Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / Common / DBConnection.cs / 1305376 / DBConnection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.ComponentModel; using System.Data; public abstract class DbConnection : Component, IDbConnection { // V1.2.3300 private StateChangeEventHandler _stateChangeEventHandler; protected DbConnection() : base() { } [ DefaultValue(""), #pragma warning disable 618 // ignore obsolete warning about RecommendedAsConfigurable to use SettingsBindableAttribute RecommendedAsConfigurable(true), #pragma warning restore 618 SettingsBindableAttribute(true), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ] abstract public string ConnectionString { get; set; } [ ResCategoryAttribute(Res.DataCategory_Data), ] virtual public int ConnectionTimeout { get { return ADP.DefaultConnectionTimeout; } } [ ResCategoryAttribute(Res.DataCategory_Data), ] abstract public string Database { get; } [ ResCategoryAttribute(Res.DataCategory_Data), ] abstract public string DataSource { // NOTE: if you plan on allowing the data source to be changed, you // should implement a ChangeDataSource method, in keeping with // the ChangeDatabase method paradigm. get; } ////// The associated provider factory for derived class. /// virtual protected DbProviderFactory DbProviderFactory { get { return null; } } internal DbProviderFactory ProviderFactory { get { return DbProviderFactory; } } [ Browsable(false), ] abstract public string ServerVersion { get; } [ Browsable(false), ResDescriptionAttribute(Res.DbConnection_State), ] abstract public ConnectionState State { get; } [ ResCategoryAttribute(Res.DataCategory_StateChange), ResDescriptionAttribute(Res.DbConnection_StateChange), ] virtual public event StateChangeEventHandler StateChange { add { _stateChangeEventHandler += value; } remove { _stateChangeEventHandler -= value; } } abstract protected DbTransaction BeginDbTransaction(IsolationLevel isolationLevel); public DbTransaction BeginTransaction() { return BeginDbTransaction(IsolationLevel.Unspecified); } public DbTransaction BeginTransaction(IsolationLevel isolationLevel) { return BeginDbTransaction(isolationLevel); } IDbTransaction IDbConnection.BeginTransaction() { return BeginDbTransaction(IsolationLevel.Unspecified); } IDbTransaction IDbConnection.BeginTransaction(IsolationLevel isolationLevel) { return BeginDbTransaction(isolationLevel); } abstract public void Close(); abstract public void ChangeDatabase(string databaseName); public DbCommand CreateCommand() { return CreateDbCommand(); } IDbCommand IDbConnection.CreateCommand() { return CreateDbCommand(); } abstract protected DbCommand CreateDbCommand(); virtual public void EnlistTransaction(System.Transactions.Transaction transaction) { // NOTE: This is virtual because not all providers may choose to support // distributed transactions. throw ADP.NotSupported(); } // these need to be here so that GetSchema is visible when programming to a dbConnection object. // they are overridden by the real implementations in DbConnectionBase virtual public DataTable GetSchema() { throw ADP.NotSupported(); } virtual public DataTable GetSchema(string collectionName) { throw ADP.NotSupported(); } virtual public DataTable GetSchema(string collectionName, string[] restrictionValues ) { throw ADP.NotSupported(); } protected virtual void OnStateChange(StateChangeEventArgs stateChange) { StateChangeEventHandler handler = _stateChangeEventHandler; if (null != handler) { handler(this, stateChange); } } abstract public void Open(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.Common { using System; using System.ComponentModel; using System.Data; public abstract class DbConnection : Component, IDbConnection { // V1.2.3300 private StateChangeEventHandler _stateChangeEventHandler; protected DbConnection() : base() { } [ DefaultValue(""), #pragma warning disable 618 // ignore obsolete warning about RecommendedAsConfigurable to use SettingsBindableAttribute RecommendedAsConfigurable(true), #pragma warning restore 618 SettingsBindableAttribute(true), RefreshProperties(RefreshProperties.All), ResCategoryAttribute(Res.DataCategory_Data), ] abstract public string ConnectionString { get; set; } [ ResCategoryAttribute(Res.DataCategory_Data), ] virtual public int ConnectionTimeout { get { return ADP.DefaultConnectionTimeout; } } [ ResCategoryAttribute(Res.DataCategory_Data), ] abstract public string Database { get; } [ ResCategoryAttribute(Res.DataCategory_Data), ] abstract public string DataSource { // NOTE: if you plan on allowing the data source to be changed, you // should implement a ChangeDataSource method, in keeping with // the ChangeDatabase method paradigm. get; } ////// The associated provider factory for derived class. /// virtual protected DbProviderFactory DbProviderFactory { get { return null; } } internal DbProviderFactory ProviderFactory { get { return DbProviderFactory; } } [ Browsable(false), ] abstract public string ServerVersion { get; } [ Browsable(false), ResDescriptionAttribute(Res.DbConnection_State), ] abstract public ConnectionState State { get; } [ ResCategoryAttribute(Res.DataCategory_StateChange), ResDescriptionAttribute(Res.DbConnection_StateChange), ] virtual public event StateChangeEventHandler StateChange { add { _stateChangeEventHandler += value; } remove { _stateChangeEventHandler -= value; } } abstract protected DbTransaction BeginDbTransaction(IsolationLevel isolationLevel); public DbTransaction BeginTransaction() { return BeginDbTransaction(IsolationLevel.Unspecified); } public DbTransaction BeginTransaction(IsolationLevel isolationLevel) { return BeginDbTransaction(isolationLevel); } IDbTransaction IDbConnection.BeginTransaction() { return BeginDbTransaction(IsolationLevel.Unspecified); } IDbTransaction IDbConnection.BeginTransaction(IsolationLevel isolationLevel) { return BeginDbTransaction(isolationLevel); } abstract public void Close(); abstract public void ChangeDatabase(string databaseName); public DbCommand CreateCommand() { return CreateDbCommand(); } IDbCommand IDbConnection.CreateCommand() { return CreateDbCommand(); } abstract protected DbCommand CreateDbCommand(); virtual public void EnlistTransaction(System.Transactions.Transaction transaction) { // NOTE: This is virtual because not all providers may choose to support // distributed transactions. throw ADP.NotSupported(); } // these need to be here so that GetSchema is visible when programming to a dbConnection object. // they are overridden by the real implementations in DbConnectionBase virtual public DataTable GetSchema() { throw ADP.NotSupported(); } virtual public DataTable GetSchema(string collectionName) { throw ADP.NotSupported(); } virtual public DataTable GetSchema(string collectionName, string[] restrictionValues ) { throw ADP.NotSupported(); } protected virtual void OnStateChange(StateChangeEventArgs stateChange) { StateChangeEventHandler handler = _stateChangeEventHandler; if (null != handler) { handler(this, stateChange); } } abstract public void Open(); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
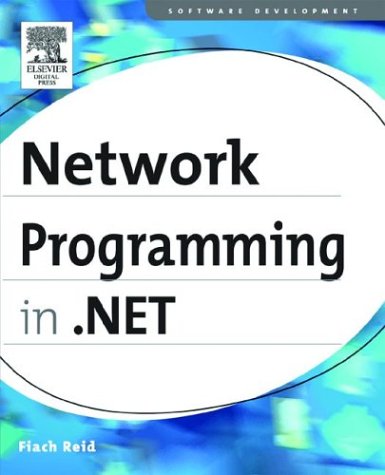
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ConditionalAttribute.cs
- ScrollViewerAutomationPeer.cs
- SqlUdtInfo.cs
- XPathChildIterator.cs
- DataGridViewRowsRemovedEventArgs.cs
- EventDescriptorCollection.cs
- ResourceSet.cs
- WebHttpBindingCollectionElement.cs
- ModuleConfigurationInfo.cs
- SelectionWordBreaker.cs
- VariantWrapper.cs
- RootProfilePropertySettingsCollection.cs
- counter.cs
- MethodBuilderInstantiation.cs
- Permission.cs
- DataGridViewComboBoxEditingControl.cs
- ReachIDocumentPaginatorSerializer.cs
- ViewGenerator.cs
- PropertyManager.cs
- EventBuilder.cs
- SqlStream.cs
- GenericsInstances.cs
- ConnectionPointCookie.cs
- ApplicationDirectory.cs
- SqlClientWrapperSmiStream.cs
- DelimitedListTraceListener.cs
- SoapSchemaImporter.cs
- BrowserTree.cs
- HTMLTagNameToTypeMapper.cs
- ModelUIElement3D.cs
- Color.cs
- MarkupExtensionParser.cs
- DataPagerFieldCollection.cs
- ReferenceList.cs
- DesignerActionService.cs
- NetSectionGroup.cs
- Bidi.cs
- SchemaNames.cs
- XmlQualifiedNameTest.cs
- Transform.cs
- PrimitiveXmlSerializers.cs
- Transform.cs
- Task.cs
- Win32PrintDialog.cs
- Emitter.cs
- ECDiffieHellmanPublicKey.cs
- ProxyWebPartManager.cs
- MetadataConversionError.cs
- Model3DGroup.cs
- CardSpaceSelector.cs
- xdrvalidator.cs
- RankException.cs
- ListMarkerSourceInfo.cs
- SmiMetaDataProperty.cs
- AppDomain.cs
- GenericAuthenticationEventArgs.cs
- securestring.cs
- MailWriter.cs
- StateMachineExecutionState.cs
- ObjectItemCollection.cs
- ColumnClickEvent.cs
- SymbolMethod.cs
- ScriptHandlerFactory.cs
- ListParaClient.cs
- ConfigViewGenerator.cs
- InputScope.cs
- FragmentNavigationEventArgs.cs
- LinkClickEvent.cs
- XmlAttributeOverrides.cs
- GuidelineSet.cs
- SafeNativeMethods.cs
- TypeForwardedToAttribute.cs
- RangeValueProviderWrapper.cs
- ManagementInstaller.cs
- WindowsAuthenticationModule.cs
- NativeBuffer.cs
- PrintDocument.cs
- LeaseManager.cs
- DNS.cs
- BeginCreateSecurityTokenRequest.cs
- IQueryable.cs
- ContextMenuStripGroup.cs
- Compress.cs
- TraceSection.cs
- CatalogPartChrome.cs
- CustomValidator.cs
- base64Transforms.cs
- DirtyTextRange.cs
- Mapping.cs
- BaseParaClient.cs
- StateDesigner.TransitionInfo.cs
- BamlTreeMap.cs
- FieldToken.cs
- DataServices.cs
- OledbConnectionStringbuilder.cs
- DocumentPaginator.cs
- XmlComment.cs
- LicFileLicenseProvider.cs
- TemplateInstanceAttribute.cs
- WindowsScrollBarBits.cs