Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / JoinTreeSlot.cs / 2 / JoinTreeSlot.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Mapping.ViewGeneration.Utils; namespace System.Data.Mapping.ViewGeneration.Structures { // A wrapper around JoinTreeNode that allows nodes to be stored as // ProjectedSlots internal class JoinTreeSlot : ProjectedSlot { #region Constructor // effects: Creates a projected slot that references the relevant // jointree node (i.e., internal JoinTreeSlot(JoinTreeNode node) { m_node = node; } #endregion #region Fields // A comparer object for comparing jointree slots by MemberPaths internal static readonly IEqualityComparerSpecificEqualityComparer = new JoinTreeSlotEqualityComparer(); private JoinTreeNode m_node; // The node that the var corresponds to #endregion #region Properties // effects: Returns the join tree node corresponding to this variable internal JoinTreeNode JoinTreeNode { get { return m_node; } } // effects: Returns the full metadata path from the root extent to // this node, e.g., Person.Adrs.zip internal MemberPath MemberPath { get { return m_node.MemberPath; } } #endregion #region Methods internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias, int indentLevel) { // Note: One might be tempted to use the Debug.Assert below; but // it is wrong: for query views, path is in SSpace and slotType // is in CSpace since you are going from SSpace to CSpace // Debug.Assert(MemberPath.MemberType.Equals(slotType), "Type mismatch // of expected slot and member slot"); // MemberPath's member type must be compatible with the outputMember Type. // Mapping must have already checked for this and hence we need to assert here. // Today we can't put the debug assert since we require to access internal property // in metadata and entitymapper is using this code, and hence fails to compile. Once // the entitymapper code is fixed to use the API's we can put this assert back // CHANEG_[....]_ // If memberpath's type and the outputmember type do not match, // we have to cast. We assume that the mapping loader has already // checked that the casts are ok and emitted warnings TypeUsage memberPathStoreTypeUsage = Helper.GetModelTypeUsage(MemberPath.LastMember); TypeUsage outputMemberStoreTypeUsage = Helper.GetModelTypeUsage(outputMember.LastMember); if (false == memberPathStoreTypeUsage.EdmType.Equals(outputMemberStoreTypeUsage.EdmType)) { builder.Append("CAST("); MemberPath.AsCql(builder, blockAlias); builder.Append(" AS "); CqlWriter.AppendEscapedTypeName(builder, outputMemberStoreTypeUsage.EdmType); builder.Append(')'); } else { MemberPath.AsCql(builder, blockAlias); } return builder; } // effects: Given a slot and the new mapping, returns the // corresponding new slot internal override ProjectedSlot RemapSlot(Dictionary remap) { JoinTreeNode remappedNode = remap[JoinTreeNode]; return new JoinTreeSlot(remappedNode); } internal override void ToCompactString(StringBuilder builder) { m_node.ToCompactString(builder); } internal string ToUserString() { return m_node.MemberPath.PathToString(false); } protected override bool IsEqualTo(ProjectedSlot right) { JoinTreeSlot rightSlot = right as JoinTreeSlot; if (rightSlot == null) { return false; } // We want equality of the paths return MemberPath.EqualityComparer.Equals(m_node.MemberPath, rightSlot.m_node.MemberPath); } protected override int GetHash() { return MemberPath.EqualityComparer.GetHashCode(m_node.MemberPath); } #endregion #region Helper methods // requires: prefix corresponds to an entity set or an association end // effects: Given the prefix, determines the slots in "slots" // that correspond to the entity key for the entity set or the // association set end. Returns the list of slots. Returns null if // even one of the key slots is not present in slots internal static List GetKeySlots(IEnumerable slots, MemberPath prefix) { // Get the entity type of the hosted end or entity set EntitySet entitySet = prefix.EntitySet; List keys = ExtentKey.GetKeysForEntityType(prefix, entitySet.ElementType); Debug.Assert(keys.Count > 0, "No keys for entity?"); Debug.Assert(keys.Count == 1, "Currently, we only support primary keys"); // Get the slots for the key List keySlots = GetSlots(slots, keys[0].KeyFields); return keySlots; } // effects: Searches for members in slots and returns the // corresponding slots in the same order as present in // members. Returns null if even one member is not present in slots internal static List GetSlots(IEnumerable slots, IEnumerable members) { List result = new List (); foreach (MemberPath member in members) { JoinTreeSlot slot = GetSlotForMember(Helpers.AsSuperTypeList (slots), member); if (slot == null) { return null; } result.Add(slot); } return result; } // effects: Searches for member in slots and returns the corresponding slot // If none is found, returns null internal static JoinTreeSlot GetSlotForMember(IEnumerable slots, MemberPath member) { foreach (JoinTreeSlot slot in slots) { if (MemberPath.EqualityComparer.Equals(slot.MemberPath, member)) { return slot; } } return null; } #endregion #region JoinTreeSlot Comparer classes // summary: Two JoinTreeSlots are equal when their MemberPaths are equal (JoinTreeSlot.IsEqualTo returns true) internal class JoinTreeSlotEqualityComparer : IEqualityComparer { // effects: Returns true if left and right are semantically equivalent public bool Equals(JoinTreeSlot left, JoinTreeSlot right) { return ProjectedSlot.EqualityComparer.Equals(left, right); } public int GetHashCode(JoinTreeSlot key) { return ProjectedSlot.EqualityComparer.GetHashCode(key); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Mapping.ViewGeneration.Utils; namespace System.Data.Mapping.ViewGeneration.Structures { // A wrapper around JoinTreeNode that allows nodes to be stored as // ProjectedSlots internal class JoinTreeSlot : ProjectedSlot { #region Constructor // effects: Creates a projected slot that references the relevant // jointree node (i.e., internal JoinTreeSlot(JoinTreeNode node) { m_node = node; } #endregion #region Fields // A comparer object for comparing jointree slots by MemberPaths internal static readonly IEqualityComparerSpecificEqualityComparer = new JoinTreeSlotEqualityComparer(); private JoinTreeNode m_node; // The node that the var corresponds to #endregion #region Properties // effects: Returns the join tree node corresponding to this variable internal JoinTreeNode JoinTreeNode { get { return m_node; } } // effects: Returns the full metadata path from the root extent to // this node, e.g., Person.Adrs.zip internal MemberPath MemberPath { get { return m_node.MemberPath; } } #endregion #region Methods internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias, int indentLevel) { // Note: One might be tempted to use the Debug.Assert below; but // it is wrong: for query views, path is in SSpace and slotType // is in CSpace since you are going from SSpace to CSpace // Debug.Assert(MemberPath.MemberType.Equals(slotType), "Type mismatch // of expected slot and member slot"); // MemberPath's member type must be compatible with the outputMember Type. // Mapping must have already checked for this and hence we need to assert here. // Today we can't put the debug assert since we require to access internal property // in metadata and entitymapper is using this code, and hence fails to compile. Once // the entitymapper code is fixed to use the API's we can put this assert back // CHANEG_[....]_ // If memberpath's type and the outputmember type do not match, // we have to cast. We assume that the mapping loader has already // checked that the casts are ok and emitted warnings TypeUsage memberPathStoreTypeUsage = Helper.GetModelTypeUsage(MemberPath.LastMember); TypeUsage outputMemberStoreTypeUsage = Helper.GetModelTypeUsage(outputMember.LastMember); if (false == memberPathStoreTypeUsage.EdmType.Equals(outputMemberStoreTypeUsage.EdmType)) { builder.Append("CAST("); MemberPath.AsCql(builder, blockAlias); builder.Append(" AS "); CqlWriter.AppendEscapedTypeName(builder, outputMemberStoreTypeUsage.EdmType); builder.Append(')'); } else { MemberPath.AsCql(builder, blockAlias); } return builder; } // effects: Given a slot and the new mapping, returns the // corresponding new slot internal override ProjectedSlot RemapSlot(Dictionary remap) { JoinTreeNode remappedNode = remap[JoinTreeNode]; return new JoinTreeSlot(remappedNode); } internal override void ToCompactString(StringBuilder builder) { m_node.ToCompactString(builder); } internal string ToUserString() { return m_node.MemberPath.PathToString(false); } protected override bool IsEqualTo(ProjectedSlot right) { JoinTreeSlot rightSlot = right as JoinTreeSlot; if (rightSlot == null) { return false; } // We want equality of the paths return MemberPath.EqualityComparer.Equals(m_node.MemberPath, rightSlot.m_node.MemberPath); } protected override int GetHash() { return MemberPath.EqualityComparer.GetHashCode(m_node.MemberPath); } #endregion #region Helper methods // requires: prefix corresponds to an entity set or an association end // effects: Given the prefix, determines the slots in "slots" // that correspond to the entity key for the entity set or the // association set end. Returns the list of slots. Returns null if // even one of the key slots is not present in slots internal static List GetKeySlots(IEnumerable slots, MemberPath prefix) { // Get the entity type of the hosted end or entity set EntitySet entitySet = prefix.EntitySet; List keys = ExtentKey.GetKeysForEntityType(prefix, entitySet.ElementType); Debug.Assert(keys.Count > 0, "No keys for entity?"); Debug.Assert(keys.Count == 1, "Currently, we only support primary keys"); // Get the slots for the key List keySlots = GetSlots(slots, keys[0].KeyFields); return keySlots; } // effects: Searches for members in slots and returns the // corresponding slots in the same order as present in // members. Returns null if even one member is not present in slots internal static List GetSlots(IEnumerable slots, IEnumerable members) { List result = new List (); foreach (MemberPath member in members) { JoinTreeSlot slot = GetSlotForMember(Helpers.AsSuperTypeList (slots), member); if (slot == null) { return null; } result.Add(slot); } return result; } // effects: Searches for member in slots and returns the corresponding slot // If none is found, returns null internal static JoinTreeSlot GetSlotForMember(IEnumerable slots, MemberPath member) { foreach (JoinTreeSlot slot in slots) { if (MemberPath.EqualityComparer.Equals(slot.MemberPath, member)) { return slot; } } return null; } #endregion #region JoinTreeSlot Comparer classes // summary: Two JoinTreeSlots are equal when their MemberPaths are equal (JoinTreeSlot.IsEqualTo returns true) internal class JoinTreeSlotEqualityComparer : IEqualityComparer { // effects: Returns true if left and right are semantically equivalent public bool Equals(JoinTreeSlot left, JoinTreeSlot right) { return ProjectedSlot.EqualityComparer.Equals(left, right); } public int GetHashCode(JoinTreeSlot key) { return ProjectedSlot.EqualityComparer.GetHashCode(key); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
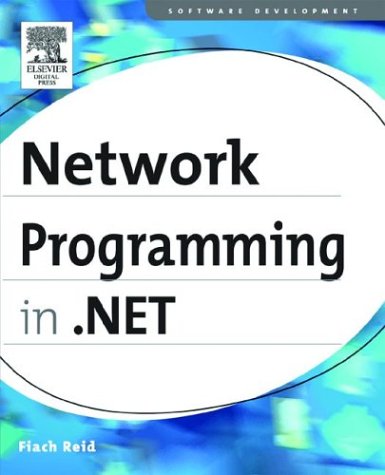
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BoolExpressionVisitors.cs
- ValidationRuleCollection.cs
- BStrWrapper.cs
- Model3DGroup.cs
- HttpVersion.cs
- ProvideValueServiceProvider.cs
- StylusLogic.cs
- MemberAssignment.cs
- GuidTagList.cs
- TableItemStyle.cs
- BindableTemplateBuilder.cs
- CanExecuteRoutedEventArgs.cs
- CounterNameConverter.cs
- PerfCounters.cs
- WindowsFormsHostPropertyMap.cs
- HttpInputStream.cs
- ProfessionalColors.cs
- ListItemParagraph.cs
- TypeInfo.cs
- SHA384.cs
- HuffModule.cs
- AssemblyUtil.cs
- InputBuffer.cs
- RelatedImageListAttribute.cs
- FtpWebRequest.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- BaseResourcesBuildProvider.cs
- BitmapSource.cs
- SqlStatistics.cs
- XmlSerializableServices.cs
- PrefixHandle.cs
- Environment.cs
- documentsequencetextcontainer.cs
- InvokeProviderWrapper.cs
- ColorPalette.cs
- VideoDrawing.cs
- COM2EnumConverter.cs
- Matrix.cs
- DesignerSerializerAttribute.cs
- CustomValidator.cs
- SchemaElement.cs
- Substitution.cs
- ApplicationDirectory.cs
- DataContractSet.cs
- StringExpressionSet.cs
- Registry.cs
- ViewgenGatekeeper.cs
- RangeValidator.cs
- OracleParameterCollection.cs
- Int32AnimationBase.cs
- AuthenticateEventArgs.cs
- BitFlagsGenerator.cs
- infer.cs
- QualificationDataItem.cs
- webeventbuffer.cs
- WinInetCache.cs
- ScriptHandlerFactory.cs
- SystemDiagnosticsSection.cs
- Enlistment.cs
- UnaryQueryOperator.cs
- RegistryPermission.cs
- Section.cs
- Serializer.cs
- WebServicesDescriptionAttribute.cs
- RuntimeConfigLKG.cs
- RenderTargetBitmap.cs
- BooleanFunctions.cs
- PropertyCollection.cs
- KnownBoxes.cs
- ValidationError.cs
- Win32.cs
- CheckableControlBaseAdapter.cs
- AuthenticationSection.cs
- reliableinputsessionchannel.cs
- SecurityContext.cs
- RangeValueProviderWrapper.cs
- ZipIOFileItemStream.cs
- IntegrationExceptionEventArgs.cs
- SubpageParaClient.cs
- ByteViewer.cs
- DictionarySurrogate.cs
- SelectionProcessor.cs
- RequiredFieldValidator.cs
- _ConnectionGroup.cs
- HitTestParameters.cs
- SurrogateChar.cs
- DoWorkEventArgs.cs
- XmlName.cs
- IconBitmapDecoder.cs
- SQLInt16Storage.cs
- SiteMapProvider.cs
- HtmlMeta.cs
- MergablePropertyAttribute.cs
- FacetDescription.cs
- DrawingVisualDrawingContext.cs
- PlanCompilerUtil.cs
- Transactions.cs
- dsa.cs
- RuntimeIdentifierPropertyAttribute.cs
- FontCacheUtil.cs