Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachFixedPageSerializer.cs / 2 / ReachFixedPageSerializer.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachFixedPageSerializer.cs Abstract: This file contains the definition of a class that defines the common functionality required to serialize a FixedPage Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Printing; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { ////// Class defining common functionality required to /// serialize a FixedPage. /// internal class FixedPageSerializer : ReachSerializer { #region Constructor ////// Constructor for class FixedPageSerializer /// /// /// The serialization manager, the services of which are /// used later in the serialization process of the type. /// public FixedPageSerializer( PackageSerializationManager manager ): base(manager) { } #endregion Constructor #region Public Methods ////// The main method that is called to serialize a FixedPage. /// /// /// Instance of object to be serialized. /// public override void SerializeObject( Object serializedObject ) { // // Create the ImageTable required by the Type Converters // The Image table at this time is shared / currentPage // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageImageTable = new Dictionary(); // // Create the ColorContextTable required by the Type Converters // The Image table at this time is shared / currentPage // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageColorContextTable = new Dictionary (); // // Create the ResourceDictionaryTable required by the Type Converters // The Image table at this time is shared / currentPage // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageResourceDictionaryTable = new Dictionary (); base.SerializeObject(serializedObject); } #endregion Public Methods #region Internal Methods /// /// The main method that is called to serialize the FixedPage /// and that is usually called from within the serialization manager /// when a node in the graph of objects is at a turn where it should /// be serialized. /// /// /// The context of the property being serialized at this time and /// it points internally to the object encapsulated by that node. /// internal override void SerializeObject( SerializablePropertyContext serializedProperty ) { // // Create the ImageTable required by the Type Converters // The Image table at this time is shared / currentPage // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageImageTable = new Dictionary(); // // Create the ColorContextTable required by the Type Converters // The Image table at this time is shared / currentPage // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageColorContextTable = new Dictionary (); // // Create the ResourceDictionaryTable required by the Type Converters // The Image table at this time is shared / currentPage // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageResourceDictionaryTable = new Dictionary (); base.SerializeObject(serializedProperty); } /// /// The method is called once the object data is discovered at that /// point of the serialization process. /// /// /// The context of the object to be serialized at this time. /// internal override void PersistObjectData( SerializableObjectContext serializableObjectContext ) { Toolbox.StartEvent(MS.Utility.EventTraceGuidId.DRXSERIALIZATIONGUID); if(serializableObjectContext == null) { throw new ArgumentNullException("serializableObjectContext"); } if( SerializationManager is XpsSerializationManager) { (SerializationManager as XpsSerializationManager).RegisterPageStart(); } FixedPage fixedPage = serializableObjectContext.TargetObject as FixedPage; ReachTreeWalker treeWalker = new ReachTreeWalker(this); treeWalker.SerializeLinksInFixedPage((FixedPage)serializableObjectContext.TargetObject); String xmlnsForType = SerializationManager.GetXmlNSForType(serializableObjectContext.TargetObject.GetType()); if(xmlnsForType == null) { XmlWriter.WriteStartElement(serializableObjectContext.Name); } else { XmlWriter.WriteStartElement(serializableObjectContext.Name); XmlWriter.WriteAttributeString(XpsS0Markup.Xmlns, xmlnsForType); XmlWriter.WriteAttributeString(XpsS0Markup.XmlnsX, XpsS0Markup.XmlnsXSchema); XmlLanguage language = fixedPage.Language; if (language == null) { //If the language property is null, assign the language to the default language = XmlLanguage.GetLanguage(XpsS0Markup.XmlLangValue); } SerializationManager.Language = language; XmlWriter.WriteAttributeString(XpsS0Markup.XmlLang, language.ToString()); } { Size fixedPageSize = new Size(fixedPage.Width, fixedPage.Height); ((XpsSerializationManager)SerializationManager).FixedPageSize = fixedPageSize; // // Before we serialize any properties on the FixedPage, we need to // serialize the FixedPage as a Visual // Visual fixedPageAsVisual = serializableObjectContext.TargetObject as Visual; bool needEndVisual = false; if(fixedPageAsVisual != null) { needEndVisual = SerializePageAsVisual(fixedPageAsVisual); } if (serializableObjectContext.IsComplexValue) { PrintTicket printTicket = ((XpsSerializationManager)SerializationManager).FixedPagePrintTicket; if(printTicket != null) { PrintTicketSerializer serializer = new PrintTicketSerializer(SerializationManager); serializer.SerializeObject(printTicket); ((XpsSerializationManager)SerializationManager).FixedPagePrintTicket = null; } SerializeObjectCore(serializableObjectContext); } else { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_WrongPropertyTypeForFixedPage)); } if(needEndVisual) { XmlWriter pageWriter = ((XpsSerializationManager)SerializationManager). PackagingPolicy.AcquireXmlWriterForPage(); XmlWriter resWriter = ((XpsSerializationManager)SerializationManager). PackagingPolicy.AcquireXmlWriterForResourceDictionary(); VisualTreeFlattener flattener = ((XpsSerializationManager)SerializationManager). VisualSerializationService.AcquireVisualTreeFlattener(resWriter, pageWriter, fixedPageSize); flattener.EndVisual(); } } ((XpsSerializationManager)SerializationManager).PackagingPolicy.PreCommitCurrentPage(); // // Copy hyperlinks into stream // treeWalker.CommitHyperlinks(); XmlWriter.WriteEndElement(); // //Release used resources // XmlWriter = null; // // Free the image table in use for this page // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageImageTable = null; // // Free the colorContext table in use for this page // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageColorContextTable = null; // // Free the resourceDictionary table in use for this page // ((XpsSerializationManager)SerializationManager).ResourcePolicy.CurrentPageResourceDictionaryTable = null; ((XpsSerializationManager)SerializationManager).VisualSerializationService.ReleaseVisualTreeFlattener(); if( SerializationManager is XpsSerializationManager) { (SerializationManager as XpsSerializationManager).RegisterPageEnd(); } // // Signal to any registered callers that the Page has been serialized // XpsSerializationProgressChangedEventArgs progressEvent = new XpsSerializationProgressChangedEventArgs(XpsWritingProgressChangeLevel.FixedPageWritingProgress, 0, 0, null); ((XpsSerializationManager)SerializationManager).OnXPSSerializationProgressChanged(progressEvent); Toolbox.EndEvent(MS.Utility.EventTraceGuidId.DRXSERIALIZATIONGUID); } ////// This method is the one that writes out the attribute within /// the xml stream when serializing simple properties. /// /// /// The property that is to be serialized as an attribute at this time. /// internal override void WriteSerializedAttribute( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } String attributeValue = String.Empty; attributeValue = GetValueOfAttributeAsString(serializablePropertyContext); if ( (attributeValue != null) && (attributeValue.Length > 0) ) { // // Emit name="value" attribute // XmlWriter.WriteAttributeString(serializablePropertyContext.Name, attributeValue); } } ////// Converts the Value of the Attribute to a String by calling into /// the appropriate type converters. /// /// /// The property that is to be serialized as an attribute at this time. /// private String GetValueOfAttributeAsString( SerializablePropertyContext serializablePropertyContext ) { if(serializablePropertyContext == null) { throw new ArgumentNullException("serializablePropertyContext"); } String valueAsString = null; Object targetObjectContainingProperty = serializablePropertyContext.TargetObject; Object propertyValue = serializablePropertyContext.Value; if(propertyValue != null) { TypeConverter typeConverter = serializablePropertyContext.TypeConverter; valueAsString = typeConverter.ConvertToInvariantString(new XpsTokenContext(SerializationManager, serializablePropertyContext), propertyValue); if (typeof(Type).IsInstanceOfType(propertyValue)) { int index = valueAsString.LastIndexOf('.'); if (index > 0) { valueAsString = valueAsString.Substring(index + 1); } valueAsString = XpsSerializationManager.TypeOfString + valueAsString + "}"; } } else { valueAsString = XpsSerializationManager.NullString; } return valueAsString; } #endregion Internal Methods #region Public Properties ////// Queries / Set the XmlWriter for a FixedPage. /// public override XmlWriter XmlWriter { get { if(base.XmlWriter == null) { base.XmlWriter = SerializationManager.AcquireXmlWriter(typeof(FixedPage)); } return base.XmlWriter; } set { base.XmlWriter = null; SerializationManager.ReleaseXmlWriter(typeof(FixedPage)); } } #endregion Public Properties #region Private Methods private bool SerializePageAsVisual( Visual fixedPageAsVisual ) { bool needEndVisual = false; ReachVisualSerializer serializer = new ReachVisualSerializer(SerializationManager); if(serializer!=null) { needEndVisual = serializer.SerializeDisguisedVisual(fixedPageAsVisual); } else { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_NoSerializer)); } return needEndVisual; } #endregion Private Methods }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
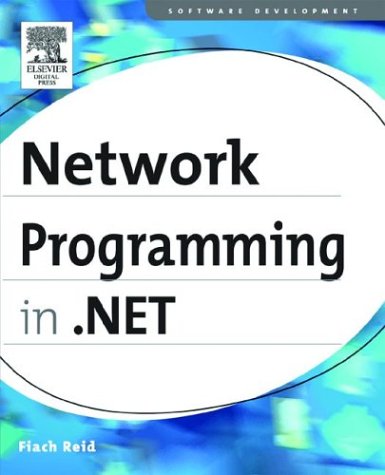
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartMovingEventArgs.cs
- ToolTipService.cs
- CustomCategoryAttribute.cs
- EditorPartCollection.cs
- XamlStyleSerializer.cs
- ArraySortHelper.cs
- DoubleIndependentAnimationStorage.cs
- Hex.cs
- BitmapScalingModeValidation.cs
- IFormattable.cs
- JulianCalendar.cs
- XmlArrayAttribute.cs
- DrawingAttributeSerializer.cs
- XhtmlConformanceSection.cs
- Size.cs
- SmtpNegotiateAuthenticationModule.cs
- InvokePattern.cs
- BorderGapMaskConverter.cs
- ConfigXmlElement.cs
- CopyOnWriteList.cs
- LinkedResourceCollection.cs
- SystemFonts.cs
- QuaternionAnimationBase.cs
- Util.cs
- QualifiedCellIdBoolean.cs
- TextBoxAutoCompleteSourceConverter.cs
- CacheEntry.cs
- XhtmlConformanceSection.cs
- DataGridLinkButton.cs
- DynamicPropertyHolder.cs
- BasicHttpSecurityElement.cs
- TraceContextRecord.cs
- Ray3DHitTestResult.cs
- DataControlFieldTypeEditor.cs
- OperatorExpressions.cs
- ArgumentsParser.cs
- DataGridViewColumnCollection.cs
- Visitors.cs
- AnnotationStore.cs
- Byte.cs
- Trace.cs
- XmlUtilWriter.cs
- ParameterCollection.cs
- FontNamesConverter.cs
- DrawingContextWalker.cs
- TemplateBindingExtension.cs
- CatalogZone.cs
- FileDialog_Vista.cs
- WebPartManagerInternals.cs
- ObjectDataSourceWizardForm.cs
- ScrollBar.cs
- TypedElement.cs
- MarkupWriter.cs
- TextBoxView.cs
- PrePrepareMethodAttribute.cs
- Scalars.cs
- ScriptReferenceBase.cs
- Connection.cs
- PolyBezierSegment.cs
- RtfControls.cs
- PolicyDesigner.cs
- OperationContext.cs
- COSERVERINFO.cs
- XmlCharacterData.cs
- SafeEventLogWriteHandle.cs
- DocumentPageView.cs
- DeferredBinaryDeserializerExtension.cs
- _LoggingObject.cs
- ShapeTypeface.cs
- nulltextnavigator.cs
- TargetControlTypeCache.cs
- AccessedThroughPropertyAttribute.cs
- ViewSimplifier.cs
- TemplateModeChangedEventArgs.cs
- BitmapPalette.cs
- NamespaceEmitter.cs
- UnsafeCollabNativeMethods.cs
- PolyQuadraticBezierSegment.cs
- ContextMenuStrip.cs
- CustomAttributeSerializer.cs
- BuildProvider.cs
- Rfc2898DeriveBytes.cs
- HotSpotCollection.cs
- BaseValidator.cs
- WebPartTransformerAttribute.cs
- LifetimeServices.cs
- SudsParser.cs
- CustomWebEventKey.cs
- WorkflowViewElement.cs
- VsPropertyGrid.cs
- ConvertersCollection.cs
- DbConnectionPool.cs
- ErrorActivity.cs
- RegexMatch.cs
- HttpFileCollection.cs
- GridViewEditEventArgs.cs
- AssociationType.cs
- TemplateParser.cs
- SQLDecimalStorage.cs
- Span.cs