Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GetCryptoTransformRequest.cs / 1 / GetCryptoTransformRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.Collections; using System.Diagnostics; using System.Security.Principal; using System.Threading; //ManualResetEvent using System.ComponentModel; //Win32Exception using System.IO; //Stream using System.Security.Cryptography; using System.Text; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Wraps a request to get a remoted ICryptoTransform interface based on a SymmetricCryptoSession. // class GetCryptoTransformRequest : ClientRequest { // // The cryptosession id we are attaching to. // int m_cryptoSession; // // The cipher mode being requested. // CipherMode m_mode; // // The padding mode being requested. // PaddingMode m_padding; // // The feedback size being requested // int m_feedbackSize; // // Is Encryption or Decryption being requested. // SymmetricCryptoSession.Direction m_direction; // // The intialization vector. // byte[] m_iv; // // The returned transform session. // TransformCryptoSession m_transformSession; // // Sumamry: // Construct a GetCryptoTransformRequest object // // Arguments: // callingProcess - The process in which the caller originated. // callingIdentity - The WindowsIdentity of the caller // rpcHandle - The handle of the native RPC request // inArgs - The stream to read input data from // outArgs - The stream to write output data to // public GetCryptoTransformRequest( Process callingProcess, WindowsIdentity callingIdentity, IntPtr rpcHandle, Stream inArgs, Stream outArgs ) : base( callingProcess, callingIdentity, rpcHandle, inArgs, outArgs ) { IDT.TraceDebug( "Intiating a GetCryptoTransform request" ); } protected override void OnMarshalInArgs() { IDT.DebugAssert( null != InArgs, "null inargs" ); BinaryReader reader = new InfoCardBinaryReader( InArgs, Encoding.Unicode ); m_cryptoSession = reader.ReadInt32(); m_mode = (CipherMode)reader.ReadInt32(); m_padding = (PaddingMode)reader.ReadInt32(); m_feedbackSize = reader.ReadInt32(); m_direction = (SymmetricCryptoSession.Direction)reader.ReadInt32(); m_iv = reader.ReadBytes( reader.ReadInt32() ); IDT.ThrowInvalidArgumentConditional( 0 == m_feedbackSize, "feedbackSize" ); } // // Summary: // Attach to the appropriate cryptosession and get a CryptoTransform // protected override void OnProcess() { SymmetricCryptoSession session = ( SymmetricCryptoSession )CryptoSession.Find( m_cryptoSession, CallerPid, RequestorIdentity.User ); m_transformSession = session.GetCryptoTransform( m_mode, m_padding, m_feedbackSize, m_direction, m_iv ); } // // Summary: // Return the TransformCryptoSession // protected override void OnMarshalOutArgs() { IDT.DebugAssert( null != OutArgs, "Null out args" ); BinaryWriter writer = new BinaryWriter( OutArgs, Encoding.Unicode ); IDT.DebugAssert( null != m_transformSession, "unexpected null outgoing transfromSession" ); m_transformSession.Write( writer ); writer.Flush(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
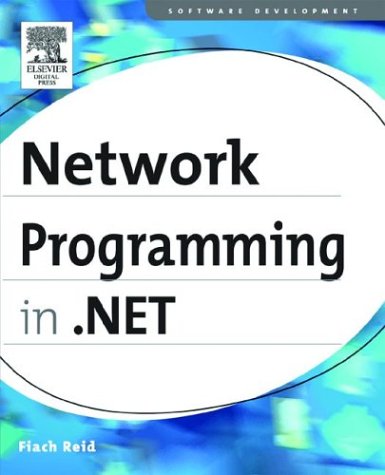
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- xmlfixedPageInfo.cs
- SchemaNames.cs
- MessageQueuePermissionAttribute.cs
- SessionEndingEventArgs.cs
- SqlParameter.cs
- XmlAnyElementAttribute.cs
- SqlAggregateChecker.cs
- StatusBar.cs
- EdmConstants.cs
- Vector3dCollection.cs
- SocketPermission.cs
- CalendarData.cs
- UrlPath.cs
- OdbcConnectionStringbuilder.cs
- InternalTypeHelper.cs
- EncodingDataItem.cs
- ObjRef.cs
- RecordsAffectedEventArgs.cs
- ExceptionUtility.cs
- TdsParserStaticMethods.cs
- EntityDataSourceDesigner.cs
- Matrix3DStack.cs
- OdbcConnectionOpen.cs
- TimeSpanValidatorAttribute.cs
- InkCanvasAutomationPeer.cs
- DateTimeOffsetAdapter.cs
- ObsoleteAttribute.cs
- DataKey.cs
- SqlCommandSet.cs
- ClientRoleProvider.cs
- IncrementalCompileAnalyzer.cs
- CustomCredentialPolicy.cs
- DBConnection.cs
- Size3D.cs
- SimpleTextLine.cs
- DeflateStream.cs
- input.cs
- UserControlAutomationPeer.cs
- Setter.cs
- AsymmetricSignatureDeformatter.cs
- Membership.cs
- SpAudioStreamWrapper.cs
- TakeQueryOptionExpression.cs
- RSAOAEPKeyExchangeDeformatter.cs
- FtpCachePolicyElement.cs
- DigitShape.cs
- SymbolType.cs
- EtwTrace.cs
- DrawingAttributeSerializer.cs
- LayoutEngine.cs
- JumpList.cs
- ComPlusInstanceProvider.cs
- SkipQueryOptionExpression.cs
- DataGridColumn.cs
- ShowExpandedMultiValueConverter.cs
- PrintingPermission.cs
- SqlBuffer.cs
- RowsCopiedEventArgs.cs
- ConfigurationFileMap.cs
- AnnotationService.cs
- PageVisual.cs
- XmlComment.cs
- RectAnimationBase.cs
- KnownTypesHelper.cs
- RangeValidator.cs
- UInt64.cs
- Label.cs
- StoreItemCollection.Loader.cs
- CursorConverter.cs
- PreProcessInputEventArgs.cs
- InternalRelationshipCollection.cs
- ResourceCategoryAttribute.cs
- BindableTemplateBuilder.cs
- TreePrinter.cs
- DataGridViewLayoutData.cs
- ApplicationException.cs
- PageStatePersister.cs
- SqlDataSourceConfigureSelectPanel.cs
- WizardPanelChangingEventArgs.cs
- OAVariantLib.cs
- HtmlCalendarAdapter.cs
- AssemblyBuilder.cs
- Identifier.cs
- ComponentDispatcher.cs
- DecoderFallback.cs
- StorageMappingItemLoader.cs
- ConnectionPool.cs
- Ray3DHitTestResult.cs
- IImplicitResourceProvider.cs
- EntitySet.cs
- DbConnectionHelper.cs
- FunctionMappingTranslator.cs
- DeviceContexts.cs
- ListItemCollection.cs
- ProxyRpc.cs
- ListParaClient.cs
- COM2TypeInfoProcessor.cs
- NetTcpSecurityElement.cs
- CommunicationObject.cs
- WeakEventTable.cs