Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / IO / FileNotFoundException.cs / 1 / FileNotFoundException.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FileNotFoundException ** ** ** Purpose: Exception for accessing a file that doesn't exist. ** ** ===========================================================*/ using System; using System.Runtime.Serialization; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; using System.Globalization; namespace System.IO { // Thrown when trying to access a file that doesn't exist on disk. [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class FileNotFoundException : IOException { private String _fileName; // The name of the file that isn't found. private String _fusionLog; // fusion log (when applicable) public FileNotFoundException() : base(Environment.GetResourceString("IO.FileNotFound")) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); } public FileNotFoundException(String message) : base(message) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); } public FileNotFoundException(String message, Exception innerException) : base(message, innerException) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); } public FileNotFoundException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); _fileName = fileName; } public FileNotFoundException(String message, String fileName, Exception innerException) : base(message, innerException) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) { if ((_fileName == null) && (HResult == System.__HResults.COR_E_EXCEPTION)) _message = Environment.GetResourceString("IO.FileNotFound"); else if( _fileName != null) _message = FileLoadException.FormatFileLoadExceptionMessage(_fileName, HResult); } } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected FileNotFoundException(SerializationInfo info, StreamingContext context) : base (info, context) { // Base class constructor will check info != null. _fileName = info.GetString("FileNotFound_FileName"); try { _fusionLog = info.GetString("FileNotFound_FusionLog"); } catch { _fusionLog = null; } } private FileNotFoundException(String fileName, String fusionLog,int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("FileNotFound_FileName", _fileName, typeof(String)); try { info.AddValue("FileNotFound_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: FileNotFoundException ** ** ** Purpose: Exception for accessing a file that doesn't exist. ** ** ===========================================================*/ using System; using System.Runtime.Serialization; using System.Security.Permissions; using SecurityException = System.Security.SecurityException; using System.Globalization; namespace System.IO { // Thrown when trying to access a file that doesn't exist on disk. [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public class FileNotFoundException : IOException { private String _fileName; // The name of the file that isn't found. private String _fusionLog; // fusion log (when applicable) public FileNotFoundException() : base(Environment.GetResourceString("IO.FileNotFound")) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); } public FileNotFoundException(String message) : base(message) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); } public FileNotFoundException(String message, Exception innerException) : base(message, innerException) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); } public FileNotFoundException(String message, String fileName) : base(message) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); _fileName = fileName; } public FileNotFoundException(String message, String fileName, Exception innerException) : base(message, innerException) { SetErrorCode(__HResults.COR_E_FILENOTFOUND); _fileName = fileName; } public override String Message { get { SetMessageField(); return _message; } } private void SetMessageField() { if (_message == null) { if ((_fileName == null) && (HResult == System.__HResults.COR_E_EXCEPTION)) _message = Environment.GetResourceString("IO.FileNotFound"); else if( _fileName != null) _message = FileLoadException.FormatFileLoadExceptionMessage(_fileName, HResult); } } public String FileName { get { return _fileName; } } public override String ToString() { String s = GetType().FullName + ": " + Message; if (_fileName != null && _fileName.Length != 0) s += Environment.NewLine + String.Format(CultureInfo.CurrentCulture, Environment.GetResourceString("IO.FileName_Name"), _fileName); if (InnerException != null) s = s + " ---> " + InnerException.ToString(); if (StackTrace != null) s += Environment.NewLine + StackTrace; try { if(FusionLog!=null) { if (s==null) s=" "; s+=Environment.NewLine; s+=Environment.NewLine; s+=FusionLog; } } catch(SecurityException) { } return s; } protected FileNotFoundException(SerializationInfo info, StreamingContext context) : base (info, context) { // Base class constructor will check info != null. _fileName = info.GetString("FileNotFound_FileName"); try { _fusionLog = info.GetString("FileNotFound_FusionLog"); } catch { _fusionLog = null; } } private FileNotFoundException(String fileName, String fusionLog,int hResult) : base(null) { SetErrorCode(hResult); _fileName = fileName; _fusionLog=fusionLog; SetMessageField(); } public String FusionLog { [SecurityPermissionAttribute( SecurityAction.Demand, Flags = SecurityPermissionFlag.ControlEvidence | SecurityPermissionFlag.ControlPolicy)] get { return _fusionLog; } } [SecurityPermissionAttribute(SecurityAction.LinkDemand, Flags=SecurityPermissionFlag.SerializationFormatter)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { // Serialize data for our base classes. base will verify info != null. base.GetObjectData(info, context); // Serialize data for this class info.AddValue("FileNotFound_FileName", _fileName, typeof(String)); try { info.AddValue("FileNotFound_FusionLog", FusionLog, typeof(String)); } catch (SecurityException) { } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
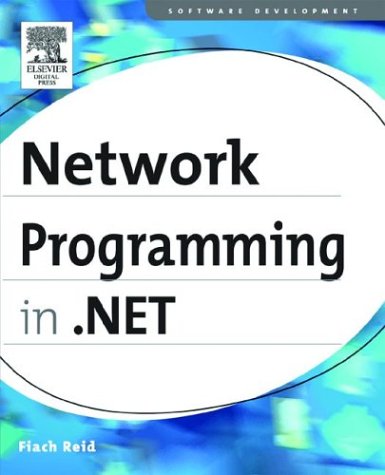
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextElementEnumerator.cs
- PageContent.cs
- ReachIDocumentPaginatorSerializer.cs
- MethodCallConverter.cs
- IPAddress.cs
- OracleTimeSpan.cs
- ListSortDescriptionCollection.cs
- SelectionGlyph.cs
- ColorDialog.cs
- VScrollBar.cs
- SafeFileHandle.cs
- CommandConverter.cs
- SettingsPropertyIsReadOnlyException.cs
- PermissionSet.cs
- Grant.cs
- SQLRoleProvider.cs
- MultipartIdentifier.cs
- VectorCollection.cs
- Decimal.cs
- ChannelBinding.cs
- MenuRenderer.cs
- SafeFindHandle.cs
- HierarchicalDataBoundControlAdapter.cs
- GC.cs
- TransformerInfo.cs
- LazyTextWriterCreator.cs
- AbstractExpressions.cs
- XPathDescendantIterator.cs
- AssemblySettingAttributes.cs
- SessionState.cs
- TcpClientChannel.cs
- CollectionBuilder.cs
- UncommonField.cs
- CollectionDataContractAttribute.cs
- EmptyControlCollection.cs
- Formatter.cs
- MembershipSection.cs
- MultipleViewPattern.cs
- WebControlsSection.cs
- InvalidAsynchronousStateException.cs
- EndOfStreamException.cs
- BrushValueSerializer.cs
- TextTreePropertyUndoUnit.cs
- BitmapEffectDrawing.cs
- DataGridViewAdvancedBorderStyle.cs
- TextTreeRootTextBlock.cs
- RenderData.cs
- MetadataProperty.cs
- PositiveTimeSpanValidator.cs
- TdsEnums.cs
- CancellationState.cs
- CLSCompliantAttribute.cs
- OleDbError.cs
- GorillaCodec.cs
- WsatProxy.cs
- util.cs
- ByteAnimationBase.cs
- EventRecordWrittenEventArgs.cs
- FlowDocumentFormatter.cs
- TextBreakpoint.cs
- versioninfo.cs
- NominalTypeEliminator.cs
- HtmlContainerControl.cs
- XmlSchemaIdentityConstraint.cs
- objectquery_tresulttype.cs
- RadioButtonBaseAdapter.cs
- StrongNameUtility.cs
- SimpleBitVector32.cs
- NetStream.cs
- DispatchChannelSink.cs
- KeyValueConfigurationCollection.cs
- FaultCode.cs
- PagesChangedEventArgs.cs
- SiteMap.cs
- SchemaManager.cs
- CommonProperties.cs
- PhysicalOps.cs
- DTCTransactionManager.cs
- _ContextAwareResult.cs
- DateTimeHelper.cs
- HopperCache.cs
- Tool.cs
- CLRBindingWorker.cs
- DateTimePicker.cs
- ImageSourceValueSerializer.cs
- ExpressionBuilderCollection.cs
- HostExecutionContextManager.cs
- WebPartDeleteVerb.cs
- ContentOnlyMessage.cs
- ConnectionManager.cs
- Types.cs
- ClipboardData.cs
- QilStrConcatenator.cs
- Popup.cs
- XmlSchemaFacet.cs
- TreeNodeConverter.cs
- ValidationErrorCollection.cs
- PrintEvent.cs
- StrokeDescriptor.cs
- CustomTypeDescriptor.cs