Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / Util / DictionaryCustomTypeDescriptor.cs / 1305376 / DictionaryCustomTypeDescriptor.cs
namespace System.Web.DynamicData.Util { using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; internal class DictionaryCustomTypeDescriptor : CustomTypeDescriptor { private IDictionary_values; private PropertyDescriptorCollection _properties; public DictionaryCustomTypeDescriptor(IDictionary values) { if (values == null) { throw new ArgumentNullException("values"); } _values = values; } public object GetValue(string name) { object value; if (_values.TryGetValue(name, out value)) { return value; } return null; } public override PropertyDescriptorCollection GetProperties() { if (_properties == null) { var dictionaryProps = _values.Keys.Select(propName => new DictionaryPropertyDescriptor(propName)); _properties = new PropertyDescriptorCollection(dictionaryProps.ToArray()); } return _properties; } } internal class DictionaryPropertyDescriptor : PropertyDescriptor { public DictionaryPropertyDescriptor(string name) : base(name, null /* attrs */) { } public override bool CanResetValue(object component) { throw new NotSupportedException(); } public override Type ComponentType { get { throw new NotSupportedException(); } } public override object GetValue(object component) { // Try to cast the component to a DictionaryCustomTypeDescriptor and get the value in the dictonary // that corresponds to this property DictionaryCustomTypeDescriptor typeDescriptor = component as DictionaryCustomTypeDescriptor; if (typeDescriptor == null) { return null; } return typeDescriptor.GetValue(Name); } public override bool IsReadOnly { get { throw new NotSupportedException(); } } public override Type PropertyType { get { throw new NotSupportedException(); } } public override void ResetValue(object component) { throw new NotSupportedException(); } public override void SetValue(object component, object value) { throw new NotSupportedException(); } public override bool ShouldSerializeValue(object component) { throw new NotSupportedException(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
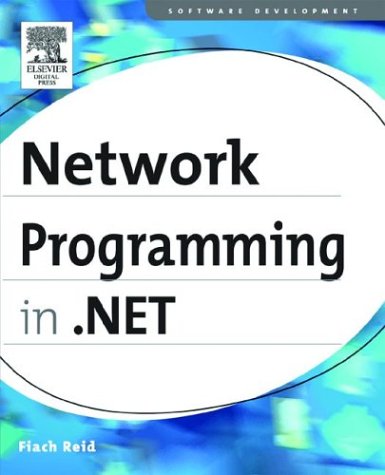
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaAnnotated.cs
- SrgsElementList.cs
- List.cs
- MatrixCamera.cs
- ProviderUtil.cs
- PackagingUtilities.cs
- MarkupCompilePass2.cs
- DbXmlEnabledProviderManifest.cs
- ExportOptions.cs
- QilValidationVisitor.cs
- PropertyInformation.cs
- AppModelKnownContentFactory.cs
- OutputScopeManager.cs
- ProviderUtil.cs
- SmtpClient.cs
- ExceptionUtil.cs
- BinaryObjectReader.cs
- CodeSnippetStatement.cs
- StagingAreaInputItem.cs
- FontCacheUtil.cs
- TextDecorationCollection.cs
- DataSet.cs
- ManualResetEvent.cs
- TypeAccessException.cs
- DocumentViewerConstants.cs
- TextTreeObjectNode.cs
- BaseParaClient.cs
- PersonalizationAdministration.cs
- StaticContext.cs
- ToolStripRenderEventArgs.cs
- ComAwareEventInfo.cs
- MatrixTransform3D.cs
- TagPrefixInfo.cs
- InfiniteIntConverter.cs
- AppDomainShutdownMonitor.cs
- CheckBoxBaseAdapter.cs
- IdentityManager.cs
- WMIInterop.cs
- ToolStripButton.cs
- CurrencyWrapper.cs
- KeyNameIdentifierClause.cs
- ScriptManager.cs
- CheckoutException.cs
- TextBoxLine.cs
- EditorBrowsableAttribute.cs
- DurableEnlistmentState.cs
- SecureConversationDriver.cs
- IWorkflowDebuggerService.cs
- ExpandCollapseProviderWrapper.cs
- DiagnosticTrace.cs
- CompatibleComparer.cs
- JobCollate.cs
- securestring.cs
- CultureMapper.cs
- cookieexception.cs
- SchemaElementDecl.cs
- EventListener.cs
- RawStylusInputCustomDataList.cs
- SiteMapNodeItemEventArgs.cs
- LoginDesigner.cs
- ScriptComponentDescriptor.cs
- SqlNotificationRequest.cs
- ObjectConverter.cs
- Durable.cs
- KeyedCollection.cs
- EllipticalNodeOperations.cs
- DocumentGrid.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- SkipStoryboardToFill.cs
- PersonalizationProviderCollection.cs
- SecurityRuntime.cs
- Sorting.cs
- AtomParser.cs
- HotSpotCollection.cs
- PersonalizableAttribute.cs
- ServiceEndpointCollection.cs
- NodeInfo.cs
- PermissionSetTriple.cs
- RadialGradientBrush.cs
- x509utils.cs
- FullTextBreakpoint.cs
- ToolStripPanelCell.cs
- X509WindowsSecurityToken.cs
- ManualWorkflowSchedulerService.cs
- DataChangedEventManager.cs
- TextureBrush.cs
- InvalidComObjectException.cs
- SafeRightsManagementPubHandle.cs
- AccessDataSource.cs
- WebPartMinimizeVerb.cs
- RegionIterator.cs
- RuntimeIdentifierPropertyAttribute.cs
- Models.cs
- FormsAuthenticationEventArgs.cs
- PerspectiveCamera.cs
- EncoderParameter.cs
- DesignerTransaction.cs
- SafeEventLogWriteHandle.cs
- ToolTip.cs
- BinaryReader.cs