Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / textformatting / CharacterString.cs / 1 / CharacterString.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2003 // // File: CharacterBufferRange.cs // // Contents: A range of character buffer // // Spec: [....]/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 2-5-2004 [....] ([....]) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Security; using MS.Internal; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { ////// A string of characters /// public struct CharacterBufferRange : IEquatable{ private CharacterBufferReference _charBufferRef; private int _length; #region Constructor /// /// Construct character buffer reference from character array /// /// character array /// character buffer offset to the first character /// character length public CharacterBufferRange( char[] characterArray, int offsetToFirstChar, int characterLength ) : this( new CharacterBufferReference(characterArray, offsetToFirstChar), characterLength ) {} ////// Construct character buffer reference from string /// /// character string /// character buffer offset to the first character /// character length public CharacterBufferRange( string characterString, int offsetToFirstChar, int characterLength ) : this( new CharacterBufferReference(characterString, offsetToFirstChar), characterLength ) {} ////// Construct character buffer reference from unsafe character string /// /// pointer to character string /// character length ////// Critical: this stores a pointer to an unmanaged string of characters /// PublicOK: The caller needs unmanaged code permission in order to pass unsafe pointers to us. /// [SecurityCritical] [CLSCompliant(false)] public unsafe CharacterBufferRange( char* unsafeCharacterString, int characterLength ) : this( new CharacterBufferReference(unsafeCharacterString, characterLength), characterLength ) {} ////// Construct a character string from character buffer reference /// /// character buffer reference /// number of characters internal CharacterBufferRange( CharacterBufferReference characterBufferReference, int characterLength ) { if (characterLength < 0) { throw new ArgumentOutOfRangeException("characterLength", SR.Get(SRID.ParameterCannotBeNegative)); } int maxLength = (characterBufferReference.CharacterBuffer != null) ? characterBufferReference.CharacterBuffer.Count - characterBufferReference.OffsetToFirstChar : 0; if (characterLength > maxLength) { throw new ArgumentOutOfRangeException("characterLength", SR.Get(SRID.ParameterCannotBeGreaterThan, maxLength)); } _charBufferRef = characterBufferReference; _length = characterLength; } ////// Construct a character string from part of another character string /// internal CharacterBufferRange( CharacterBufferRange characterBufferRange, int offsetToFirstChar, int characterLength ) : this ( characterBufferRange.CharacterBuffer, characterBufferRange.OffsetToFirstChar + offsetToFirstChar, characterLength ) {} ////// Construct a character string object from string /// internal CharacterBufferRange( string charString ) : this( new StringCharacterBuffer(charString), 0, charString.Length ) {} ////// Construct character buffer from memory buffer /// internal CharacterBufferRange( CharacterBuffer charBuffer, int offsetToFirstChar, int characterLength ) : this( new CharacterBufferReference(charBuffer, offsetToFirstChar), characterLength ) {} ////// Construct a character string object by extracting text info from text run /// internal CharacterBufferRange(TextRun textRun) { _charBufferRef = textRun.CharacterBufferReference; _length = textRun.Length; } #endregion ////// Compute hash code /// public override int GetHashCode() { return _charBufferRef.GetHashCode() ^ _length; } ////// Test equality with the input object /// /// The object to test public override bool Equals(object obj) { if (obj is CharacterBufferRange) { return Equals((CharacterBufferRange)obj); } return false; } ////// Test equality with the input CharacterBufferRange /// /// The CharacterBufferRange value to test public bool Equals(CharacterBufferRange value) { return _charBufferRef.Equals(value._charBufferRef) && _length == value._length; } ////// Compare two CharacterBufferRange for equality /// /// left operand /// right operand ///whether or not two operands are equal public static bool operator == ( CharacterBufferRange left, CharacterBufferRange right ) { return left.Equals(right); } ////// Compare two CharacterBufferRange for inequality /// /// left operand /// right operand ///whether or not two operands are equal public static bool operator != ( CharacterBufferRange left, CharacterBufferRange right ) { return !(left == right); } ////// reference to the character buffer of a string /// public CharacterBufferReference CharacterBufferReference { get { return _charBufferRef; } } ////// number of characters in text source character store /// public int Length { get { return _length; } } ////// Getting an empty character string /// public static CharacterBufferRange Empty { get { return new CharacterBufferRange(); } } ////// Indicate whether the character string object contains no information /// internal bool IsEmpty { get { return _charBufferRef.CharacterBuffer == null || _length <= 0; } } ////// character memory buffer /// internal CharacterBuffer CharacterBuffer { get { return _charBufferRef.CharacterBuffer; } } ////// character offset relative to the beginning of buffer to /// the first character of the run. /// internal int OffsetToFirstChar { get { return _charBufferRef.OffsetToFirstChar; } } ////// Return a character from the range, index is relative to the beginning of the range /// internal char this[int index] { get { Invariant.Assert(index >= 0 && index < _length); return _charBufferRef.CharacterBuffer[_charBufferRef.OffsetToFirstChar + index]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
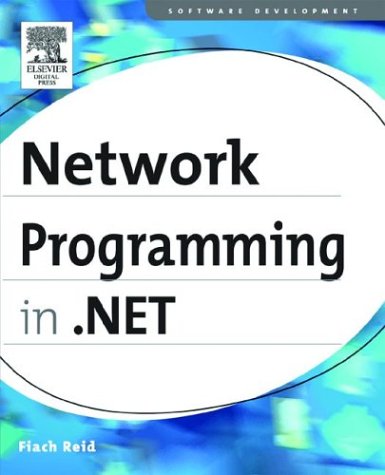
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RecordBuilder.cs
- DesignerActionVerbItem.cs
- PolygonHotSpot.cs
- METAHEADER.cs
- ServiceKnownTypeAttribute.cs
- AttributeAction.cs
- WriteTimeStream.cs
- Site.cs
- RpcAsyncResult.cs
- Highlights.cs
- ApplicationGesture.cs
- Stack.cs
- XPathMultyIterator.cs
- CompositeFontParser.cs
- Int32CollectionConverter.cs
- DataGridRowsPresenter.cs
- ToolStripDropDownClosingEventArgs.cs
- CapiSymmetricAlgorithm.cs
- AssemblyLoader.cs
- ManipulationDeltaEventArgs.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- Button.cs
- XmlDataImplementation.cs
- sqlcontext.cs
- XmlQueryType.cs
- ZipIOExtraFieldPaddingElement.cs
- ADMembershipUser.cs
- ValidationPropertyAttribute.cs
- BaseValidatorDesigner.cs
- ArrayConverter.cs
- XmlSchemaSet.cs
- WorkflowEnvironment.cs
- WmlPhoneCallAdapter.cs
- InfoCardTraceRecord.cs
- String.cs
- ToolStripSplitStackLayout.cs
- IdnMapping.cs
- ListViewItemEventArgs.cs
- TreeView.cs
- SRGSCompiler.cs
- DesignerActionKeyboardBehavior.cs
- DefaultAssemblyResolver.cs
- UserControl.cs
- AdornerHitTestResult.cs
- XmlNamedNodeMap.cs
- ExtractCollection.cs
- Registration.cs
- SectionXmlInfo.cs
- PagesSection.cs
- TaskbarItemInfo.cs
- CodeExporter.cs
- EndEvent.cs
- StyleSheet.cs
- PersonalizationAdministration.cs
- CustomWebEventKey.cs
- OdbcReferenceCollection.cs
- HttpStreamXmlDictionaryReader.cs
- SqlDataSourceQuery.cs
- xsdvalidator.cs
- NamedPermissionSet.cs
- WebControl.cs
- NullableConverter.cs
- ToolStripRenderer.cs
- SoapSchemaMember.cs
- InternalsVisibleToAttribute.cs
- QueuePathDialog.cs
- StreamGeometry.cs
- VSWCFServiceContractGenerator.cs
- UInt16Storage.cs
- infer.cs
- TreeViewCancelEvent.cs
- RoutingSection.cs
- ListViewDeleteEventArgs.cs
- WmpBitmapDecoder.cs
- Pointer.cs
- InstanceCollisionException.cs
- MethodAccessException.cs
- XmlConvert.cs
- KeyManager.cs
- DataTablePropertyDescriptor.cs
- Binding.cs
- GeneralTransform3DGroup.cs
- DbMetaDataCollectionNames.cs
- EditorResources.cs
- HttpVersion.cs
- GridViewRow.cs
- FileSecurity.cs
- VisualTreeHelper.cs
- OptimalTextSource.cs
- AssemblyInfo.cs
- ToolStripDropTargetManager.cs
- JavaScriptString.cs
- TraceSection.cs
- TableStyle.cs
- MimeParameter.cs
- DBSqlParserTable.cs
- CompilationSection.cs
- SqlError.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- AuthenticateEventArgs.cs