConverter, PresentationFramework, Version=" + Microsoft.Internal.BuildInfo.WCP_VERSION + ", Culture=neutral, PublicKeyToken=" + Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_TOKEN + ", Custom=null")] [Localizability(LocalizationCategory.NeverLocalize)] // cannot be localized public ICommand Command { get { return _command; } [SecurityCritical] set { if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(value, _gesture); _command = value; } } } ////// A parameter for the command. /// public object CommandParameter { get { return _commandParameter; } set { lock (_dataLock) { _commandParameter = value; } } } ////// Where the command should be raised. /// public IInputElement CommandTarget { get { return _commandTarget; } set { lock (_dataLock) { _commandTarget = value; } } } ////// InputGesture associated with the Command /// ////// Critical - may associate a secure command with a gesture, in /// these cases we need to demand the appropriate permission. /// PublicOk - Calls CheckSecureCommand which does the appropriate demand. /// public virtual InputGesture Gesture { // We would like to make this getter non-virtual but that's not legal // in C#. Luckily there is no security issue with leaving it virtual. get { return _gesture; } [SecurityCritical] set { if (value == null) throw new ArgumentNullException("value"); lock (_dataLock) { // Check before assignment to avoid continuation // CheckSecureCommand(_command, value); _gesture = value; } } } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- ////// Critical - determines if a command will later make an /// assert. This is critical to be right, because /// later we assume that the binding was protected. /// TreatAsSafe - Demand() is not an unsafe operation /// [SecurityCritical, SecurityTreatAsSafe] void CheckSecureCommand(ICommand command, InputGesture gesture) { ISecureCommand secure = command as ISecureCommand; if (secure != null) { secure.UserInitiatedPermission.Demand(); } } //------------------------------------------------------ // // Private Fields // //------------------------------------------------------ #region Private Fields private ICommand _command = null ; private InputGesture _gesture = null ; private object _commandParameter; private IInputElement _commandTarget; internal static object _dataLock = new object(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
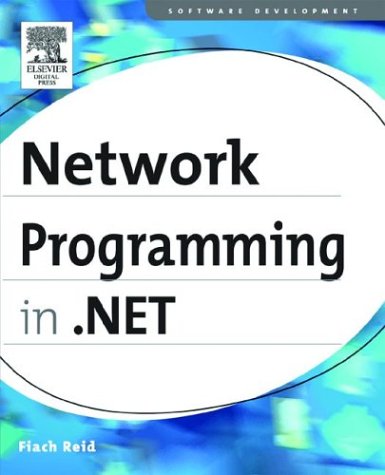
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KnowledgeBase.cs
- RecognizerInfo.cs
- EntityContainer.cs
- DataGridViewButtonColumn.cs
- XmlAnyElementAttribute.cs
- SignatureDescription.cs
- LoadWorkflowByInstanceKeyCommand.cs
- PropertyDescriptorComparer.cs
- ChannelFactory.cs
- smtppermission.cs
- HttpModuleActionCollection.cs
- PointAnimationBase.cs
- BitHelper.cs
- PlacementWorkspace.cs
- VirtualPathData.cs
- CardSpaceShim.cs
- PagePropertiesChangingEventArgs.cs
- Icon.cs
- ThousandthOfEmRealPoints.cs
- BuildResult.cs
- SurrogateSelector.cs
- CopyOfAction.cs
- ProtocolElement.cs
- JoinTreeSlot.cs
- Int16AnimationUsingKeyFrames.cs
- StorageRoot.cs
- AnnotationResourceChangedEventArgs.cs
- TextPattern.cs
- ProjectionPath.cs
- HttpResponse.cs
- PropertyChangedEventManager.cs
- ActivityBindForm.cs
- oledbconnectionstring.cs
- RayHitTestParameters.cs
- TypeLibConverter.cs
- DataGridColumnHeaderCollection.cs
- BindingManagerDataErrorEventArgs.cs
- FtpWebRequest.cs
- ArcSegment.cs
- NGCPageContentCollectionSerializerAsync.cs
- XmlWrappingReader.cs
- ColumnCollection.cs
- ScrollableControl.cs
- DataFormats.cs
- WebPartAddingEventArgs.cs
- BitmapInitialize.cs
- DbParameterCollectionHelper.cs
- ConnectionInterfaceCollection.cs
- GridViewCellAutomationPeer.cs
- HtmlShimManager.cs
- HideDisabledControlAdapter.cs
- MeshGeometry3D.cs
- TransformerTypeCollection.cs
- MD5CryptoServiceProvider.cs
- InsufficientMemoryException.cs
- SubtreeProcessor.cs
- COM2ColorConverter.cs
- DeferredTextReference.cs
- XmlSchemaException.cs
- LinqDataSourceSelectEventArgs.cs
- ExtendedProperty.cs
- ModifierKeysConverter.cs
- VisualProxy.cs
- BasicCommandTreeVisitor.cs
- MenuCommand.cs
- safex509handles.cs
- FlowchartDesigner.xaml.cs
- UnsafeNativeMethodsTablet.cs
- IProvider.cs
- CodeDomLocalizationProvider.cs
- RecognizedPhrase.cs
- InfoCardBaseException.cs
- WmlListAdapter.cs
- ServiceBehaviorAttribute.cs
- DataRowIndexBuffer.cs
- XmlRootAttribute.cs
- DrawingContextDrawingContextWalker.cs
- SuppressMergeCheckAttribute.cs
- CmsUtils.cs
- CngKey.cs
- DynamicResourceExtension.cs
- ConnectorRouter.cs
- ParameterRefs.cs
- SafeProcessHandle.cs
- DataError.cs
- MouseEvent.cs
- SettingsProviderCollection.cs
- __ConsoleStream.cs
- EnumMemberAttribute.cs
- MethodImplAttribute.cs
- Int32RectConverter.cs
- JsonUriDataContract.cs
- IndividualDeviceConfig.cs
- Expressions.cs
- ScrollEvent.cs
- EpmContentSerializerBase.cs
- Int32Animation.cs
- FileClassifier.cs
- ResourceDisplayNameAttribute.cs
- ImplicitInputBrush.cs