Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / TypeSystem / AssemblyLoader.cs / 1305376 / AssemblyLoader.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.Collections.Generic; using System.Reflection; using System.ComponentModel.Design; using System.IO; internal class AssemblyLoader { private Assembly assembly = null; private AssemblyName assemblyName = null; private TypeProvider typeProvider = null; private bool isLocalAssembly; internal AssemblyLoader(TypeProvider typeProvider, string filePath) { this.isLocalAssembly = false; this.typeProvider = typeProvider; if (File.Exists(filePath)) { AssemblyName asmName = AssemblyName.GetAssemblyName(filePath); if (asmName != null) { // Try loading the assembly using type resolution service first. ITypeResolutionService trs = (ITypeResolutionService)typeProvider.GetService(typeof(ITypeResolutionService)); if (trs != null) { try { this.assembly = trs.GetAssembly(asmName); // if (this.assembly == null && asmName.GetPublicKeyToken() != null && (asmName.GetPublicKeyToken().GetLength(0) == 0) && asmName.GetPublicKey() != null && (asmName.GetPublicKey().GetLength(0) == 0)) { AssemblyName partialName = (AssemblyName)asmName.Clone(); partialName.SetPublicKey(null); partialName.SetPublicKeyToken(null); this.assembly = trs.GetAssembly(partialName); } } catch { // Eat up any exceptions! } } // If type resolution service wasn't available or it failed use Assembly.Load if (this.assembly == null) { try { if (MultiTargetingInfo.MultiTargetingUtilities.IsFrameworkReferenceAssembly(filePath)) { this.assembly = Assembly.Load(asmName.FullName); } else { this.assembly = Assembly.Load(asmName); } } catch { // Eat up any exceptions! } } } // If Assembly.Load also failed, use Assembly.LoadFrom if (this.assembly == null) { this.assembly = Assembly.LoadFrom(filePath); } } else { // TypeProvider will handle this and report the error throw new FileNotFoundException(); } } internal AssemblyLoader(TypeProvider typeProvider, Assembly assembly, bool isLocalAssembly) { this.isLocalAssembly = isLocalAssembly; this.typeProvider = typeProvider; this.assembly = assembly; } internal Type GetType(string typeName) { // if (this.assembly != null) { Type type = null; try { type = this.assembly.GetType(typeName); } catch (ArgumentException) { // we eat these exeptions in our type system } if ((type != null) && (type.IsPublic || type.IsNestedPublic || (this.isLocalAssembly && type.Attributes != TypeAttributes.NestedPrivate))) return type; } return null; } internal Type[] GetTypes() { ListfilteredTypes = new List (); if (this.assembly != null) { // foreach (Type type in this.assembly.GetTypes()) { // if(type.IsPublic || (this.isLocalAssembly && type.Attributes != TypeAttributes.NestedPrivate)) filteredTypes.Add(type); } } return filteredTypes.ToArray(); } // we cache the AssemblyName as Assembly.GetName() is expensive internal AssemblyName AssemblyName { get { if (this.assemblyName == null) this.assemblyName = this.assembly.GetName(true); return this.assemblyName; } } internal Assembly Assembly { get { return this.assembly; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
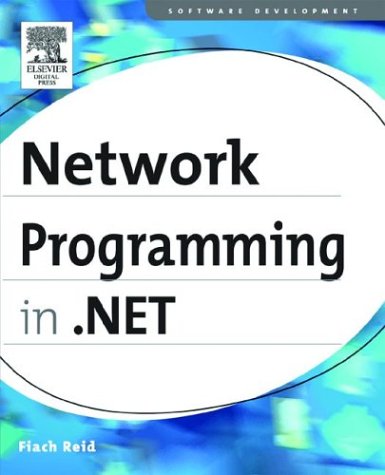
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XhtmlStyleClass.cs
- DeviceSpecificDesigner.cs
- ContractMapping.cs
- KeyboardInputProviderAcquireFocusEventArgs.cs
- CollectionType.cs
- SetStoryboardSpeedRatio.cs
- DataGridDefaultColumnWidthTypeConverter.cs
- EpmTargetPathSegment.cs
- ControlTemplate.cs
- RenderData.cs
- PathParser.cs
- CollectionChangeEventArgs.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- SchemaTypeEmitter.cs
- DesignerSerializationOptionsAttribute.cs
- FixedSOMTableRow.cs
- Overlapped.cs
- ResponseStream.cs
- IriParsingElement.cs
- Msmq.cs
- AccessViolationException.cs
- ReadOnlyNameValueCollection.cs
- AssemblyNameProxy.cs
- MouseGesture.cs
- DataExchangeServiceBinder.cs
- DataSourceView.cs
- SearchExpression.cs
- LicenseContext.cs
- HtmlMeta.cs
- QueryContinueDragEvent.cs
- ItemsChangedEventArgs.cs
- ToolStripItemImageRenderEventArgs.cs
- MessageSmuggler.cs
- HttpFormatExtensions.cs
- StreamGeometryContext.cs
- SrgsSemanticInterpretationTag.cs
- TextSelectionHelper.cs
- MenuItemStyle.cs
- TypographyProperties.cs
- SQLInt64Storage.cs
- Matrix3D.cs
- Number.cs
- RelationshipConstraintValidator.cs
- XmlText.cs
- ParseElement.cs
- WebPartPersonalization.cs
- TextSyndicationContentKindHelper.cs
- DesignerAttribute.cs
- Stylus.cs
- PartBasedPackageProperties.cs
- EnterpriseServicesHelper.cs
- ParamArrayAttribute.cs
- ValidatorCompatibilityHelper.cs
- WindowsStatusBar.cs
- CharAnimationUsingKeyFrames.cs
- TextServicesDisplayAttributePropertyRanges.cs
- WorkflowLayouts.cs
- HttpException.cs
- NamedPermissionSet.cs
- ComPlusServiceLoader.cs
- HwndStylusInputProvider.cs
- SignatureDescription.cs
- ToggleProviderWrapper.cs
- SqlExpander.cs
- SiteMapProvider.cs
- ViewDesigner.cs
- EventSetter.cs
- RequestCachePolicy.cs
- DataGridRowHeader.cs
- ProcessModelSection.cs
- DbTransaction.cs
- DataGridDesigner.cs
- FixedSchema.cs
- RefreshInfo.cs
- EllipseGeometry.cs
- ValidatorCollection.cs
- UInt64Converter.cs
- RoutedEventValueSerializer.cs
- SspiNegotiationTokenProviderState.cs
- ContextMarshalException.cs
- ProcessInfo.cs
- Paragraph.cs
- SecUtil.cs
- WindowInteropHelper.cs
- InputLanguageCollection.cs
- ActivityBindForm.cs
- DataGridViewRowsAddedEventArgs.cs
- SettingsPropertyWrongTypeException.cs
- AttachInfo.cs
- ConnectionsZoneAutoFormat.cs
- WebControlAdapter.cs
- TextTreeNode.cs
- CaseKeyBox.xaml.cs
- ComponentCommands.cs
- CommandHelpers.cs
- BuildProviderAppliesToAttribute.cs
- SmuggledIUnknown.cs
- DisplayNameAttribute.cs
- WaitHandleCannotBeOpenedException.cs
- cookieexception.cs