Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / Binding / EntityChangedParams.cs / 1305376 / EntityChangedParams.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// EntityChangedParams class // // //--------------------------------------------------------------------- namespace System.Data.Services.Client { ///Encapsulates the arguments of EntityChanged delegate [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704", Justification = "Name gets too long with Parameters")] public sealed class EntityChangedParams { #region Fields ///Context associated with the BindingObserver. private readonly DataServiceContext context; ///The entity object that has changed. private readonly object entity; ///The property of the entity that has changed. private readonly string propertyName; ///The current value of the target property. private readonly object propertyValue; ///Entity set to which the entity object belongs private readonly string sourceEntitySet; ///Entity set to which the target propertyValue entity belongs private readonly string targetEntitySet; #endregion #region Constructor ////// Construct an EntityChangedParams object. /// /// Context to which the entity and propertyValue belong. /// The entity object that has changed. /// The property of the target entity object that has changed. /// The current value of the entity property. /// Entity set to which the entity object belongs /// Entity set to which the target propertyValue entity belongs internal EntityChangedParams( DataServiceContext context, object entity, string propertyName, object propertyValue, string sourceEntitySet, string targetEntitySet) { this.context = context; this.entity = entity; this.propertyName = propertyName; this.propertyValue = propertyValue; this.sourceEntitySet = sourceEntitySet; this.targetEntitySet = targetEntitySet; } #endregion #region Properties ///Context associated with the BindingObserver. public DataServiceContext Context { get { return this.context; } } ///The entity object that has changed. public object Entity { get { return this.entity; } } ///The property of the target entity object that has changed. public string PropertyName { get { return this.propertyName; } } ///The current value of the entity property. public object PropertyValue { get { return this.propertyValue; } } ///Entity set to which the entity object belongs public string SourceEntitySet { get { return this.sourceEntitySet; } } ///Entity set to which the target propertyValue entity belongs public string TargetEntitySet { get { return this.targetEntitySet; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// EntityChangedParams class // // //--------------------------------------------------------------------- namespace System.Data.Services.Client { ///Encapsulates the arguments of EntityChanged delegate [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704", Justification = "Name gets too long with Parameters")] public sealed class EntityChangedParams { #region Fields ///Context associated with the BindingObserver. private readonly DataServiceContext context; ///The entity object that has changed. private readonly object entity; ///The property of the entity that has changed. private readonly string propertyName; ///The current value of the target property. private readonly object propertyValue; ///Entity set to which the entity object belongs private readonly string sourceEntitySet; ///Entity set to which the target propertyValue entity belongs private readonly string targetEntitySet; #endregion #region Constructor ////// Construct an EntityChangedParams object. /// /// Context to which the entity and propertyValue belong. /// The entity object that has changed. /// The property of the target entity object that has changed. /// The current value of the entity property. /// Entity set to which the entity object belongs /// Entity set to which the target propertyValue entity belongs internal EntityChangedParams( DataServiceContext context, object entity, string propertyName, object propertyValue, string sourceEntitySet, string targetEntitySet) { this.context = context; this.entity = entity; this.propertyName = propertyName; this.propertyValue = propertyValue; this.sourceEntitySet = sourceEntitySet; this.targetEntitySet = targetEntitySet; } #endregion #region Properties ///Context associated with the BindingObserver. public DataServiceContext Context { get { return this.context; } } ///The entity object that has changed. public object Entity { get { return this.entity; } } ///The property of the target entity object that has changed. public string PropertyName { get { return this.propertyName; } } ///The current value of the entity property. public object PropertyValue { get { return this.propertyValue; } } ///Entity set to which the entity object belongs public string SourceEntitySet { get { return this.sourceEntitySet; } } ///Entity set to which the target propertyValue entity belongs public string TargetEntitySet { get { return this.targetEntitySet; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
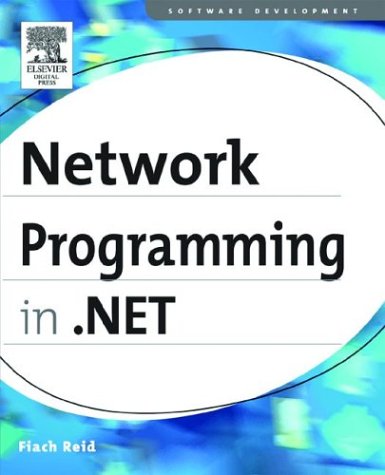
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListItemParagraph.cs
- SliderAutomationPeer.cs
- OleDbPermission.cs
- ResourceExpression.cs
- SoapElementAttribute.cs
- LookupBindingPropertiesAttribute.cs
- BmpBitmapEncoder.cs
- KeyValuePair.cs
- RSAPKCS1SignatureFormatter.cs
- FacetDescription.cs
- MouseGesture.cs
- BypassElementCollection.cs
- SizeAnimation.cs
- HttpResponseInternalBase.cs
- AssertFilter.cs
- XmlEntityReference.cs
- ConfigWriter.cs
- TableCell.cs
- NullableBoolConverter.cs
- DesignerForm.cs
- CodeGenerator.cs
- Binding.cs
- EntitySetBase.cs
- ReturnType.cs
- WebPartTransformerAttribute.cs
- InitializationEventAttribute.cs
- ExpressionConverter.cs
- MulticastNotSupportedException.cs
- DbConnectionPoolGroup.cs
- COM2ColorConverter.cs
- SqlDependencyUtils.cs
- InkCollectionBehavior.cs
- VideoDrawing.cs
- Timeline.cs
- PeerCustomResolverElement.cs
- StdValidatorsAndConverters.cs
- Repeater.cs
- ObjectResult.cs
- RangeValidator.cs
- SmtpCommands.cs
- DataTrigger.cs
- ConnectionPoint.cs
- VariableAction.cs
- ToolStripDropTargetManager.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- HttpMethodAttribute.cs
- DecimalKeyFrameCollection.cs
- WindowProviderWrapper.cs
- HandleRef.cs
- ClrPerspective.cs
- ActivityScheduledQuery.cs
- CodeAttributeDeclaration.cs
- TransportManager.cs
- CompilerInfo.cs
- WindowsTitleBar.cs
- KoreanLunisolarCalendar.cs
- XamlStackWriter.cs
- TextEffectCollection.cs
- BinaryExpression.cs
- UserControl.cs
- Point3DValueSerializer.cs
- EntityModelBuildProvider.cs
- EntityExpressionVisitor.cs
- NonVisualControlAttribute.cs
- WinHttpWebProxyFinder.cs
- BrowserTree.cs
- ActivityExecutionFilter.cs
- FactoryGenerator.cs
- CacheVirtualItemsEvent.cs
- ServiceAuthorizationManager.cs
- COMException.cs
- BridgeDataReader.cs
- RuntimeComponentFilter.cs
- DesignerActionVerbItem.cs
- SubMenuStyle.cs
- ClassGenerator.cs
- BinaryObjectReader.cs
- GeneralTransform.cs
- Formatter.cs
- DataGridViewIntLinkedList.cs
- TouchEventArgs.cs
- Matrix3DConverter.cs
- OperatingSystemVersionCheck.cs
- DataViewManagerListItemTypeDescriptor.cs
- TimeSpanOrInfiniteValidator.cs
- ClientApiGenerator.cs
- LineServicesRun.cs
- EntityDataSourceDesignerHelper.cs
- NodeLabelEditEvent.cs
- SystemIPv6InterfaceProperties.cs
- odbcmetadatacolumnnames.cs
- IIS7UserPrincipal.cs
- PrinterSettings.cs
- IfAction.cs
- Base64Decoder.cs
- PersistenceTypeAttribute.cs
- AVElementHelper.cs
- DoubleConverter.cs
- InputLangChangeRequestEvent.cs
- Membership.cs