Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / uribuilder.cs / 1 / uribuilder.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System { using System.Net; using System.Text; using System.Globalization; using System.Threading; ////// public class UriBuilder { // fields private bool m_changed = true; private string m_fragment = String.Empty; private string m_host = "localhost"; private string m_password = String.Empty; private string m_path = "/"; private int m_port = -1; private string m_query = String.Empty; private string m_scheme = "http"; private string m_schemeDelimiter = Uri.SchemeDelimiter; private Uri m_uri; private string m_username = String.Empty; // constructors ///[To be supplied.] ////// public UriBuilder() { } ///[To be supplied.] ////// public UriBuilder(string uri) { // setting allowRelative=true for a string like www.acme.org Uri tryUri = new Uri(uri, UriKind.RelativeOrAbsolute); if (tryUri.IsAbsoluteUri) { Init(tryUri); } else { uri = Uri.UriSchemeHttp + Uri.SchemeDelimiter + uri; Init(new Uri(uri)); } } ///[To be supplied.] ////// public UriBuilder(Uri uri) { Init(uri); } private void Init(Uri uri) { m_fragment = uri.Fragment; m_query = uri.Query; m_host = uri.Host; m_path = uri.AbsolutePath; m_port = uri.Port; m_scheme = uri.Scheme; m_schemeDelimiter = uri.HasAuthority? Uri.SchemeDelimiter: ":"; string userInfo = uri.UserInfo; if (!ValidationHelper.IsBlankString(userInfo)) { int index = userInfo.IndexOf(':'); if (index != -1) { m_password = userInfo.Substring(index + 1); m_username = userInfo.Substring(0, index); } else { m_username = userInfo; } } SetFieldsFromUri(uri); } ///[To be supplied.] ////// public UriBuilder(string schemeName, string hostName) { Scheme = schemeName; Host = hostName; } ///[To be supplied.] ////// public UriBuilder(string scheme, string host, int portNumber) : this(scheme, host) { Port = portNumber; } ///[To be supplied.] ////// public UriBuilder(string scheme, string host, int port, string pathValue ) : this(scheme, host, port) { Path = pathValue; } ///[To be supplied.] ////// public UriBuilder(string scheme, string host, int port, string path, string extraValue ) : this(scheme, host, port, path) { try { Extra = extraValue; } catch (Exception exception) { if (exception is ThreadAbortException || exception is StackOverflowException || exception is OutOfMemoryException) { throw; } throw new ArgumentException("extraValue"); } } // properties private string Extra { set { if (value == null) { value = String.Empty; } if (value.Length > 0) { if (value[0] == '#') { Fragment = value.Substring(1); } else if (value[0] == '?') { int end = value.IndexOf('#'); if (end == -1) { end = value.Length; } else { Fragment = value.Substring(end+1); } Query = value.Substring(1, end-1); } else { throw new ArgumentException("value"); } } else { Fragment = String.Empty; Query = String.Empty; } } } ///[To be supplied.] ////// public string Fragment { get { return m_fragment; } set { if (value == null) { value = String.Empty; } if (value.Length > 0) { value = '#' + value; } m_fragment = value; m_changed = true; } } ///[To be supplied.] ////// public string Host { get { return m_host; } set { if (value == null) { value = String.Empty; } m_host = value; //probable ipv6 address - if (m_host.IndexOf(':') >= 0) { //set brackets if (m_host[0] != '[') m_host = "[" + m_host + "]"; } m_changed = true; } } ///[To be supplied.] ////// public string Password { get { return m_password; } set { if (value == null) { value = String.Empty; } m_password = value; } } ///[To be supplied.] ////// public string Path { get { return m_path; } set { if ((value == null) || (value.Length == 0)) { value = "/"; } //if ((value[0] != '/') && (value[0] != '\\')) { // value = '/' + value; //} m_path = Uri.InternalEscapeString(ConvertSlashes(value)); m_changed = true; } } ///[To be supplied.] ////// public int Port { get { return m_port; } set { if (value < -1 || value > 0xFFFF) { throw new ArgumentOutOfRangeException("value"); } m_port = value; m_changed = true; } } ///[To be supplied.] ////// public string Query { get { return m_query; } set { if (value == null) { value = String.Empty; } if (value.Length > 0) { value = '?' + value; } m_query = value; m_changed = true; } } ///[To be supplied.] ////// public string Scheme { get { return m_scheme; } set { if (value == null) { value = String.Empty; } int index = value.IndexOf(':'); if (index != -1) { value = value.Substring(0, index); } if (value.Length != 0) { if (!Uri.CheckSchemeName(value)) { throw new ArgumentException("value"); } value = value.ToLower(CultureInfo.InvariantCulture); } m_scheme = value; m_changed = true; } } ///[To be supplied.] ////// public Uri Uri { get { if (m_changed) { m_uri = new Uri(ToString()); SetFieldsFromUri(m_uri); m_changed = false; } return m_uri; } } ///[To be supplied.] ////// public string UserName { get { return m_username; } set { if (value == null) { value = String.Empty; } m_username = value; } } // methods private string ConvertSlashes(string path) { StringBuilder sb = new StringBuilder(path.Length); char ch; for (int i = 0; i < path.Length; ++i) { ch = path[i]; if (ch == '\\') { ch = '/'; } sb.Append(ch); } return sb.ToString(); } ///[To be supplied.] ////// public override bool Equals(object rparam) { if (rparam == null) { return false; } return Uri.Equals(rparam.ToString()); } ///[To be supplied.] ////// public override int GetHashCode() { return Uri.GetHashCode(); } private void SetFieldsFromUri(Uri uri) { m_fragment = uri.Fragment; m_query = uri.Query; m_host = uri.Host; m_path = uri.AbsolutePath; m_port = uri.Port; m_scheme = uri.Scheme; m_schemeDelimiter = uri.HasAuthority? Uri.SchemeDelimiter: ":"; string userInfo = uri.UserInfo; if (userInfo.Length > 0) { int index = userInfo.IndexOf(':'); if (index != -1) { m_password = userInfo.Substring(index + 1); m_username = userInfo.Substring(0, index); } else { m_username = userInfo; } } } ///[To be supplied.] ////// public override string ToString() { if (m_username.Length == 0 && m_password.Length > 0) { throw new UriFormatException(SR.GetString(SR.net_uri_BadUserPassword)); } if (m_scheme.Length != 0) { UriParser syntax = UriParser.GetSyntax(m_scheme); if (syntax != null) m_schemeDelimiter = syntax.InFact(UriSyntaxFlags.MustHaveAuthority) || (m_host.Length != 0 && syntax.NotAny(UriSyntaxFlags.MailToLikeUri) && syntax.InFact(UriSyntaxFlags.OptionalAuthority )) ? Uri.SchemeDelimiter : ":"; else m_schemeDelimiter = m_host.Length != 0? Uri.SchemeDelimiter: ":"; } string result = m_scheme.Length != 0? (m_scheme + m_schemeDelimiter): string.Empty; return result + m_username + ((m_password.Length > 0) ? (":" + m_password) : String.Empty) + ((m_username.Length > 0) ? "@" : String.Empty) + m_host + (((m_port != -1) && (m_host.Length > 0)) ? (":" + m_port) : String.Empty) + (((m_host.Length > 0) && (m_path.Length != 0) && (m_path[0] != '/')) ? "/" : String.Empty) + m_path + m_query + m_fragment; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System { using System.Net; using System.Text; using System.Globalization; using System.Threading; ////// public class UriBuilder { // fields private bool m_changed = true; private string m_fragment = String.Empty; private string m_host = "localhost"; private string m_password = String.Empty; private string m_path = "/"; private int m_port = -1; private string m_query = String.Empty; private string m_scheme = "http"; private string m_schemeDelimiter = Uri.SchemeDelimiter; private Uri m_uri; private string m_username = String.Empty; // constructors ///[To be supplied.] ////// public UriBuilder() { } ///[To be supplied.] ////// public UriBuilder(string uri) { // setting allowRelative=true for a string like www.acme.org Uri tryUri = new Uri(uri, UriKind.RelativeOrAbsolute); if (tryUri.IsAbsoluteUri) { Init(tryUri); } else { uri = Uri.UriSchemeHttp + Uri.SchemeDelimiter + uri; Init(new Uri(uri)); } } ///[To be supplied.] ////// public UriBuilder(Uri uri) { Init(uri); } private void Init(Uri uri) { m_fragment = uri.Fragment; m_query = uri.Query; m_host = uri.Host; m_path = uri.AbsolutePath; m_port = uri.Port; m_scheme = uri.Scheme; m_schemeDelimiter = uri.HasAuthority? Uri.SchemeDelimiter: ":"; string userInfo = uri.UserInfo; if (!ValidationHelper.IsBlankString(userInfo)) { int index = userInfo.IndexOf(':'); if (index != -1) { m_password = userInfo.Substring(index + 1); m_username = userInfo.Substring(0, index); } else { m_username = userInfo; } } SetFieldsFromUri(uri); } ///[To be supplied.] ////// public UriBuilder(string schemeName, string hostName) { Scheme = schemeName; Host = hostName; } ///[To be supplied.] ////// public UriBuilder(string scheme, string host, int portNumber) : this(scheme, host) { Port = portNumber; } ///[To be supplied.] ////// public UriBuilder(string scheme, string host, int port, string pathValue ) : this(scheme, host, port) { Path = pathValue; } ///[To be supplied.] ////// public UriBuilder(string scheme, string host, int port, string path, string extraValue ) : this(scheme, host, port, path) { try { Extra = extraValue; } catch (Exception exception) { if (exception is ThreadAbortException || exception is StackOverflowException || exception is OutOfMemoryException) { throw; } throw new ArgumentException("extraValue"); } } // properties private string Extra { set { if (value == null) { value = String.Empty; } if (value.Length > 0) { if (value[0] == '#') { Fragment = value.Substring(1); } else if (value[0] == '?') { int end = value.IndexOf('#'); if (end == -1) { end = value.Length; } else { Fragment = value.Substring(end+1); } Query = value.Substring(1, end-1); } else { throw new ArgumentException("value"); } } else { Fragment = String.Empty; Query = String.Empty; } } } ///[To be supplied.] ////// public string Fragment { get { return m_fragment; } set { if (value == null) { value = String.Empty; } if (value.Length > 0) { value = '#' + value; } m_fragment = value; m_changed = true; } } ///[To be supplied.] ////// public string Host { get { return m_host; } set { if (value == null) { value = String.Empty; } m_host = value; //probable ipv6 address - if (m_host.IndexOf(':') >= 0) { //set brackets if (m_host[0] != '[') m_host = "[" + m_host + "]"; } m_changed = true; } } ///[To be supplied.] ////// public string Password { get { return m_password; } set { if (value == null) { value = String.Empty; } m_password = value; } } ///[To be supplied.] ////// public string Path { get { return m_path; } set { if ((value == null) || (value.Length == 0)) { value = "/"; } //if ((value[0] != '/') && (value[0] != '\\')) { // value = '/' + value; //} m_path = Uri.InternalEscapeString(ConvertSlashes(value)); m_changed = true; } } ///[To be supplied.] ////// public int Port { get { return m_port; } set { if (value < -1 || value > 0xFFFF) { throw new ArgumentOutOfRangeException("value"); } m_port = value; m_changed = true; } } ///[To be supplied.] ////// public string Query { get { return m_query; } set { if (value == null) { value = String.Empty; } if (value.Length > 0) { value = '?' + value; } m_query = value; m_changed = true; } } ///[To be supplied.] ////// public string Scheme { get { return m_scheme; } set { if (value == null) { value = String.Empty; } int index = value.IndexOf(':'); if (index != -1) { value = value.Substring(0, index); } if (value.Length != 0) { if (!Uri.CheckSchemeName(value)) { throw new ArgumentException("value"); } value = value.ToLower(CultureInfo.InvariantCulture); } m_scheme = value; m_changed = true; } } ///[To be supplied.] ////// public Uri Uri { get { if (m_changed) { m_uri = new Uri(ToString()); SetFieldsFromUri(m_uri); m_changed = false; } return m_uri; } } ///[To be supplied.] ////// public string UserName { get { return m_username; } set { if (value == null) { value = String.Empty; } m_username = value; } } // methods private string ConvertSlashes(string path) { StringBuilder sb = new StringBuilder(path.Length); char ch; for (int i = 0; i < path.Length; ++i) { ch = path[i]; if (ch == '\\') { ch = '/'; } sb.Append(ch); } return sb.ToString(); } ///[To be supplied.] ////// public override bool Equals(object rparam) { if (rparam == null) { return false; } return Uri.Equals(rparam.ToString()); } ///[To be supplied.] ////// public override int GetHashCode() { return Uri.GetHashCode(); } private void SetFieldsFromUri(Uri uri) { m_fragment = uri.Fragment; m_query = uri.Query; m_host = uri.Host; m_path = uri.AbsolutePath; m_port = uri.Port; m_scheme = uri.Scheme; m_schemeDelimiter = uri.HasAuthority? Uri.SchemeDelimiter: ":"; string userInfo = uri.UserInfo; if (userInfo.Length > 0) { int index = userInfo.IndexOf(':'); if (index != -1) { m_password = userInfo.Substring(index + 1); m_username = userInfo.Substring(0, index); } else { m_username = userInfo; } } } ///[To be supplied.] ////// public override string ToString() { if (m_username.Length == 0 && m_password.Length > 0) { throw new UriFormatException(SR.GetString(SR.net_uri_BadUserPassword)); } if (m_scheme.Length != 0) { UriParser syntax = UriParser.GetSyntax(m_scheme); if (syntax != null) m_schemeDelimiter = syntax.InFact(UriSyntaxFlags.MustHaveAuthority) || (m_host.Length != 0 && syntax.NotAny(UriSyntaxFlags.MailToLikeUri) && syntax.InFact(UriSyntaxFlags.OptionalAuthority )) ? Uri.SchemeDelimiter : ":"; else m_schemeDelimiter = m_host.Length != 0? Uri.SchemeDelimiter: ":"; } string result = m_scheme.Length != 0? (m_scheme + m_schemeDelimiter): string.Empty; return result + m_username + ((m_password.Length > 0) ? (":" + m_password) : String.Empty) + ((m_username.Length > 0) ? "@" : String.Empty) + m_host + (((m_port != -1) && (m_host.Length > 0)) ? (":" + m_port) : String.Empty) + (((m_host.Length > 0) && (m_path.Length != 0) && (m_path[0] != '/')) ? "/" : String.Empty) + m_path + m_query + m_fragment; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
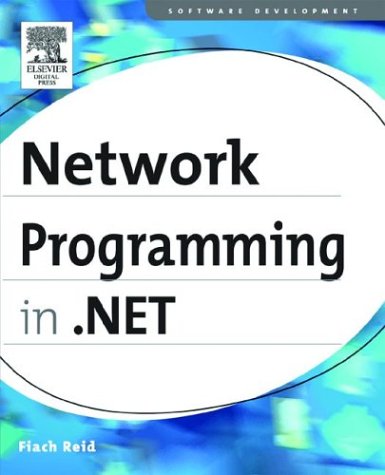
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListDesigner.cs
- TextSelectionHelper.cs
- RetrieveVirtualItemEventArgs.cs
- DesignTimeParseData.cs
- RawMouseInputReport.cs
- EdmRelationshipRoleAttribute.cs
- HwndProxyElementProvider.cs
- JournalEntry.cs
- Ref.cs
- SolidColorBrush.cs
- InvalidPropValue.cs
- _IPv4Address.cs
- ToolStripScrollButton.cs
- HtmlButton.cs
- EventLogEntryCollection.cs
- AtomServiceDocumentSerializer.cs
- RestClientProxyHandler.cs
- CodeVariableReferenceExpression.cs
- TreeNodeBindingCollection.cs
- WindowsFormsEditorServiceHelper.cs
- Hash.cs
- MetadataPropertyvalue.cs
- StyleSelector.cs
- Context.cs
- securitycriticaldata.cs
- URIFormatException.cs
- GridViewEditEventArgs.cs
- XmlEnumAttribute.cs
- ConstantExpression.cs
- InvalidOleVariantTypeException.cs
- SortQueryOperator.cs
- ImageBrush.cs
- _UncName.cs
- Touch.cs
- CodeRegionDirective.cs
- SmtpLoginAuthenticationModule.cs
- HttpBrowserCapabilitiesWrapper.cs
- EventToken.cs
- DataGridViewColumnConverter.cs
- XamlWriter.cs
- ChannelServices.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- ListItemsPage.cs
- DefaultExpression.cs
- SyndicationSerializer.cs
- SmtpFailedRecipientException.cs
- PreloadedPackages.cs
- DrawTreeNodeEventArgs.cs
- xml.cs
- GridViewCancelEditEventArgs.cs
- TextMetrics.cs
- TransportContext.cs
- SqlRowUpdatedEvent.cs
- TypeExtensions.cs
- WinFormsUtils.cs
- AssemblyAttributes.cs
- DataBoundLiteralControl.cs
- Subordinate.cs
- XsltLibrary.cs
- BamlStream.cs
- SyndicationSerializer.cs
- CacheVirtualItemsEvent.cs
- BitmapCodecInfo.cs
- Ticks.cs
- DataRecordInternal.cs
- ToolBarButton.cs
- ConstraintStruct.cs
- DefaultValueMapping.cs
- SqlCacheDependencySection.cs
- XmlArrayItemAttributes.cs
- PageRanges.cs
- WebSysDisplayNameAttribute.cs
- TreeNode.cs
- Bold.cs
- HitTestResult.cs
- XhtmlBasicLinkAdapter.cs
- XmlValidatingReader.cs
- SystemUnicastIPAddressInformation.cs
- XamlTemplateSerializer.cs
- WmpBitmapEncoder.cs
- LocalizedNameDescriptionPair.cs
- FullTrustAssembliesSection.cs
- SiteMapProvider.cs
- ConfigurationPermission.cs
- SparseMemoryStream.cs
- ContextMenuStripGroup.cs
- LocationReferenceValue.cs
- ErrorActivity.cs
- BufferAllocator.cs
- CustomAttribute.cs
- OletxTransactionManager.cs
- ObjectListDataBindEventArgs.cs
- XmlCountingReader.cs
- FilterException.cs
- BulletChrome.cs
- ProgressiveCrcCalculatingStream.cs
- CapabilitiesPattern.cs
- GraphicsContext.cs
- JobInputBins.cs
- SqlBinder.cs