Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Data / System / Data / Odbc / OdbcReferenceCollection.cs / 1 / OdbcReferenceCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; namespace System.Data.Odbc { sealed internal class OdbcReferenceCollection : DbReferenceCollection { internal const int Closing = 0; internal const int Recover = 1; internal const int CommandTag = 1; override public void Add(object value, int tag) { base.AddItem(value, tag); } override protected bool NotifyItem(int message, int tag, object value) { switch (message) { case Recover: if (CommandTag == tag) { ((OdbcCommand) value).RecoverFromConnection(); } else { Debug.Assert(false, "shouldn't be here"); } break; case Closing: if (CommandTag == tag) { ((OdbcCommand) value).CloseFromConnection(); } else { Debug.Assert(false, "shouldn't be here"); } break; default: Debug.Assert(false, "shouldn't be here"); break; } return false; // remove it from the collection } override public void Remove(object value) { base.RemoveItem(value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.Data; using System.Data.Common; using System.Data.ProviderBase; using System.Diagnostics; namespace System.Data.Odbc { sealed internal class OdbcReferenceCollection : DbReferenceCollection { internal const int Closing = 0; internal const int Recover = 1; internal const int CommandTag = 1; override public void Add(object value, int tag) { base.AddItem(value, tag); } override protected bool NotifyItem(int message, int tag, object value) { switch (message) { case Recover: if (CommandTag == tag) { ((OdbcCommand) value).RecoverFromConnection(); } else { Debug.Assert(false, "shouldn't be here"); } break; case Closing: if (CommandTag == tag) { ((OdbcCommand) value).CloseFromConnection(); } else { Debug.Assert(false, "shouldn't be here"); } break; default: Debug.Assert(false, "shouldn't be here"); break; } return false; // remove it from the collection } override public void Remove(object value) { base.RemoveItem(value); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
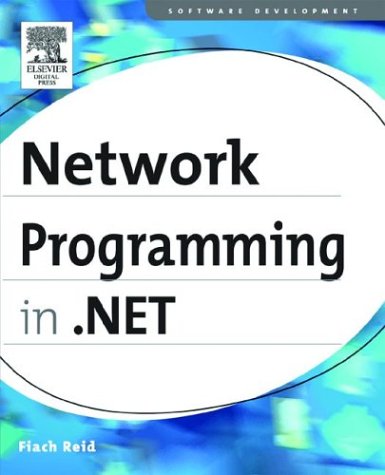
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PriorityQueue.cs
- GetCryptoTransformRequest.cs
- DataControlExtensions.cs
- Odbc32.cs
- SecurityValidationBehavior.cs
- DataBinding.cs
- TextSimpleMarkerProperties.cs
- SqlDataSource.cs
- WindowsAltTab.cs
- RotateTransform.cs
- Html32TextWriter.cs
- StylusPointPropertyId.cs
- ClipboardProcessor.cs
- DataBoundLiteralControl.cs
- HttpEncoderUtility.cs
- ServiceChannel.cs
- SharedPerformanceCounter.cs
- ShapingWorkspace.cs
- COM2PictureConverter.cs
- Item.cs
- NamespaceList.cs
- BaseInfoTable.cs
- DateTimeConverter.cs
- SessionStateModule.cs
- CriticalHandle.cs
- BrowserCapabilitiesCodeGenerator.cs
- ComplexPropertyEntry.cs
- PictureBox.cs
- IImplicitResourceProvider.cs
- ItemsControl.cs
- BackgroundFormatInfo.cs
- DistinctQueryOperator.cs
- RecognizeCompletedEventArgs.cs
- ClientConfigurationHost.cs
- LocationReferenceValue.cs
- DataGridViewColumnHeaderCell.cs
- DataGridAddNewRow.cs
- DBCommandBuilder.cs
- Debug.cs
- GotoExpression.cs
- TextCompositionEventArgs.cs
- FunctionImportMapping.cs
- UseManagedPresentationElement.cs
- MenuItemStyleCollection.cs
- IndexerNameAttribute.cs
- XMLUtil.cs
- ThreadNeutralSemaphore.cs
- StyleTypedPropertyAttribute.cs
- CodeDefaultValueExpression.cs
- StringCollection.cs
- PersistChildrenAttribute.cs
- DateTimeSerializationSection.cs
- OperationDescription.cs
- WebPartExportVerb.cs
- XmlEncoding.cs
- FontStyleConverter.cs
- DoubleLinkListEnumerator.cs
- HostExecutionContextManager.cs
- Invariant.cs
- DataComponentMethodGenerator.cs
- TraceContextRecord.cs
- IntegerValidatorAttribute.cs
- Bits.cs
- SegmentInfo.cs
- WebPartEditorOkVerb.cs
- ListControlActionList.cs
- ApplicationHost.cs
- TemplatingOptionsDialog.cs
- cache.cs
- UserControl.cs
- DropDownHolder.cs
- XmlStringTable.cs
- DesignerTransaction.cs
- StylusPointProperty.cs
- Cursor.cs
- OracleDateTime.cs
- XmlBindingWorker.cs
- SectionInformation.cs
- XPathDocumentIterator.cs
- HtmlTableRowCollection.cs
- Image.cs
- BoundField.cs
- Cursors.cs
- ExtensionWindow.cs
- XsdCachingReader.cs
- SrgsRulesCollection.cs
- Lease.cs
- SecurityTokenAuthenticator.cs
- EdmError.cs
- XamlReader.cs
- DockingAttribute.cs
- CommandLibraryHelper.cs
- ObsoleteAttribute.cs
- Span.cs
- BrowserInteropHelper.cs
- X509CertificateCollection.cs
- ComEventsHelper.cs
- XmlMapping.cs
- control.ime.cs
- ViewStateModeByIdAttribute.cs